Angular JS Interview Questions with Explanation
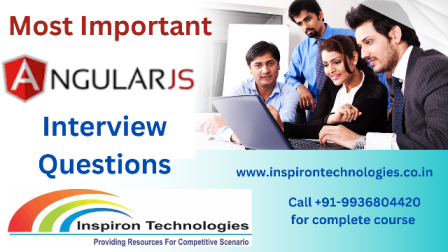
Angular JS Interview Questions with Explanation
1. What is AngularJS?
AngularJS is a JavaScript-based open-source front-end web application framework that is designed to make it easier to build dynamic, single-page applications. It extends HTML with new attributes and constructs to define dynamic views and supports two-way data binding, dependency injection, and modular architecture.
2. What is data binding in AngularJS?
Data binding is a key feature of AngularJS that establishes a connection between the application's data and the UI. It allows you to automatically synchronize data between the model (JavaScript variables) and the view (HTML representation). There are two types of data binding in AngularJS: one-way binding (from the model to the view) and two-way binding (bidirectional binding between the model and the view).
3. What are directives in AngularJS?
Directives are markers on a DOM element that tell AngularJS to attach specific behaviors to that element or transform it in some way. They are one of the core building blocks of AngularJS and can be used to create custom HTML tags, attributes, classes, and comments. AngularJS provides built-in directives such as ng-model, ng-bind, ng-repeat, etc., and also allows you to create custom directives.
4. What is the difference between AngularJS and Angular?
AngularJS (also known as Angular 1.x) and Angular (also known as Angular 2+ or just Angular) are two different frameworks. AngularJS is the older version and is based on JavaScript, while Angular is a complete rewrite of the framework and is based on TypeScript. Angular introduced significant changes, improved performance, and a more modular architecture compared to AngularJS. The syntax and concepts in Angular are quite different from AngularJS.
5. What is dependency injection in AngularJS?
Dependency injection (DI) is a software design pattern used in AngularJS to manage the dependencies between different components of an application. It allows you to declare the dependencies of a component in a separate configuration, and AngularJS takes care of creating and injecting those dependencies when the component is instantiated. DI helps in decoupling components, promoting reusability, and making the code more testable and maintainable.
6. What is a service in AngularJS?
In AngularJS, a service is a reusable singleton object that provides specific functionality and can be injected into different parts of the application. Services are commonly used for sharing data, performing business logic, and interacting with remote servers. AngularJS provides built-in services like $http for making HTTP requests, $timeout for delaying code execution, etc., and allows you to create custom services.
7. What is the AngularJS scope?
The scope in AngularJS is an object that serves as the binding part between the HTML (view) and the JavaScript (controller). It acts as a context for storing and accessing data. The scope allows you to define properties and functions that can be accessed within the HTML template. It enables two-way data binding, as changes made in the scope are automatically reflected in the view and vice versa.
8. What are filters in AngularJS?
Filters in AngularJS are used to format and transform data before displaying it in the view. They can be applied to expressions or binding values using the pipe character (|). AngularJS provides several built-in filters such as uppercase, lowercase, currency, date, filter, etc. You can also create custom filters to meet specific formatting requirements.
9. What is routing in AngularJS?
Routing in AngularJS allows you to navigate between different views or pages without refreshing the entire page. It is achieved by using the ngRoute module, which provides routing capabilities. With routing, you can define routes for different URLs and associate them with specific templates and controllers. When a user navigates to a specific URL, AngularJS loads the corresponding template and controller, updating only the necessary portion of the page.
10. What is the controller in AngularJS?
A controller in AngularJS is a JavaScript function that acts as the intermediary between the view and the model. It is responsible for defining the behavior and business logic of a particular view. Controllers are used to initialize the scope, handle user interactions, process data, and perform other operations. In the HTML template, controllers are associated with specific elements or sections using the ng-controller directive.
11. What is the digest cycle in AngularJS?
The digest cycle in AngularJS is a process that updates the bindings and synchronizes the model and the view. It is responsible for checking if any changes have occurred in the model and updating the corresponding parts of the view. During the digest cycle, AngularJS compares the current value of each watch expression (bindings) with its previous value, and if there is a change, it updates the view. The digest cycle continues until there are no more changes to propagate.
12. What is the use of the $http service in AngularJS?
The $http service in AngularJS is used to make HTTP requests to remote servers and retrieve data. It provides methods like get, post, put, delete, etc., for sending HTTP requests. The $http service returns a promise object that allows you to handle success and error responses asynchronously. It is commonly used to interact with RESTful APIs and retrieve data from a backend server.
These additional questions cover important concepts in AngularJS, including scope, filters, routing, controllers, the digest cycle, and the $http service. Understanding these concepts will help you develop more complex and interactive applications using AngularJS.
13. What are directives in AngularJS?
Directives in AngularJS are markers on DOM elements that extend the functionality of HTML. They allow you to create reusable components and attach specific behaviors to elements. Directives are defined using the directive function, and they can be used as attributes, elements, class names, or comments in the HTML. AngularJS provides built-in directives like ngModel, ngClick, ngRepeat, etc., and you can also create custom directives.
14. What is dependency injection in AngularJS?
Dependency injection (DI) in AngularJS is a design pattern that allows you to inject dependencies into a component rather than having the component create or manage its dependencies. DI helps to decouple components, improve testability, and promote modular code. AngularJS provides an injector subsystem that automatically resolves dependencies and injects them into components when they are instantiated.
15. What is the role of services in AngularJS?
Services in AngularJS are singleton objects that are used to organize and share code across different parts of an application. Services provide a way to encapsulate business logic, data access, or any other functionality that needs to be shared among multiple components. AngularJS provides several built-in services like $http, $timeout, $location, etc., and you can also create custom services.
16. What is the difference between $scope and controllerAs syntax?
In AngularJS, the $scope is an object that acts as a glue between the controller and the view. It allows the controller to communicate with the view and vice versa. With the $scope syntax, you bind model properties and functions directly to the $scope object in the controller and access them in the view using the ng-model and ng-click directives.
On the other hand, the controllerAs syntax allows you to bind controller properties and functions directly to the controller instance itself, rather than the $scope object. This syntax promotes the use of the this keyword in the controller, making the code more readable and avoiding potential scope-related issues.
17. What are AngularJS modules?
AngularJS modules are containers that encapsulate different parts of an application, such as controllers, services, directives, filters, etc. Modules provide a way to organize and modularize your code. They can depend on other modules and can be dependent on by other modules. Modules are defined using the angular.module function, and you can specify dependencies between modules when creating them.
18. What is the purpose of the $routeProvider in AngularJS?
The $routeProvider in AngularJS is used to configure routes and enable navigation between different views in a single-page application. It is part of the ngRoute module and provides methods to define routes, associate them with templates and controllers, and specify other configuration options. The $routeProvider allows you to create a navigation flow and handle URL routing within your AngularJS application.
These additional questions cover important concepts in AngularJS, including directives, dependency injection, services, $scope and controllerAs syntax, modules, and the $routeProvider. Understanding these concepts will help you build more robust and maintainable AngularJS applications.
19. What are factories and services in AngularJS?
Factories and services in AngularJS are two types of providers that are used to create reusable code and share data and functionality across different parts of an application. Both factories and services are singletons and can be injected into other components.
Factories are functions that return an object or function, and they can be used to create and configure objects. Services, on the other hand, are instantiated using the new keyword, and they can be used to define constructor functions. Both factories and services provide a way to encapsulate and share business logic, data access, and other common functionalities.
20. What is the difference between $watch and $watchCollection in AngularJS?
$watch and $watchCollection are two methods provided by the scope object in AngularJS to observe changes in data and trigger callbacks when those changes occur.
$watch is used to watch for changes in an expression, such as a scope property or a function, and it compares the current and previous values of the expression. If a change is detected, the associated callback function is invoked.
$watchCollection is used to watch for changes in an array or object and detect modifications to the collection itself, as well as changes to its properties or elements. It performs a shallow comparison of the collection to identify changes.
21. What is the use of $rootScope in AngularJS?
$rootScope is the top-level scope in an AngularJS application. It is a parent scope for all other scopes and can be used to store global data or functions that need to be accessible across multiple controllers or components. However, it is generally recommended to use $rootScope sparingly, as excessive use can lead to scope pollution and make the code harder to maintain.
22. What is the role of the ngModel directive in AngularJS?
The ngModel directive in AngularJS is used for two-way data binding between an input element and a scope property. It allows you to bind the value of an input field to a variable in the scope, so changes in the input field are automatically reflected in the model, and vice versa. The ngModel directive is often used with input elements like <input>, <textarea>, and <select>.
23. What is the use of the ngRepeat directive in AngularJS?
The ngRepeat directive in AngularJS is used to iterate over a collection, such as an array or an object, and generate HTML elements for each item in the collection. It allows you to create repeated blocks of HTML code based on the elements in the collection. The ngRepeat directive provides various features like filtering, sorting, and grouping of the repeated elements.
24. What is a digest cycle and how does it work in AngularJS?
The digest cycle in AngularJS is a mechanism that checks for changes in the model and updates the view accordingly. It is triggered by AngularJS whenever an event occurs, such as a user action or a timeout. During the digest cycle, AngularJS goes through all the watchers (bindings) in the scope hierarchy and checks if the watched values have changed. If a change is detected, AngularJS updates the corresponding part of the view.
The digest cycle continues until all the watchers have been checked and no more changes are detected. The digest cycle is essential for AngularJS to maintain the two-way data binding and keep the model and the view in sync.
These additional questions cover important concepts in AngularJS, including factories and services, $watch and $watchCollection, $rootScope, ngModel directive
25. What is the role of the $http service in AngularJS?
The $http service in AngularJS is used to make HTTP requests to retrieve or send data to a server. It provides methods like get, post, put, and delete to interact with RESTful APIs or any other HTTP-based services. The $http service returns a promise object, which allows you to handle the response asynchronously using .then() and .catch() methods.
26. What is the purpose of the $timeout service in AngularJS?
The $timeout service in AngularJS is used to delay the execution of a function by a specified amount of time. It is similar to the setTimeout() function in JavaScript but integrates seamlessly with AngularJS's digest cycle. The $timeout service ensures that the changes made inside the timeout function are properly digested, updating the view accordingly.
27. What is the purpose of the $interval service in AngularJS?
The $interval service in AngularJS is used to repeatedly execute a function at a specified interval. It is similar to the setInterval() function in JavaScript but integrates with AngularJS's digest cycle. The $interval service ensures that changes made inside the interval function are properly digested, allowing the view to be updated.
28. What is the use of the $location service in AngularJS?
The $location service in AngularJS provides access to the URL and allows you to interact with the browser's URL. It can be used to retrieve the current URL, navigate to a different URL, modify the URL parameters, and more. The $location service also integrates with AngularJS's routing system, allowing you to manipulate the URL based on the defined routes.
29. What is the role of the ngInclude directive in AngularJS?
The ngInclude directive in AngularJS is used to dynamically include an external HTML template into the current view. It allows you to reuse HTML code across multiple pages or sections of your application. The ngInclude directive fetches the specified template and inserts its content into the element where the directive is placed.
30. What are filters in AngularJS and how are they used?
Filters in AngularJS are used to format and manipulate data before it is displayed in the view. They can be used with expressions or binding values using the pipe character (|). AngularJS provides built-in filters like uppercase, lowercase, currency, date, and more. Filters can also be combined and chained to achieve complex transformations. Additionally, you can create custom filters to fulfill specific formatting or data manipulation requirements.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Angular JS, Node JS, Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
Leave a comment