PHP Interview Questions
..
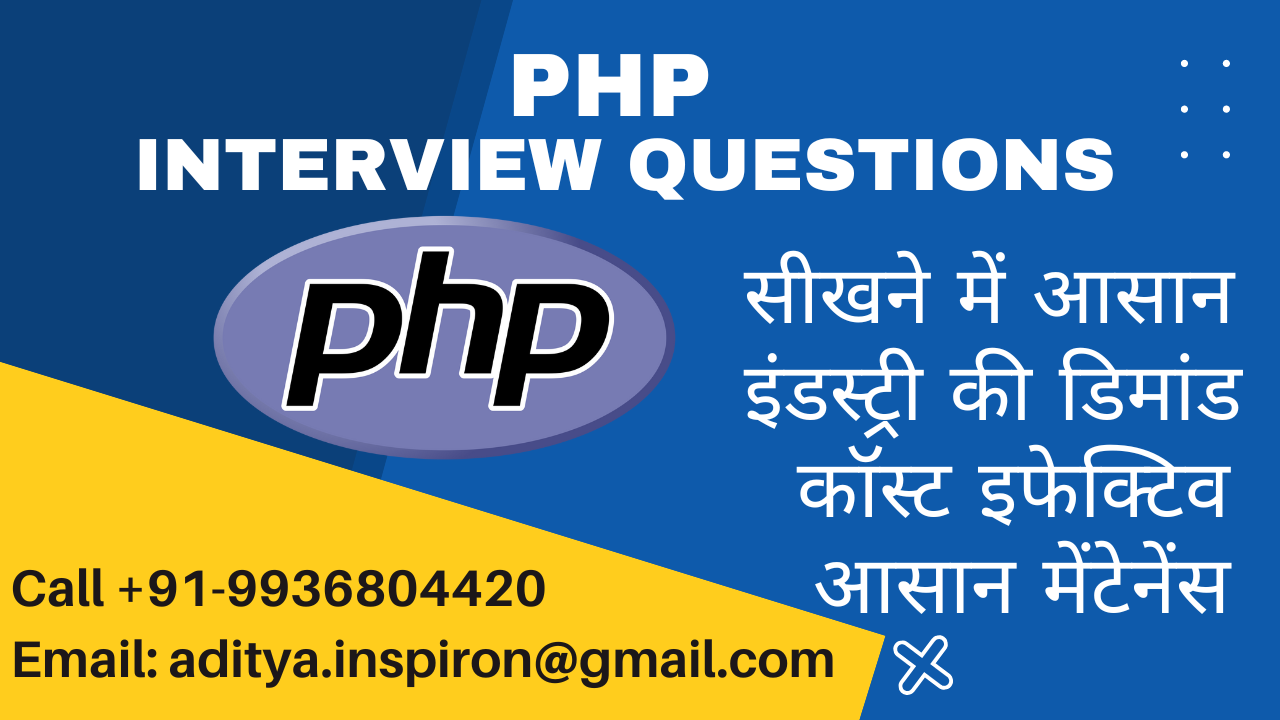
PHP Interview Questions
- PHP function is a piece of coda.
- That can be reused many time
- That can be used only once
- That can’t be used directly
- That can be used only at the initial level of the program
- PHP Functions
- Do not return a value c) delete the value
- Return a value d) always multiply the value
- In PHP, we can define
- Conditional Function c) Recursive Function
- Function within Function d) All of the above
- The advantages of PHP functions are
- Code Reusability c) Easy to understand
- Less code d) All of the above
- PHP Constants can be defined by
- Using define() function c) Both a and b
- Using const keyword d) None of the above
- Which of the following function in PHP returns the time of sunrise of a particular day and location?
- date_sunrise() b) date-sunrise()
- sunrise() d) None of the above
- Which PHP function converts an English text datetime into a Unix timestamp?
- str_to_time() c) strtotime()
- strtodate() d) None of the above
- Which function is used to calculate the products of an array?
- array_product() c) add_array_product()
- cal_array_product() d) save_array_product()
- What will be the output of the following program?
<?php
$a = array(16, 5, 2);
echo array_product($a);
?>
- 160 c) 80
- 1652 d) 32
- Name of Scripting Engine in PHP?
- SpiderMonkey
- Zend Engine
- VB Script
- CS Script
- Which function is used for testing the type of PHP variable?
- whattype()
- showtype()
- gettype()
- settype()
- Variables always start with a _____ in PHP
- Euro-sign
- Pond-sign
- Yen-sign
- Dollar-sign
- ______ Symbol is a newline character.
- \n c. /n
- \r d. /r
- _______ Variable is not a predefined variable.
- $GET c. $ASK
- $POST d. $REQUEST
- PHP is an example of _______ scripting language.
- Browser Side c. Server-Side
- Client Side d. In-Side
- Which method sends input to a script via a URL?
- GET c. POST
- Both d. None
- <?php
$x = 8;
$y = 8.0;
echo ($x === $y);
?>
- No output c. 0
- 8===8 d. 1
- Which programming language does PHP resemble?
- JavaScript c. Perl and C
- VBScript d. All of the above
- Does PHP support multiple inheritances?
- Yes c. Single and Multiple inheritance are supported
- No d. single and Multi level inheritance are supported
- We can use multiple inheritance with the help of
- Using interfaces in PHP c. Both A and B
- Using traits instead of Classes d. None of the above
- Correct syntax to write the php code within html is
- <?php echo $a;?> c. <?php echo $a ?>
- <?php echo $a;> d. <?php echo a;?
- Can PHP send or receive cookies?
- True c. PHP new version cannot but old can
- False d. PHP old version cant but new can
- The usage of strcmp() function in PHP is?
- To compare the strings excluding case
- To compare the uppercase strings
- To compare the lowercase strings
- To compare the strings including case
- The use of fopen() function in PHP is?
- To open folders in PHP
- To open remote server
- To open files in PHP
- None of the above
- The use of isset() function is ?
- To check whether variable is set or not
- To check whether the variable is free or not
- To check whether the variable is string or not
- None of the above
- Which of the following function is used to unset a variable in PHP?
- delete() c. unlink()
- unset() d. None of the above
- Which of the following function is used to sort an array in descending order?
- sort() c. dsort()
- asrot() d. rsort()
- Which of the following is/are the code editors in PHP?
- Notepad++ c. Adobe Dreamweaver
- Notepad d. All of the above
- Which of the following is used to end a statement in PHP?
- . (dot) c. ! (exclamation)
- ; (semicolon) d. / (slash)
- Which of the following function is used to get the ASCII value of a character in PHP?
- val() c. ascii()
- asc() d. chr()
- Which of the following function is used to find files in PHP?
- glob() c. file()
- fold() d. None of the above
- Which function is used to send mails in PHP?
- send_mail() c. mail()
- mail_to() d. share_mail()
- We can send the following thing using mail () function in PHP.
- Text Message c. Attachment with Message
- HTML Message d. All of the above
- The return type of mail function is
- String c. Integer
- Char d. Boolean
- $to in mail($to,$subject,$message,$header) refers to
- Receiver c. Transmitter
- Sender d. None of the above
- Which form is inappropriate for specifying receiver or receivers of the mail?
- user@example.com
- user@example.com, anotheruser@example.com
- User <user@example.com>
- None of the above
- Which function is used to remove whitespaces and other predefined characters from both the sides of the string?
- trimmer(); c) trim_str();
- trim(); d) str_trim();
- What is the output of the program?
<?php
define("MSG","Welcome to Inspiron Technologies",false);
echo msg;
?>
- Welcome to Inspiron Technologies c) Use of undefined constant msg
- No Output d) No of the above
- Which of the following function is used to compute the difference between two arrays in PHP?
- diff_array c) arrays_diff
- array_diff d) diff_arrays
- PHP parser is
- <?php > c) <?php ?
- <php > d) <?php ?>
- A cookie is used to
- Forget a user c) install the apps
- Identify a user d) brows the apps
- The scope of Cookie in PHP is
- Single Page c) Multiple Pages
- Single Function d) Multiple Functions
- We can store only __________ in a cookie.
- Objects c) Both A and B
- String d) None of the above
- The maximum size of a cookie is
- 5096 bytes c) 1096 bytes
- 4096 bytes d) 1506 bytes
- The Initial size of the cookie is
- 100 bytes c) 20 bytes
- 50 bytes d) 70 bytes
- How many cookies can be created in one website or web app?
- 30 cookies c) 10 cookies
- 20 cookies d) 40 cookies
- By default, cookies are
- Temporary c) saved in the browser only
- Transitory d) All of the above
- The include() function is used to
- Put data of one file into another file. c) Browses data of a file.
- Updates data of one file d) Counts data of a file.
- If errors occur while including a file into another file using include() function
- It stops the execution of the script
- It does not given any warning
- Produces a warning and continue to execute
- Neither error nor warning takes place.
- The Require() function is used to
- Put data of one file into another file. c) Browses data of a file.
- Updates data of one file d) Counts data of a file.
Leave a comment