PHP AJAX interview questions with answers
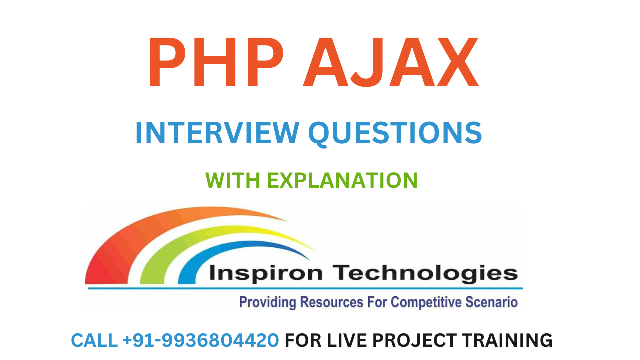
PHP AJAX interview questions with answers
Q: What is AJAX?
A: AJAX stands for Asynchronous JavaScript and XML. It is a technique used for creating fast and dynamic web pages that update content without reloading the entire page.
Q: What is the role of PHP in AJAX?
A: PHP is often used as the server-side scripting language for AJAX applications. It is responsible for processing the requests sent by the client-side JavaScript and returning the appropriate responses.
Q: How do you make an AJAX request in PHP?
A: You can make an AJAX request in PHP using the XMLHttpRequest object in JavaScript. Here's an example:
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php", true);
xhttp.send();
Q: What is the difference between GET and POST methods in AJAX?
A: The GET method sends data in the URL, while the POST method sends data in the request body. The GET method is suitable for requesting data from the server, while the POST method is suitable for submitting data to the server.
Q: How do you handle errors in AJAX requests?
A: You can handle errors in AJAX requests using the onerror event in JavaScript. Here's an example:
var xhttp = new XMLHttpRequest();
xhttp.onerror = function() {
// Handle the error
};
xhttp.open("GET", "ajax.php", true);
xhttp.send();
Q: How do you send data in an AJAX request using PHP?
A: You can send data in an AJAX request using the data parameter in the XMLHttpRequest.open() method. Here's an example:
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("POST", "ajax.php", true);
xhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
xhttp.send("name=John&age=30");
In this example, we're sending two parameters, "name" and "age", to the "ajax.php" script using the POST method. The setRequestHeader() method sets the content type to "application/x-www-form-urlencoded", which is the default content type for sending form data in HTTP requests.
Q: How do you return JSON data from a PHP script in an AJAX request?
A: You can return JSON data from a PHP script using the json_encode() function. Here's an example:
$data = array("name" => "John", "age" => 30);
echo json_encode($data);
This code will return the following JSON data: {"name":"John","age":30}
Q: How do you handle JSON data in an AJAX response in JavaScript?
A: You can handle JSON data in an AJAX response using the JSON.parse() method in JavaScript. Here's an example:
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var data = JSON.parse(this.responseText);
// Do something with the data
}
};
xhttp.open("GET", "ajax.php", true);
xhttp.send();
In this example, we're parsing the JSON data returned by the "ajax.php" script and storing it in the "data" variable. We can then use this data to update the content of our web page.
Q: How do you implement a progress bar for an AJAX request in PHP?
A: You can implement a progress bar for an AJAX request in PHP using the onprogress event in JavaScript and the Content-Length header in PHP. Here's an example:
var xhttp = new XMLHttpRequest();
xhttp.onprogress = function(e) {
var percent = (e.loaded / e.total) * 100;
// Update the progress bar
};
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php", true);
xhttp.send();
In this example, we're updating the progress bar based on the percentage of the file that has been loaded. We can calculate this percentage using the "loaded" and "total" properties of the event object. On the PHP side, we can set the Content-Length header to the size of the file being sent, which will allow the client-side JavaScript to track the progress of the request.
Q: How do you prevent cross-site scripting (XSS) attacks in AJAX requests?
A: You can prevent cross-site scripting attacks in AJAX requests by sanitizing user input and using the htmlspecialchars() function in PHP. Here's an example:
$name = htmlspecialchars($_POST['name']);
$age = intval($_POST['age']);
In this example, we're using the htmlspecialchars() function to convert any special characters in the user input to HTML entities, which prevents them from being interpreted as HTML code. We're also using the intval() function to convert the user input to an integer, which prevents SQL injection attacks.
Q: How do you debug AJAX requests in PHP?
A: You can debug AJAX requests in PHP using the error_log() function and the console.log() function in JavaScript. Here's an example:
error_log('Request received: ' . print_r($_POST, true));
console.log('Response received: ' + JSON.stringify(data));
In this example, we're logging the request data on the PHP side using the error_log() function, and we're logging the response data on the client-side JavaScript using the console.log() function. This allows us to track the flow of data between the client and server and identify any errors that may occur.
These are some of the common PHP AJAX interview questions with answers. It's important to have a good understanding of both PHP and AJAX in order to develop fast and dynamic web applications.
Q: How do you handle errors in AJAX requests in PHP?
A: You can handle errors in AJAX requests in PHP by using the header() function to set the HTTP response code and the die() function to terminate the script. Here's an example:
if (!isset($_POST['username'])) {
header('HTTP/1.1 400 Bad Request');
die('Error: Username not specified');
}
In this example, we're checking if the username field is set in the POST data. If it's not set, we're setting the HTTP response code to 400 (Bad Request) and returning an error message.
Q: How do you send and receive JSON data in AJAX requests in PHP?
A: You can send and receive JSON data in AJAX requests in PHP using the json_encode() and json_decode() functions. Here's an example:
// Send JSON data from PHP
$data = array('name' => 'John', 'age' => 30);
echo json_encode($data);
// Receive JSON data in JavaScript
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var data = JSON.parse(this.responseText);
// Do something with the JSON data
}
};
xhttp.open("GET", "ajax.php", true);
xhttp.send();
In this example, we're sending JSON data from PHP using the json_encode() function. In the client-side JavaScript, we're using the JSON.parse() function to convert the JSON data into a JavaScript object.
Q: How do you implement pagination in AJAX requests in PHP?
A: You can implement pagination in AJAX requests in PHP by sending the current page number as a parameter in the AJAX request and using the LIMIT and OFFSET clauses in the SQL query. Here's an example:
// AJAX request
var page = 1;
function loadPage(page) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php?page=" + page, true);
xhttp.send();
}
// PHP code
$limit = 10;
$offset = ($page - 1) * $limit;
$sql = "SELECT * FROM table LIMIT $limit OFFSET $offset";
In this example, we're sending the current page number as a parameter in the AJAX request and using it to calculate the LIMIT and OFFSET clauses in the SQL query. This allows us to fetch only the required data and implement pagination in the AJAX request.
These are some additional PHP AJAX interview questions with answers that can help you prepare for your interview. It's important to have a good understanding of the core concepts and techniques used in PHP and AJAX development in order to create efficient and effective web applications.
Q: How do you handle file uploads in AJAX requests in PHP?
A: You can handle file uploads in AJAX requests in PHP by using the FormData() object and the move_uploaded_file() function. Here's an example:
// AJAX request
var formData = new FormData();
formData.append('file', fileInput.files[0]);
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("POST", "ajax.php", true);
xhttp.send(formData);
// PHP code
if (isset($_FILES['file'])) {
$file = $_FILES['file'];
$filename = $file['name'];
$tmp_name = $file['tmp_name'];
$destination = "uploads/" . $filename;
move_uploaded_file($tmp_name, $destination);
}
In this example, we're using the FormData() object to create a new form data object and append the file input to it. In the PHP code, we're checking if the file is set in the $_FILES array and using the move_uploaded_file() function to move the file to a specified destination.
Q: How do you implement a search functionality in AJAX requests in PHP?
A: You can implement a search functionality in AJAX requests in PHP by sending the search query as a parameter in the AJAX request and using the LIKE clause in the SQL query. Here's an example:
// AJAX request
var searchQuery = document.getElementById('searchQuery').value;
function search() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php?search=" + searchQuery, true);
xhttp.send();
}
// PHP code
if (isset($_GET['search'])) {
$searchQuery = $_GET['search'];
$sql = "SELECT * FROM table WHERE column LIKE '%$searchQuery%'";
}
In this example, we're sending the search query as a parameter in the AJAX request and using it to construct the SQL query with the LIKE clause. This allows us to fetch only the relevant data and implement search functionality in the AJAX request.
Q: How do you handle authentication in AJAX requests in PHP?
A: You can handle authentication in AJAX requests in PHP by sending the authentication token as a parameter in the AJAX request and verifying it on the server-side using session variables or database queries. Here's an example:
// AJAX request
var authToken = localStorage.getItem('authToken');
function sendRequest() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php?authToken=" + authToken, true);
xhttp.send();
}
// PHP code
if (isset($_GET['authToken'])) {
$authToken = $_GET['authToken'];
// Verify the authentication token using session variables or database queries
if (/* authentication successful */) {
// Process the AJAX request
} else {
header('HTTP/1.1 401 Unauthorized');
die('Error: Authentication failed');
}
}
In this example, we're sending the authentication token as a parameter in the AJAX request and verifying it on the server-side using session variables or database queries. If the authentication is successful, we process the AJAX request. Otherwise, we set the HTTP response code to 401 (Unauthorized) and return
Q: How do you implement pagination in AJAX requests in PHP?
A: You can implement pagination in AJAX requests in PHP by sending the page number as a parameter in the AJAX request and using it to construct the SQL query with the LIMIT and OFFSET clauses. Here's an example:
// AJAX request
var pageNumber = 1;
function nextPage() {
pageNumber++;
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("GET", "ajax.php?page=" + pageNumber, true);
xhttp.send();
}
// PHP code
if (isset($_GET['page'])) {
$page = $_GET['page'];
$resultsPerPage = 10;
$offset = ($page - 1) * $resultsPerPage;
$sql = "SELECT * FROM table LIMIT $resultsPerPage OFFSET $offset";
}
In this example, we're sending the page number as a parameter in the AJAX request and using it to construct the SQL query with the LIMIT and OFFSET clauses. This allows us to fetch only the relevant data and implement pagination functionality in the AJAX request.
Q: How do you prevent CSRF attacks in AJAX requests in PHP?
A: You can prevent CSRF (Cross-Site Request Forgery) attacks in AJAX requests in PHP by using a CSRF token. Here's an example:
// AJAX request
var csrfToken = localStorage.getItem('csrfToken');
function sendRequest() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Do something with the response
}
};
xhttp.open("POST", "ajax.php", true);
xhttp.setRequestHeader("X-CSRF-Token", csrfToken);
xhttp.send();
}
// PHP code
session_start();
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$requestToken = $_SERVER['HTTP_X_CSRF_TOKEN'] ?? '';
if (!hash_equals($_SESSION['csrfToken'], $requestToken)) {
header('HTTP/1.1 403 Forbidden');
die('Error: CSRF token validation failed');
}
// Process the AJAX request
}
In this example, we're using a CSRF token to prevent CSRF attacks. The token is generated on the server-side and stored in the user's session. When the AJAX request is sent, the token is included in the request header. On the server-side, we check if the request token is equal to the session token. If they're not equal, we return a 403 (Forbidden) response and an error message.
Q: What is the difference between GET and POST methods in AJAX requests?
A: The GET method in AJAX requests sends data in the URL query string, while the POST method sends data in the request body. The GET method is typically used for fetching data, while the POST method is used for submitting data to the server.
Q: How do you handle errors in AJAX requests in PHP?
A: You can handle errors in AJAX requests in PHP by using try-catch blocks and returning error messages in the response. Here's an example:
// AJAX request
function sendRequest() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4) {
if (this.status == 200) {
// Do something with the response
} else {
console.log(this.statusText);
}
}
};
xhttp.open("POST", "ajax.php", true);
xhttp.send();
}
// PHP code
try {
// Process the AJAX request
$response = ['success' => true, 'data' => $data];
echo json_encode($response);
} catch (Exception $e) {
$response = ['success' => false, 'error' => $e->getMessage()];
echo json_encode($response);
}
In this example, we're using a try-catch block to handle errors in the AJAX request. If an error occurs, we catch the exception and return an error message in the response. On the client-side, we check if the response has a success property, and if it's false, we display the error message.
Q: How do you implement autocomplete functionality in AJAX requests in PHP?
A: You can implement autocomplete functionality in AJAX requests in PHP by sending the search term as a parameter in the AJAX request and returning matching results in the response. Here's an example:
// AJAX request
function search(query) {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var results = JSON.parse(this.responseText);
// Display the results
}
};
xhttp.open("POST", "ajax.php", true);
xhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
xhttp.send("query=" + query);
}
// PHP code
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$query = $_POST['query'] ?? '';
$results = []; // Search the database for matching results
echo json_encode($results);
}
In this example, we're sending the search term as a parameter in the AJAX request and searching the database for matching results on the server-side. We're returning the results in the response as a JSON-encoded array. On the client-side, we're parsing the JSON response and displaying the results in the autocomplete dropdown.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
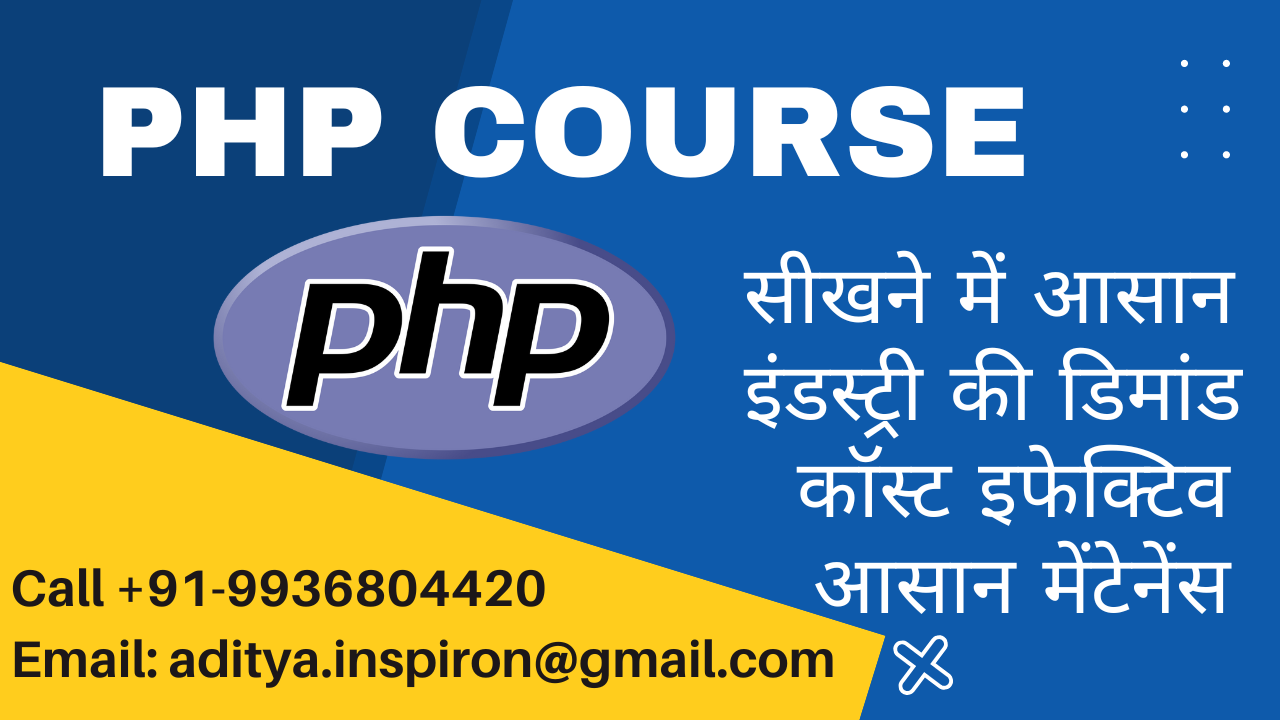
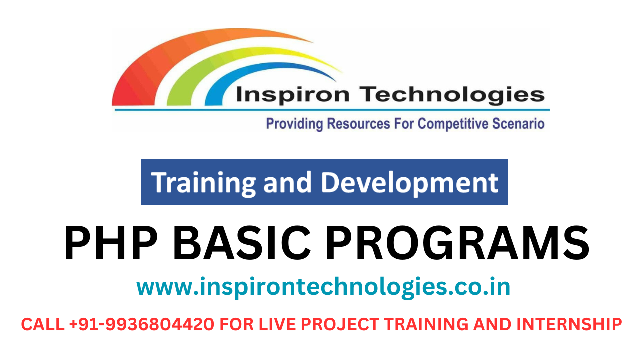
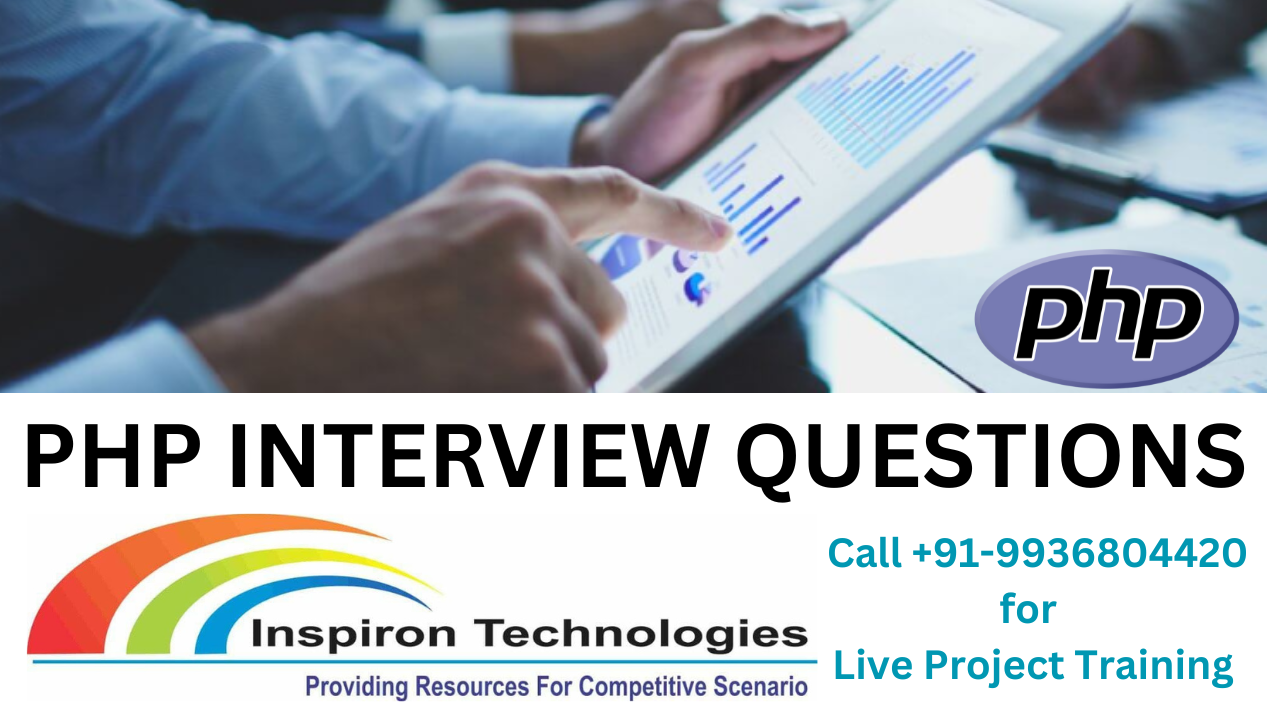
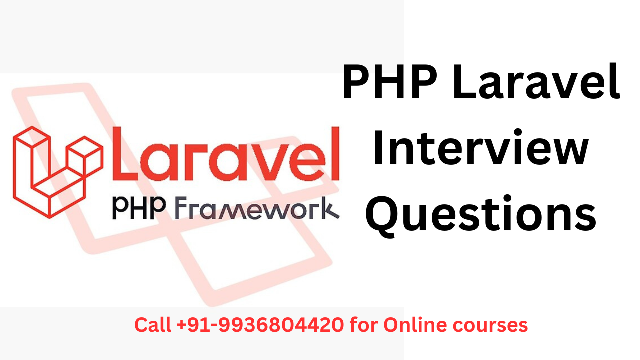
.png)
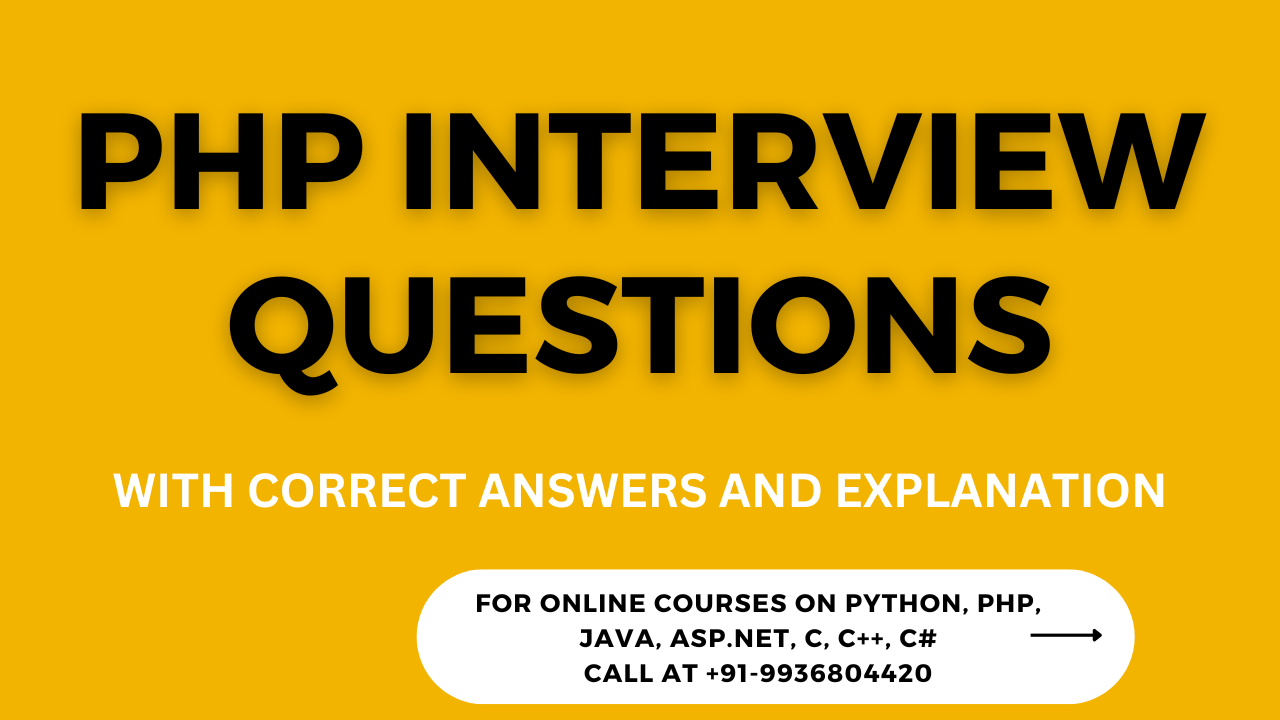
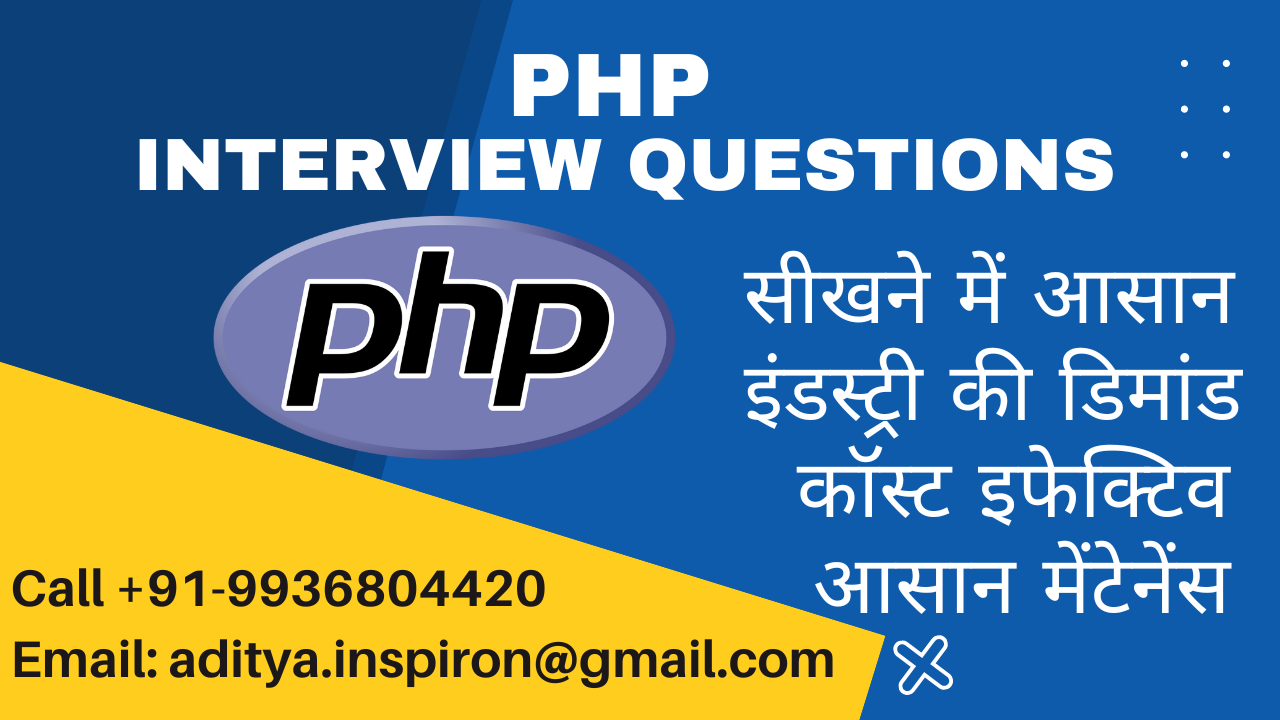
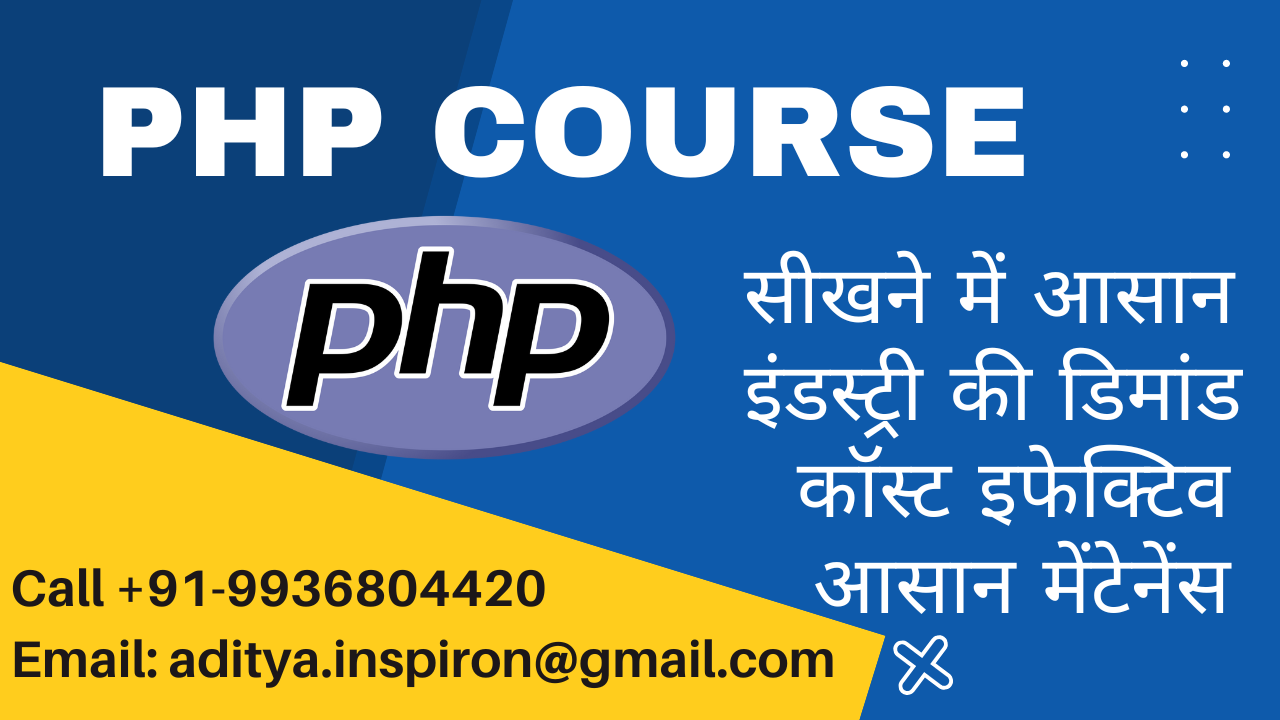
Categories
Popular Post
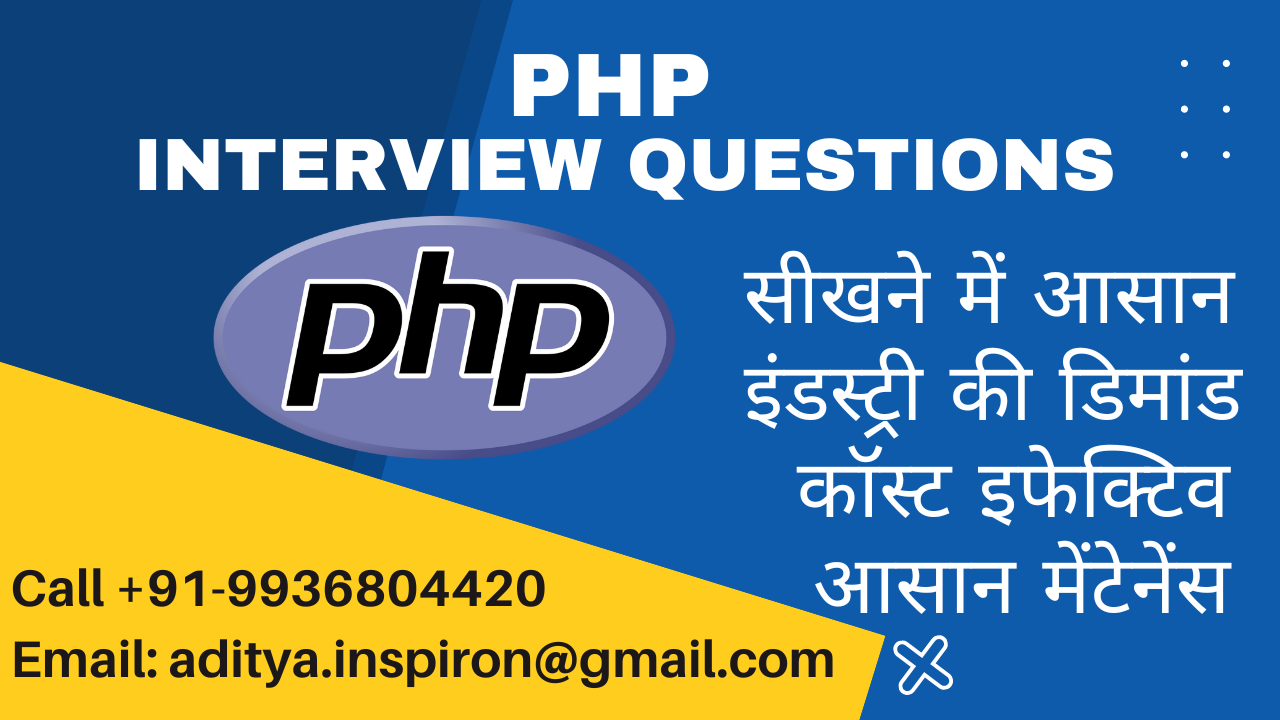
.png)
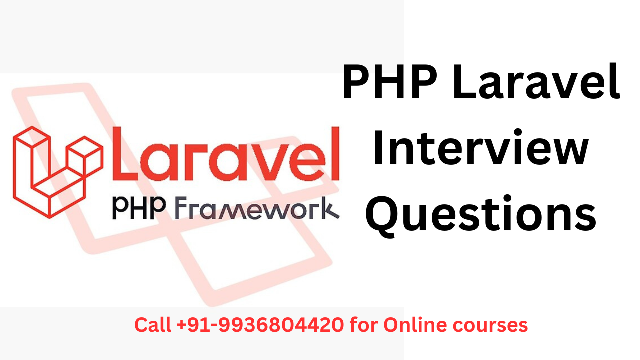
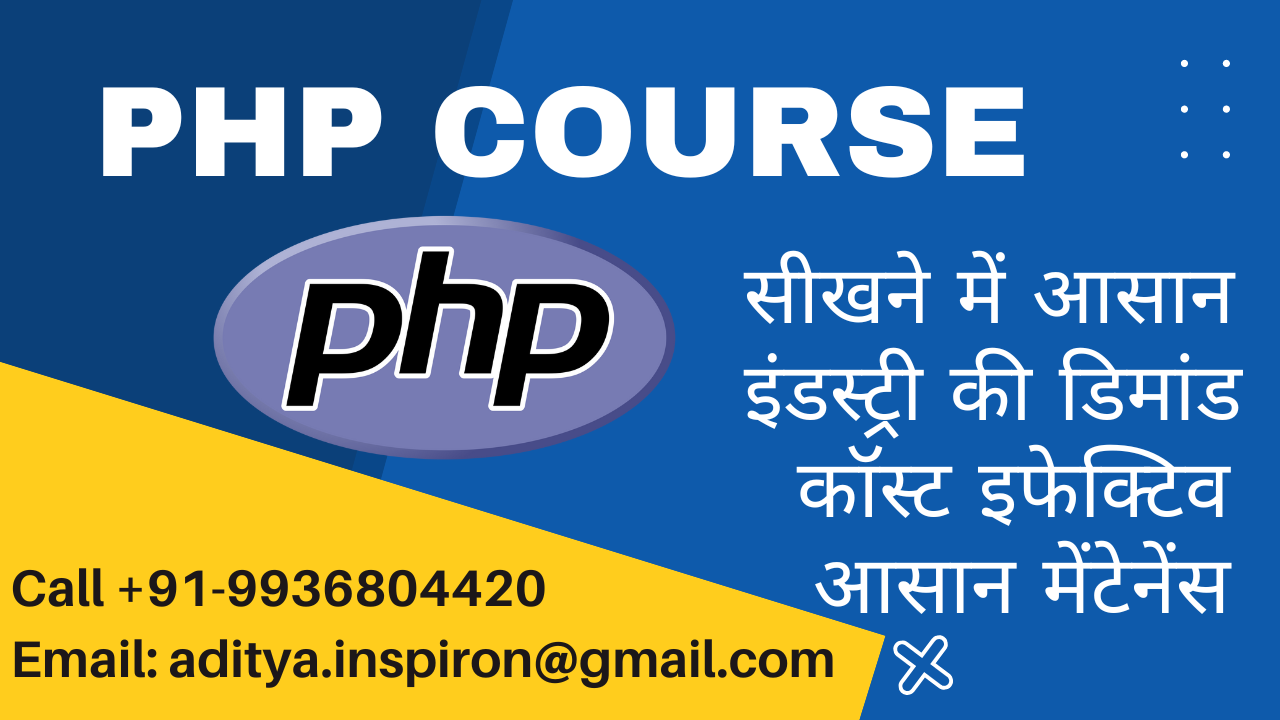
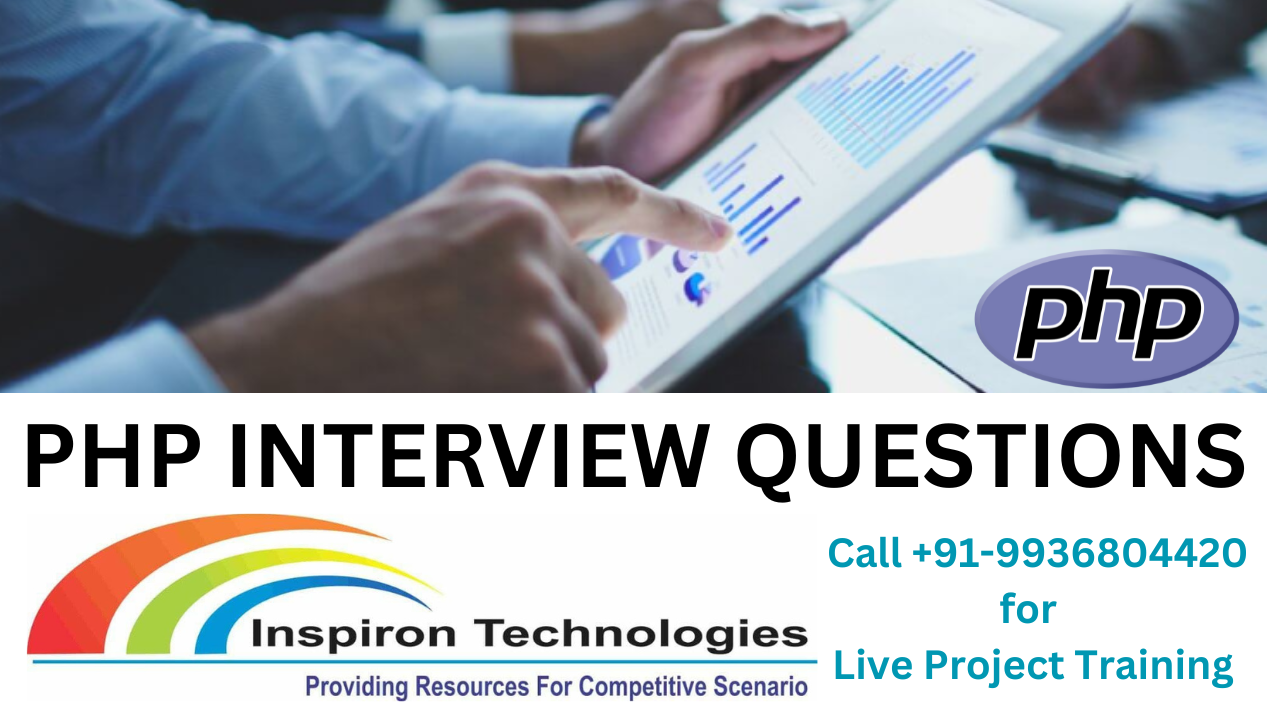
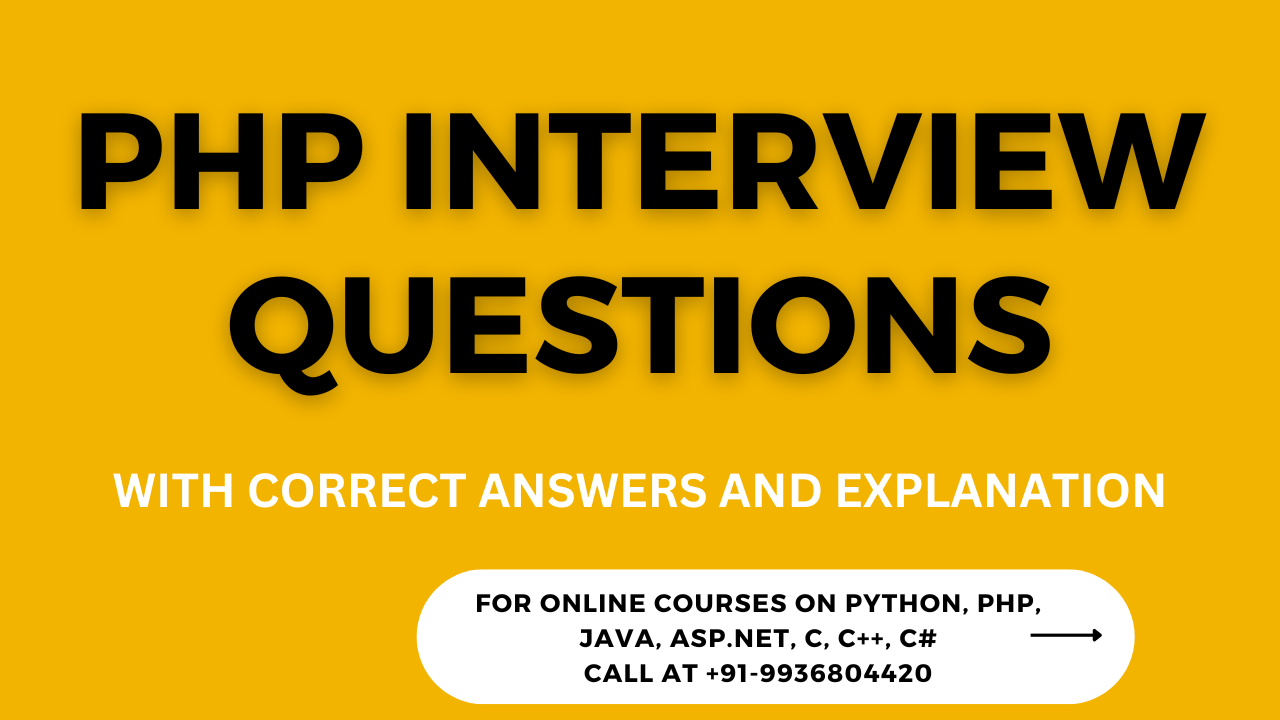
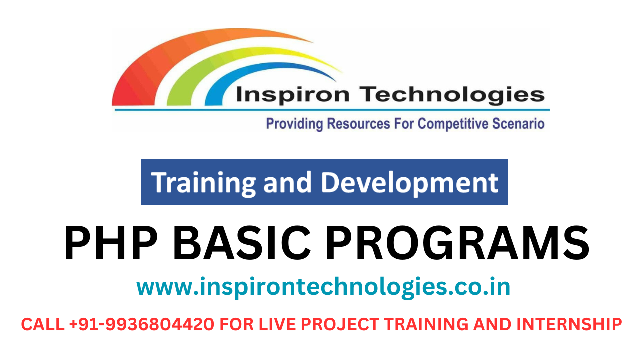
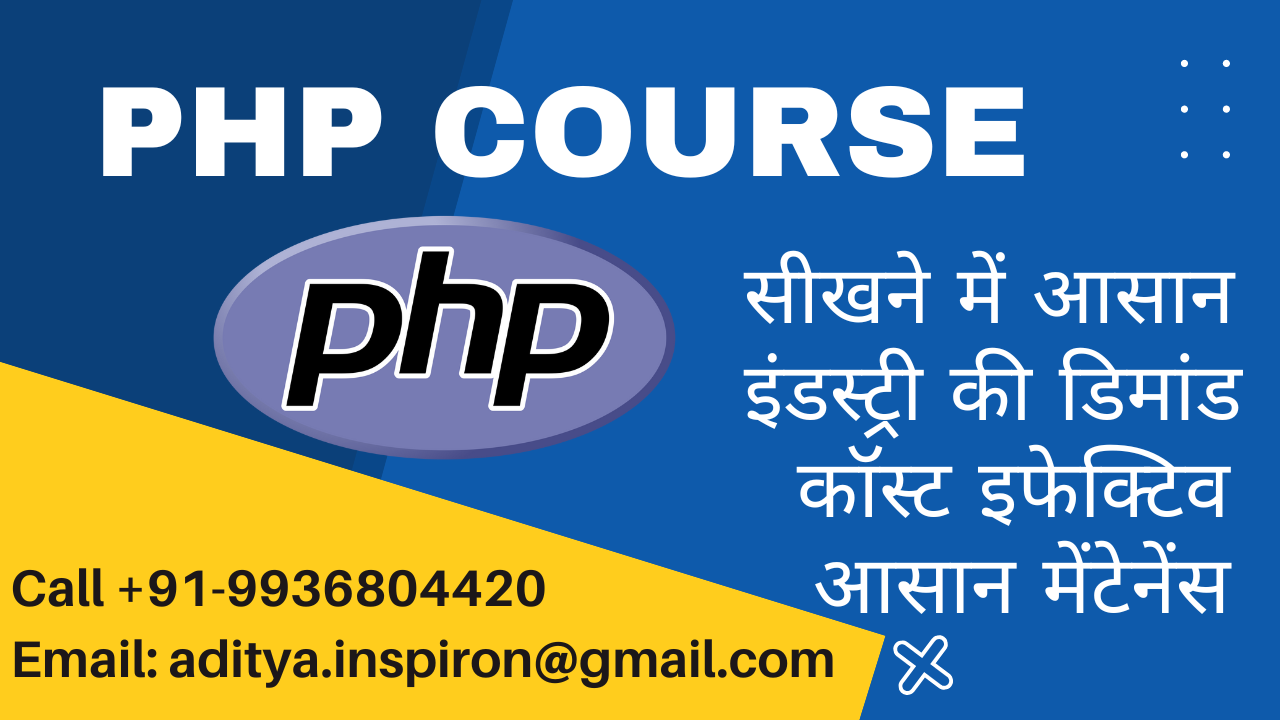
Leave a comment