PHP Programs for Beginners
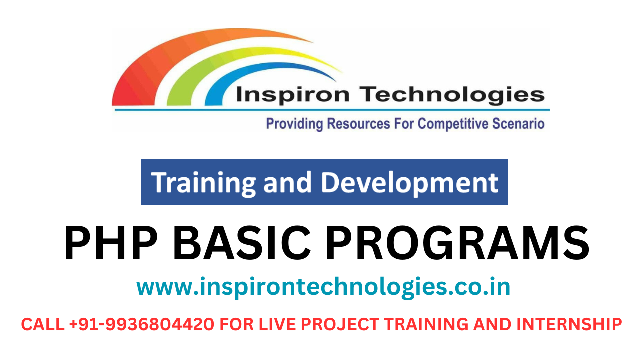
PHP Basic Programs for Beginners : PHP Tutorial
1. What is the output of the following PHP code snippet?
$a = 10;
$b = 5;
echo $a + $b . " " . $a - $b;
Explanation: The output of this code will be 15 10. In PHP, the . operator is used for string concatenation. The expression $a + $b evaluates to 15, which is concatenated with a space character. Then, the expression $a - $b is evaluated and the result 10 is concatenated with the previous string. Finally, the resulting string 15 10 is displayed using the echo statement.
2. What will be the output of the following code snippet?
$num = 4;
$result = ($num % 2 == 0) ? "Even" : "Odd";
echo $result;
Explanation: The output will be Even. In this code, the ternary operator ? : is used to assign the value of $result. The expression $num % 2 == 0 checks if $num is divisible by 2 with no remainder, which is true for the value 4. Therefore, the value assigned to $result is "Even", and it is displayed using the echo statement.
3. What will be the output of the following code snippet?
function multiply($a, $b = 2) {
return $a * $b;
}
$result1 = multiply(5);
$result2 = multiply(3, 4);
echo $result1 . " " . $result2;
Explanation: The output will be 10 12. The multiply() function takes two parameters, $a and $b, where $b has a default value of 2 if not provided. In the first call to multiply(), only one argument 5 is passed, so the default value of $b is used. Thus, $result1 is 5 * 2 = 10. In the second call, both arguments 3 and 4 are provided, resulting in $result2 being 3 * 4 = 12. Finally, the two results are concatenated with a space character and displayed using the echo statement.
4. What will be the output of the following code snippet?
$x = 5;
function increment() {
global $x;
$x++;
}
increment();
echo $x;
Explanation: The output will be 6. In this code, the global keyword is used to access the global variable $x within the increment() function. The function increments the value of $x by one. Therefore, after the increment() function is called, the value of $x becomes 6, which is then displayed using the echo statement.
5. What will be the output of the following code snippet?
$string = "Hello World";
$length = strlen($string);
echo $length;
Explanation: The output will be 11. The strlen() function is used to calculate the length of a string. In this code, the string variable $string contains the value "Hello World". The strlen() function counts the number of characters in the string, including spaces. Therefore, the length of the string is 11, which is then displayed using the echo statement.
6. What will be the output of the following code snippet?
$numbers = array(3, 1, 4, 1, 5);
$sum = array_sum($numbers);
echo $sum;
Explanation: The output will be 14. The array_sum() function is used to calculate the sum of all values in an array. In this code, the array $numbers contains the values [3, 1, 4, 1, 5]. The array_sum() function adds up all the values in the array, resulting in a sum of 14, which is then displayed using the echo statement.
7. What is the output of the following code snippet?
$a = 10;
$b = "5";
$result = $a + $b;
echo $result;
Explanation: The output will be 15. In this code, the variable $a is an integer with a value of 10, and the variable $b is a string with a value of "5". When performing the addition operation $a + $b, PHP performs automatic type conversion and converts the string "5" to an integer before performing the addition. Therefore, the result of the addition is 10 + 5 = 15, which is then displayed using the echo statement.
8. What is the output of the following code snippet?
$a = 5;
function square(&$num) {
$num *= $num;
}
square($a);
echo $a;
Explanation: The output will be 25. In this code, the square() function accepts a parameter $num passed by reference (&$num). This means any changes made to $num within the function will affect the original variable outside the function. The function squares the value of $num by multiplying it with itself. When the square() function is called with the argument $a, the value of $a is modified to 25. Therefore, when $a is displayed using the echo statement, it outputs 25.
9. What is the output of the following code snippet?
$x = 0;
while ($x < 5) {
echo $x . " ";
$x++;
}
Explanation: The output will be 0 1 2 3 4 . In this code, a while loop is used to iterate as long as the condition $x < 5 is true. Within the loop, the value of $x is displayed using the echo statement. Initially, $x is 0, so 0 is echoed. Then, $x is incremented by 1 using $x++. This process continues until $x reaches 5. At that point, the condition $x < 5 becomes false, and the loop terminates.
10. What will be the output of the following code snippet?
$a = 5;
$b = 10;
function multiply() {
global $a, $b;
$result = $a * $b;
return $result;
}
$product = multiply();
echo $product;
Explanation: The output will be 50. In this code, the multiply() function is defined and it accesses the global variables $a and $b using the global keyword. The function multiplies the values of $a and $b and stores the result in the local variable $result. The function then returns the value of $result. When the multiply() function is called and its return value is assigned to the variable $product, it becomes 50. Finally, $product is displayed using the echo statement.
11. What will be the output of the following code snippet?
$num = 8;
if ($num % 2 == 0) {
echo "Even";
} else {
echo "Odd";
}
Explanation: The output will be Even. In this code, an if statement is used to check if $num is divisible by 2 with no remainder. Since 8 is divisible by 2, the condition $num % 2 == 0 evaluates to true, and the code inside the if block is executed. Therefore, the string "Even" is displayed using the echo statement.
12. What will be the output of the following code snippet?
$name = "John Doe";
$parts = explode(" ", $name);
$first_name = $parts[0];
$last_name = $parts[1];
echo $first_name . " " . $last_name;
Explanation: The output will be John Doe. In this code, the explode() function is used to split the string $name into an array of substrings using the space character as the delimiter. The resulting array $parts will contain the elements ["John", "Doe"]. The first element of the array ($parts[0]) represents the first name, which is assigned to the variable $first_name. The second element ($parts[1]) represents the last name, assigned to $last_name. Finally, both variables are concatenated with a space character using the echo statement, resulting in John Doe being displayed.
13. What is the output of the following code snippet?
$num = 10;
for ($i = 0; $i < $num; $i++) {
if ($i % 2 == 0) {
continue;
}
echo $i . " ";
}
Explanation: The output will be 1 3 5 7 9. In this code, a for loop is used to iterate from $i = 0 to $i < $num, incrementing $i by 1 in each iteration. Within the loop, an if statement is used to check if $i is divisible by 2 with no remainder. If it is, the continue statement is encountered, which skips the remaining code inside the loop for that iteration and moves on to the next iteration. Therefore, when $i is even, the continue statement is executed, skipping the echo statement. When $i is odd, the echo statement is executed, displaying the value of $i followed by a space character.
14. What is the output of the following code snippet?
$numbers = [1, 2, 3, 4, 5];
foreach ($numbers as &$value) {
$value *= 2;
}
echo implode(" ", $numbers);
Explanation: The output will be 2 4 6 8 10. In this code, a foreach loop is used to iterate over each element of the $numbers array. The &$value notation is used to create a reference to each element of the array, allowing us to modify the original values. Within the loop, each element is multiplied by 2. Therefore, the array elements are modified to [2, 4, 6, 8, 10]. Finally, the implode() function is used to concatenate the array elements into a string, separated by a space character, and the resulting string is displayed using the echo statement.
15. What is the output of the following code snippet?
function greet($name = "Guest") {
echo "Hello, " . $name . "!";
}
greet("John");
Explanation: The output will be Hello, John!. In this code, a greet() function is defined with a parameter $name that has a default value of "Guest". When the function is called with the argument "John", the value of $name within the function becomes "John". Therefore, the echo statement displays "Hello, John!".
16. What is the output of the following code snippet?
$a = 5;
function increment(&$num) {
$num++;
}
increment($a);
echo $a;
Explanation: The output will be 6. In this code, the increment() function accepts a parameter $num passed by reference (&$num). This means any changes made to $num within the function will affect the original variable outside the function. The function increments the value of $num by one. When the increment() function is called with the argument $a, the value of $a is modified to 6. Therefore, when $a is displayed using the echo statement, it outputs 6.
17. What is the output of the following code snippet?
$fruits = ["Apple", "Banana", "Orange"];
foreach ($fruits as $fruit) {
echo $fruit . " ";
if ($fruit == "Banana") {
break;
}
}
Explanation: The output will be Apple Banana . In this code, a foreach loop is used to iterate over each element of the $fruits array. The variable $fruit takes the value of each element in the array in each iteration. Within the loop, the value of $fruit is displayed using the echo statement. When the value of $fruit becomes "Banana", the if condition is met and the break statement is executed, which terminates the loop. Therefore, only the elements "Apple" and "Banana" are displayed before the loop is exited.
18. What is the output of the following code snippet?
function factorial($n) {
if ($n <= 1) {
return 1;
} else {
return $n * factorial($n - 1);
}
}
echo factorial(5);
Explanation: The output will be 120. In this code, the factorial() function is recursively defined to calculate the factorial of a given number. The base case is when $n is less than or equal to 1, in which case 1 is returned. For other values of $n, the function recursively calls itself with the argument $n - 1 and multiplies the current value of $n with the factorial of $n - 1. When factorial(5) is called, it calculates 5 * 4 * 3 * 2 * 1, which is 120. Finally, 120 is displayed using the echo statement.
19. What is the output of the following code snippet?
$a = 10;
$b = 5;
$result = ($a > $b) ? "Greater" : "Smaller";
echo $result;
Explanation: The output will be Greater. In this code, a ternary operator (? :) is used to assign a value to the variable $result based on the condition $a > $b. If the condition is true, i.e., if $a is greater than $b, then the value "Greater" is assigned to $result. Otherwise, if the condition is false, the value "Smaller" is assigned. Since 10 is greater than 5, the condition is true, and "Greater" is assigned to $result. Finally, $result is displayed using the echo statement.
20. What is the output of the following code snippet?
$str = "Hello World";
$reversed = strrev($str);
echo $reversed;
Explanation: The output will be dlroW olleH. In this code, the strrev() function is used to reverse the characters in a string. The string variable $str contains the value "Hello World". The strrev() function reverses the characters in the string, resulting in "dlroW olleH". Finally, the reversed string is displayed using the echo statement.
21. What is the output of the following code snippet?
$numbers = [2, 4, 6, 8, 10];
$squared = array_map(function($num) {
return $num * $num;
}, $numbers);
echo implode(" ", $squared);
Explanation: The output will be 4 16 36 64 100. In this code, the array_map() function is used to apply a callback function to each element of the $numbers array. The callback function takes each element, squares it by multiplying it with itself, and returns the squared value. The resulting array $squared will contain the squared values [4, 16, 36, 64, 100]. Finally, the implode() function is used to concatenate the array elements into a string, separated by a space character, and the resulting string is displayed using the echo statement.
22. What is the output of the following code snippet?
$counter = 0;
while ($counter < 5) {
$counter++;
if ($counter == 3) {
continue;
}
echo $counter . " ";
}
Explanation: The output will be 1 2 4 5. In this code, a while loop is used to iterate as long as the condition $counter < 5 is true. Within the loop, $counter is incremented by 1 using $counter++. The if statement checks if $counter is equal to 3. If it is, the continue statement is encountered, which skips the remaining code inside the loop for that iteration and moves on to the next iteration. Therefore, when $counter is 3, the continue statement is executed, skipping the echo statement. When $counter is not equal to 3, the echo statement is executed, displaying the value of $counter followed by a space character.
23. What is the output of the following code snippet?
function isEven($num) {
return $num % 2 == 0;
}
$numbers = [1, 2, 3, 4, 5];
foreach ($numbers as $number) {
if (isEven($number)) {
continue;
}
echo $number . " ";
}
Explanation: The output will be 1 3 5. In this code, a foreach loop is used to iterate over each element of the $numbers array. The variable $number takes the value of each element in the array in each iteration. Within the loop, the isEven() function is called to check if $number is even. If it is, the continue statement is executed, which skips the remaining code inside the loop for that iteration and moves on to the next iteration. Therefore, when $number is even, the continue statement is executed, skipping the echo statement. When $number is odd, the echo statement is executed, displaying the value of $number followed by a space character.
24. What is the output of the following code snippet?
$x = 5;
do {
echo $x . " ";
$x--;
} while ($x > 0);
Explanation: The output will be 5 4 3 2 1. In this code, a do-while loop is used. The loop body is executed first, and then the condition $x > 0 is checked. The variable $x is displayed using the echo statement, and then it is decremented by 1 using $x--. This process continues until $x becomes 0. At that point, the condition $x > 0 becomes false, but the loop body is still executed once more because it is a do-while loop. Therefore, the value 1 is displayed before the loop terminates.
25. What is the output of the following code snippet?
for ($i = 1; $i <= 10; $i++) {
if ($i % 2 == 0) {
continue;
}
echo $i . " ";
}
Explanation: The output will be 1 3 5 7 9. In this code, a for loop is used to iterate from $i = 1 to $i <= 10, incrementing $i by 1 in each iteration. Within the loop, an if statement is used to check if $i is divisible by 2 with no remainder. If it is, the continue statement is executed, which skips the remaining code inside the loop for that iteration and moves on to the next iteration. Therefore, when $i is even, the continue statement is executed, skipping the echo statement. When $i is odd, the echo statement is executed, displaying the value of $i followed by a space character.
26. What is the output of the following code snippet?
$name = "John";
switch ($name) {
case "John":
echo "Hello John!";
break;
case "Jane":
echo "Hello Jane!";
break;
default:
echo "Hello!";
}
Explanation: The output will be Hello John!. In this code, a switch statement is used to match the value of $name against different cases. In this case, since $name is "John", the first case is matched. The code block within that case is executed, which displays the string "Hello John!" using the echo statement. The break statement is used to exit the switch statement once a match is found, so the code execution does not continue to the subsequent cases.
27. What is the output of the following code snippet?
$number = 3;
for ($i = 1; $i <= 5; $i++) {
echo $i . " ";
if ($i == $number) {
break 2;
}
}
Explanation: The output will be 1 2 3. In this code, a for loop is used to iterate from $i = 1 to $i <= 5, incrementing $i by 1 in each iteration. Within the loop, the value of $i is displayed using the echo statement. The if statement checks if $i is equal to $number. If it is, the break 2 statement is executed, which breaks out of both the inner loop and the outer loop. Therefore, when $i is 3, the condition is met, and the loops are terminated. The output includes the numbers 1, 2, and 3.
28. What is the purpose of the continue statement in PHP?
Explanation: The continue statement is used in PHP to skip the remaining code inside a loop for the current iteration and move on to the next iteration. It allows you to control the flow of execution within a loop based on certain conditions. When the continue statement is encountered, the loop immediately jumps to the next iteration without executing any code below it for the current iteration.
29. How does the continue statement differ from the break statement in PHP?
Explanation: The continue statement and the break statement serve different purposes in PHP loops. While continue is used to skip the remaining code in the current iteration and move to the next iteration, break is used to exit the loop entirely and continue with the code execution after the loop.
30. In which loop structures can the continue statement be used in PHP?
Explanation: The continue statement can be used with for, foreach, while, and do-while loops in PHP. It allows you to skip the remaining code within the loop for the current iteration and move on to the next iteration.
31. What happens if the continue statement is used outside of a loop in PHP?
Explanation: If the continue statement is used outside of a loop in PHP, it will cause a fatal error because the continue statement is designed to be used within loop structures. Using it outside of a loop will result in a syntax error and the script will terminate.
32. What is the output of the following code snippet?
for ($i = 1; $i <= 5; $i++) {
if ($i % 2 == 0) {
continue;
}
echo $i . " ";
}
Explanation: The output will be 1 3 5. In this code, a for loop is used to iterate from $i = 1 to $i <= 5, incrementing $i by 1 in each iteration. Within the loop, an if statement is used to check if $i is divisible by 2 with no remainder. If it is, the continue statement is executed, which skips the remaining code inside the loop for that iteration and moves on to the next iteration. Therefore, when $i is even, the continue statement is executed, skipping the echo statement. When $i is odd, the echo statement is executed, displaying the value of $i followed by a space character.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
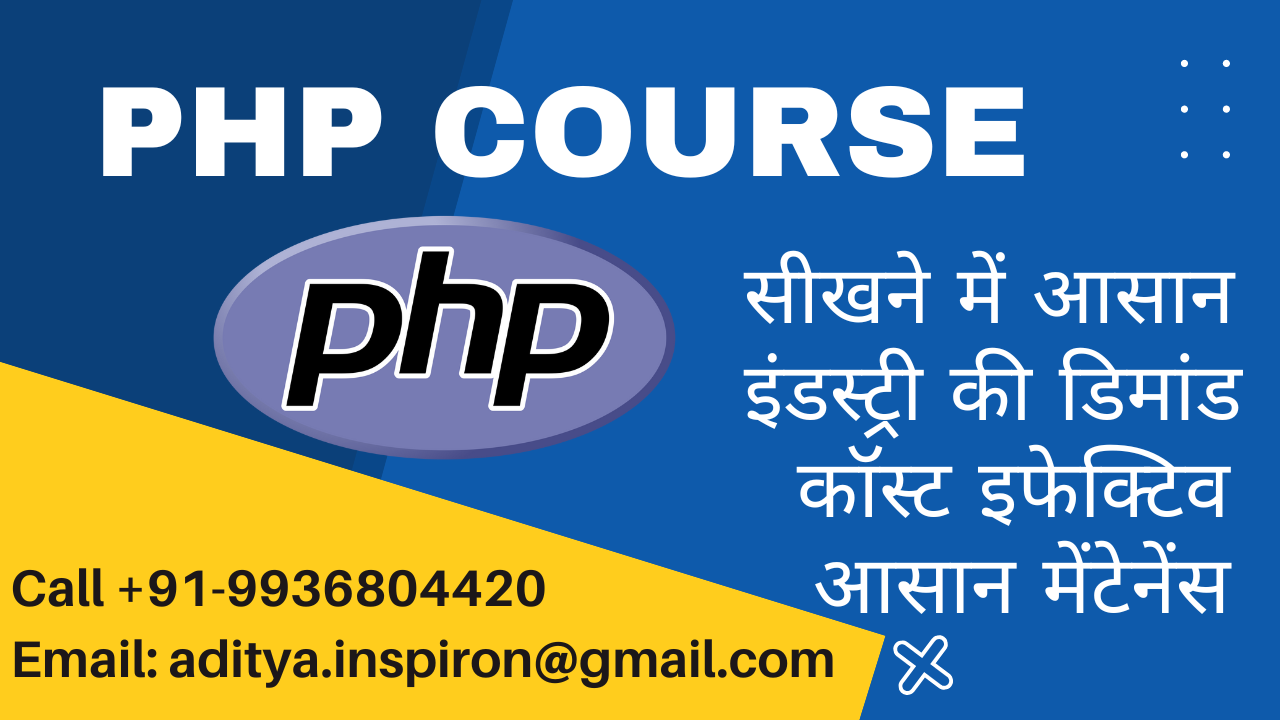
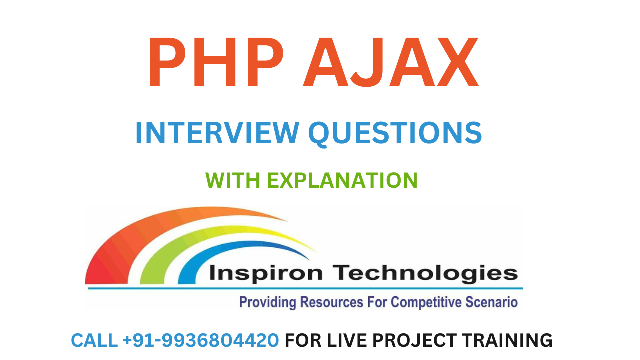
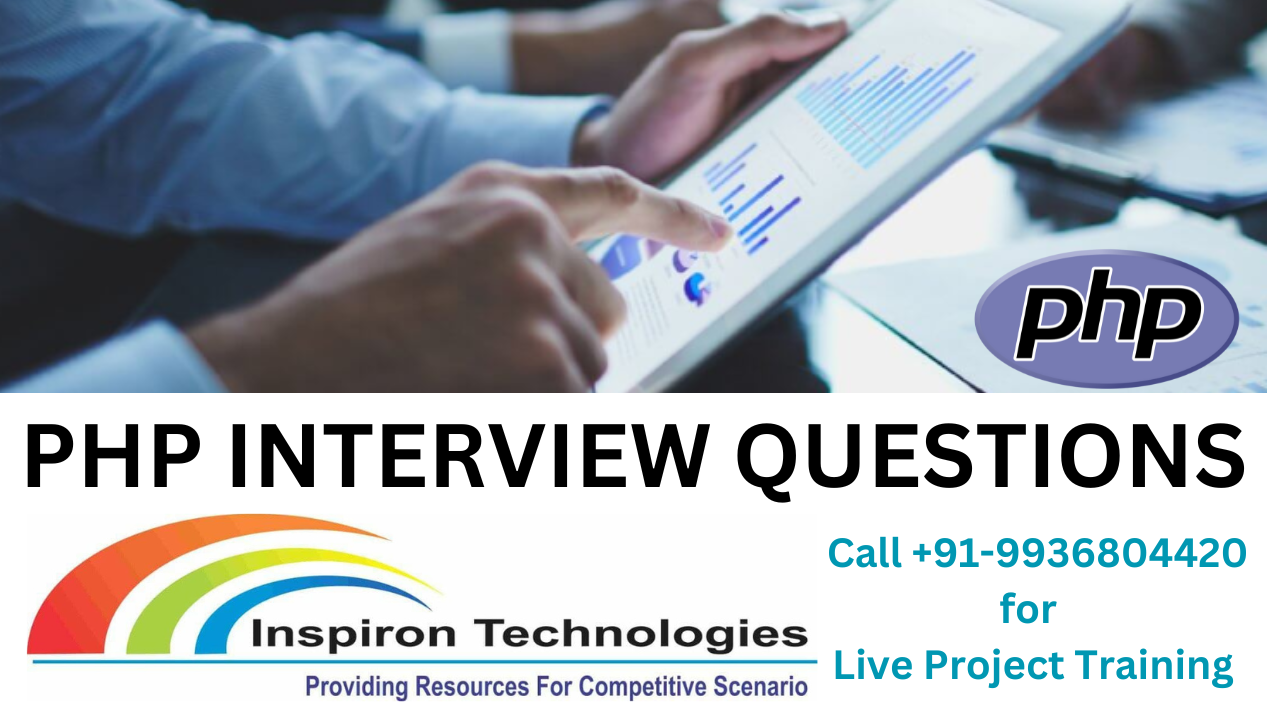
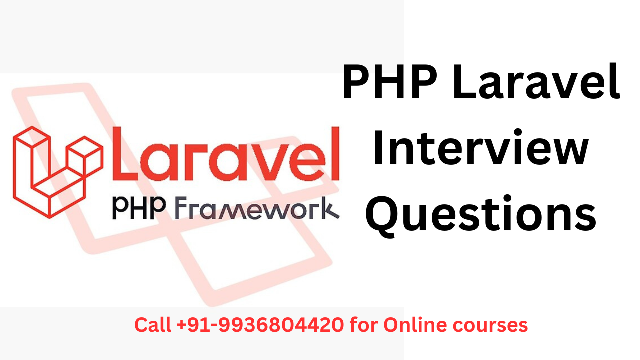
.png)
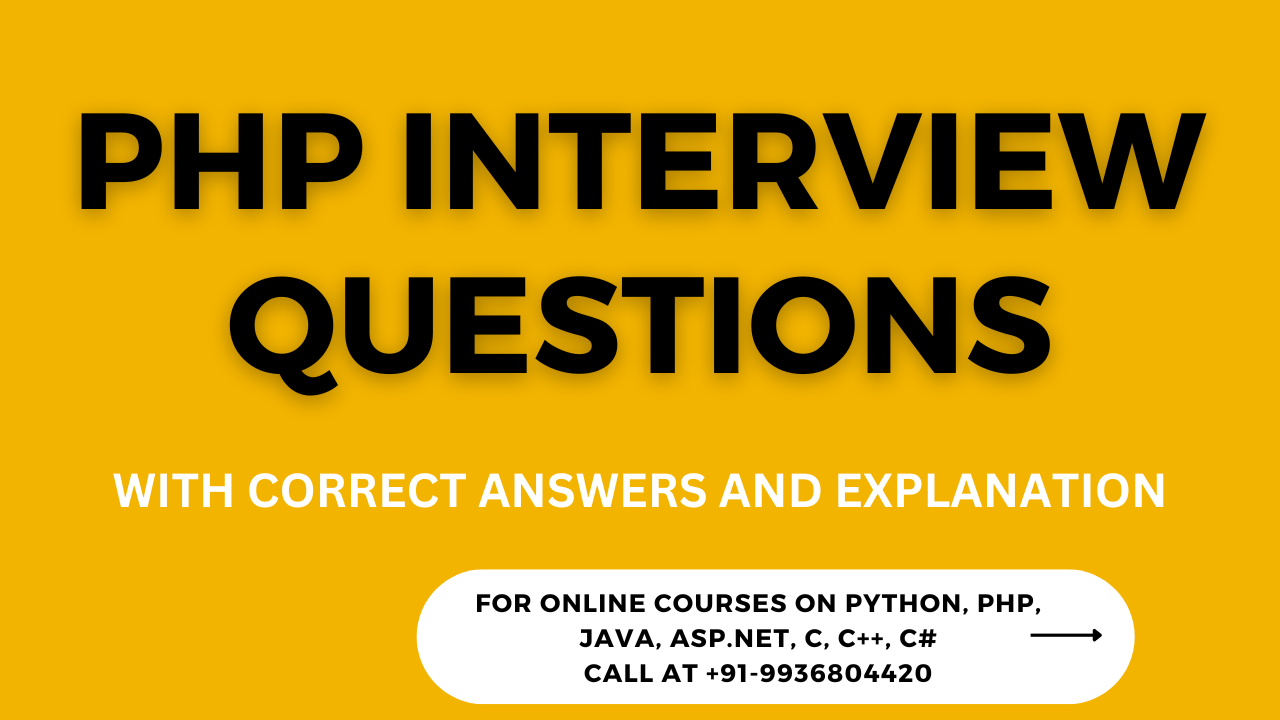
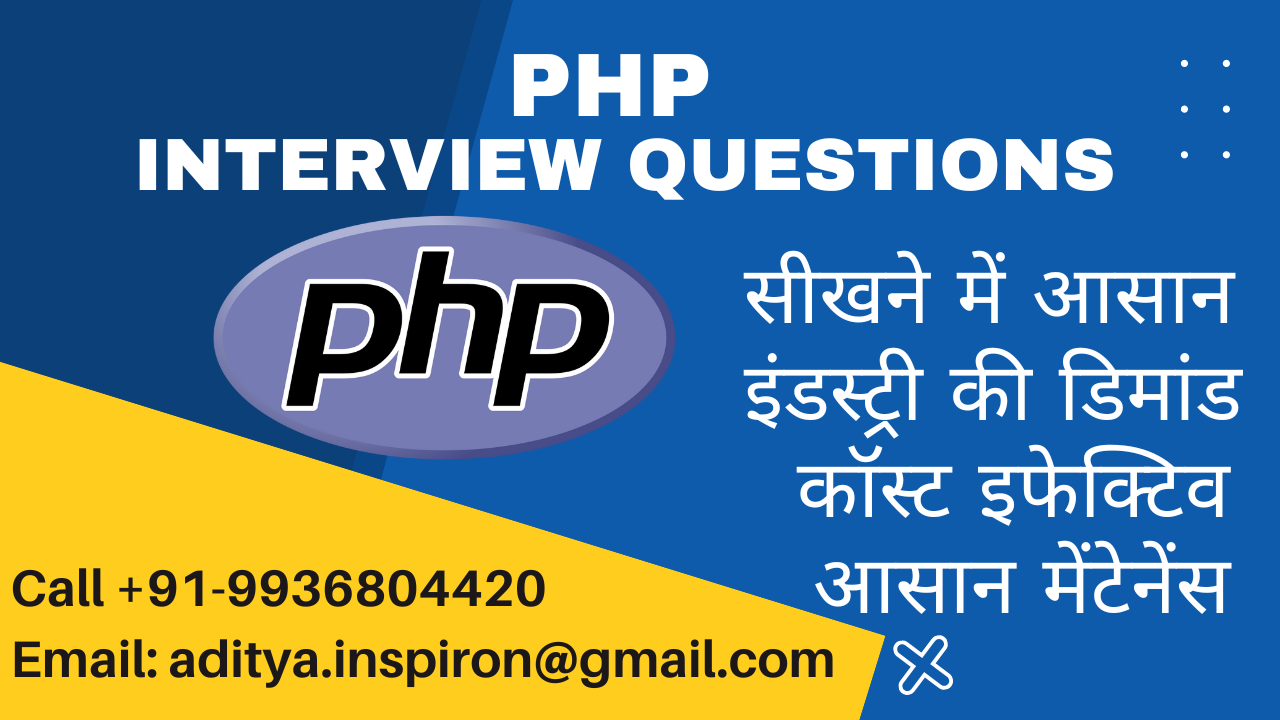
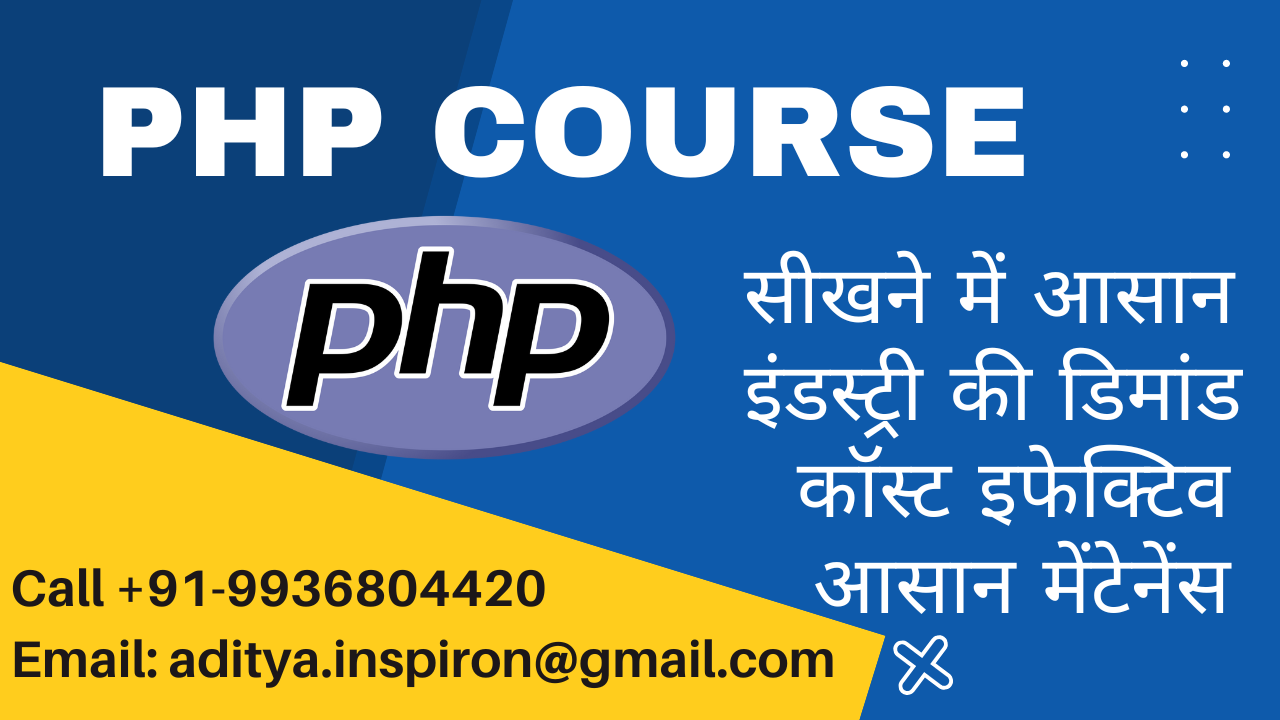
Categories
Popular Post
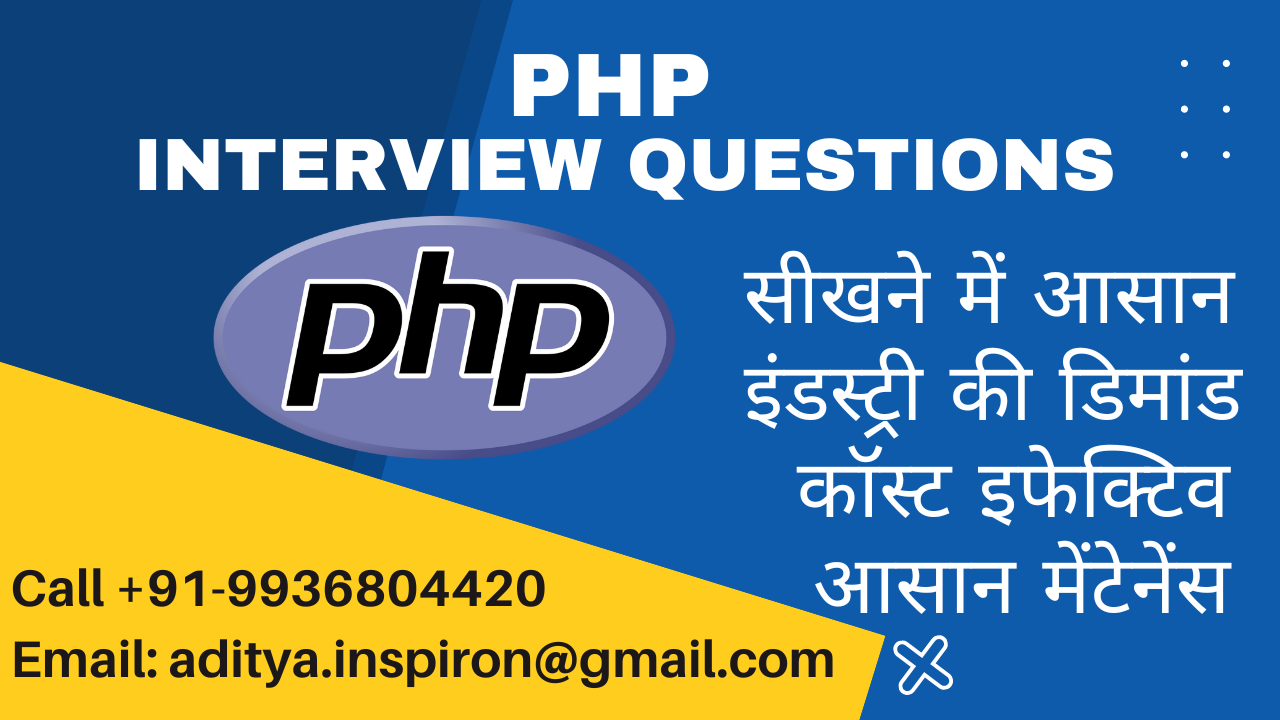
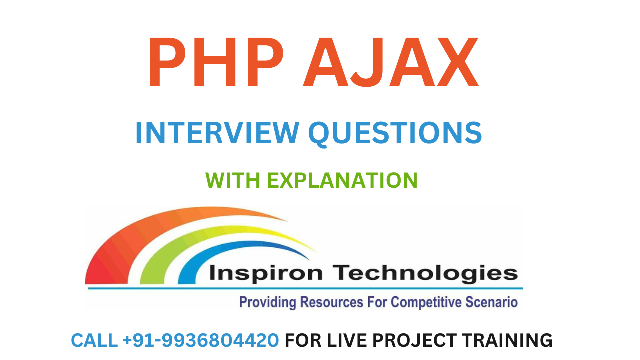
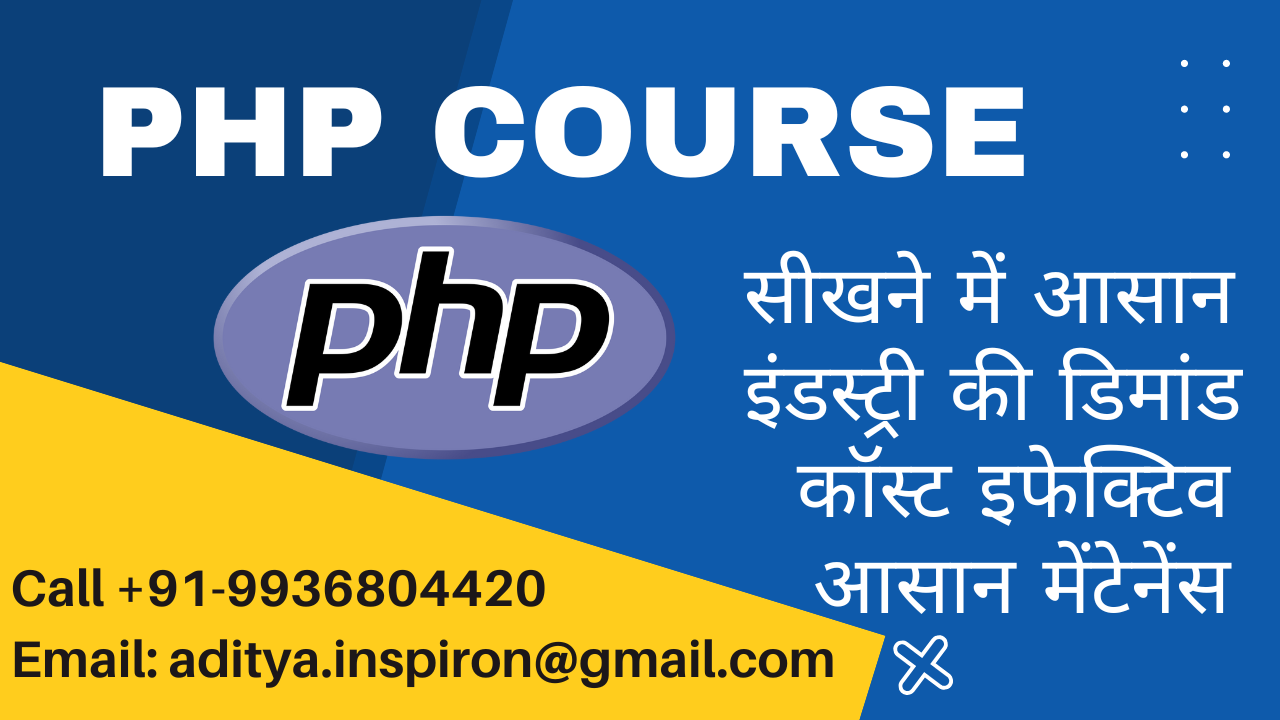
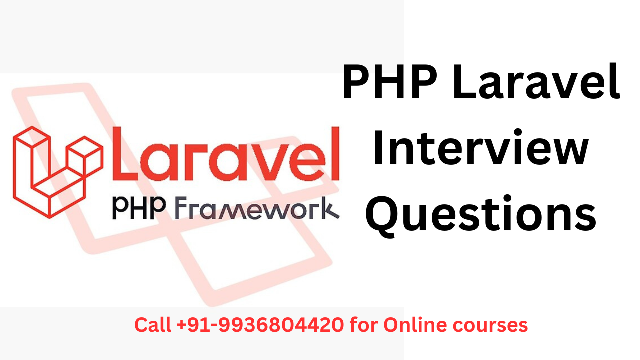
.png)
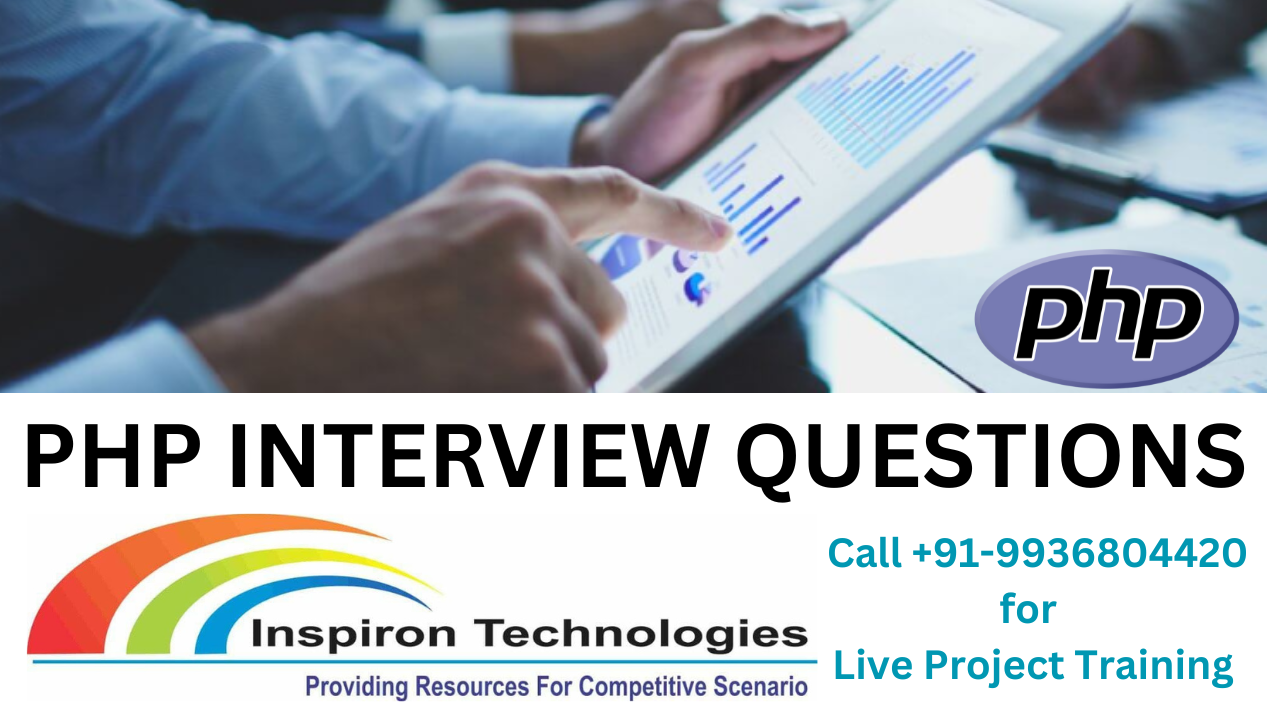
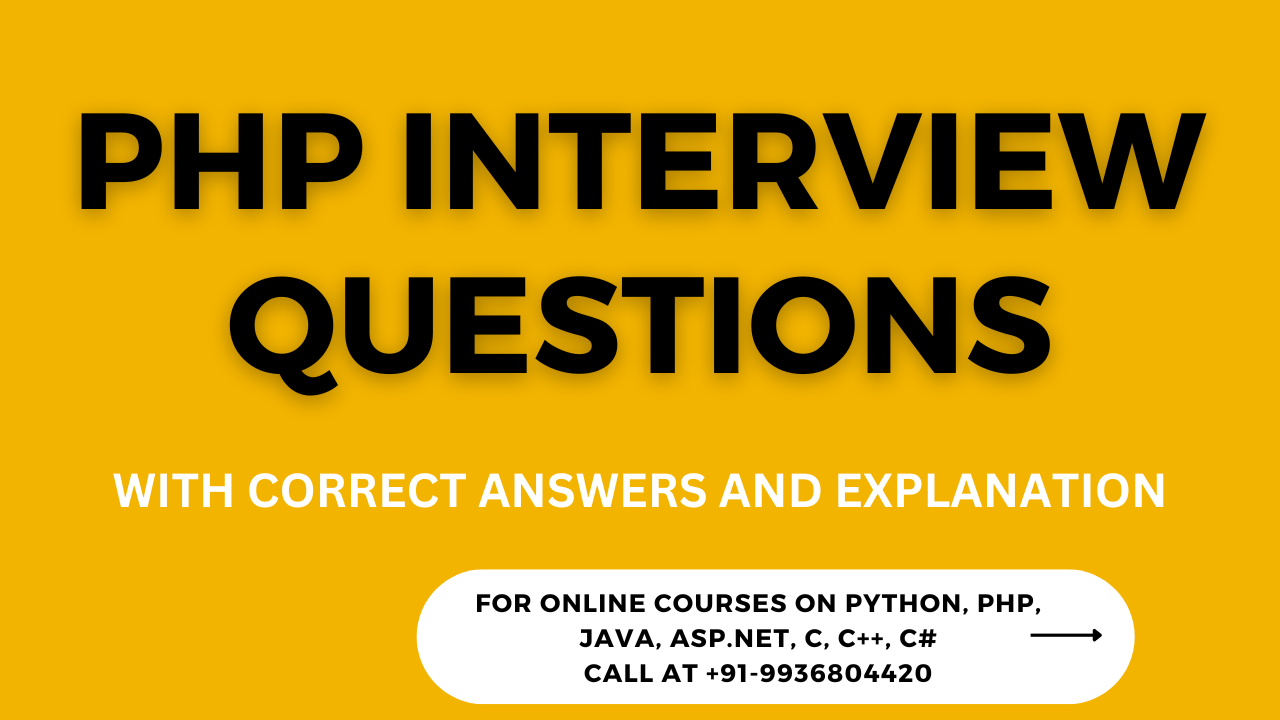
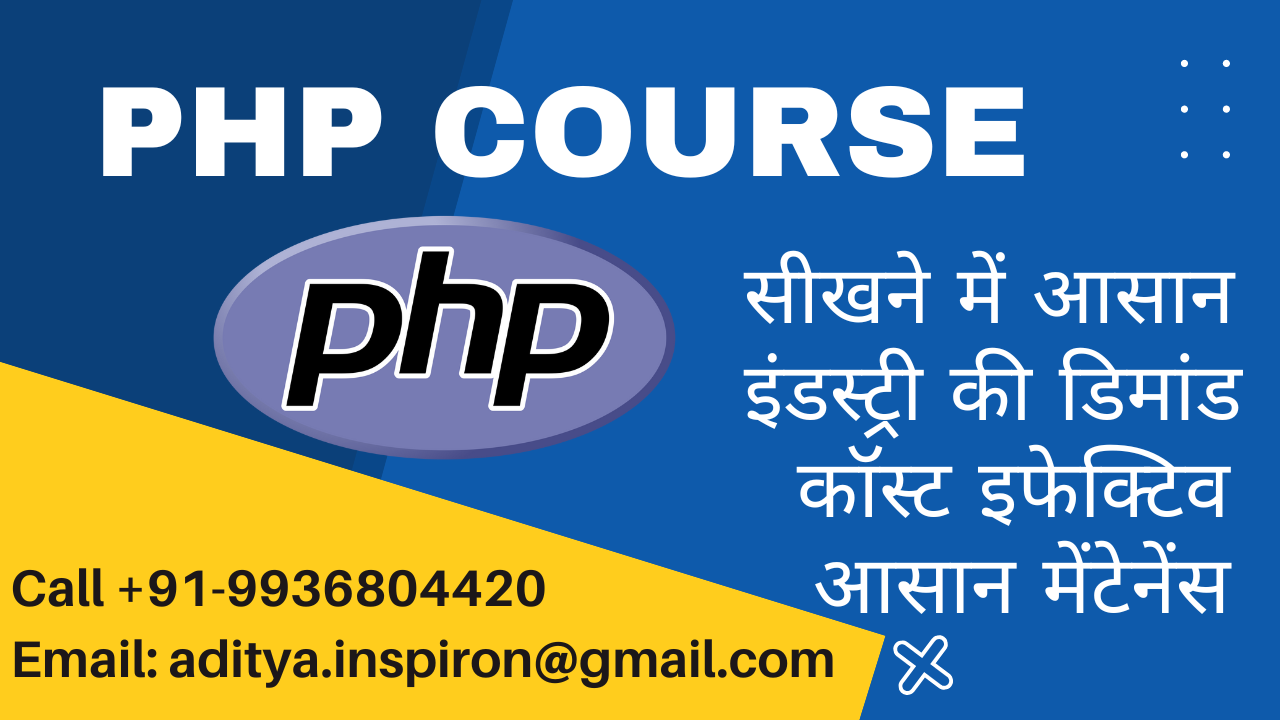
Leave a comment