Django Interview Questions
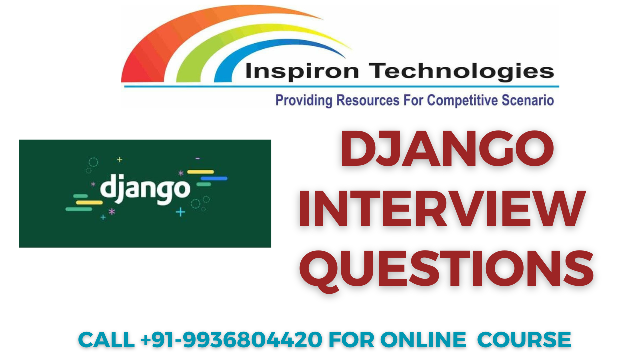
Django Interview Questions with Explanation
1. What is Django?
Answer: Django is a high-level Python web framework that enables the development of fast and secure web applications with minimal code.
Explanation: Django provides several features, such as a powerful Object-Relational Mapping (ORM) system, a robust URL routing system, built-in security features, and a templating system. These features allow developers to quickly build complex web applications while adhering to best practices.
2. What is a model in Django?
Answer: A model in Django is a Python class that defines the structure and behavior of data in a database.
Explanation: Models are used to create, read, update, and delete data in a database. They represent tables in the database and are defined in Python code. Models are created by extending the Django base class models.Model.
3. What is an ORM in Django?
Answer: An ORM (Object-Relational Mapping) in Django is a technique for mapping data from an object-oriented programming language, such as Python, to a relational database.
Explanation: Django's ORM allows developers to interact with a database using Python code instead of SQL. This makes it easier to work with databases and reduces the amount of code required to perform database operations.
4. What is a template in Django?
Answer: A template in Django is a text file that defines the structure and layout of a web page.
Explanation: Templates are used to generate HTML pages dynamically. They can contain placeholders for dynamic content, such as data from a database or user input. Django's template system provides several features, such as template inheritance, filters, and tags, to make it easier to create complex templates.
5. What is a view in Django?
Answer: A view in Django is a Python function that handles HTTP requests and returns an HTTP response.
Explanation: Views are the main entry point for handling HTTP requests in Django. They are responsible for processing user input, interacting with a database, and returning a response to the client. Views can be defined as functions or classes, and they can be decorated with various decorators to add functionality, such as authentication or caching.
7. What is middleware in Django?
Answer: Middleware in Django is a component that sits between the web server and the view, allowing developers to modify requests and responses.
Explanation: Middleware can be used to perform a variety of tasks, such as authentication, logging, and caching. Middleware is defined as a Python class that implements one or more methods, such as process_request or process_response, which are called by Django during the request/response cycle.
8. What is the Django admin site?
Answer: The Django admin site is a built-in web-based interface for managing a Django application's data.
Explanation: The admin site provides a user-friendly interface for performing common administrative tasks, such as creating, reading, updating, and deleting data. The admin site is customizable and can be extended with custom views, forms, and templates.
9. What is a form in Django?
Answer: A form in Django is a Python class that represents an HTML form and provides validation and processing of form data.
Explanation: Forms are used to collect and validate user input. They can be defined as classes that inherit from Django's built-in forms.Form or forms.ModelForm classes. Forms provide several features, such as field validation, automatic HTML rendering, and error handling.
10. What is Django REST framework?
Answer: Django REST framework is a third-party package that allows developers to build APIs for their Django applications.
Explanation: Django REST framework provides a set of tools and conventions for building APIs, such as serializers for converting model instances to JSON or XML, authentication and permission classes for controlling access to API endpoints, and support for various HTTP methods, such as GET, POST, PUT, and DELETE.
11. What is a Django project?
Answer: A Django project is a collection of settings and configurations for a web application.
Explanation: A Django project consists of one or more applications, which can be reused across different projects. To create a Django project, you can use the django-admin startproject command. This command creates a project directory with a settings file and a main URL configuration file. You can then add applications to the project using the python manage.py startapp command. Once the project and applications are set up, you can define models, views, templates, and URLs to build a complete web application.
12. What is a Django app?
Answer: A Django app is a standalone module that performs a specific functionality in a Django project.
Explanation: Django apps are reusable components that can be plugged into multiple Django projects. They typically contain models, views, templates, and static files related to a specific feature or functionality of a web application.
13. What is the difference between a Django project and a Django app?
Answer: A Django project is a collection of settings and configurations for a web application, while a Django app is a standalone module that performs a specific functionality in a Django project.
Explanation: A Django project provides the overall structure and configuration for a web application, while a Django app is a modular component that can be reused across multiple projects.
14. What is a virtual environment in Django?
Answer: A virtual environment in Django is an isolated Python environment that allows developers to install and manage packages without affecting the system's global Python environment.
Explanation: Virtual environments in Django help manage package dependencies and ensure consistency across different projects.
15. What is the Django shell?
Answer: The Django shell is an interactive Python environment that allows developers to interact with the Django ORM and perform database operations.
Explanation: The Django shell can be accessed by running the python manage.py shell command. It provides a command-line interface to interact with the Django ORM and execute Python code.
16. What is the Django testing framework?
Answer: The Django testing framework is a set of tools and utilities provided by Django to write and run tests for Django applications.
Explanation: The Django testing framework provides several utilities, such as the TestCase class, which allows developers to write unit tests for models, views, and forms. It also provides tools for testing views and templates, testing client-server interactions, and generating test data.
17. What is a migration in Django?
Answer: A migration in Django is a file that contains instructions for changing the database schema to match the current state of the models.
Explanation: Migrations are generated automatically by Django whenever a change is made to a model. They are used to apply or revert database schema changes during the development and deployment of a Django application.
18. What is the purpose of the Django URL dispatcher?
Answer: The Django URL dispatcher is responsible for mapping URLs to view functions.
Explanation: The URL dispatcher is defined in the urls.py file of a Django app or project. It matches incoming URLs with patterns defined in the URLconf and calls the corresponding view function to generate a response.
19. What is a Django signal?
Answer: A Django signal is a notification that one part of a Django application sends to another part of the application when a specific event occurs.
Explanation: Signals allow decoupling of different parts of a Django application and can be used to perform tasks asynchronously or trigger custom behavior.
20. What is CSRF protection in Django?
Answer: CSRF (Cross-Site Request Forgery) protection in Django is a security feature that prevents unauthorized requests to a web application.
Explanation: CSRF protection in Django involves adding a hidden input to forms that contains a CSRF token. When a form is submitted, Django checks that the CSRF token matches the one in the session to ensure that the request is legitimate.
21. What is caching in Django?
Answer: Caching in Django is the process of storing the results of expensive operations in memory or on disk to improve the performance of a web application.
Explanation: Django provides several caching backends, such as memcached and Redis, that can be used to cache database queries, rendered templates, or other expensive operations.
22. What is middleware in Django and how is it used?
Answer: Middleware in Django is a component that sits between the web server and the view, allowing developers to perform operations on requests and responses before they are processed by the view.
Explanation: Middleware in Django is used to perform tasks such as authentication, CSRF protection, caching, compression, or logging. It is defined in the MIDDLEWARE setting of a Django project and is executed in the order they are defined.
23. What is the purpose of the Django admin interface?
Answer: The Django admin interface is a web-based tool that provides a user interface for managing the data and functionality of a Django application.
Explanation: The Django admin interface is built using the Django ORM and allows authorized users to perform CRUD (Create, Read, Update, Delete) operations on the data of the application. It can be customized using ModelAdmin classes and templates.
24. What is the difference between a GET request and a POST request in Django?
Answer: A GET request in Django is used to retrieve data from a web server, while a POST request is used to submit data to a web server for processing.
Explanation: GET requests in Django typically result in read-only operations, such as retrieving data from a database or rendering a template. POST requests, on the other hand, are used to modify data on the server, such as adding or updating records in a database.
25. What is Django REST framework?
Answer: Django REST framework is a powerful and flexible toolkit for building Web APIs.
Explanation: Django REST framework allows developers to build Web APIs using the Django framework. It provides a set of powerful and flexible tools for authenticating, serializing, and validating data, as well as for handling requests and responses.
27. What is a serializer in Django REST framework?
Answer: A serializer in Django REST framework is a class that translates complex data types, such as Django models, into JSON or other formats that can be sent over HTTP.
Explanation: Serializers in Django REST framework provide a way to convert Django models and other complex data types into simple representations that can be easily serialized and deserialized. They can also perform validation and handle nested data structures.
28. What is pagination in Django REST framework?
Answer: Pagination in Django REST framework is a mechanism for breaking down large sets of data into smaller, more manageable chunks.
Explanation: Pagination in Django REST framework allows clients to request a subset of a large data set by specifying a page number and a page size. It can improve the performance and usability of a Web API by reducing the amount of data that needs to be transferred over the network.
29. What is the purpose of the Django settings file?
Answer: The Django settings file contains the configuration settings for a Django project, such as database settings, middleware, installed apps, and security settings.
Explanation: The Django settings file is typically named settings.py and is located in the root directory of a Django project. It is used to configure various aspects of a Django application, including its database, middleware, installed apps, and security settings.
30. What is the purpose of the Django context processors?
Answer: The Django context processors are functions that add data to the context of a template, making it available to all templates rendered with that context.
Explanation: Context processors in Django are defined as functions that take a request object as input and return a dictionary of data to be added to the template context. They are registered in the context_processors setting of a Django project.
31. What is the Django Debug Toolbar?
Answer: The Django Debug Toolbar is a third-party package that provides a set of panels displaying various debug information about the current request/response.
Explanation: The Django Debug Toolbar is a popular third-party package that provides a range of panels showing debug information about the current request/response, including SQL queries, cache hits/misses, template rendering times, and HTTP headers.
32. What is the purpose of Django migrations?
Answer:The purpose of Django migrations is to manage changes to the database schema over time, allowing developers to evolve the data model of a Django application in a safe and structured way.
Explanation: Django migrations are a system for managing database schema changes in a structured and safe way. They allow developers to define changes to the database schema using Python code and then apply those changes to the database in a way that preserves existing data and ensures consistency. Migrations can be used to add new tables, modify existing tables, add or remove columns, and perform other database schema changes. They are generated automatically by Django based on changes to the models defined in the application.
33. What is the difference between a Django project and a Django application?
Answer: A Django project is a collection of settings and configurations for a specific website, while a Django application is a module that provides specific functionality for a Django project.
Explanation: A Django project is a top-level directory that contains settings, configurations, and other files for a specific website or web application. A Django application, on the other hand, is a self-contained module that provides specific functionality for the project, such as user authentication, blog posts, or product reviews.
34. What is Django's ORM?
Answer: Django's ORM (Object-Relational Mapping) is a powerful tool that allows developers to interact with databases using Python objects.
Explanation: Django's ORM provides a high-level, Pythonic interface to interact with databases. It allows developers to define database models as Python classes, and then interact with those models using a set of methods provided by the ORM. This makes it easy to create, read, update, and delete data from databases, without needing to write SQL queries directly.
35. What is the Django template language?
Answer: The Django template language is a simple and powerful language used to create dynamic HTML templates.
Explanation: The Django template language provides a simple and powerful syntax for creating dynamic HTML templates. It allows developers to insert variables, loops, conditionals, and other control structures into HTML templates, making it easy to create complex and dynamic web pages.
Categories
Popular Post
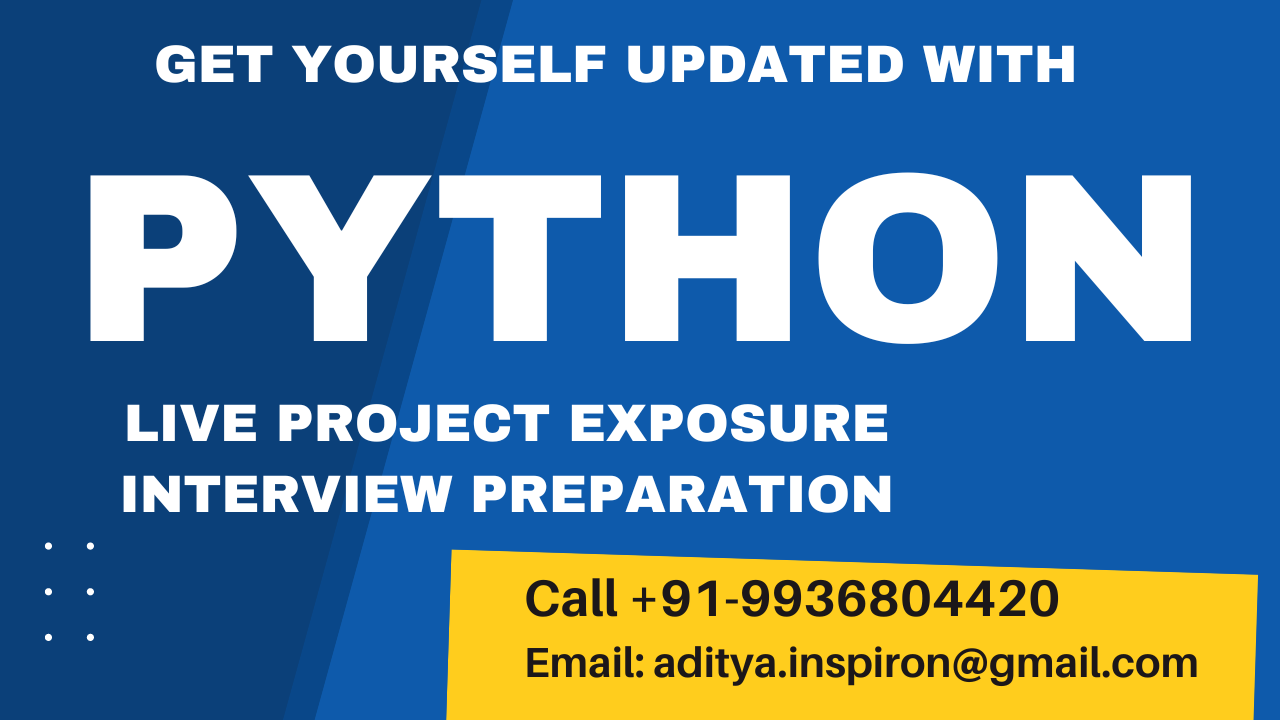
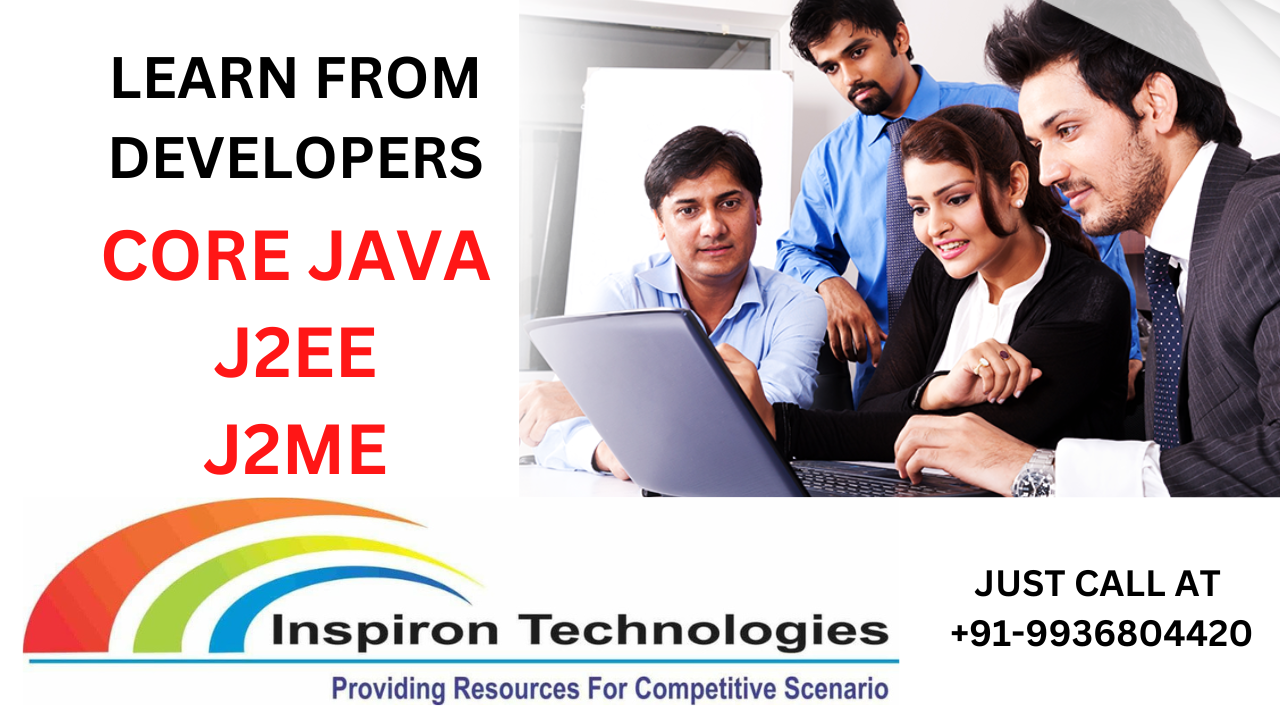
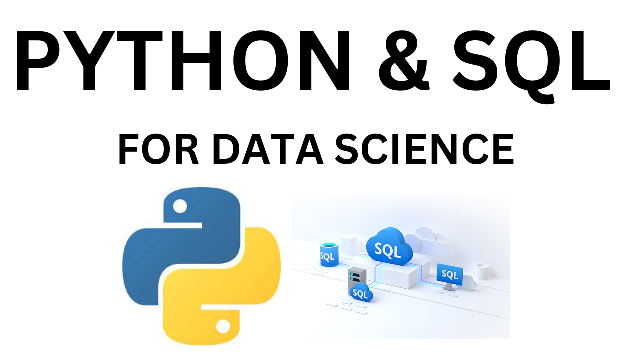
Leave a comment