Node JS Interview Questions with Explanation
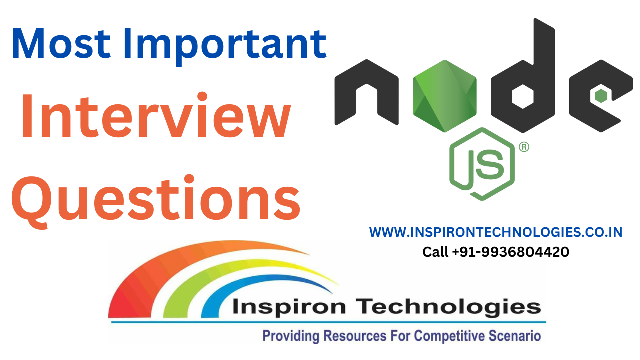
Most Important Interview Questions of Node JS
1. What is Node.js?
Node.js is an open-source runtime environment built on Chrome's V8 JavaScript engine. It allows developers to run JavaScript code outside of a web browser, enabling server-side and networking applications.
2. What are the key features of Node.js?
Node.js has several key features, including:
Asynchronous and event-driven programming model
Non-blocking I/O operations
Single-threaded, but with support for concurrency through event loop
Scalability and high performance
Large and active ecosystem of libraries and packages (npm)
3. What is an event loop in Node.js?
The event loop is a crucial component of Node.js. It handles and dispatches events, ensuring non-blocking, asynchronous behavior. It allows Node.js to perform I/O operations efficiently by delegating them to the operating system and then handling the results once they are ready.
4. Explain the concept of blocking and non-blocking operations in Node.js?
In a blocking operation, the application is halted until the operation completes. In contrast, non-blocking operations allow the application to continue executing other tasks while waiting for the operation to complete. Node.js employs non-blocking I/O operations, allowing multiple requests to be processed concurrently and maximizing resource utilization.
5. What is npm?
npm (Node Package Manager) is the default package manager for Node.js. It provides a vast repository of reusable JavaScript packages and modules. npm allows developers to easily install, manage, and update dependencies for their Node.js projects.
6. How does Node.js handle child threads or multiple cores?
Node.js uses a single-threaded event loop model, but it can take advantage of multiple cores through child processes. The
child_process
module in Node.js provides functionality to create and manage child processes. By utilizing the cluster
module or a process manager like PM2, developers can take advantage of multiple cores and distribute the workload effectively.
7. What is the purpose of the
module.exports
object in Node.js?In Node.js, the
module.exports
object is used to define the public interface of a module. It allows you to expose functions, objects, or values that can be accessed by other modules using the require
function. By assigning properties to module.exports
, you make them available for other parts of your application.
8. Explain the concept of middleware in Express.js.
Express.js is a popular web application framework for Node.js. Middleware functions in Express.js sit between the incoming request and the final handler. They can modify the request or response objects, perform additional processing, or terminate the request-response cycle. Middleware functions are often used for tasks such as authentication, logging, error handling, and routing.
9. How can you handle errors in Node.js?
Node.js provides the
try-catch
statement for handling synchronous errors. For asynchronous code, you can use the error-first callback pattern, where the first parameter of the callback function is reserved for an error object. Promises and async/await syntax also offer error handling mechanisms. Additionally, frameworks like Express.js provide middleware for error handling.
10. What is the purpose of the
Buffer
class in Node.js?The
Buffer
class in Node.js is used to handle binary data. It allows you to work with raw binary data, such as reading from or writing to streams or interacting with network protocols. Buffers are essentially fixed-size chunks of memory that can store binary data in various encodings, such as UTF-8 or Base64.What is the purpose of the
exports
object in Node.js? In Node.js, the exports
object is a reference to module.exports
. It allows you to add properties or methods directly to exports
, making them available for other modules when importing. However, unlike module.exports
, you cannot assign a new object directly to exports
.
11. What are streams in Node.js?
Streams are a core concept in Node.js for handling continuous data flow, especially when dealing with large amounts of data or network communication. Streams provide an abstraction layer to read from or write to a source incrementally, without loading the entire data into memory at once. There are several types of streams in Node.js, including readable, writable, duplex, and transform streams.
12. What is the difference between
setImmediate()
and setTimeout()
in Node.js?Both
setImmediate()
and setTimeout()
are used to schedule code execution in Node.js, but they have slight differences. When invoked, setImmediate()
executes the callback function after the I/O events in the current event loop iteration, while setTimeout()
schedules the callback function to be executed after a specified delay, regardless of the I/O events. In other words, setImmediate()
has a higher priority than setTimeout()
.
13. How can you handle file uploads in Node.js?
To handle file uploads in Node.js, you can use middleware such as
multer
or formidable
. These libraries help parse incoming form data and handle file uploads. They provide an API to access the uploaded files, validate them, and store them on the server. By configuring the appropriate middleware, you can handle file uploads seamlessly in your Node.js applications.
14. Explain the concept of Promises in Node.js?
Promises in Node.js are a way to handle asynchronous operations and manage their results. A Promise represents a value that may not be available yet but will be resolved or rejected in the future. Promises provide a clean syntax for handling asynchronous code, using methods like
.then()
and .catch()
. They help avoid callback hell and make asynchronous code more readable and maintainable.
15. What is clustering in Node.js?
Clustering is a technique used in Node.js to leverage the capabilities of multiple CPU cores. By creating a cluster of worker processes, each running on a separate core, you can distribute the workload and handle more concurrent requests. The
cluster
module in Node.js provides an API for creating and managing clusters, allowing you to scale your Node.js applications effectively.
16. How does error handling work in Express.js?
In Express.js, error handling is done through middleware functions. When an error occurs in an Express application, you can define a middleware function with four parameters (
err
, req
, res
, next
) to handle the error. This middleware should be defined after all other routes and middleware. By calling next(err)
, you can pass the error to this error-handling middleware, where you can customize the error response or perform logging.
17. Explain the concept of WebSocket and its usage in Node.js.
WebSocket is a communication protocol that provides full-duplex communication channels over a single TCP connection. It enables real-time, bidirectional communication between clients and servers. In Node.js, libraries like
socket.io
provide support for WebSocket connections, allowing you to build real-time applications such as chat systems, collaborative tools, and live dashboards.
18. What is the role of the
__dirname
variable in Node.js?__dirname
is a global variable in Node.js that represents the current directory name of the current module file. It provides the absolute path of the directory containing the currently executing script. You can use __dirname
to construct file paths relative to the current module file, regardless of the working directory.
19. How can you improve the performance of a Node.js application?
There are several techniques to improve the performance of a Node.js application, such as:
Caching: Implementing caching mechanisms to avoid redundant computations or I/O operations.
Using efficient algorithms and data structures.
Optimizing database queries and using appropriate indexes.
Scaling horizontally by distributing the workload across multiple instances or servers.
Employing a load balancer to evenly distribute incoming requests.
Profiling and optimizing code using tools like Node.js Profiler or performance monitoring tools.
20. What is the purpose of the
process
object in Node.js?The
process
object is a global object in Node.js that provides information and control over the current Node.js process. It allows you to access command-line arguments, environment variables, exit the process, listen to process events, and more. The process
object is commonly used for process management and interaction in Node.js applications.
21. How can you handle cross-origin resource sharing (CORS) in Node.js?
To handle CORS in Node.js, you can use the
cors
middleware. The cors
middleware adds the necessary headers to the HTTP responses, allowing cross-origin requests to be processed by the browser. By configuring the middleware, you can define the allowed origins, methods, headers, and other CORS-related settings.
22. What is the purpose of the
crypto
module in Node.js?The
crypto
module in Node.js provides cryptographic functionality, including secure hash algorithms, encryption, decryption, signing, and verification. It allows you to perform various cryptographic operations such as generating hashes, encrypting data, creating digital signatures, and more. The crypto
module is often used for data security and authentication purposes.
23. How can you handle environment-specific configuration in Node.js?
To handle environment-specific configuration, you can use configuration files or environment variables. Configuration files (such as JSON, YAML, or .env files) can store different configuration settings for each environment (e.g., development, production). Alternatively, you can use environment variables to store and access configuration values directly from the operating system. Node.js provides the
process.env
object to access environment variables.
24. Explain the concept of middleware in the context of Connect.js.
Connect.js is a middleware framework that is often used as the foundation for Express.js. Middleware in Connect.js are functions that have access to the
req
(request) and res
(response) objects and the ability to invoke the next middleware function in the stack. Connect.js middleware can be used to perform tasks such as authentication, logging, compression, and more.
25. What are the differences between EventEmitter and Streams in Node.js?
EventEmitter and Streams are both core concepts in Node.js, but they serve different purposes. EventEmitter is a mechanism for implementing the observer pattern, allowing objects to emit named events and register listeners to those events. Streams, on the other hand, are a way to handle continuous data flow. Streams provide an abstraction for reading from or writing to a source incrementally, without loading the entire data into memory at once.
26. What is the purpose of the
net
module in Node.js?The
net
module in Node.js provides an asynchronous network API, allowing you to create TCP or UNIX socket servers and clients. It provides functionality for creating network servers, listening for connections, sending and receiving data, and handling socket events. The net
module is commonly used for building network-related applications such as chat servers, real-time data exchange, and more.
27. How can you handle file I/O in Node.js?
Node.js provides several built-in modules for handling file I/O, such as
fs
(File System) and path
. The fs
module allows you to read from and write to files, manipulate directories, and perform various file-related operations. The path
module provides utilities for working with file and directory paths, resolving file paths, and handling file extensions.
28. What is the purpose of the
url
module in Node.js?The
url
module in Node.js provides utilities for working with URLs. It allows you to parse URL strings, extract components like protocol, hostname, path, query parameters, and construct URLs from individual components. The url
module is commonly used for URL manipulation, routing, and handling in Node.js applications.
29. How can you handle asynchronous errors in Node.js?
To handle asynchronous errors in Node.js, you can use error-first callbacks, Promises, or async/await syntax. With error-first callbacks, the first parameter of the callback function is reserved for an error object. For Promises, you can use
.catch()
to handle errors, and with async/await, you can wrap the code in a try-catch block. Additionally, you can use tools like try...catch
in conjunction with the domain
module to handle errors across multiple asynchronous operations.
30. What is the purpose of the
cluster
module in Node.js?The
cluster
module in Node.js allows you to create and manage a cluster of worker processes. It enables you to take advantage of multi-core systems by forking multiple instances of your Node.js application. Each worker runs in a separate process, and the master process handles load balancing and communication between the workers. This approach improves scalability and performance of your Node.js application.
31. Explain the concept of garbage collection in Node.js?
Garbage collection is the automatic memory management process in Node.js. It tracks objects and variables in memory that are no longer in use and frees up the memory occupied by those objects. Node.js uses a mark-and-sweep algorithm for garbage collection, where it identifies and marks active objects and sweeps away the unused ones. This process helps prevent memory leaks and optimizes memory usage in Node.js applications.
32. What is the purpose of the
os
module in Node.js?The
os
module in Node.js provides operating system-related utility functions. It allows you to access information about the operating system, such as CPU architecture, network interfaces, free memory, and more. The os
module is useful for gathering system-related data and making decisions or optimizations based on the underlying operating system.
33. How can you handle sessions in Node.js?
To handle sessions in Node.js, you can use middleware like
express-session
. The express-session
middleware manages session data and maintains session state between HTTP requests. It uses a session ID stored as a cookie or passed in the URL to identify and associate session data with a specific user. You can configure the session middleware to store session data in memory, a database, or other storage mechanisms.
34. What is the purpose of the
child_process
module in Node.js?The
child_process
module in Node.js provides functionality to create and interact with child processes. It allows you to spawn child processes, execute commands in a separate process, communicate with child processes using standard input/output, and more. The child_process
module is useful when you need to perform tasks that are computationally expensive or require interaction with external processes.
35. Explain the concept of the event-driven architecture in Node.js?
The event-driven architecture is a core principle of Node.js. In an event-driven architecture, the flow of the program is determined by events that are triggered or emitted. Instead of following a sequential execution model, Node.js utilizes an event loop that listens for events and invokes appropriate event handlers or callbacks. This architecture enables non-blocking I/O and allows multiple operations to run concurrently, enhancing the performance and responsiveness of Node.js applications.
36. How can you handle database operations in Node.js?
In Node.js, you can use various libraries and modules to handle database operations. Popular choices include the
mysql
, mongodb
, and pg
modules for relational databases like MySQL, MongoDB, and PostgreSQL, respectively. These modules provide APIs to establish connections, execute queries, handle transactions, and interact with the database. Additionally, object-relational mapping (ORM) libraries like Sequelize or Mongoose provide higher-level abstractions for working with databases.
37. What is the purpose of the
util
module in Node.js?The
util
module in Node.js provides utility functions that are commonly used in Node.js applications. It includes functions for debugging, error handling, object manipulation, and more. For example, the util.promisify
function can be used to convert callback-based functions into Promise-based functions. The util
module is a helpful toolset for simplifying common programming tasks in Node.js.
38. Explain the concept of a microservice architecture in Node.js?
A microservice architecture is an architectural style where applications are built as a collection of small, loosely coupled services. Each microservice focuses on a specific business capability and can be developed, deployed, and scaled independently. In Node.js, you can implement microservices using frameworks like Express.js or Fastify. Node.js, with its lightweight and scalable nature, is well-suited for building microservices that can be easily managed and scaled in distributed systems.
39. What is the purpose of the
process.nextTick()
method in Node.js?The
process.nextTick()
method in Node.js is used to schedule a callback function to be executed asynchronously in the next iteration of the event loop. It allows you to defer the execution of a function until the current execution phase completes. This method is commonly used to ensure that a callback is called as soon as possible, just after the current code block completes execution.
40. What is the purpose of the
Async/Await
syntax in Node.js?The
Async/Await
syntax is a modern approach to handle asynchronous operations in a synchronous manner. It allows you to write asynchronous code that resembles synchronous code, making it easier to read and understand. By using the async
keyword before a function and the await
keyword within the function, you can pause the execution of the function until an asynchronous operation completes and then continue executing the remaining code.We hope that you must have found this exercise quite useful. If you wish to join online courses on Angular JS, Node JS, Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
Leave a comment