Hibernate Interview Questions with Answer
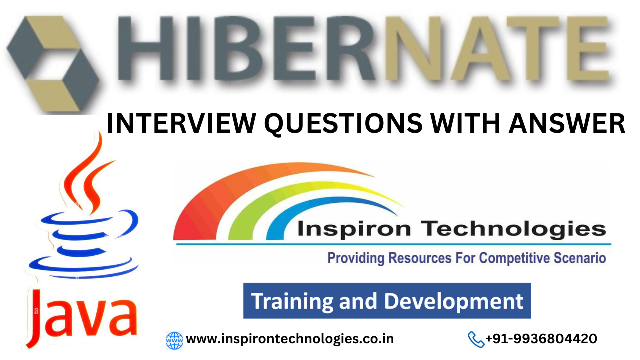
Answer: Hibernate is an open-source, object-relational mapping (ORM) framework for Java that simplifies database interaction by mapping Java objects to database tables and vice versa. Answer: The Java Hibernate FrameWork Interview Questions
1. What is Hibernate?
2. Explain the key features of Hibernate.
Answer: Hibernate offers features like automatic table generation, caching, lazy loading, and a powerful query language (HQL). It provides a high-level, object-oriented API for working with databases.
3. What are the core interfaces of Hibernate?
Answer: The core interfaces of Hibernate are
Session
, SessionFactory
, Configuration
, and Transaction
.
4. What is the role of a SessionFactory in Hibernate?
Answer:
SessionFactory
is a factory class responsible for creating and managing Session
instances. It is a heavyweight object, typically created once during application initialization.
5. Explain the difference between
Session
and SessionFactory
in Hibernate.Answer:
Session
represents a single-threaded unit of work, while SessionFactory
is a factory for creating Session
instances. You typically have one SessionFactory
per application, while you have one Session
per request or unit of work.
6. What is lazy loading in Hibernate?
Answer: Lazy loading is a feature in Hibernate that delays the loading of associated objects until they are explicitly accessed. It can help improve performance by avoiding unnecessary database queries.
7. Explain the difference between eager loading and lazy loading.
Answer: Eager loading fetches associated objects along with the main object in a single query, while lazy loading fetches them only when they are accessed, resulting in separate queries.
8. What is HQL (Hibernate Query Language)?
Answer: HQL is a query language similar to SQL but used to query Hibernate objects. It is object-oriented and understands the relationships between entities in the database.
9. What is a Hibernate mapping file (hbm.xml file)?
Answer: A Hibernate mapping file is an XML file that defines the mapping between Java classes and database tables. It specifies how class properties are mapped to database columns.
10. Explain the concept of cascading in Hibernate.
Answer: Cascading in Hibernate refers to the ability to propagate an operation (e.g., save, update, delete) from a parent entity to its associated child entities. It simplifies the management of object relationships.
11. What is a Hibernate Cache, and why is it used?
Answer: Hibernate provides caching mechanisms to improve application performance by reducing the number of database calls. It includes first-level (session) and second-level (SessionFactory) caches.
12. What is the purpose of the Hibernate
@Entity
annotation?Answer: The
@Entity
annotation is used to mark a Java class as a persistent entity, indicating that instances of the class can be stored in the database.
13. What is the difference between transient, persistent, and detached objects in Hibernate?
Answer:
Transient: Objects not associated with any Hibernate session.
Persistent: Objects associated with a Hibernate session.
Detached: Objects were associated with a session but are no longer in the persistent state.
14. Explain the various ways to perform object-relational mapping in Hibernate.
Answer: Object-relational mapping in Hibernate can be done using XML mapping files, annotations, and the Hibernate Mapping API.
15. What is the purpose of the Hibernate
@Id
annotation?Answer: The
@Id
annotation is used to specify the primary key property of an entity class.
16. What is a Hibernate Proxy, and why is it used?
Answer: A Hibernate proxy is a lazy-loading placeholder for an entity. It allows you to avoid unnecessary database queries until the entity is actually accessed.
17. Explain the concept of a composite key in Hibernate.
Answer: A composite key is a key that consists of multiple columns. In Hibernate, you can map a composite key using the
@EmbeddedId
or @IdClass
annotation.These questions cover a range of Hibernate topics commonly discussed in interviews. Be sure to review the Hibernate documentation and practice working with Hibernate to solidify your understanding.
18. What is the purpose of the Hibernate
@GeneratedValue
annotation?Answer: The
@GeneratedValue
annotation is used to specify how the primary key is generated. It can be used with the @Id
annotation to define various strategies such as IDENTITY
, SEQUENCE
, or TABLE
for key generation.
19. Explain the concept of a unidirectional and bidirectional association in Hibernate.
Answer:
Unidirectional Association: In a unidirectional association, one entity has a reference to another entity, but the other entity does not have a reference back. For example, a
Person
has an Address
reference, but the Address
doesn't reference the Person
.Bidirectional Association: In a bidirectional association, both entities have references to each other. For example, a
Parent
has references to their Children
, and the Children
have a reference back to their Parent
.
20. What is the purpose of the Hibernate
@ManyToOne
and @OneToMany
annotations?Answer: The
@ManyToOne
annotation is used to define a many-to-one association, indicating that the annotated entity belongs to another entity. The @OneToMany
annotation is used to define a one-to-many association on the reverse side.
21. Explain the different inheritance mapping strategies in Hibernate.
Answer: Hibernate supports various inheritance mapping strategies, including Single Table, Joined Subclass, and Table per Concrete Class. Each strategy defines how Java class hierarchies map to database tables.
22. What is the purpose of the Hibernate
@JoinColumn
annotation?Answer: The
@JoinColumn
annotation is used to specify the column used for joining an entity association. It allows you to customize the foreign key column name and other attributes.
23. What is the purpose of the Hibernate
@Cacheable
annotation?Answer: The
@Cacheable
annotation is used to enable or disable caching for a specific entity. When an entity is marked as cacheable, it can be cached in the second-level cache.
24. Explain the difference between a first-level cache and a second-level cache in Hibernate.
Answer:
First-level Cache: The first-level cache is associated with a Hibernate
Session
. It stores objects that are currently being used within a session and provides automatic dirty checking.Second-level Cache: The second-level cache is a shared cache that can be used across multiple sessions and even across different parts of the application. It stores data that is frequently accessed and can improve performance.
25. What is the purpose of the Hibernate
@Version
annotation?Answer: The
@Version
annotation is used to specify a version or timestamp property for optimistic locking. It helps prevent concurrent updates to the same entity by checking if the version has changed during an update operation.
26. Explain the concept of Hibernate DDL auto-generation.
Answer: Hibernate can automatically generate the database schema based on the mapping information provided. You can configure this using the
hibernate.hbm2ddl.auto
property, which can have values like update
, create
, or validate
.
27. What is the purpose of the Hibernate
Criteria
API?Answer: The
Criteria
API is a programmatic and type-safe way to create queries in Hibernate. It allows you to build complex queries using a fluent and object-oriented approach.
28. Explain the role of the Hibernate
Query
interface.Answer: The
Query
interface in Hibernate is used to create and execute HQL or native SQL queries. It allows you to retrieve and manipulate data from the database.
29. What is the purpose of the Hibernate
SessionFactory
openSession() and getCurrentSession() methods?Answer: The
openSession()
method creates a new Session
every time it is called, while getCurrentSession()
is used to obtain the current Session
associated with the current transaction. It is typically used in a managed environment, such as within a Java EE container.
30. Explain the difference between a transient object and a detached object in Hibernate.
Answer:
Transient Object: An object is transient if it is not associated with any Hibernate session. It is not persistent in the database.
Detached Object: An object is detached if it was previously associated with a session but is no longer in that session's scope. Detached objects can be reattached to a session for further processing.
These additional Hibernate interview questions should help you prepare for discussions about Hibernate in depth. Remember to practice and explore Hibernate's various features and capabilities to strengthen your knowledge.
31. What is the purpose of the
SessionFactory
and Session
caches in Hibernate?SessionFactory
cache (second-level cache) stores objects that can be shared across different sessions to improve performance. The Session
cache (first-level cache) stores objects that are specific to a particular session and ensures that changes are tracked within that session.32. Explain the concept of a composite element in Hibernate collections.
Answer: In Hibernate, a composite element is a value type that can be used to represent a collection element. It allows you to map complex structures, such as components or embedded objects, as elements in a collection.
33. What is the purpose of the
@Formula
annotation in Hibernate?Answer: The
@Formula
annotation is used to define a formula or SQL expression that is calculated and returned as a property when querying the entity. It's useful for deriving properties that are not directly stored in the database.
34. What is the purpose of the
@NaturalId
annotation in Hibernate?Answer: The
@NaturalId
annotation is used to mark a property of an entity as a natural identifier. Natural identifiers are used to uniquely identify an entity, and Hibernate can optimize queries using natural IDs.
35. Explain the benefits and drawbacks of using Hibernate in a project.
Answer:
Benefits: Hibernate simplifies database interaction, reduces boilerplate code, and provides a clean object-oriented interface for data access. It helps with portability, caching, and improves developer productivity.
Drawbacks: Hibernate has a learning curve, can lead to performance issues if misused, and might generate complex SQL queries. It may not be suitable for every project.
36. What are the different fetching strategies in Hibernate, and when should they be used?
Answer: Hibernate provides fetching strategies like
EAGER
and LAZY
. EAGER
fetches related entities immediately, while LAZY
defers fetching until accessed. The choice depends on the specific use case, and it's important to balance performance and efficiency.
37. Explain the use of the
@DynamicInsert
and @DynamicUpdate
annotations in Hibernate.Answer: The
@DynamicInsert
and @DynamicUpdate
annotations are used to control whether Hibernate includes all columns in the generated SQL INSERT and UPDATE statements, respectively. They are typically used to optimize database writes by excluding unchanged columns.
38. What is the purpose of the
@Immutable
annotation in Hibernate?Answer: The
@Immutable
annotation marks an entity as read-only, indicating that its state will not change. This can be useful for performance optimization, as Hibernate doesn't need to track changes and flush updates.
39. Explain the role of the
hibernate.cfg.xml
file in a Hibernate project.Answer: The
hibernate.cfg.xml
file is a configuration file that specifies various settings for Hibernate, such as database connection details, dialect, and cache configuration. It is essential for setting up Hibernate within an application.
40. What is the purpose of the
@MappedSuperclass
annotation in Hibernate?Answer: The
@MappedSuperclass
annotation is used to define a superclass whose mapping information is inherited by its subclasses. It's useful for reusing common mappings and reducing redundancy in entity classes.These questions cover more advanced topics in Hibernate and should help you prepare for in-depth discussions during interviews. Make sure to thoroughly understand Hibernate's features and capabilities and practice using them in real-world scenarios.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Networking Concepts, Machine Learning, Angular JS, Node JS, Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
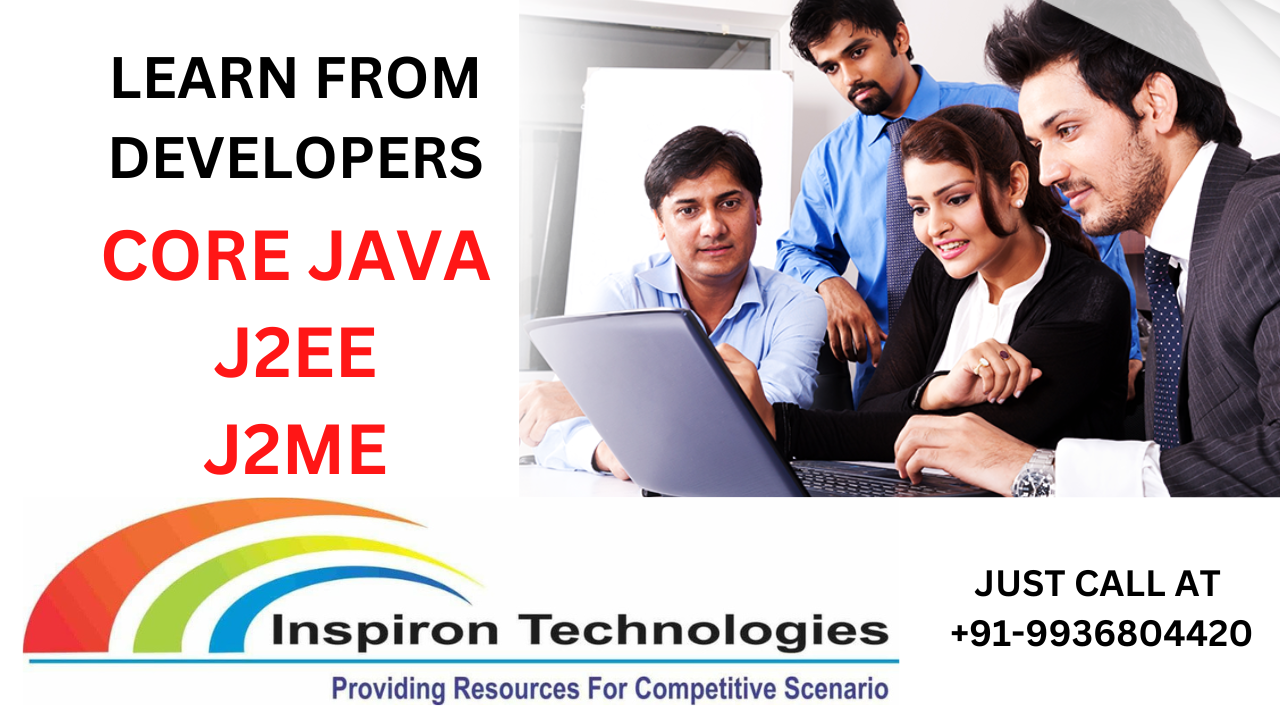
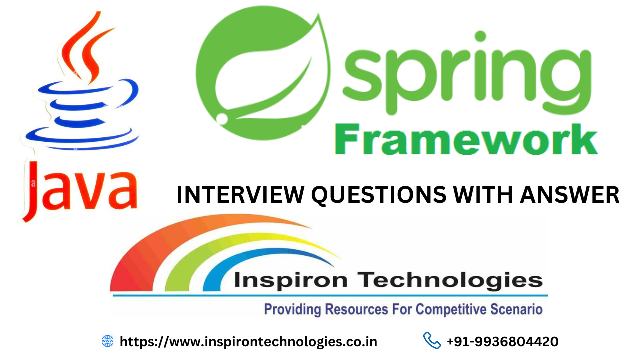
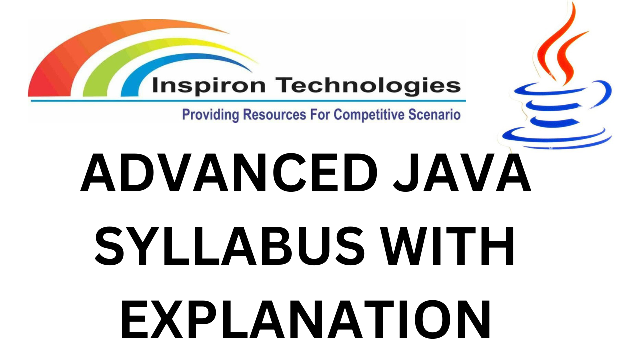
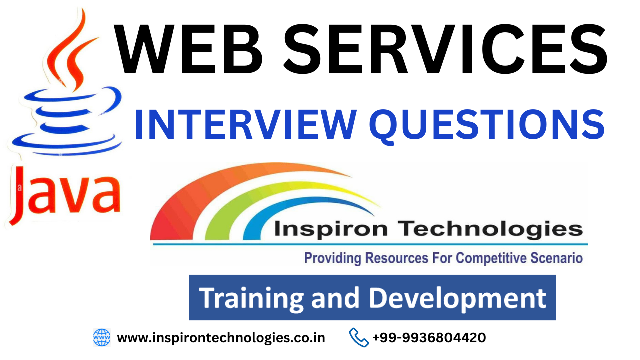
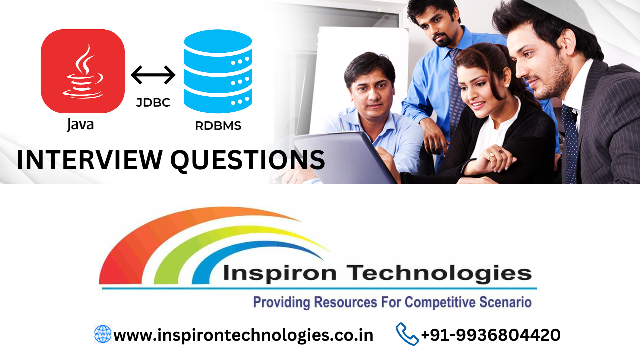
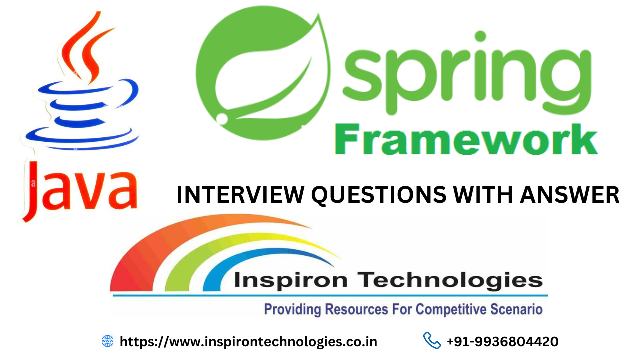
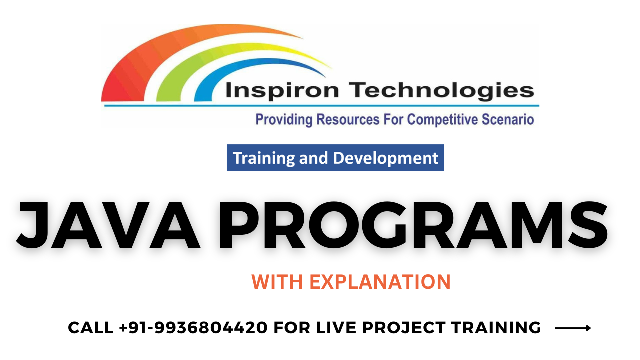
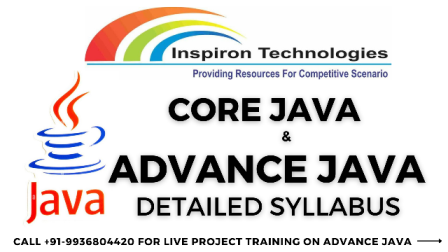
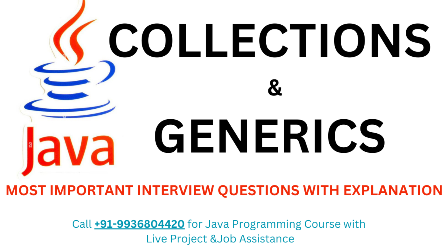
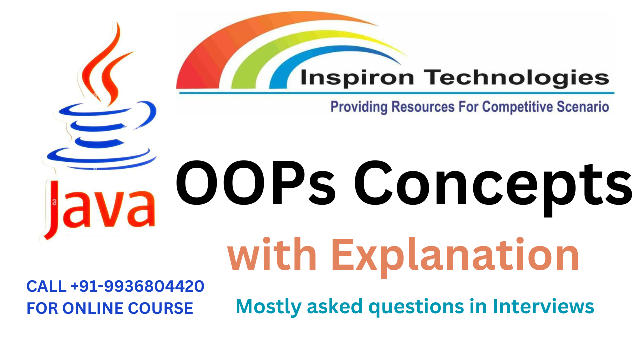
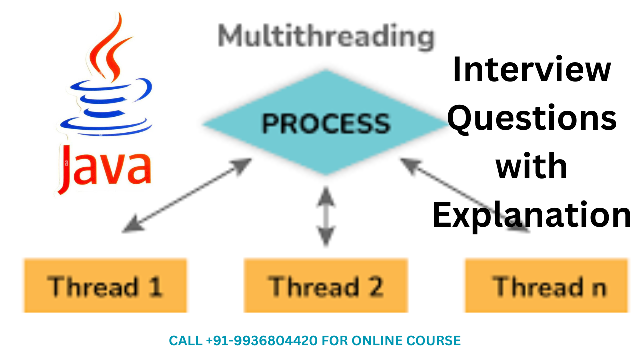
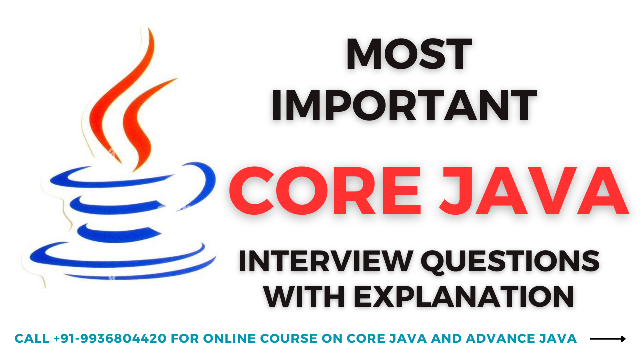
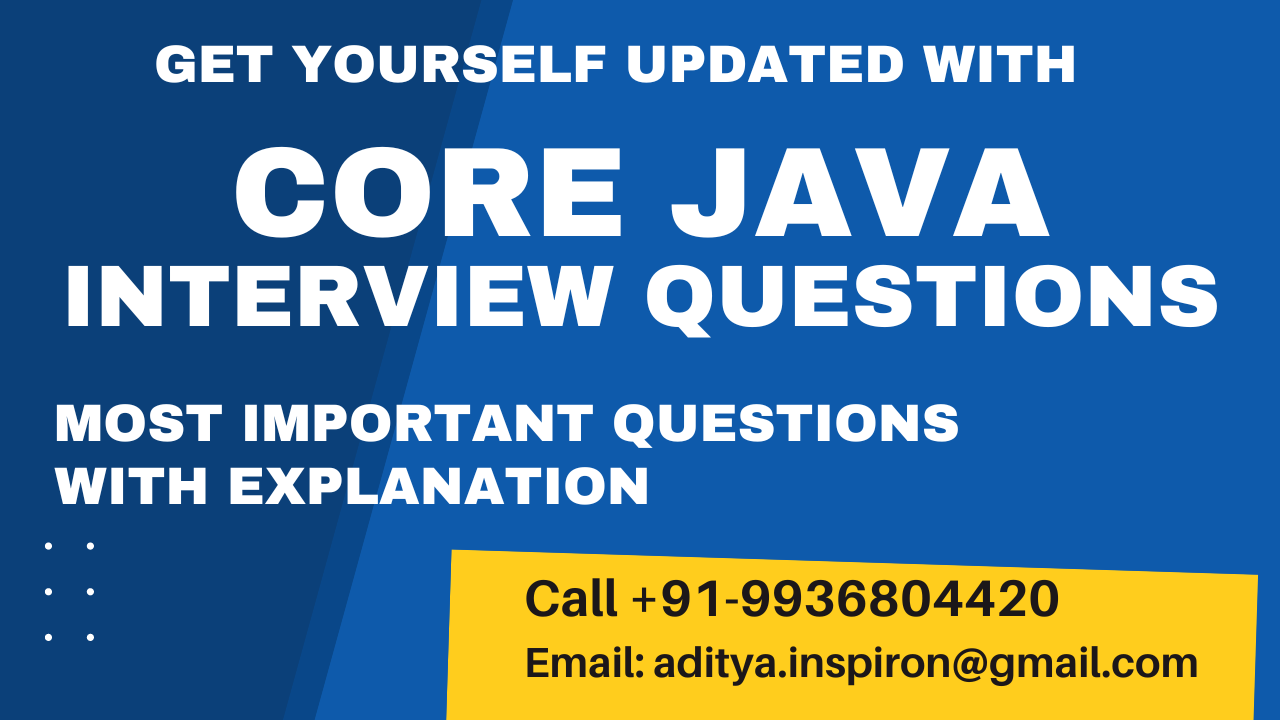
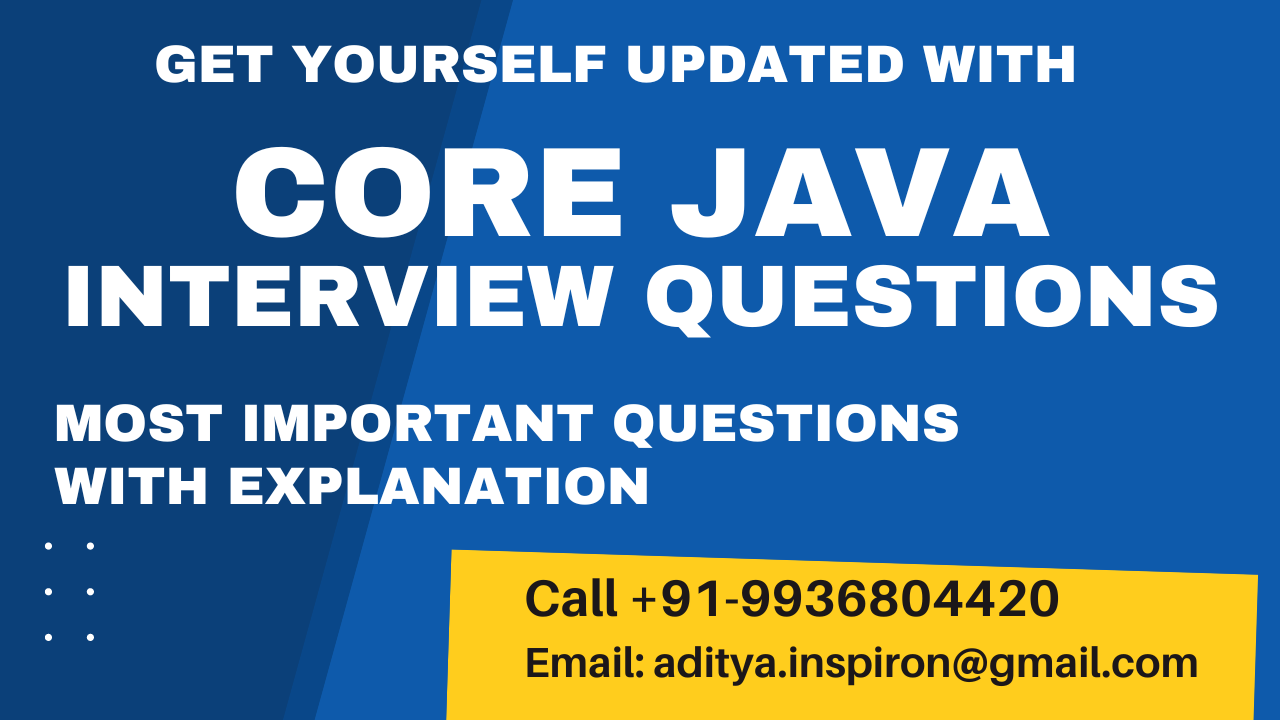
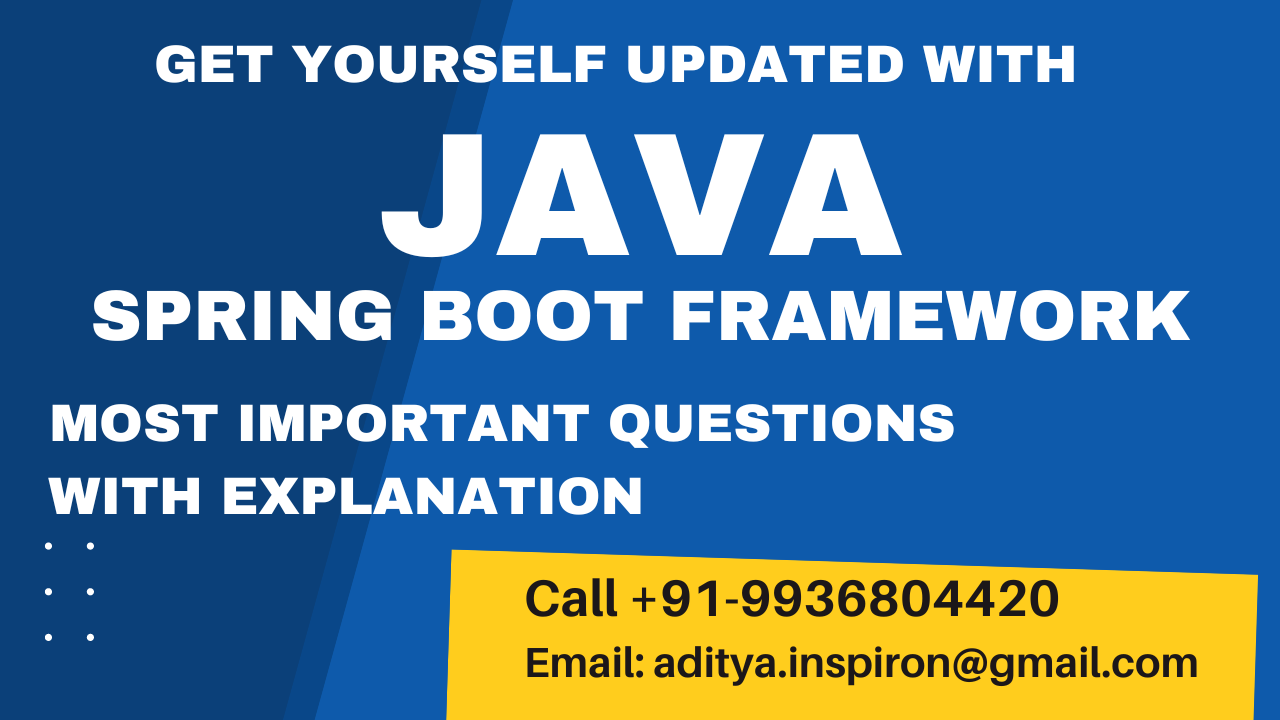
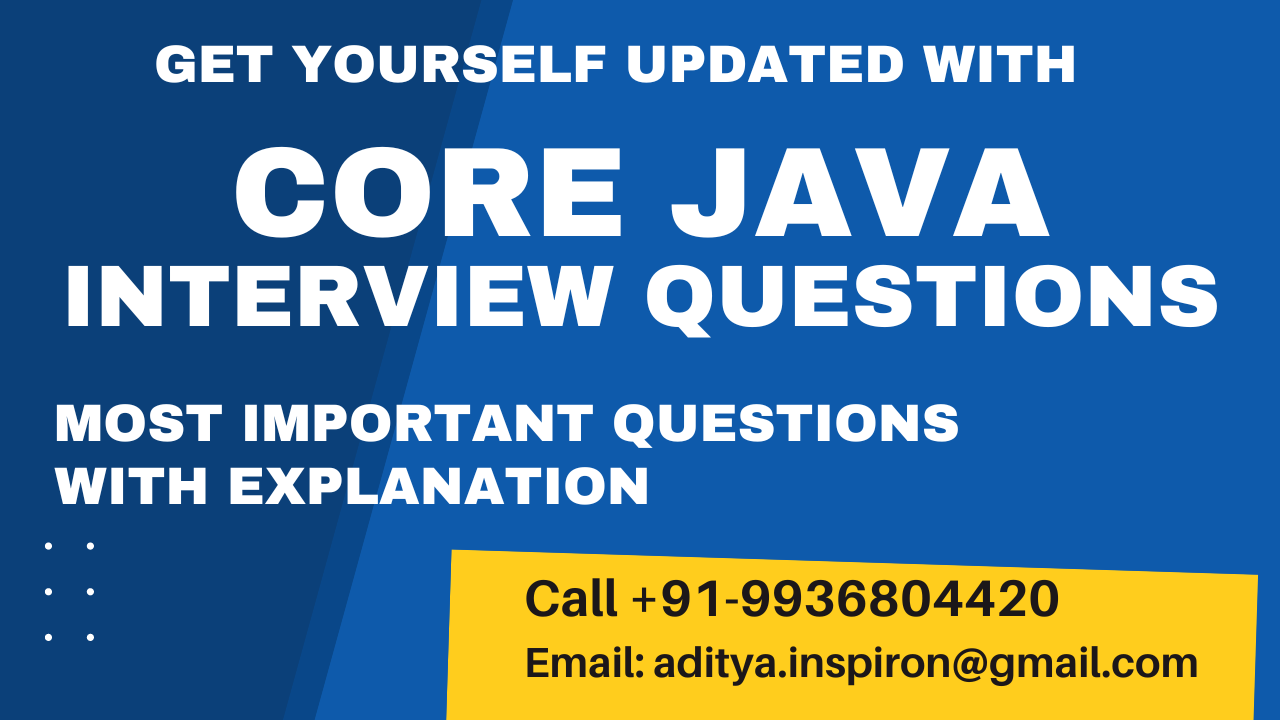
Categories
Popular Post
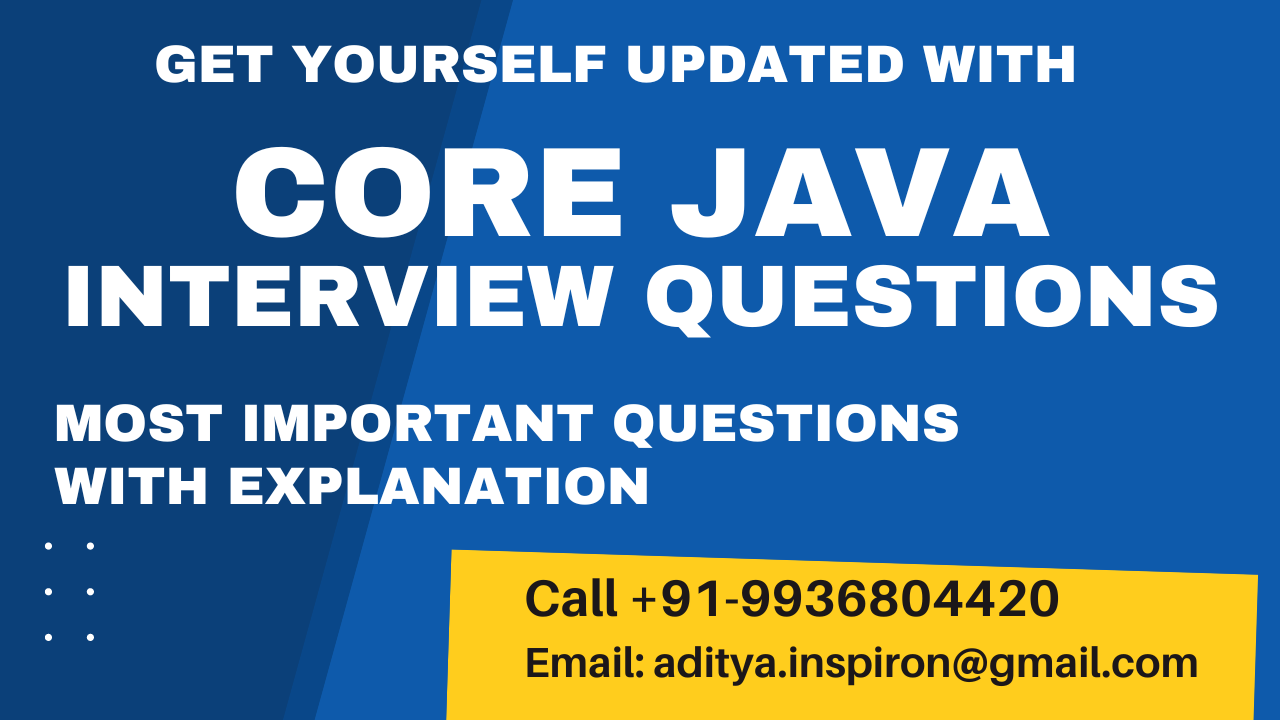
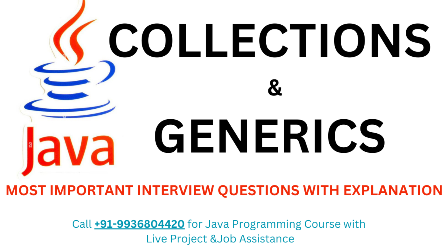
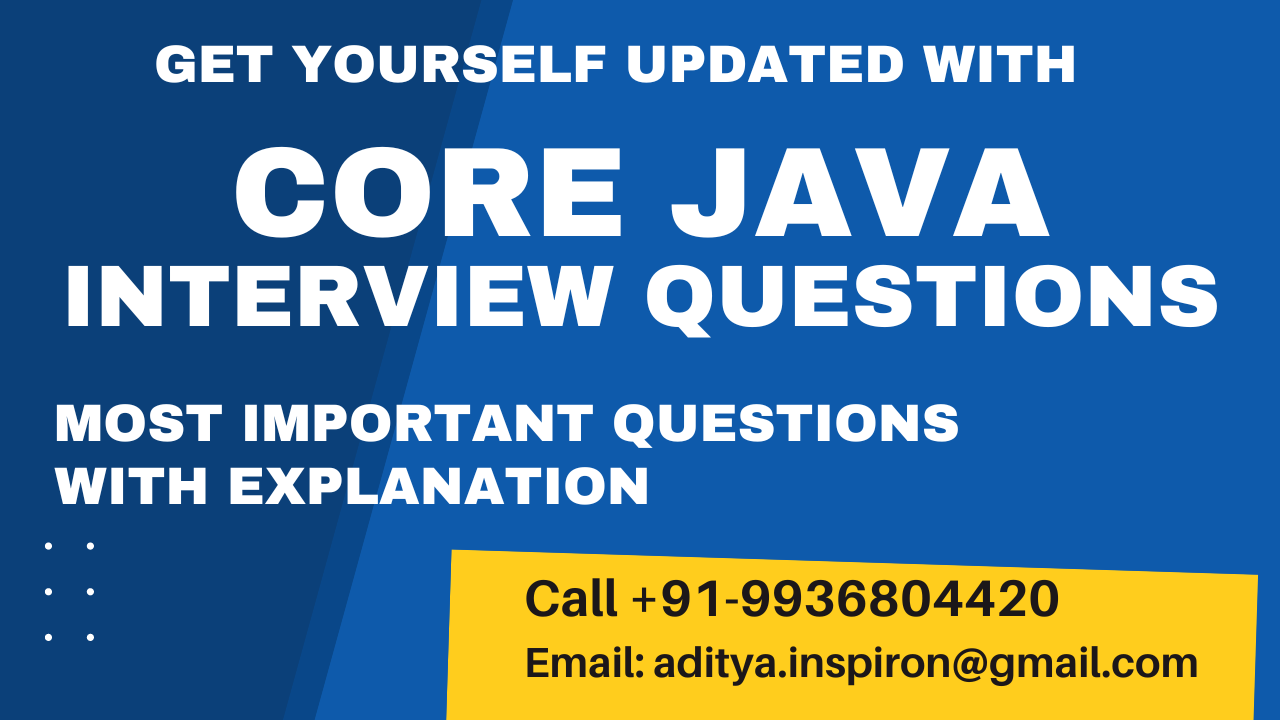
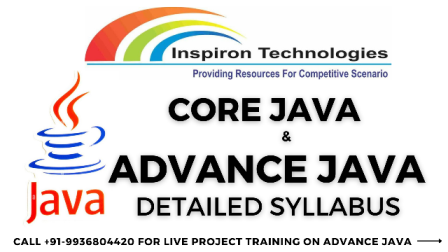
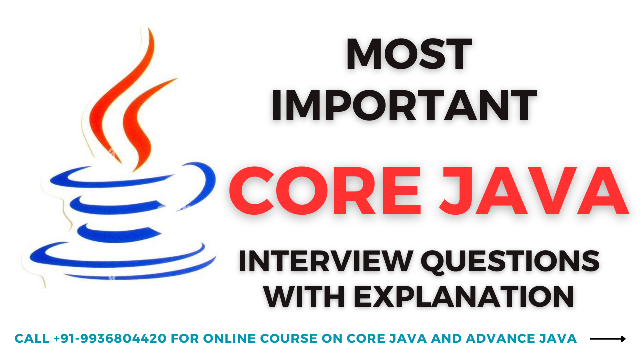
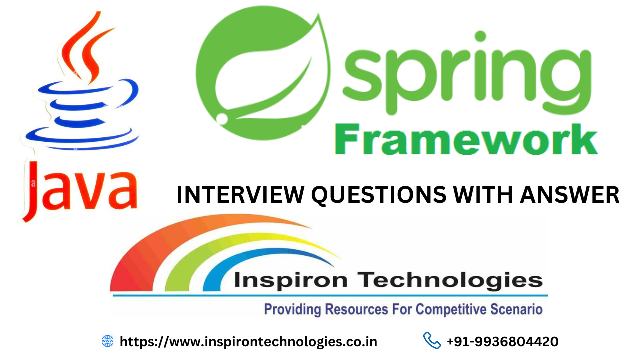
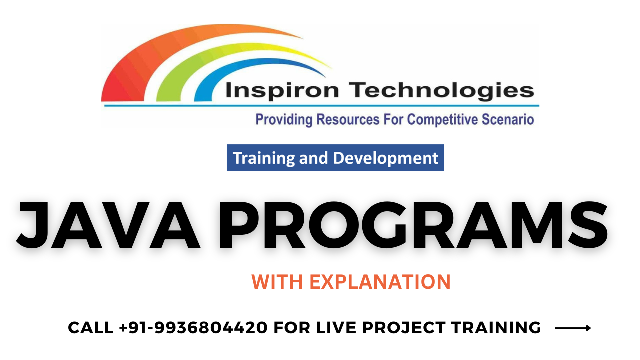
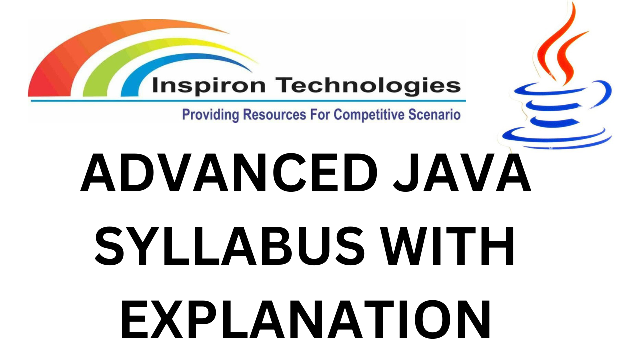
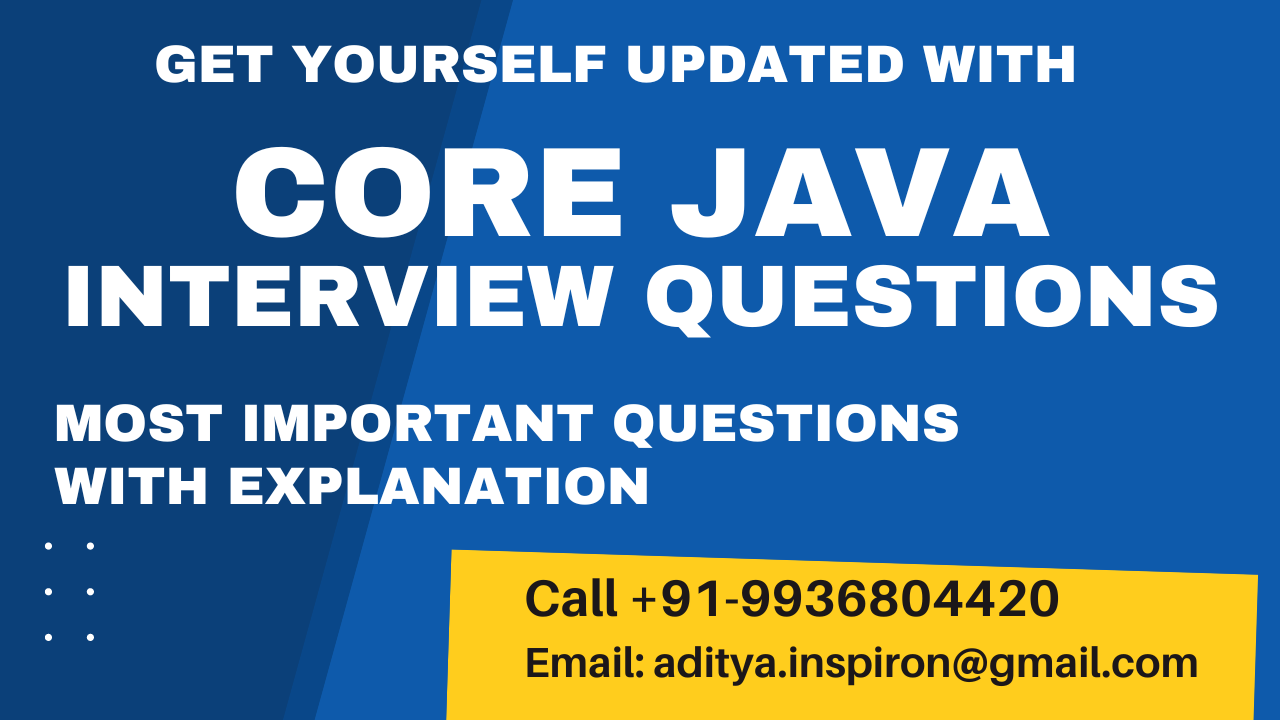
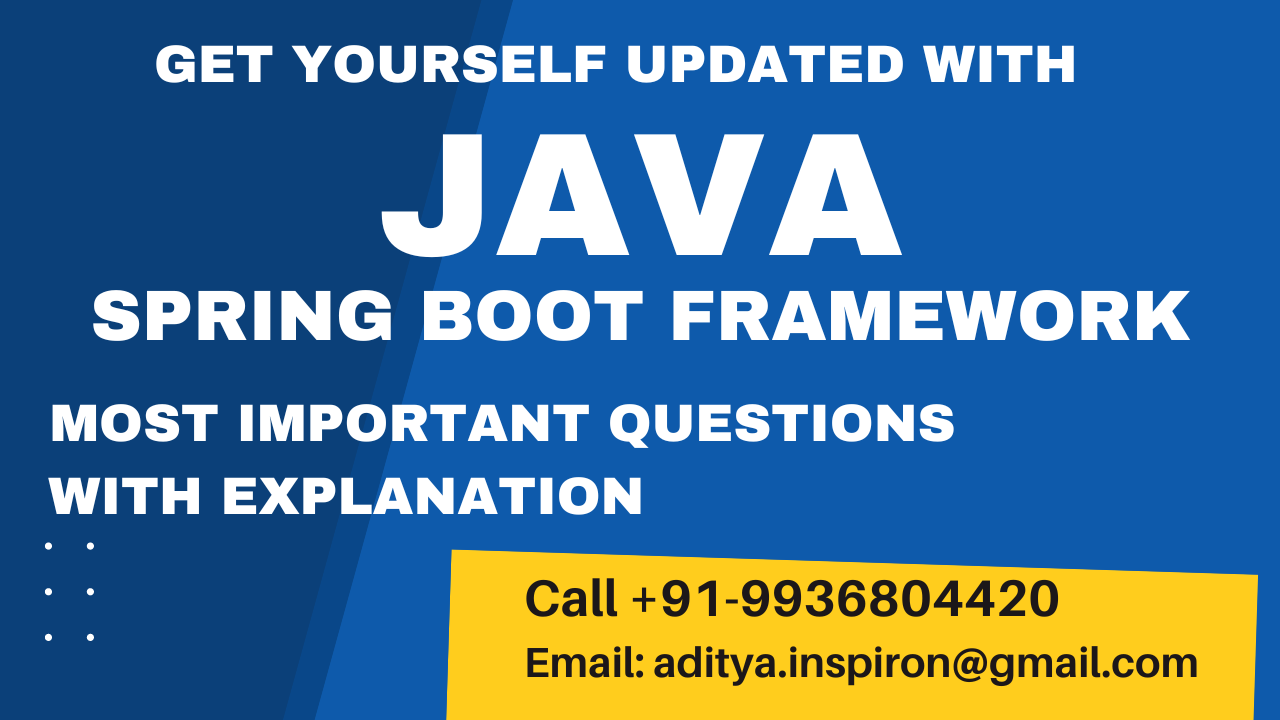
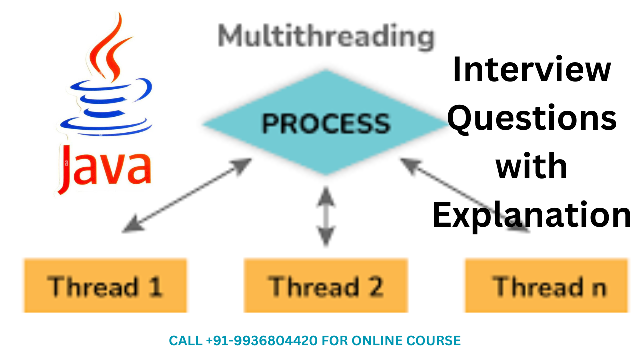
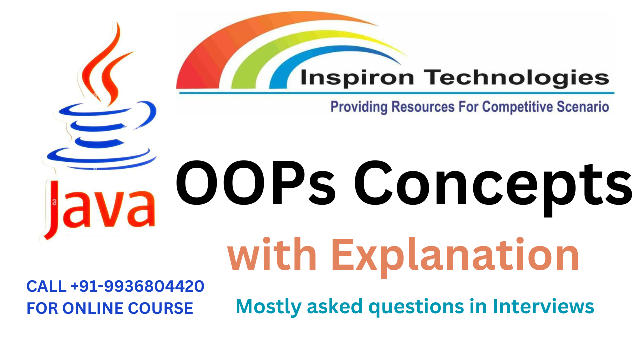
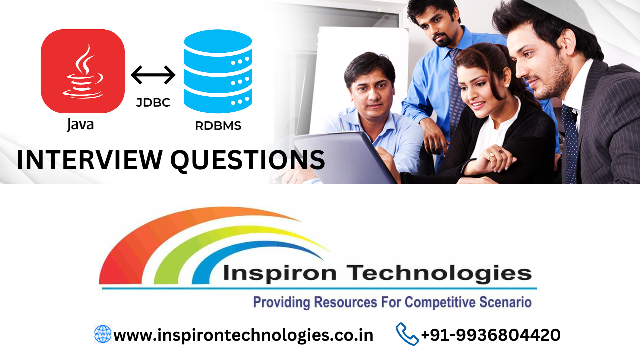
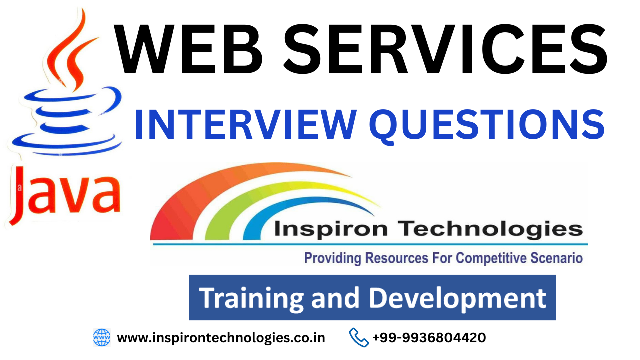
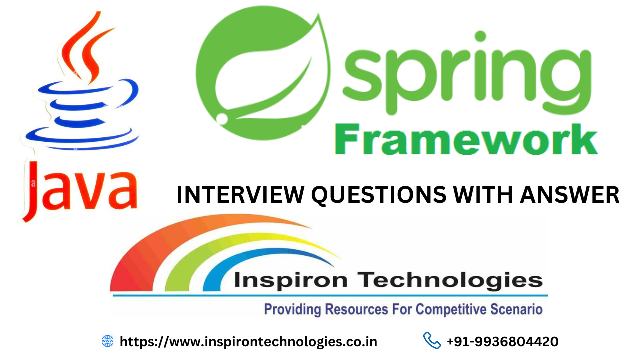
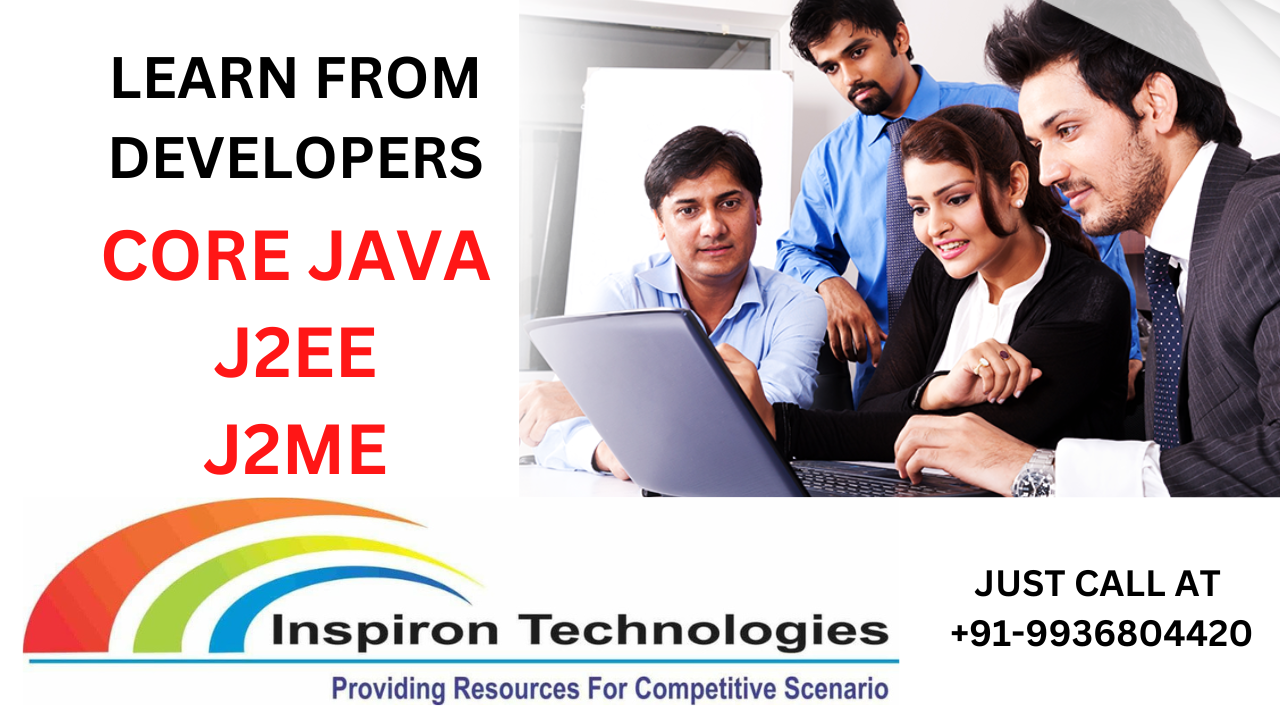
Leave a comment