Java Multithreading Interview Questions
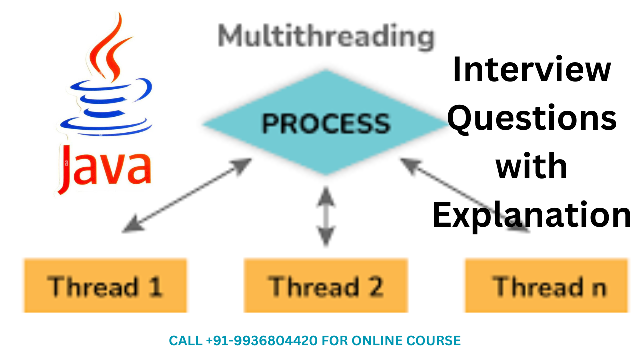
Java Multithreading Interview Questions with Correct Answer and Explanation
1. What is a thread in Java?
Answer: A thread is a lightweight sub-process that executes a sequence of instructions, independently of the main thread.
Explanation: In Java, a thread is a separate flow of execution that runs concurrently with the main program. It allows a program to perform multiple tasks at the same time by executing different threads simultaneously.
2. What is multithreading in Java?
Answer: Multithreading is a feature of Java that allows concurrent execution of two or more parts of a program for maximum utilization of CPU.
Explanation: Multithreading allows a Java program to perform multiple tasks at the same time by dividing the program into smaller threads that run concurrently. This enables the program to execute multiple tasks simultaneously, thereby improving performance and responsiveness.
3. What is the difference between process and thread?
Answer: A process is a heavyweight entity that runs independently of other processes, while a thread is a lightweight entity that runs within the context of a process.
Explanation: A process has its own memory space, while a thread shares the memory space of the process that created it. A process can have multiple threads, each of which executes independently of the other threads.
4. What is a deadlock in Java?
Answer: A deadlock occurs when two or more threads are blocked forever, waiting for each other to release a resource that they need to proceed.
Explanation: A deadlock occurs when two or more threads are blocked forever, waiting for each other to release a resource that they need to proceed. Deadlocks can be difficult to detect and fix, as they involve multiple threads that are all waiting for each other.
5. What is a race condition in Java?
Answer: A race condition occurs when the outcome of a program depends on the relative timing of two or more threads executing a shared piece of code.
Explanation: A race condition occurs when two or more threads are accessing a shared resource or executing a shared piece of code simultaneously, and the outcome of the program depends on the order in which the threads execute. Race conditions can lead to unpredictable and incorrect behavior in a program.
6. What is synchronization in Java?
Answer: Synchronization is a feature of Java that allows multiple threads to access a shared resource in a mutually exclusive manner, preventing race conditions and other concurrency issues.
Explanation: Synchronization allows multiple threads to access a shared resource in a mutually exclusive manner, so that only one thread can access the resource at a time. This prevents race conditions and other concurrency issues that can occur when multiple threads access the same resource simultaneously.
7. What is the difference between wait() and sleep() methods in Java?
Answer: The wait() method is used to release the lock held by the current thread and wait for another thread to notify it, while the sleep() method is used to pause the execution of the current thread for a specified amount of time.
Explanation: The wait() method is used to release the lock held by the current thread and wait for another thread to notify it when a certain condition is met. The sleep() method is used to pause the execution of the current thread for a specified amount of time, without releasing any locks.
8. What is the purpose of the yield() method in Java?
Answer: The yield() method is used to temporarily pause the execution of the current thread, allowing other threads with the same priority to execute.
Explanation: The yield() method is used to temporarily pause the execution of the current thread, allowing other threads with the same priority to execute. It does not guarantee that the current thread will stop executing, and may result in the current thread continuing to execute immediately after the yield() call.
9. What is a thread pool in Java?
Answer: A thread pool is a collection of pre-initialized threads.
10. What is the purpose of multithreading in Java?
A. The purpose of multithreading in Java is to improve the performance of the program by allowing multiple threads to execute concurrently.
11. What are the two ways to create a thread in Java?
A. The two ways to create a thread in Java are by extending the Thread class and by implementing the Runnable interface.
12, What is the difference between extending the Thread class and implementing the Runnable interface?
A. When a class extends the Thread class, it cannot extend any other class, whereas implementing the Runnable interface allows the class to extend another class. Additionally, extending the Thread class defines a new thread, while implementing the Runnable interface allows the object to be executed by a thread.
13. What is the difference between a process and a thread?
A. A process is a standalone program, whereas a thread is a part of a process. A process has its own memory space, whereas threads share the same memory space.
14. What is synchronization in Java?
A. Synchronization in Java is the process of controlling the access of multiple threads to shared resources to prevent race conditions and ensure consistency.
15. What is the purpose of the synchronized keyword in Java?
A. The synchronized keyword in Java is used to define a synchronized method or block, which ensures that only one thread can access a shared resource at a time.
16. What is a deadlock in Java?
A. A deadlock in Java is a situation where two or more threads are blocked, waiting for each other to release a resource, resulting in a stalemate.
17. What is the purpose of the wait() method in Java?
A. The wait() method in Java is used to make a thread wait for a certain amount of time until another thread notifies it to resume execution.
18. What is the purpose of the notify() method in Java?
A. The notify() method in Java is used to wake up a thread that is waiting on a shared resource to become available.
19. What is the purpose of the yield() method in Java?
A. The yield() method in Java is used to make a thread voluntarily give up the CPU and allow other threads to run.
20. What is the purpose of the join() method in Java?
A. The join() method in Java is used to wait for a thread to finish its execution before continuing with the execution of the current thread.
21. What is a daemon thread in Java?
A. A daemon thread in Java is a thread that runs in the background and provides services to other threads, without blocking the main program from exiting.
22. What is the purpose of the setDaemon() method in Java?
A. The setDaemon() method in Java is used to mark a thread as a daemon thread, which means that the thread will not prevent the program from exiting when all non-daemon threads have completed.
23. What is the purpose of the interrupt() method in Java?
A. The interrupt() method in Java is used to interrupt a thread that is waiting or sleeping, causing it to throw an InterruptedException and wake up.
Explanation:
Multithreading is an important concept in Java, and it allows programs to execute multiple threads concurrently, thereby improving the program's performance. The creation of a thread can be done by either extending the Thread class or implementing the Runnable interface. Synchronization is used to control access to shared resources and ensure consistency, and the synchronized keyword is used to define a synchronized method or block. Deadlocks can occur when two or more threads are blocked, waiting for each other to
24. What is concurrency in Java?
Answer: Concurrency is the ability of a program to perform multiple tasks simultaneously.
25. What is a thread in Java?
Answer: A thread in Java is a lightweight, independent unit of execution that runs within a program.
26. What is the difference between process and thread?
Answer: A process is an independent program that has its own memory space, whereas a thread is a unit of execution that runs within a process and shares the process's memory space.
27. How can you create a thread in Java?
Answer: You can create a thread in Java by extending the Thread class or implementing the Runnable interface.
28. What is the purpose of the start() method in Java threads?
Answer: The start() method is used to start a new thread of execution.
29. What is the purpose of the join() method in Java threads?
Answer: The join() method is used to wait for a thread to complete its execution before continuing with the rest of the program.
30. What is the purpose of the sleep() method in Java threads?
Answer: The sleep() method is used to pause the execution of a thread for a specified amount of time.
31. What is synchronization in Java threads?
Answer: Synchronization is the process of controlling access to shared resources in a multi-threaded environment to prevent conflicts.
32. What is the purpose of the synchronized keyword in Java?
Answer: The synchronized keyword is used to create a synchronized block or method to ensure that only one thread at a time can access the code within the block or method.
33. What is a deadlock in Java threads?
Answer: A deadlock is a situation in which two or more threads are blocked waiting for each other to release a resource that they need to proceed.
24. What is a race condition in Java threads?
Answer: A race condition is a situation in which the behavior of a program depends on the relative timing of two or more threads executing concurrently.
25. What is a thread pool in Java?
Answer: A thread pool is a collection of pre-created threads that can be reused to perform multiple tasks in a multi-threaded environment.
26. What is the purpose of the Executor framework in Java?
Answer: The Executor framework provides a way to decouple the task submission from the task execution, allowing for greater flexibility and control over the execution of tasks in a multi-threaded environment.
27. What is the purpose of the ReentrantLock class in Java?
Answer: The ReentrantLock class is used to create a lock that can be acquired and released by the same thread multiple times, allowing for greater flexibility and control over synchronization in a multi-threaded environment.
28. What is the purpose of the Condition interface in Java?
Answer: The Condition interface provides a way for threads to wait for a certain condition to be met before proceeding, allowing for greater control over synchronization in a multi-threaded environment.
Explanation:
Java concurrency is a critical topic in multi-threaded programming. These questions and answers cover various concepts related to Java concurrency, such as threads, synchronization, thread pools, the Executor framework, ReentrantLock, and Condition. These questions and answers provide a basic understanding of concurrency in Java, which is essential for developing efficient and reliable multi-threaded applications.
29. What is the purpose of the synchronized keyword in Java?
Answer: The synchronized keyword is used to create mutually exclusive blocks of code, ensuring that only one thread can access that block of code at a time. This is useful in preventing data races and ensuring data consistency.
30. What is the difference between a thread and a process in Java?
Answer: A process is a standalone program that can run independently, while a thread is a lightweight unit of execution within a process. Processes have their own memory space, while threads share the same memory space as the process that spawned them.
31. What is a deadlock in Java concurrency?
Answer: A deadlock is a situation in which two or more threads are blocked and waiting for each other to release resources they are holding. This can lead to a situation where none of the threads can make progress, and the program becomes stuck.
32. What is the purpose of the wait() method in Java concurrency?
Answer: The wait() method is used to temporarily suspend a thread's execution until some condition is met. It can be used to implement synchronization between threads, where one thread waits for another thread to perform some action before continuing.
33. What is a race condition in Java concurrency?
Answer: A race condition is a situation in which the outcome of a program depends on the relative timing or ordering of events between threads. This can lead to unexpected results or data inconsistencies.
34. What is the purpose of the volatile keyword in Java?
Answer: The volatile keyword is used to indicate that a variable may be modified by multiple threads, and that changes to the variable should be immediately visible to all threads. It is used to prevent data caching and ensure data consistency across threads.
35. What is the purpose of the notify() method in Java concurrency?
Answer: The notify() method is used to wake up a single thread that is waiting on a shared resource. This can be used to signal to a waiting thread that a resource it needs is now available.
36. What is the difference between the wait() and sleep() methods in Java?
Answer: The wait() method is used to temporarily suspend a thread's execution until some condition is met, while the sleep() method is used to pause a thread's execution for a specified period of time. Additionally, the wait() method can only be called on an object that is being used for synchronization, while the sleep() method can be called on any thread.
37. What is the purpose of the join() method in Java concurrency?
Answer: The join() method is used to wait for a thread to complete before continuing with the current thread's execution. This is useful for coordinating the execution of multiple threads.
38. What is a thread pool in Java concurrency?
Answer: A thread pool is a collection of pre-created threads that can be used to execute tasks in parallel. Thread pools are used to avoid the overhead of creating and destroying threads for each task, and to limit the number of threads that can be created at any given time.
39. What is a semaphore in Java concurrency?
Answer: A semaphore is a synchronization object that is used to control access to a shared resource. It maintains a set of permits that can be acquired or released by threads, allowing a fixed number of threads to access the resource at any given time.
40. What is a countdown latch in Java concurrency?
Answer: A countdown latch is a synchronization object that allows one or more threads to wait until a specified number of events have occurred before continuing. It is initialized with a count, and each event decrements the count until it reaches zero, at which point the waiting threads are released.
41. What is a cyclic barrier in Java concurrency?
Answer: A cyclic barrier is a synchronization object that allows a set of threads to wait for each other to reach a common barrier point. Once all threads have reached the barrier, they can be released and continue executing.
42. What is a thread-safe class in Java?
Answer: A thread-safe class is a class that is designed to be used safely by multiple threads concurrently. This is typically achieved by ensuring that all shared data is accessed in a synchronized manner, and that the class's methods are designed to handle concurrent access without causing data races or other synchronization issues.
43. What is the purpose of the ThreadLocal class in Java concurrency?
Answer: The ThreadLocal class is used to create thread-local variables, which are variables that are unique to each thread that accesses them. This can be useful for storing state that is specific to a particular thread, such as user context or database connections.
44. What is the purpose of the Executor framework in Java concurrency?
Answer: The Executor framework provides a way to execute tasks in parallel using a pool of threads. It provides a simple way to create and manage threads, and to execute tasks asynchronously without having to explicitly manage thread creation and destruction.
45. What is the difference between a Callable and a Runnable in Java concurrency?
Answer: A Runnable is a simple interface that defines a task that can be executed in a separate thread, while a Callable is similar but can return a result and throw an exception. Additionally, the Callable interface is used with the ExecutorService framework to submit tasks and receive results.
46. What is a thread dump in Java concurrency?
Answer: A thread dump is a snapshot of the current state of all threads in a Java program, including their current stack traces and state. It can be used to diagnose and debug concurrency issues and deadlocks.
47. What is the purpose of the Atomic classes in Java concurrency?
Answer: The Atomic classes provide a set of thread-safe operations on primitive types, such as int and long, as well as on complex objects. They are designed to be used without explicit locking or synchronization, and can help to avoid data races and synchronization issues.
48. What is the purpose of the ReentrantLock class in Java concurrency?
Answer: The ReentrantLock class is a synchronization object that provides more fine-grained locking than the synchronized keyword. It allows a thread to acquire and release a lock multiple times, and provides additional methods for controlling the lock's behavior, such as fair and non-fair locking.
We hope that you must have found this exercise quite useful. If you wish to join Oracle, Full Stack Developer program, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
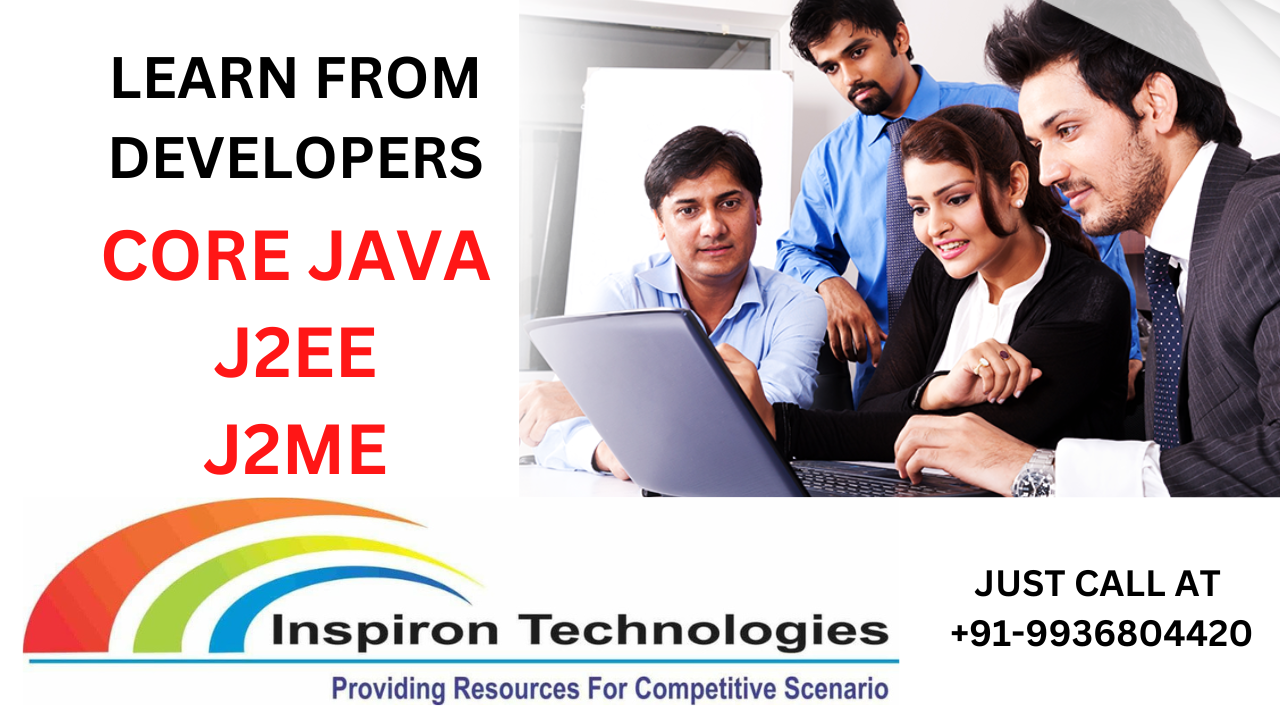
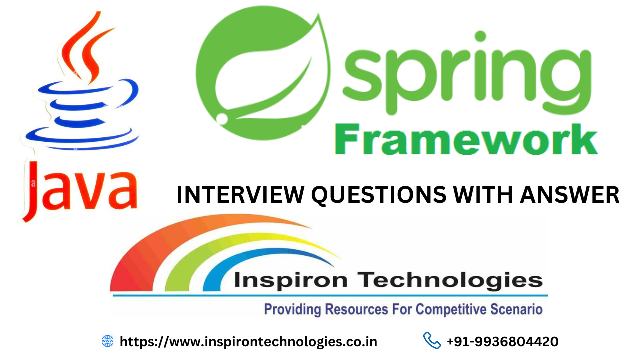
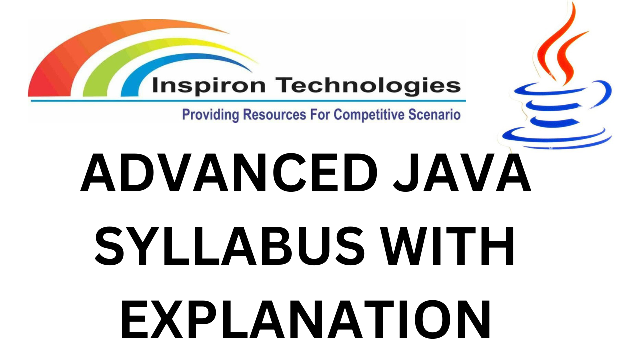
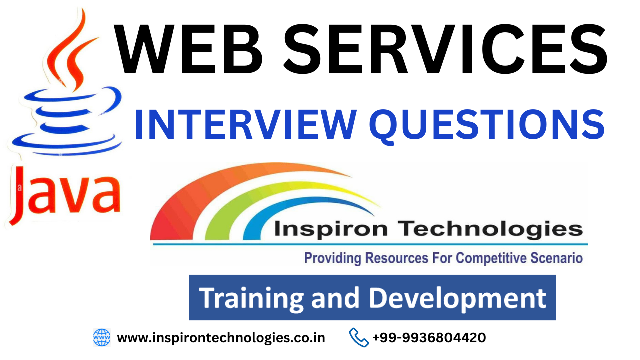
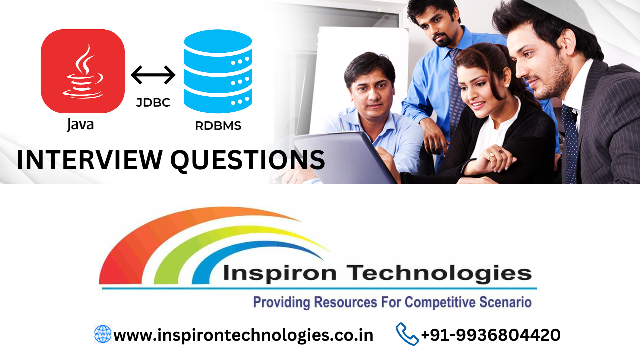
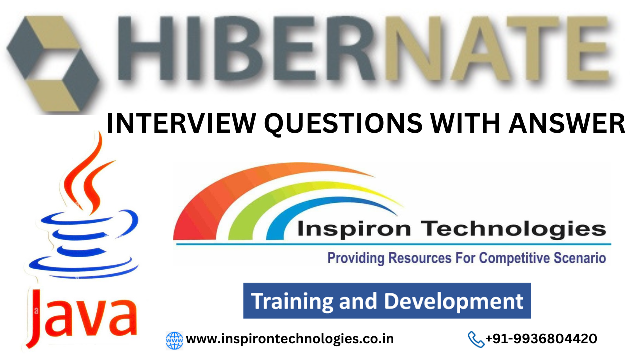
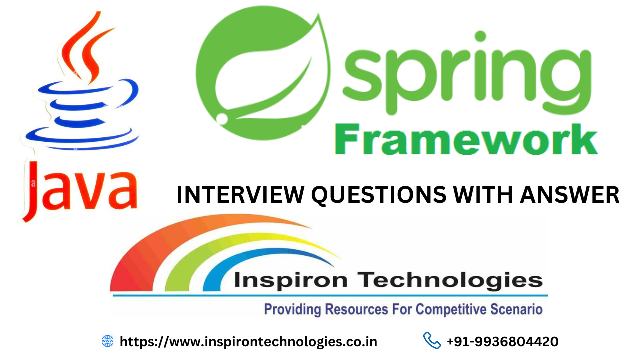
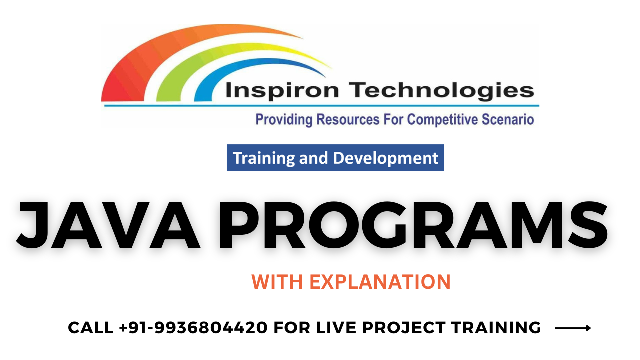
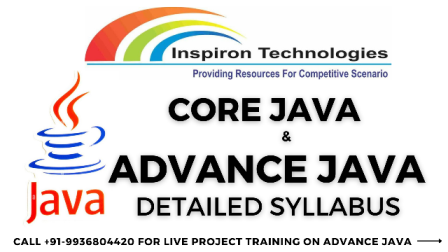
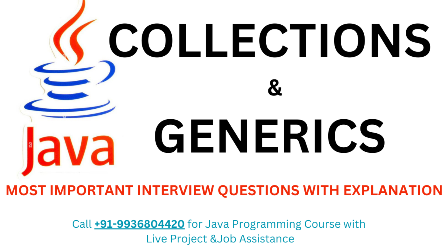
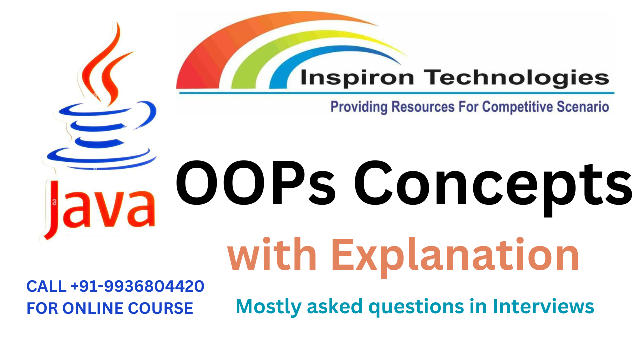
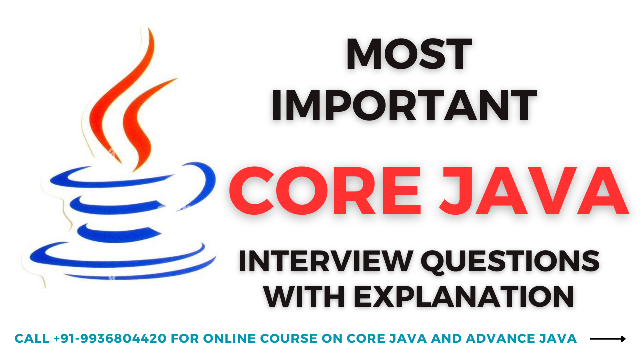
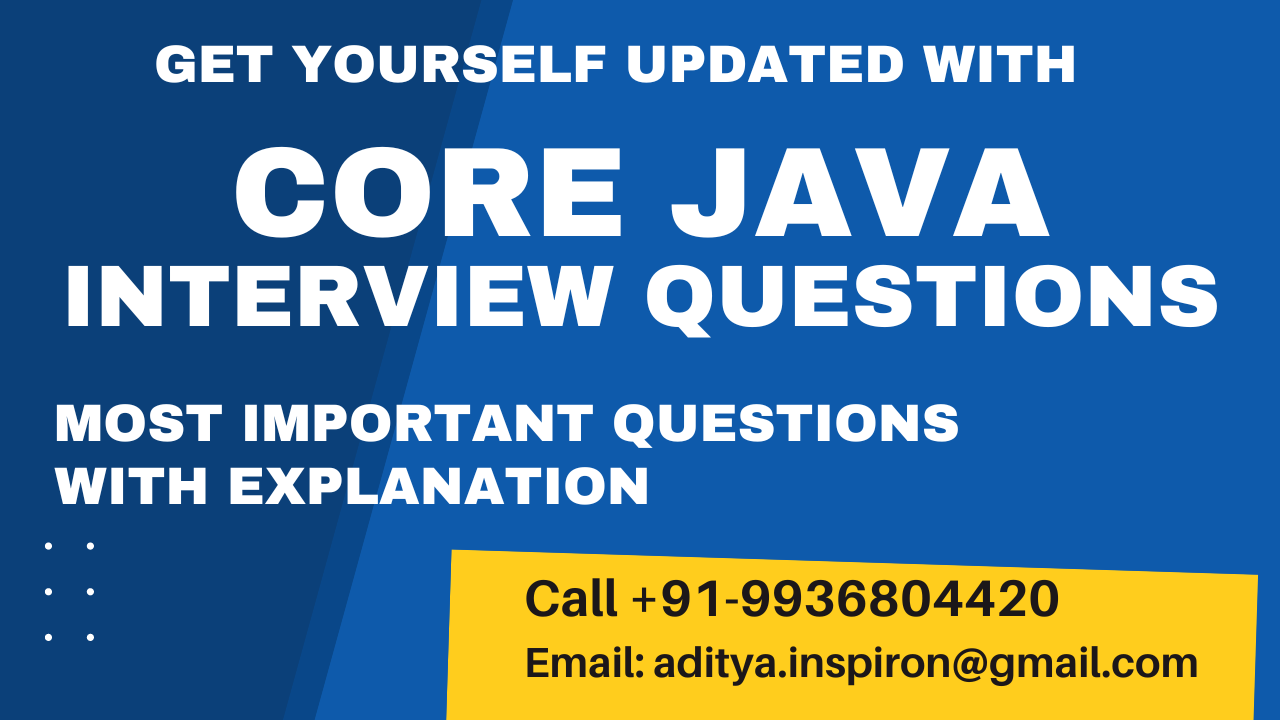
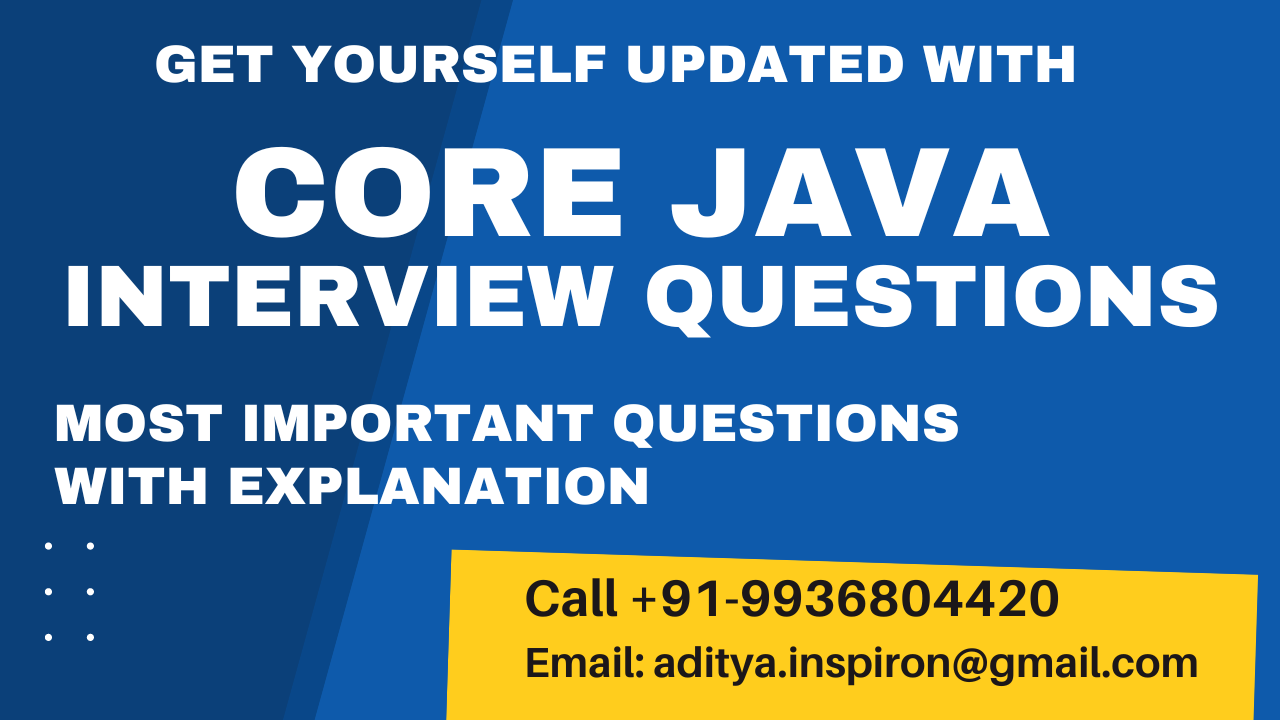
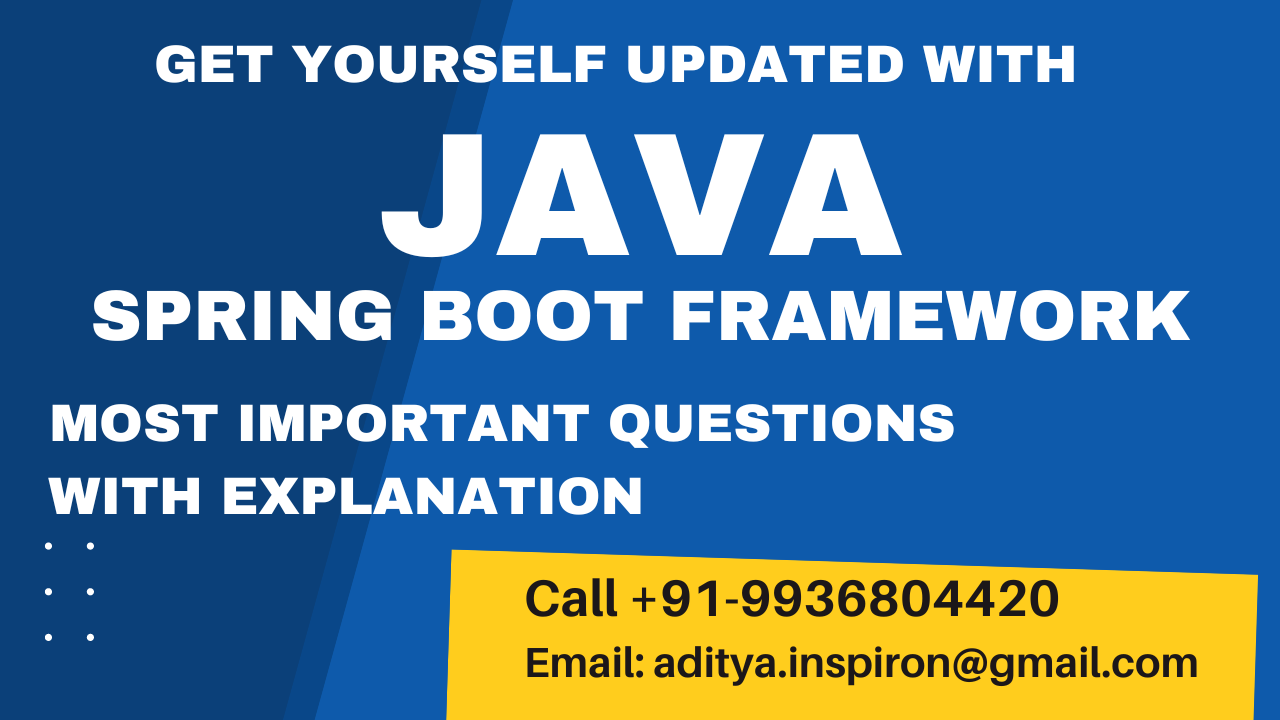
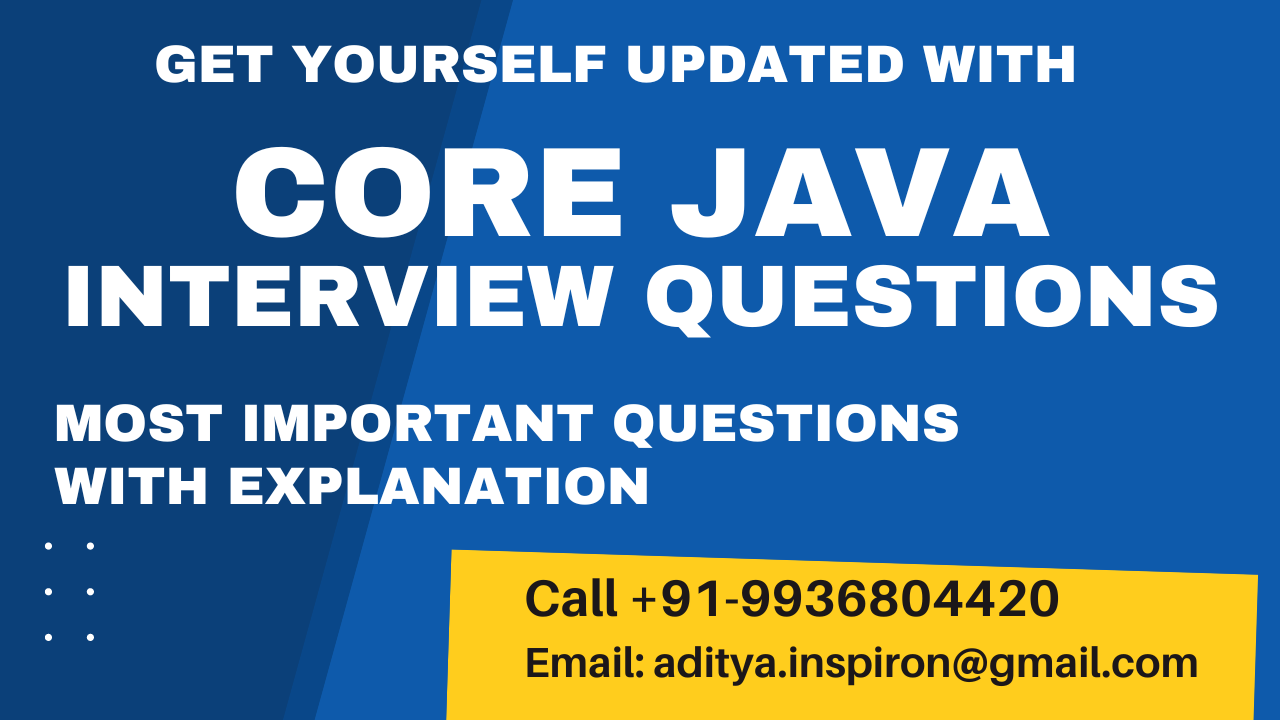
Categories
Popular Post
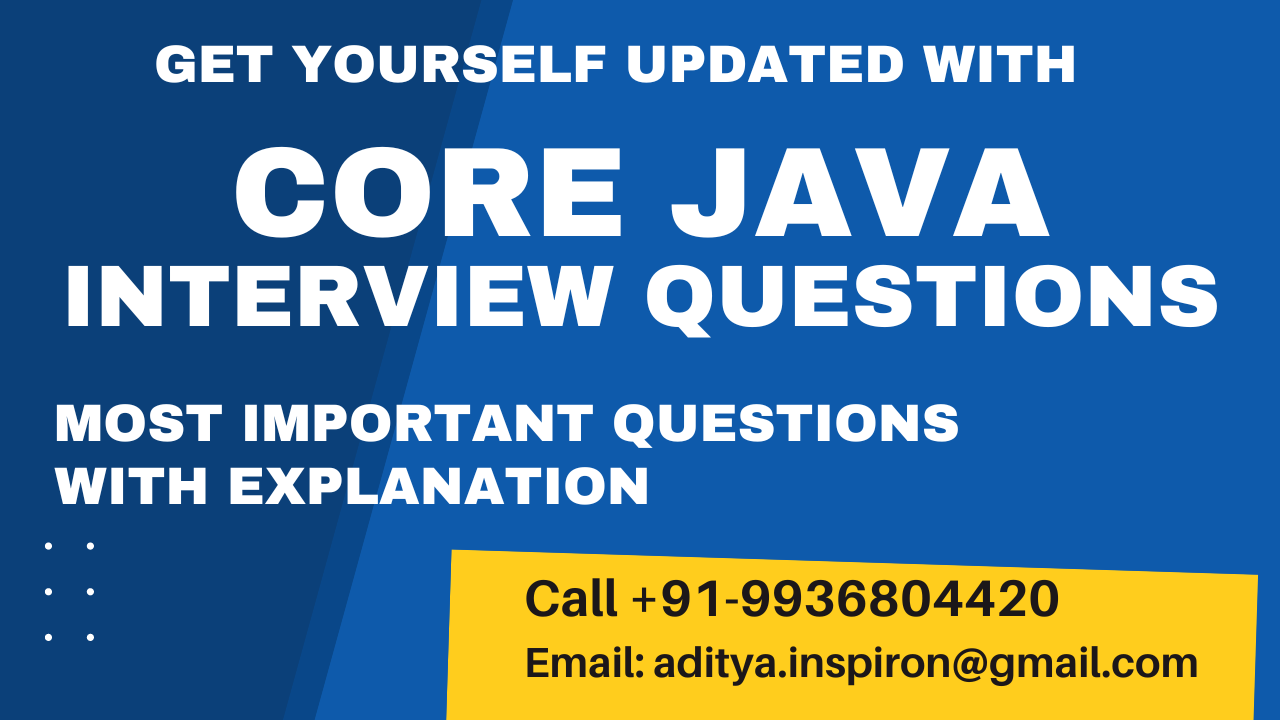
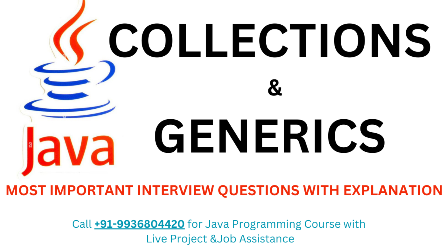
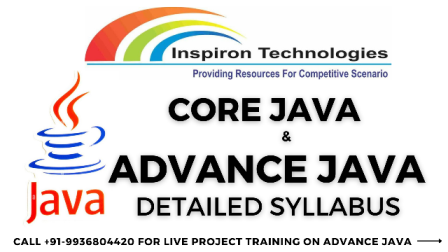
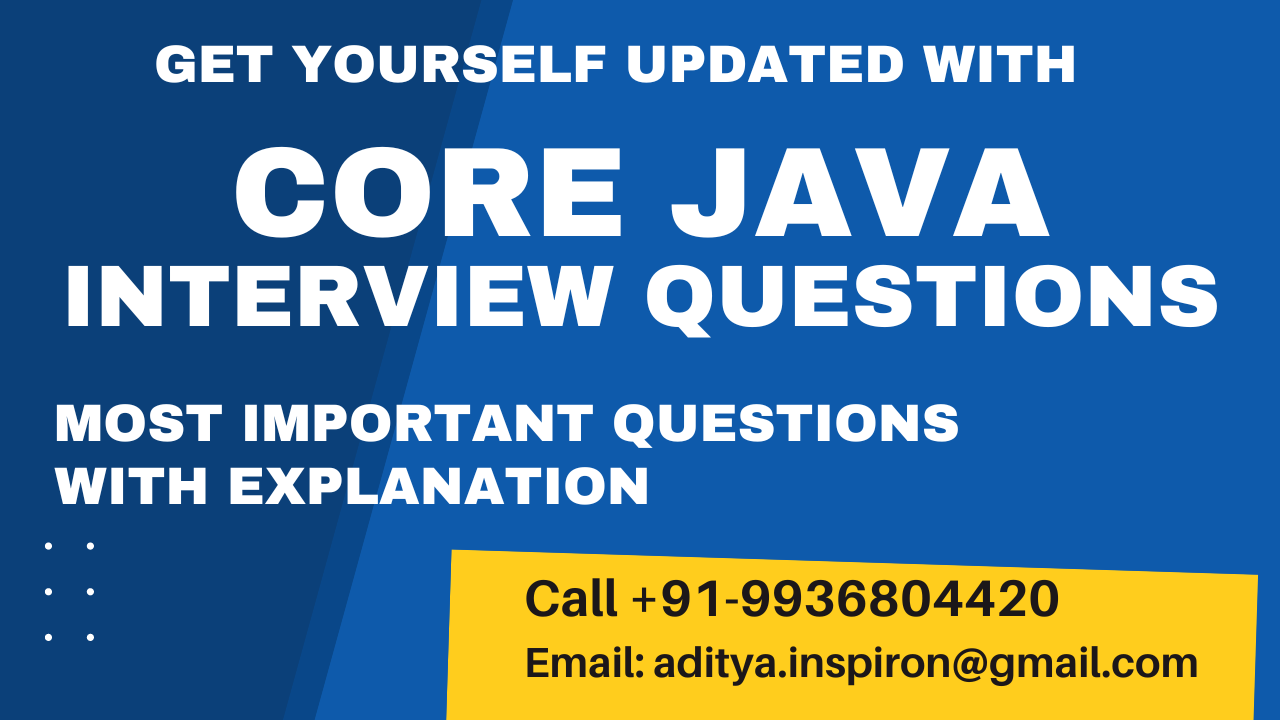
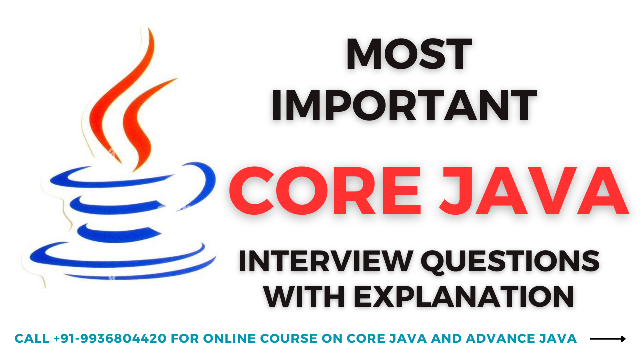
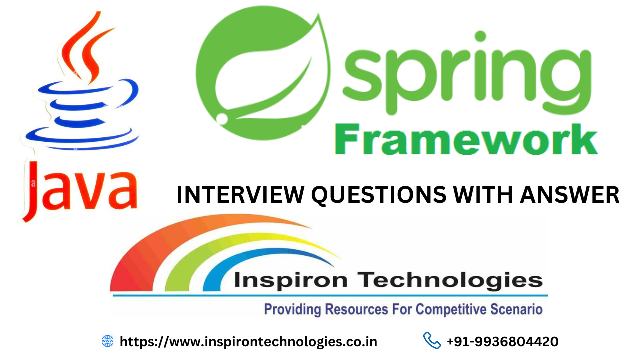
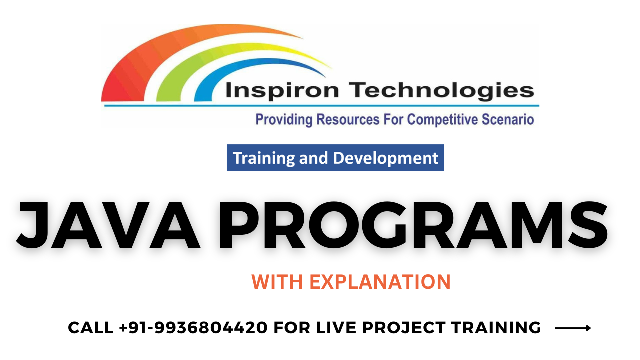
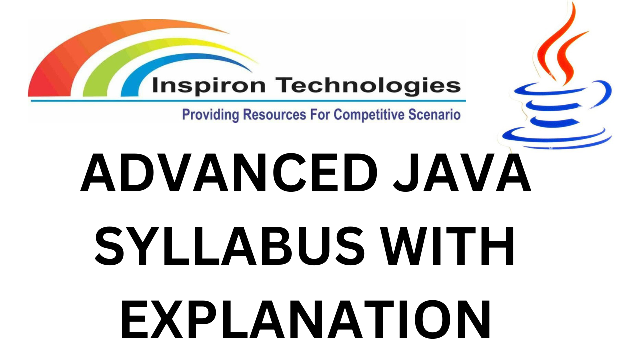
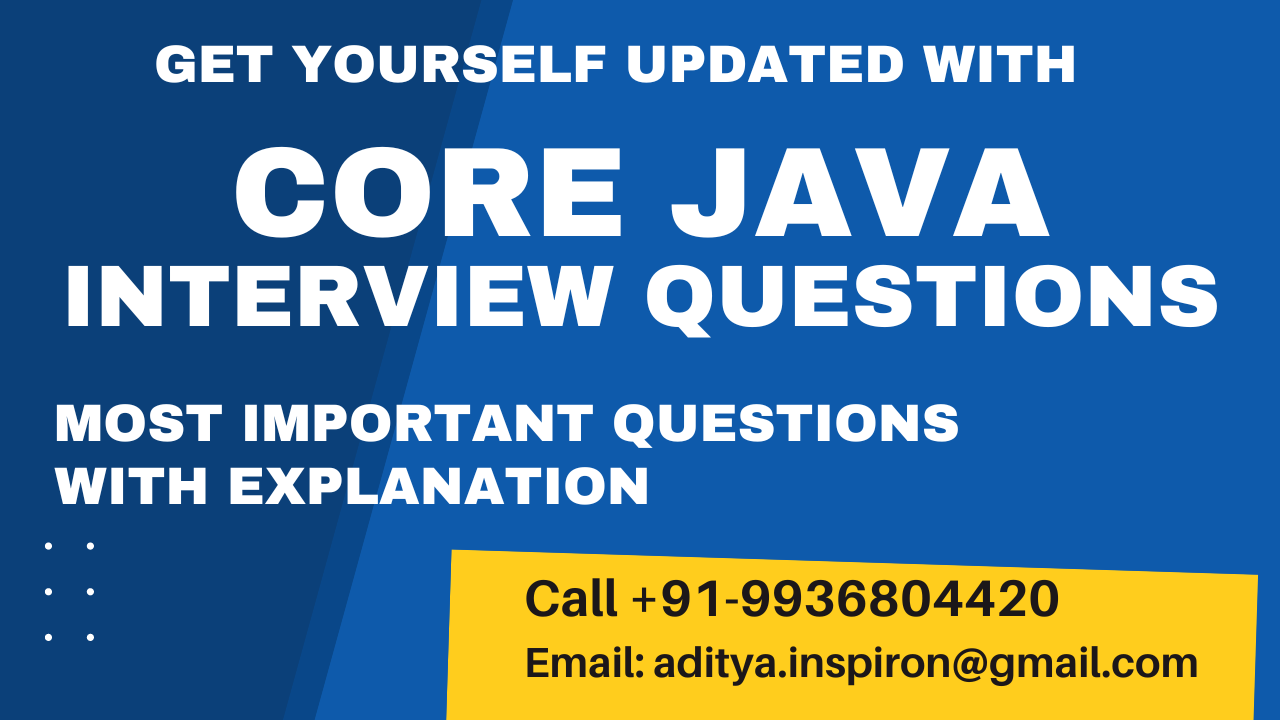
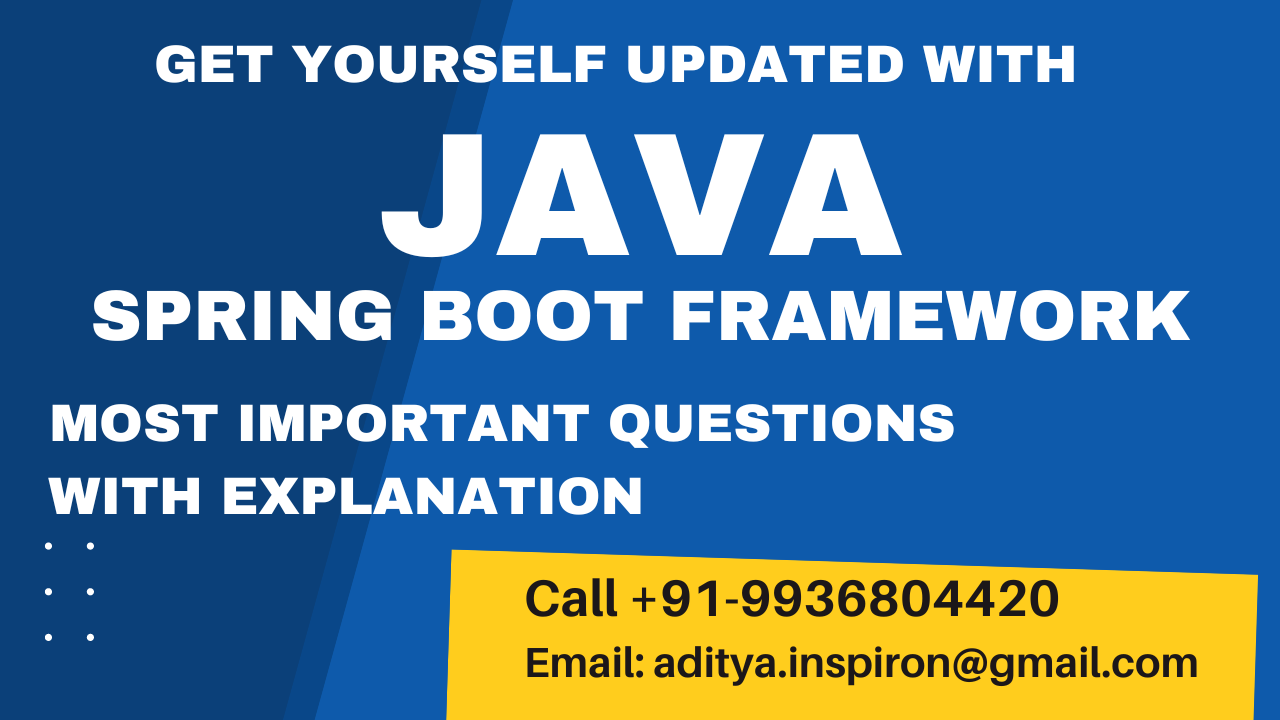
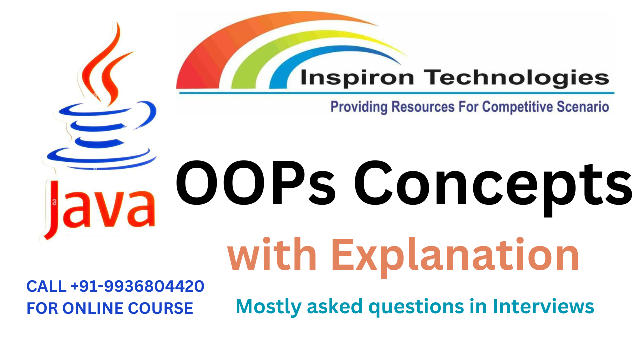
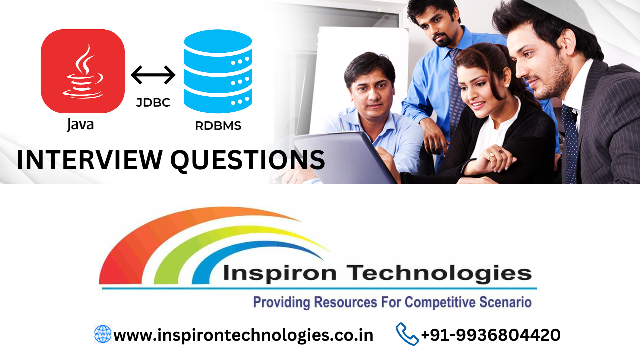
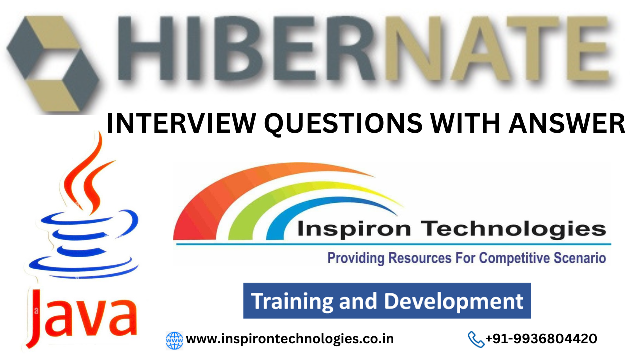
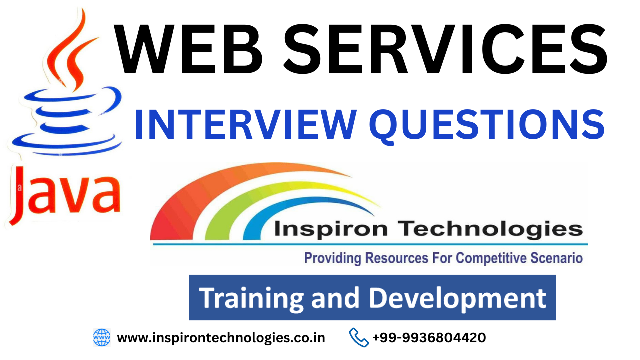
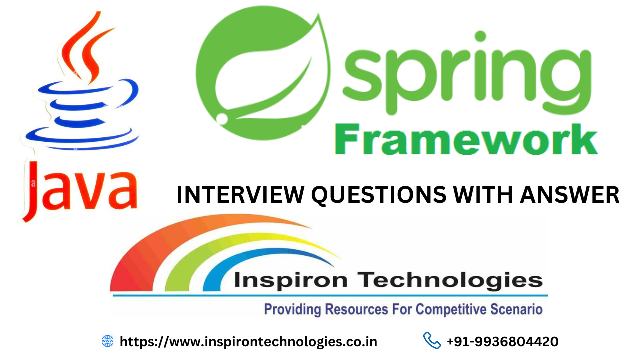
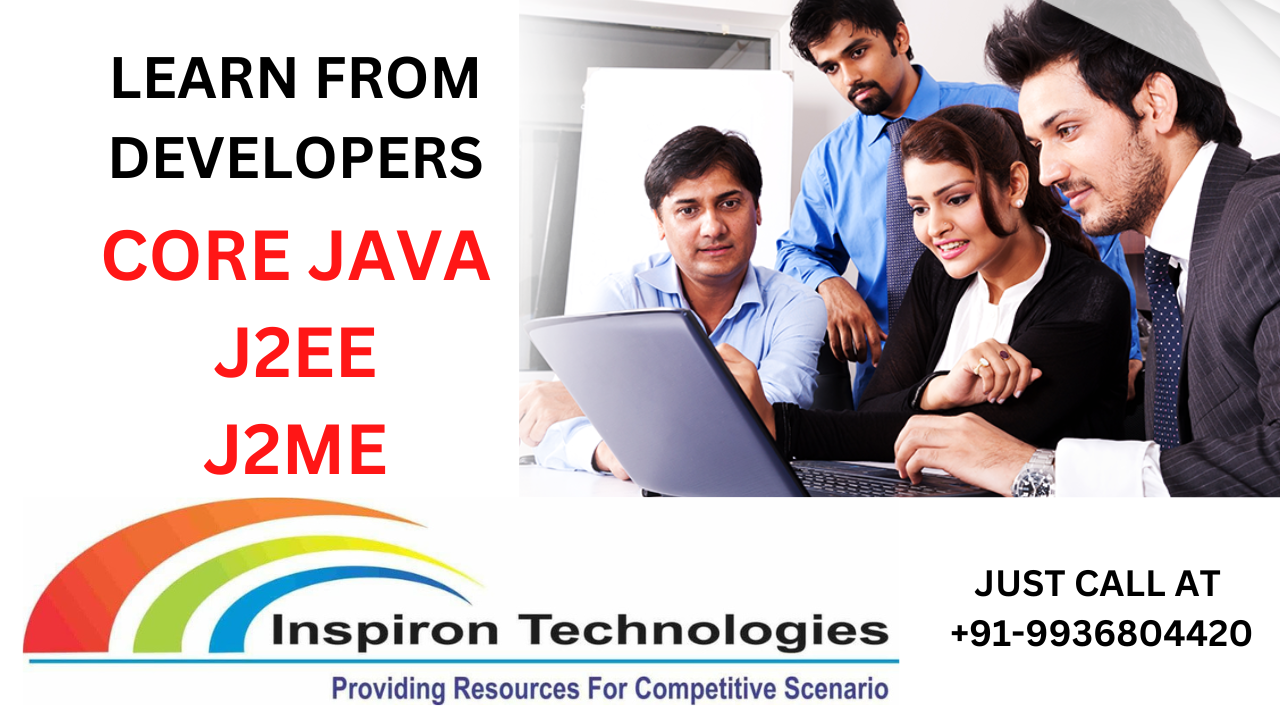
Leave a comment