Java OOPs Concepts
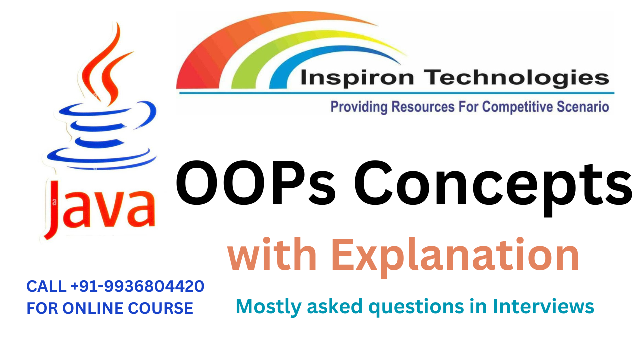
Java OOPs Concepts with Explanation
1. What is the main principle of OOPs?
a) Inheritance
b) Encapsulation
c) Abstraction
d) Polymorphism
Answer: b) Encapsulation
Explanation: Encapsulation is one of the main principles of OOPs, and it refers to the bundling of data with the methods that operate on that data. It is a technique that keeps data and code safe from external interference and misuse.
2. Which of the following is an example of inheritance in Java?
a) A class containing private fields and public methods
b) A class inheriting from multiple parent classes
c) A subclass inheriting the methods and properties of its parent class
d) A subclass overriding the methods of its parent class
Answer: c) A subclass inheriting the methods and properties of its parent class
Explanation: Inheritance is the process by which one class acquires the properties and methods of another class. In Java, a subclass can inherit the methods and properties of its parent class using the "extends" keyword.
3. What is the purpose of abstraction in Java?
a) To simplify the code
b) To reduce the number of classes in a program
c) To hide the complexity of the code and provide a simpler interface
d) To improve performance
Answer: c) To hide the complexity of the code and provide a simpler interface
Explanation: Abstraction is the process of hiding the complexity of the code and providing a simpler interface for the user. In Java, abstraction is achieved using abstract classes and interfaces.
4. What is the difference between overloading and overriding in Java?
a) Overloading allows multiple methods with the same name in the same class, while overriding allows a subclass to provide a specific implementation of a method already defined in its parent class.
b) Overloading allows a subclass to provide a specific implementation of a method already defined in its parent class, while overriding allows multiple methods with the same name in the same class.
c) Overloading and overriding are the same thing.
d) Overloading and overriding are not possible in Java.
Answer: a) Overloading allows multiple methods with the same name in the same class, while overriding allows a subclass to provide a specific implementation of a method already defined in its parent class.
Explanation: Overloading is a feature that allows a class to have multiple methods with the same name but different parameters, while overriding is a feature that allows a subclass to provide a specific implementation of a method already defined in its parent class.
5. What is a constructor in Java?
a) A method that is used to initialize objects
b) A method that is used to copy objects
c) A method that is used to compare objects
d) A method that is used to destroy objects
Answer: a) A method that is used to initialize objects
Explanation: A constructor is a special method in Java that is used to initialize objects of a class. It is called when an object of the class is created and is used to set the initial values of the object's fields.
6. What is polymorphism in Java?
a) The ability of a class to inherit from multiple parent classes
b) The ability of a subclass to provide a specific implementation of a method already defined in its parent class
c) The ability of a class to have multiple methods with the same name but different parameters
d) The ability of a class to hide its complexity and provide a simpler interface
Answer: c) The ability of a class to have multiple methods with the same name but different parameters
Explanation: Polymorphism is a feature in Java that allows a class to have multiple methods with the same name but different parameters.
7. What is inheritance in Java?
A. Inheritance is a mechanism in which one class acquires the properties (methods and fields) of another class. The class that inherits the properties is known as a subclass or derived class, and the class whose properties are inherited is known as the superclass or base class.
8. What is encapsulation in Java?
A. Encapsulation is a mechanism that bundles the data and methods that operate on the data within a single unit, called a class. It protects the data from being accessed by other classes directly and provides control over data access by providing getters and setters methods.
9. What is polymorphism in Java?
A. Polymorphism is a mechanism that allows objects of different classes to be treated as if they belong to the same class. It can be achieved in Java through method overloading and method overriding.
10. What is abstraction in Java?
A. Abstraction is a mechanism that allows us to hide the implementation details and provide a simplified interface for the user. It can be achieved in Java through abstract classes and interfaces.
11. What is a constructor in Java?
A. A constructor is a special method that is used to initialize the object of a class. It has the same name as the class and doesn't have a return type.
12. What is method overloading in Java?
A. Method overloading is a feature in Java that allows a class to have multiple methods with the same name but different parameters. The method that gets invoked depends on the arguments passed to it.
13. What is method overriding in Java?
A. Method overriding is a feature in Java that allows a subclass to provide its own implementation of a method that is already defined in its superclass.
14. What is the difference between an abstract class and an interface in Java?
A. An abstract class can have both abstract and non-abstract methods, while an interface can only have abstract methods. An abstract class can have instance variables, but an interface cannot. A class can extend only one abstract class, but it can implement multiple interfaces.
15. What is the purpose of the final keyword in Java?
A. The final keyword can be used to make a variable, method, or class constant and unchangeable. A final variable cannot be reassigned, a final method cannot be overridden, and a final class cannot be subclassed.
16. What is the difference between static and non-static methods in Java?
A. A static method belongs to the class, and it can be called without creating an object of the class. A non-static method belongs to an object of the class, and it can be called only through an object of the class.
17. What is concurrency in Java?
a) It is the ability to execute multiple threads in parallel
b) It is the ability to execute multiple threads in sequence
c) It is the ability to execute a single thread in parallel
d) It is the ability to execute a single thread in sequence
Answer: a) It is the ability to execute multiple threads in parallel.
Explanation: Concurrency is the ability to execute multiple threads simultaneously, allowing for better utilization of resources and faster program execution.
18. What is the purpose of synchronization in Java?
a) To prevent multiple threads from accessing shared resources simultaneously
b) To allow multiple threads to access shared resources simultaneously
c) To improve the performance of the program
d) None of the above
Answer: a) To prevent multiple threads from accessing shared resources simultaneously.
Explanation: Synchronization is the process of ensuring that only one thread can access a shared resource at a time, preventing race conditions and other concurrency issues.
19. Which of the following is an example of a synchronized method in Java?
a) public void add(int x) { count += x; }
b) public synchronized void add(int x) { count += x; }
c) public static synchronized void add(int x) { count += x; }
d) Both b and c
Answer: d) Both b and c.
Explanation: In Java, a synchronized method is a method that is marked with the synchronized keyword, which ensures that only one thread can execute the method at a time. Both option b and c are examples of synchronized methods.
20. What is the difference between a thread and a process in Java?
a) A thread is a lightweight process and shares the same memory space as the parent process, while a process is a heavyweight entity and has its own memory space
b) A process is a lightweight entity and shares the same memory space as the parent thread, while a thread is a heavyweight entity and has its own memory space
c) A thread and a process are the same thing in Java
d) None of the above
Answer: a) A thread is a lightweight process and shares the same memory space as the parent process, while a process is a heavyweight entity and has its own memory space.
Explanation: In Java, a thread is a lightweight process that runs within the context of a parent process and shares its memory space, while a process is a heavyweight entity that has its own memory space and runs independently of other processes.
21. Which of the following methods can be used to start a new thread in Java?
a) run()
b) start()
c) yield()
d) wait()
Answer: b) start().
Explanation: To start a new thread in Java, you must call the start() method on an instance of the Thread class. The run() method is the code that will be executed when the thread is started, but it should not be called directly.
21. What is a deadlock in Java?
a) A situation where two or more threads are waiting for each other to release a resource, resulting in a state of permanent waiting
b) A situation where a thread enters an infinite loop
c) A situation where a thread consumes too much memory
d) None of the above
Answer: a) A situation where two or more threads are waiting for each other to release a resource, resulting in a state of permanent waiting.
Explanation: Deadlock is a situation where two or more threads are waiting for each other to release a resource, resulting in a state of permanent waiting and a program that is stuck and cannot continue.
22. What is the difference between a thread and a process in Java?
Answer: A thread is a lightweight sub-process, while a process is a heavyweight sub-process. Threads share a common memory space, while processes do not. Multiple threads within the same process can share data and communicate with each other, while processes have their own memory space and cannot share data directly.
23. What is the purpose of the synchronized keyword in Java?
Answer: The synchronized keyword is used to provide exclusive access to shared resources in a multi-threaded environment. When a method or block of code is marked as synchronized, only one thread can execute that code at a time, preventing multiple threads from accessing the shared resource simultaneously and leading to potential data corruption or inconsistency.
24. What is the Java Executor framework?
Answer: The Java Executor framework is a high-level API for managing threads and thread pools. It provides a simple interface for creating and executing tasks asynchronously, allowing for efficient use of system resources and minimizing the overhead associated with thread creation and destruction. The framework includes a number of pre-defined thread pool implementations and allows for custom configuration and tuning.
25. What is a deadlock in Java?
Answer: A deadlock occurs in Java when two or more threads are waiting for each other to release a resource that they both need to proceed, resulting in a situation where none of the threads can make progress. This can lead to a system freeze or other undesirable behavior. Deadlocks can occur when locking resources with synchronized blocks or when using low-level synchronization mechanisms such as wait and notify.
26. What is the purpose of the volatile keyword in Java?
Answer: The volatile keyword in Java is used to indicate that a variable's value may be modified by multiple threads and therefore should not be cached in a thread's local memory. When a variable is declared as volatile, it is guaranteed to be read and written to directly from main memory, ensuring that all threads have access to the latest value. This can help prevent race conditions and other concurrency issues.
People also read
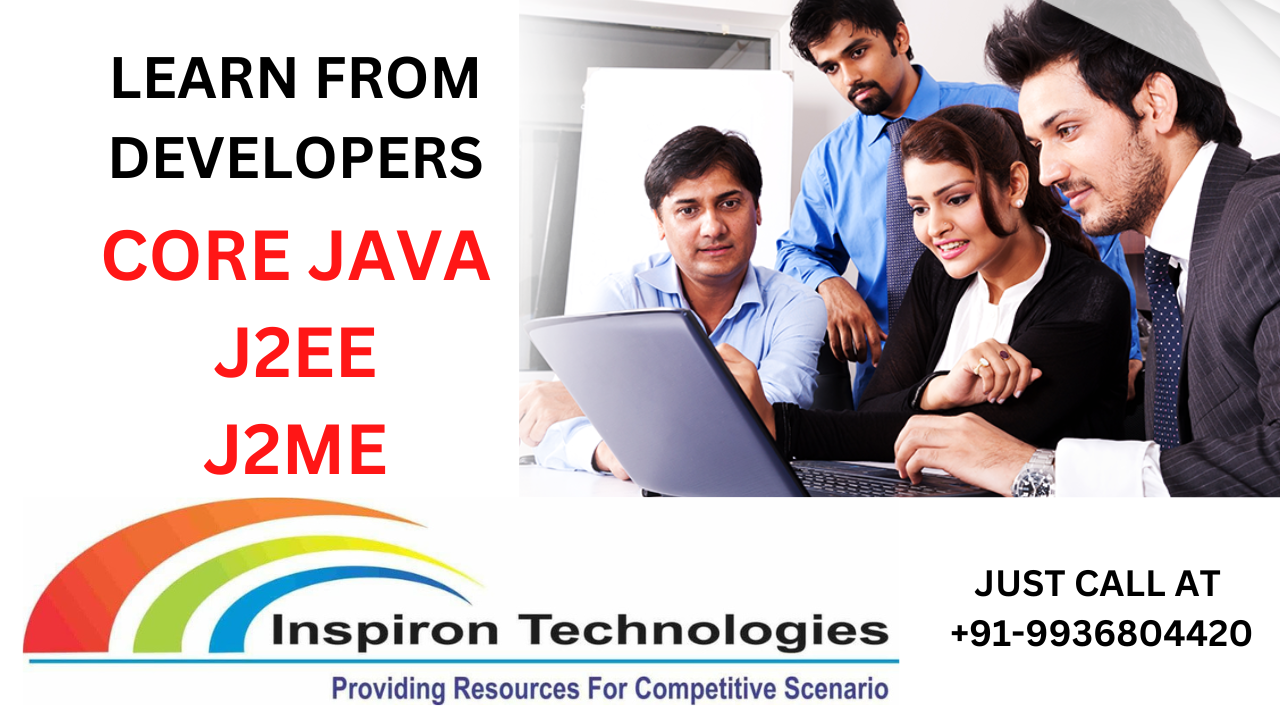
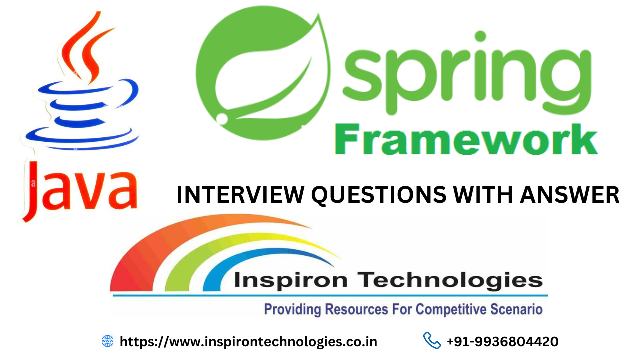
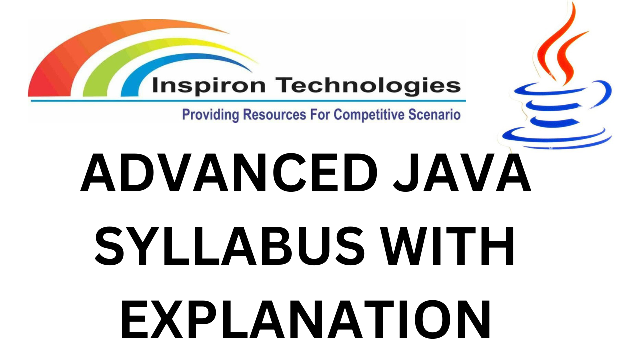
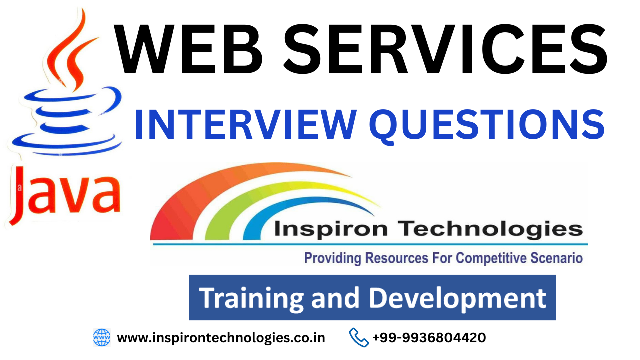
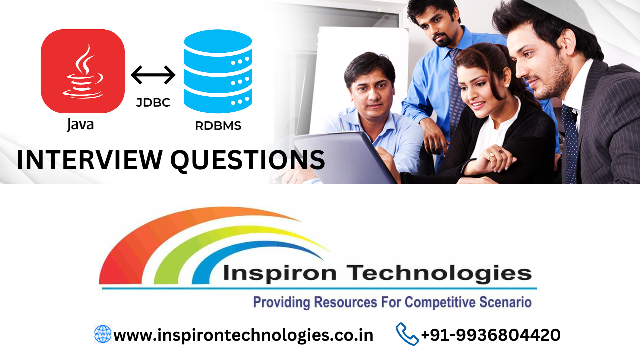
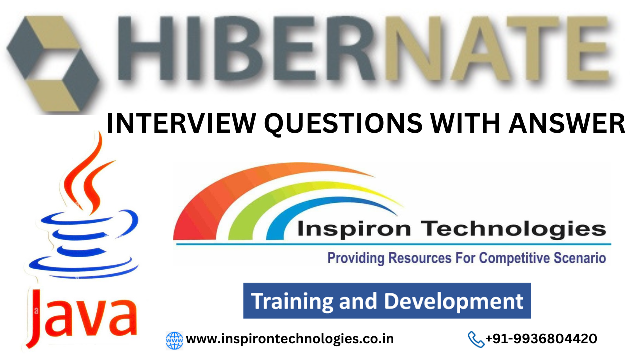
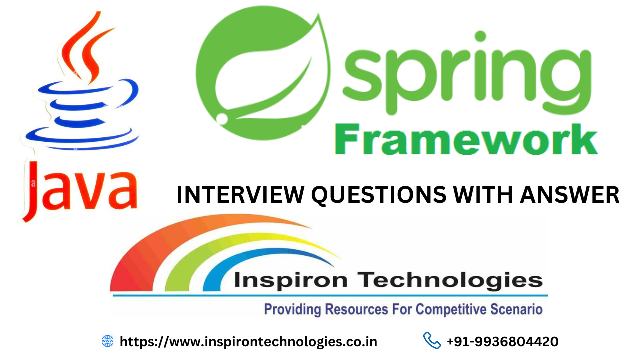
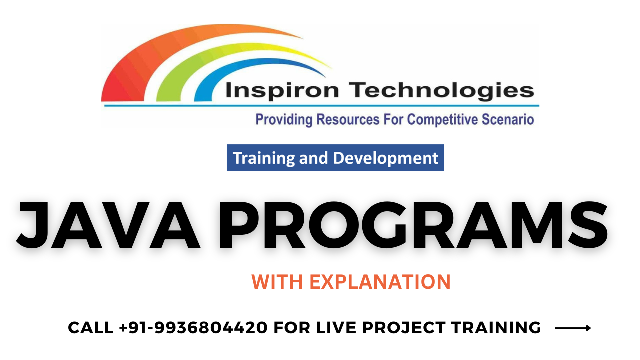
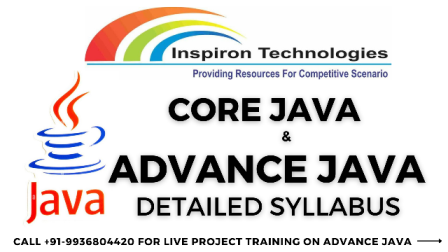
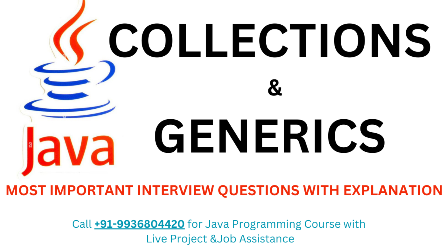
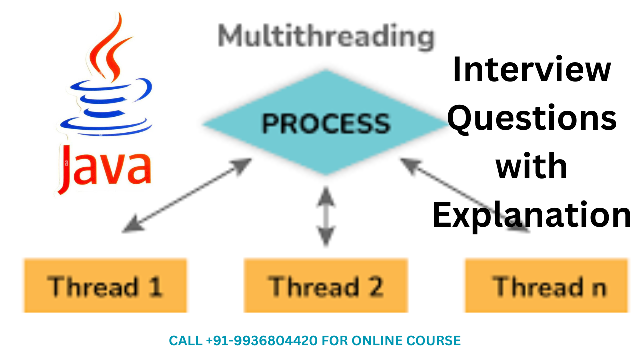
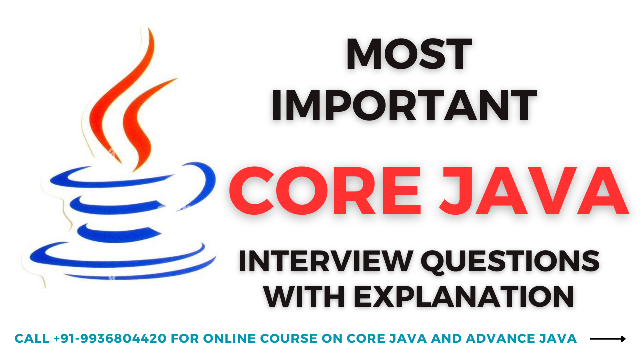
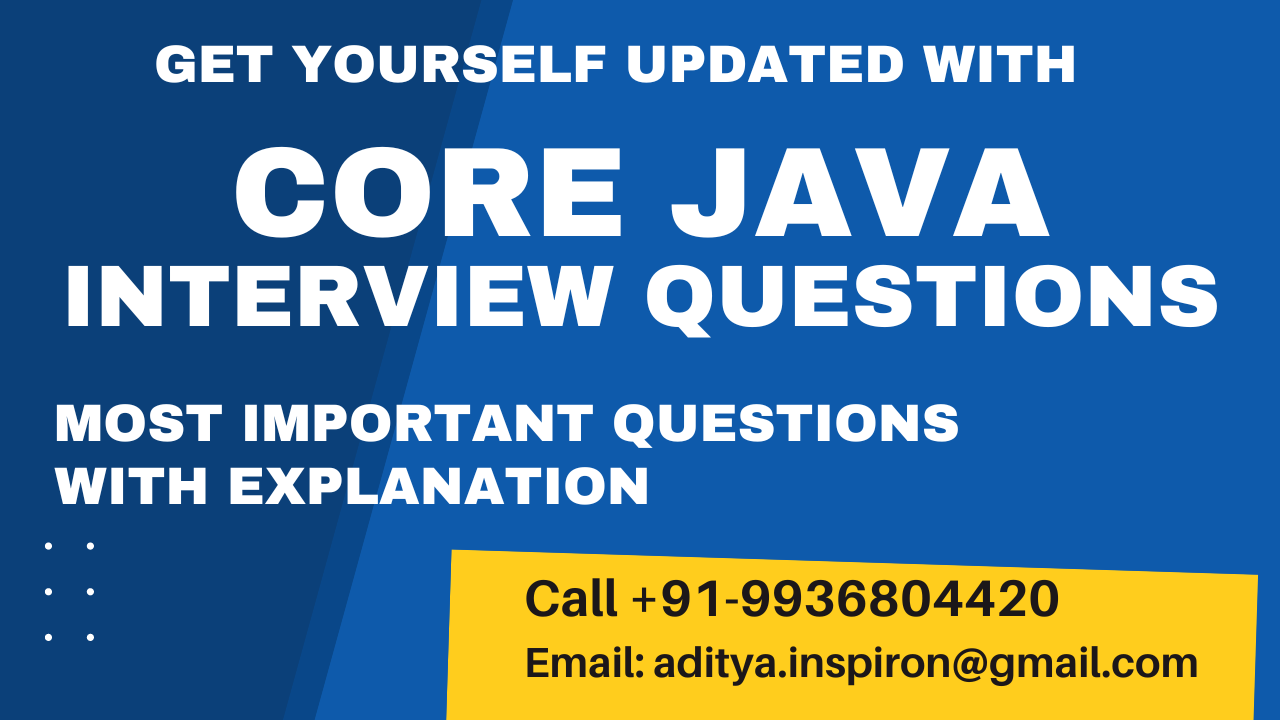
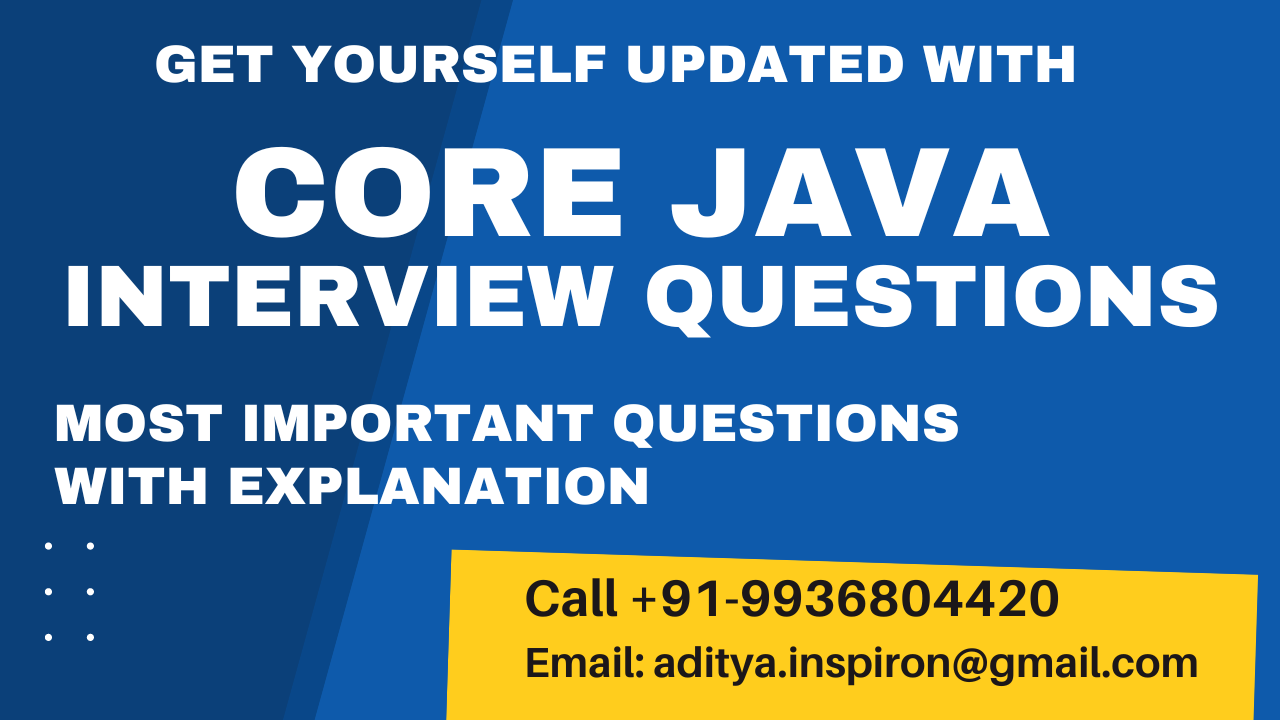
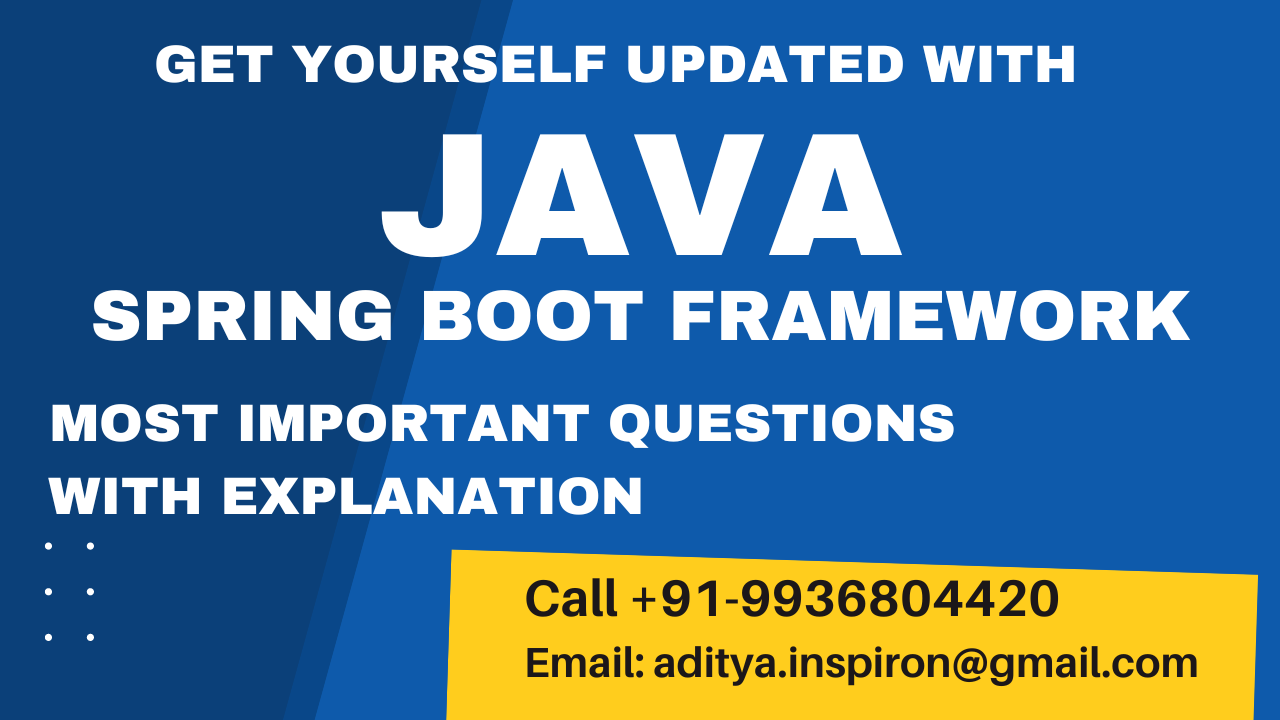
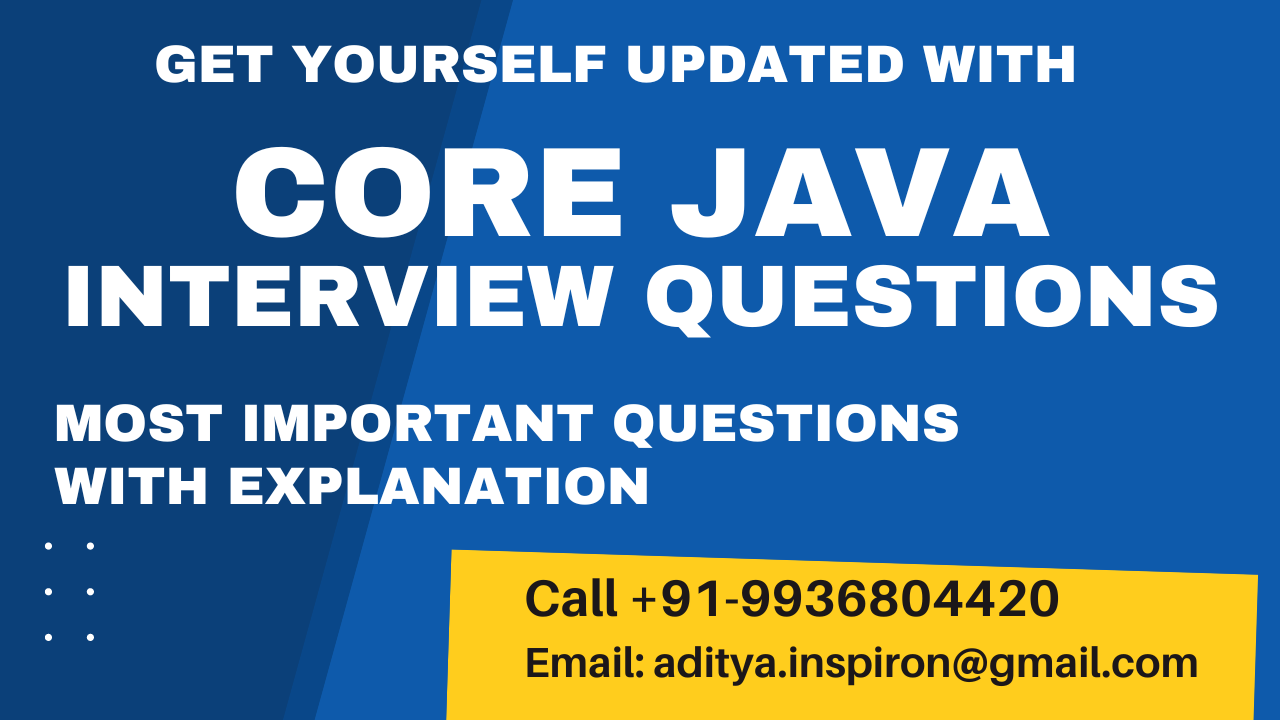
Categories
Popular Post
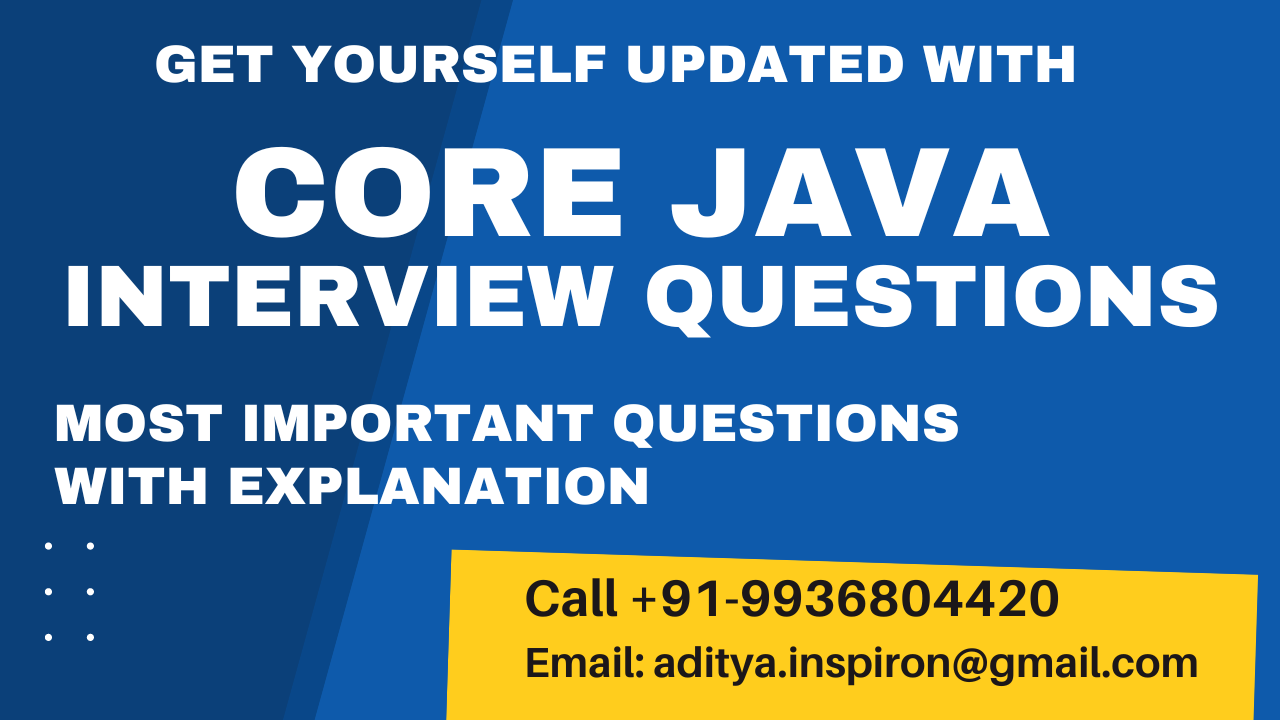
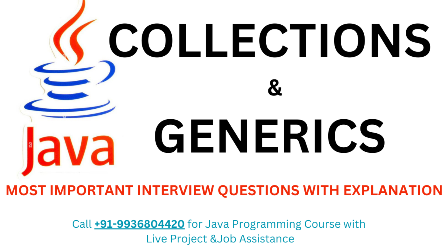
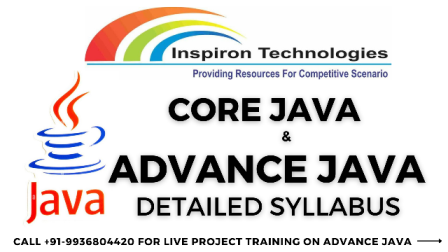
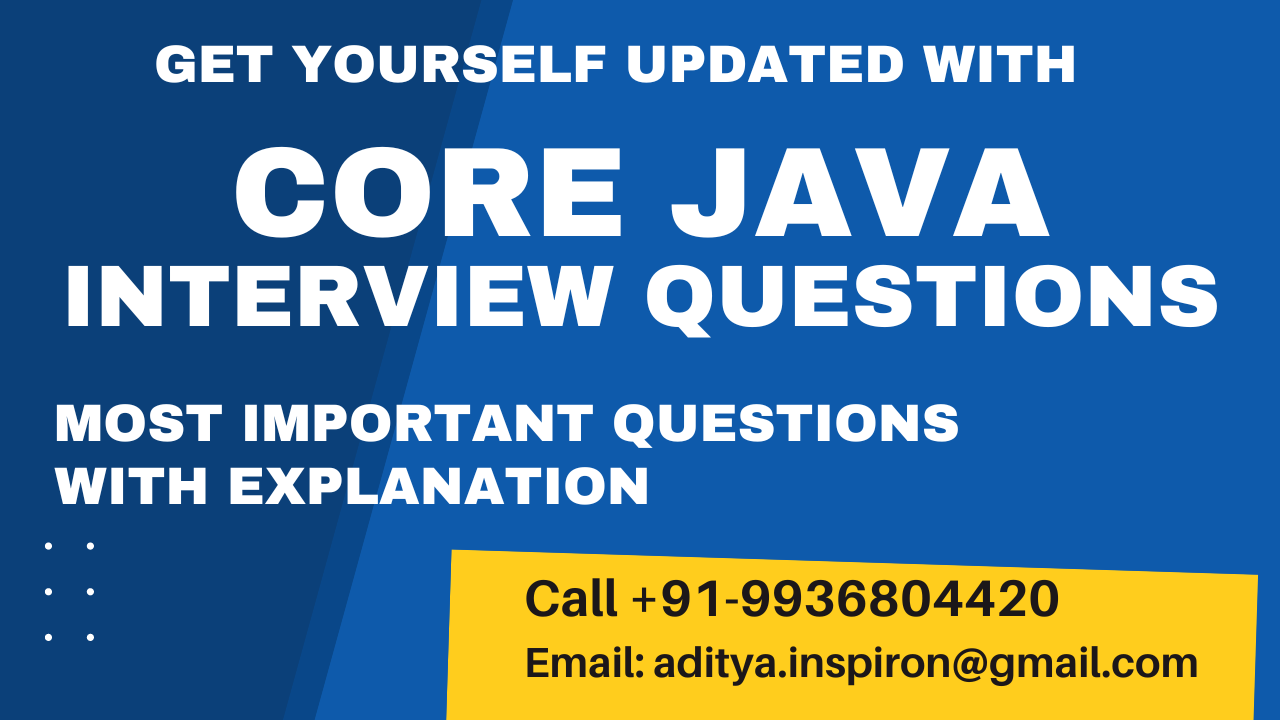
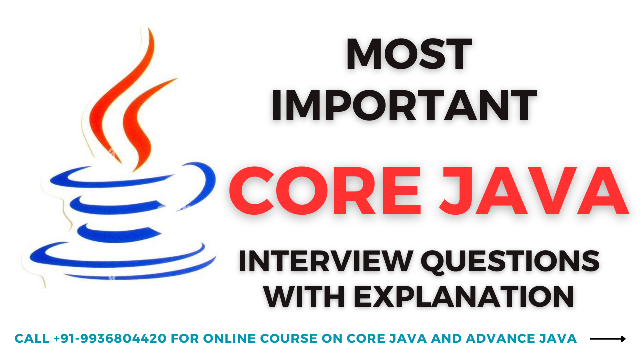
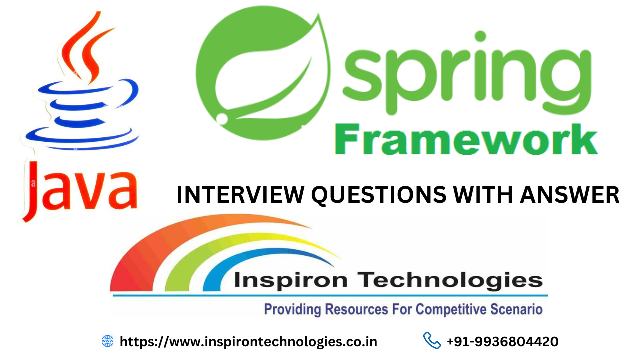
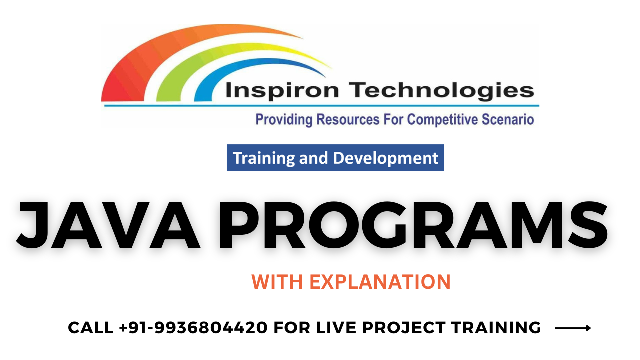
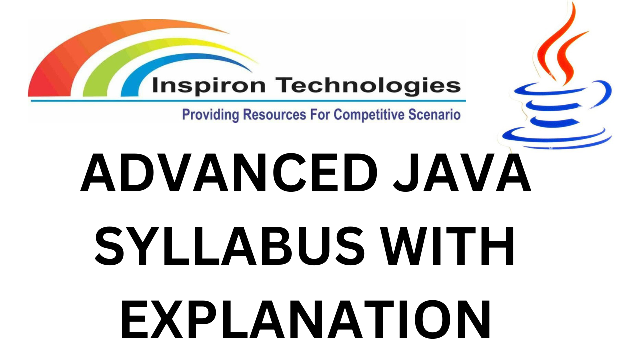
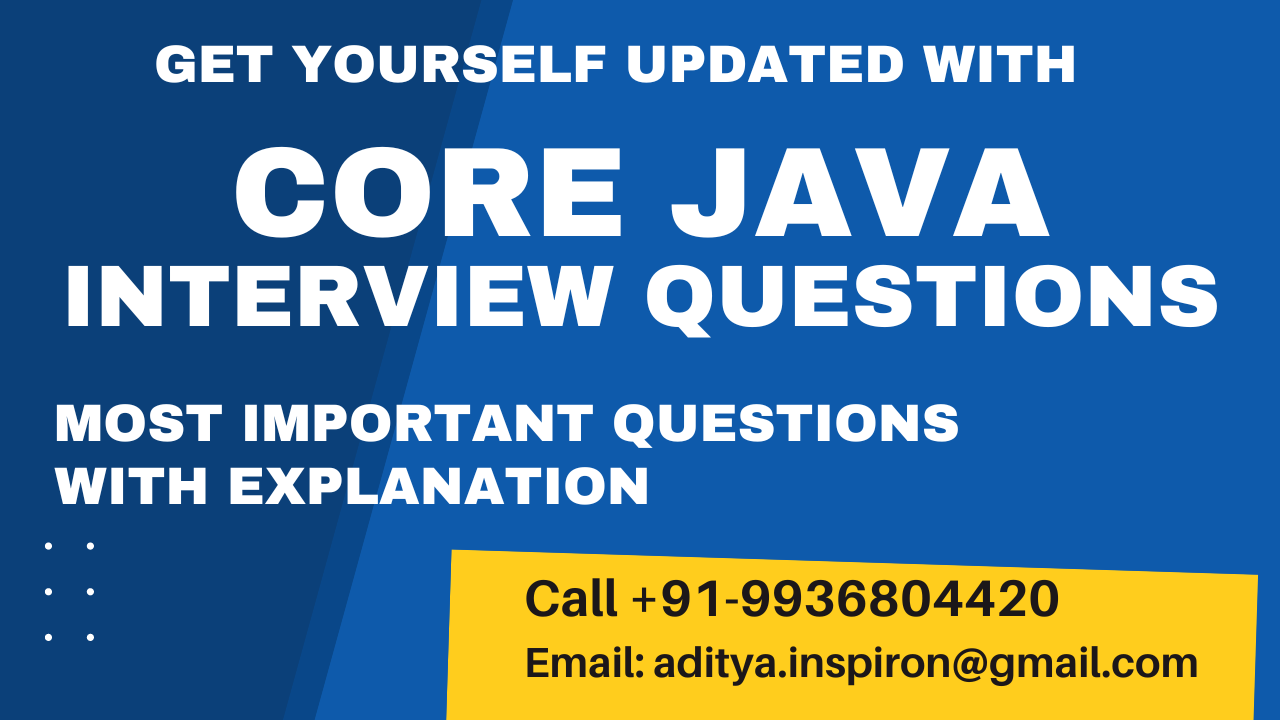
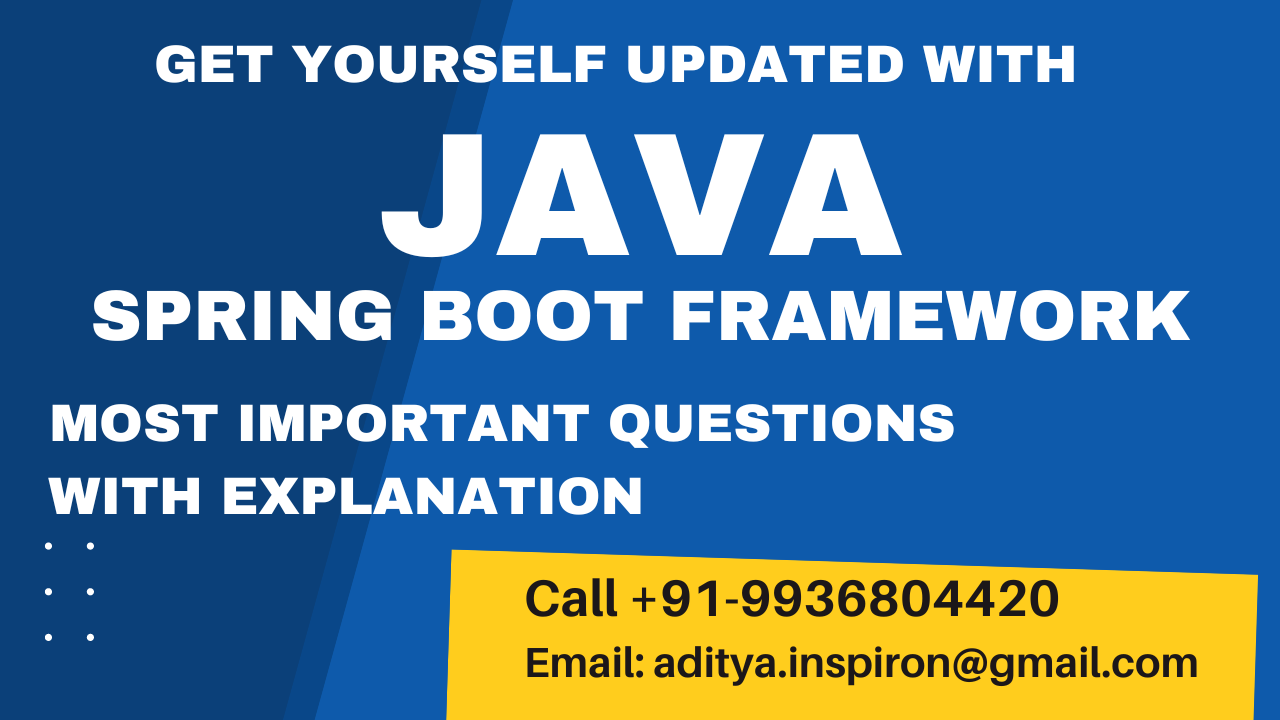
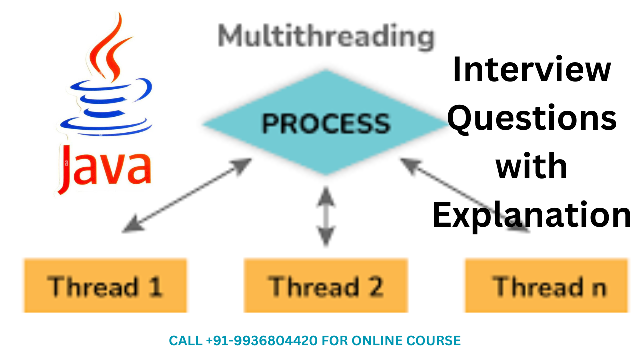
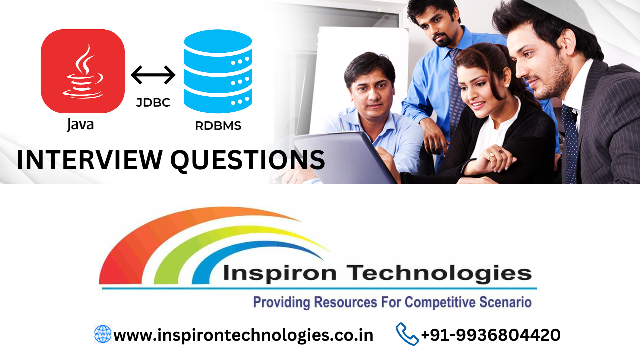
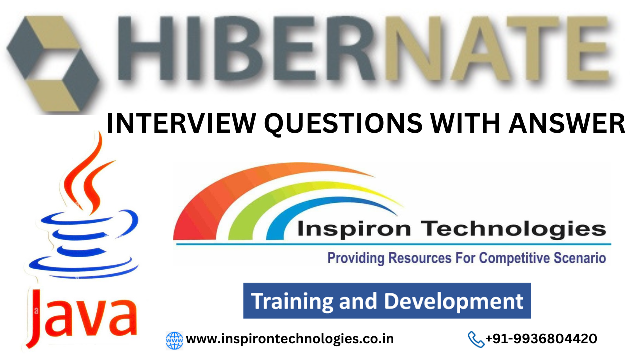
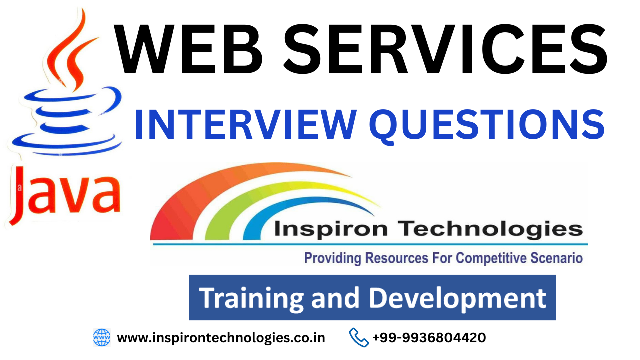
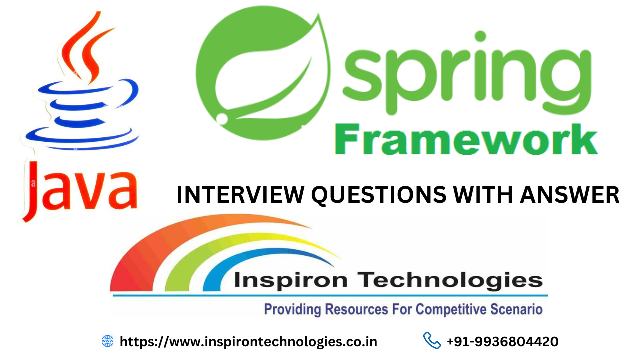
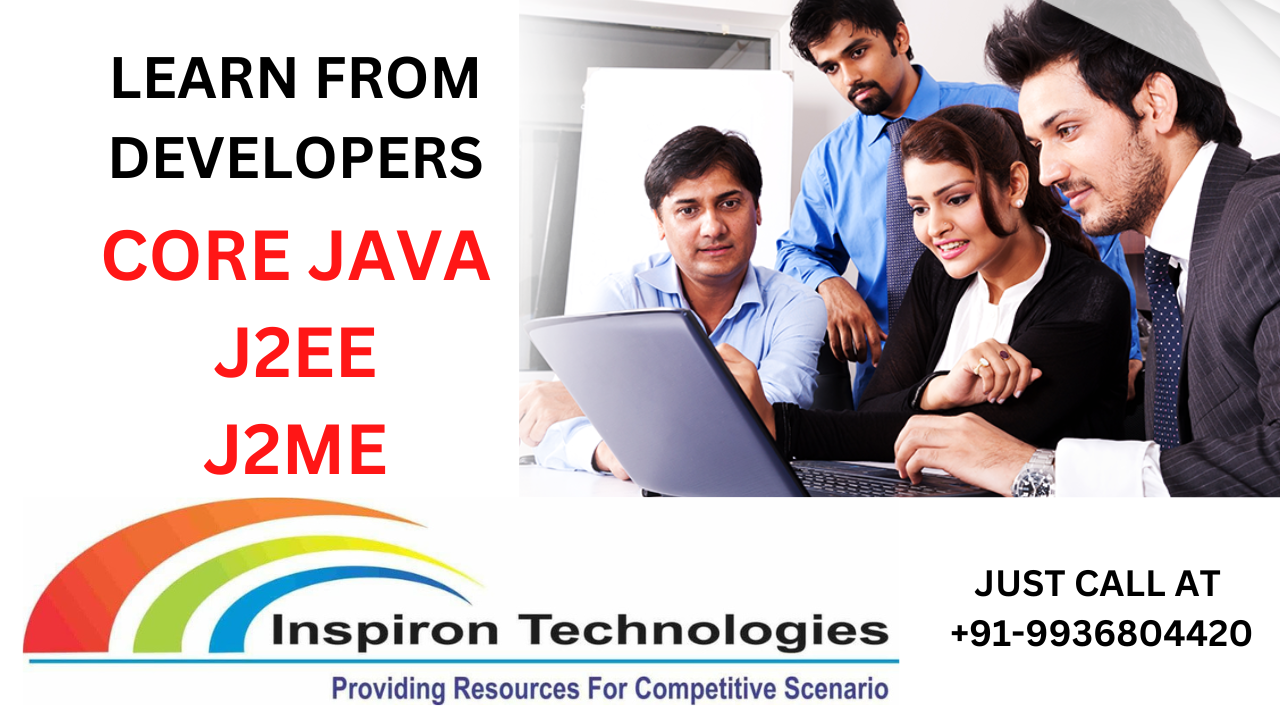
Leave a comment