Java Programs for Beginners
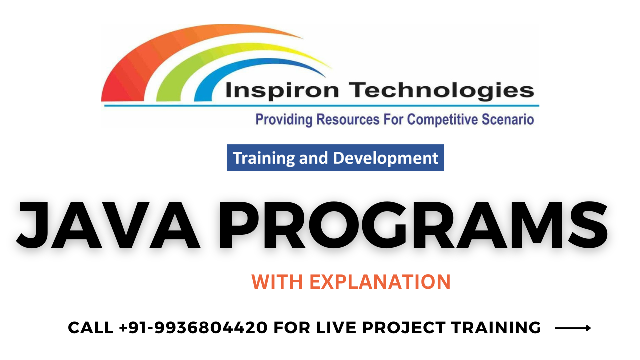
Java Programs for Practice | Java Programming Examples
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Explanation: This program simply prints the message "Hello, World!" to the console. It demonstrates the basic structure of a Java program, where the main method is the entry point.
Sum of Two Numbers:
public class Sum {
public static void main(String[] args) {
int num1 = 5;
int num2 = 10;
int sum = num1 + num2;
System.out.println("Sum: " + sum);
}
}
Explanation: This program calculates the sum of two numbers, num1 and num2. It initializes the variables with the values 5 and 10, respectively, and adds them to obtain the sum. Finally, it displays the result using System.out.println().
Fibonacci Series:
public class Fibonacci {
public static void main(String[] args) {
int n = 10;
int firstNum = 0;
int secondNum = 1;
System.out.print("Fibonacci Series: " + firstNum + ", " + secondNum);
for (int i = 2; i < n; i++) {
int nextNum = firstNum + secondNum;
System.out.print(", " + nextNum);
firstNum = secondNum;
secondNum = nextNum;
}
}
}
Explanation: This program generates and prints the Fibonacci series up to a given number, n. It starts with the first two numbers, 0 and 1, and then calculates the next number by adding the previous two numbers. The loop iterates n-2 times and displays each number in the series.
Factorial Calculation:
public class Factorial {
public static void main(String[] args) {
int n = 5;
int factorial = 1;
for (int i = 1; i <= n; i++) {
factorial *= i;
}
System.out.println("Factorial of " + n + " is: " + factorial);
}
}
Explanation: This program calculates the factorial of a given number, n. It uses a loop to multiply the numbers from 1 to n to obtain the factorial. The final result is displayed using System.out.println().
Prime Number Check:
public class PrimeNumber {
public static void main(String[] args) {
int number = 13;
boolean isPrime = true;
if (number <= 1) {
isPrime = false;
} else {
for (int i = 2; i <= Math.sqrt(number); i++) {
if (number % i == 0) {
isPrime = false;
break;
}
}
}
if (isPrime) {
System.out.println(number + " is a prime number.");
} else {
System.out.println(number + " is not a prime number.");
}
}
}
Explanation: This program checks whether a given number is prime or not. It first checks if the number is less than or equal to 1, in which case it is not considered prime. Otherwise, it iterates from 2 to the square root of the number and checks if any number divides the given number evenly. If such a number is found, it sets isPrime to false and breaks out of the loop. Finally, it displays the result based on the value of isPrime.
Reverse a String:
public class ReverseString {
public static void main(String[] args) {
String originalString = "Hello, World!";
String reversedString = "";
for (int i = originalString.length() - 1; i >= 0; i--) {
reversedString += originalString.charAt(i);
}
System.out.println("Original String: " + originalString);
System.out.println("Reversed String: " + reversedString);
}
}
Explanation: This program reverses a given string. It starts from the last character of the original string and iterates backwards, appending each character to the reversedString variable. Finally, it displays both the original and reversed strings using System.out.println().
Leap Year Check:
public class LeapYear {
public static void main(String[] args) {
int year = 2024;
boolean isLeapYear = false;
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
isLeapYear = true;
}
} else {
isLeapYear = true;
}
}
if (isLeapYear) {
System.out.println(year + " is a leap year.");
} else {
System.out.println(year + " is not a leap year.");
}
}
}
Explanation: This program checks if a given year is a leap year. It follows the rules for leap year calculation, where if a year is divisible by 4, it is a leap year. However, if it is also divisible by 100, it must also be divisible by 400 to be considered a leap year. The program sets the isLeapYear flag based on these conditions and displays the result accordingly.
Palindrome Check:
public class Palindrome {
public static void main(String[] args) {
String word = "level";
boolean isPalindrome = true;
for (int i = 0; i < word.length() / 2; i++) {
if (word.charAt(i) != word.charAt(word.length() - 1 - i)) {
isPalindrome = false;
break;
}
}
if (isPalindrome) {
System.out.println(word + " is a palindrome.");
} else {
System.out.println(word + " is not a palindrome.");
}
}
}
Explanation: This program checks whether a given word is a palindrome or not. It compares the characters from the beginning and end of the word, gradually moving towards the middle. If at any point the characters don't match, it sets isPalindrome to false and breaks out of the loop. Finally, it displays the result based on the value of isPalindrome.
Array Sum:
public class ArraySum {
public static void main(String[] args) {
int[] numbers = {2, 4, 6, 8, 10};
int sum = 0;
for (int number : numbers) {
sum += number;
}
System.out.println("Sum: " + sum);
}
}
Explanation: This program calculates the sum of all the elements in an array. It uses an enhanced for loop to iterate through each element and adds it to the sum variable. Finally, it displays the sum using System.out.println().
Armstrong Number Check:
public class ArmstrongNumber {
public static void main(String[] args) {
int number = 153;
int originalNumber = number;
int result = 0;
int remainder;
while (originalNumber != 0) {
remainder = originalNumber % 10;
result += Math.pow(remainder, 3);
originalNumber /= 10;
}
if (result == number) {
System.out.println(number + " is an Armstrong number.");
} else {
System.out.println(number + " is not an Armstrong number.");
}
}
}
Explanation: This program checks whether a given number is an Armstrong number or not. It calculates the sum of cubes of individual digits in the number. The program uses a while loop to extract each digit from the original number and adds its cube to the result variable. Finally, it compares the result with the original number and displays the result accordingly.
Prime Numbers in a Range:
public class PrimeNumbersInRange {
public static void main(String[] args) {
int start = 10;
int end = 50;
System.out.println("Prime numbers between " + start + " and " + end + ":");
for (int number = start; number <= end; number++) {
boolean isPrime = true;
if (number <= 1) {
isPrime = false;
} else {
for (int i = 2; i <= Math.sqrt(number); i++) {
if (number % i == 0) {
isPrime = false;
break;
}
}
}
if (isPrime) {
System.out.print(number + " ");
}
}
}
}
Explanation: This program prints all prime numbers within a given range. It iterates through each number in the range and performs a prime number check using a similar logic as explained earlier. If a number is prime, it is printed to the console.
Factorial using Recursion:
public class FactorialRecursion {
public static void main(String[] args) {
int n = 5;
int factorial = calculateFactorial(n);
System.out.println("Factorial of " + n + " is: " + factorial);
}
public static int calculateFactorial(int num) {
if (num == 0) {
return 1;
} else {
return num * calculateFactorial(num - 1);
}
}
}
Explanation: This program calculates the factorial of a given number using recursion. The calculateFactorial method is defined to recursively calculate the factorial. It checks the base case where the number is 0 and returns 1. Otherwise, it multiplies the number with the factorial of the number minus one. The result is obtained by calling this method and displayed in the main method.
Binary Search:
public class BinarySearch {
public static void main(String[] args) {
int[] numbers = {2, 5, 8, 12, 16, 23, 38, 56, 72, 91};
int target = 23;
int index = performBinarySearch(numbers, target);
if (index != -1) {
System.out.println("Element found at index " + index);
} else {
System.out.println("Element not found");
}
}
public static int performBinarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
}
Explanation: This program performs a binary search on a sorted array to find a target element. The performBinarySearch method takes the array and target element as parameters. It initializes the left and right pointers to the start and end of the array, respectively. The method iteratively divides the array in half, compares the middle element with the target, and adjusts the pointers accordingly. If the target element is found, the method returns the index; otherwise, it returns -1.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
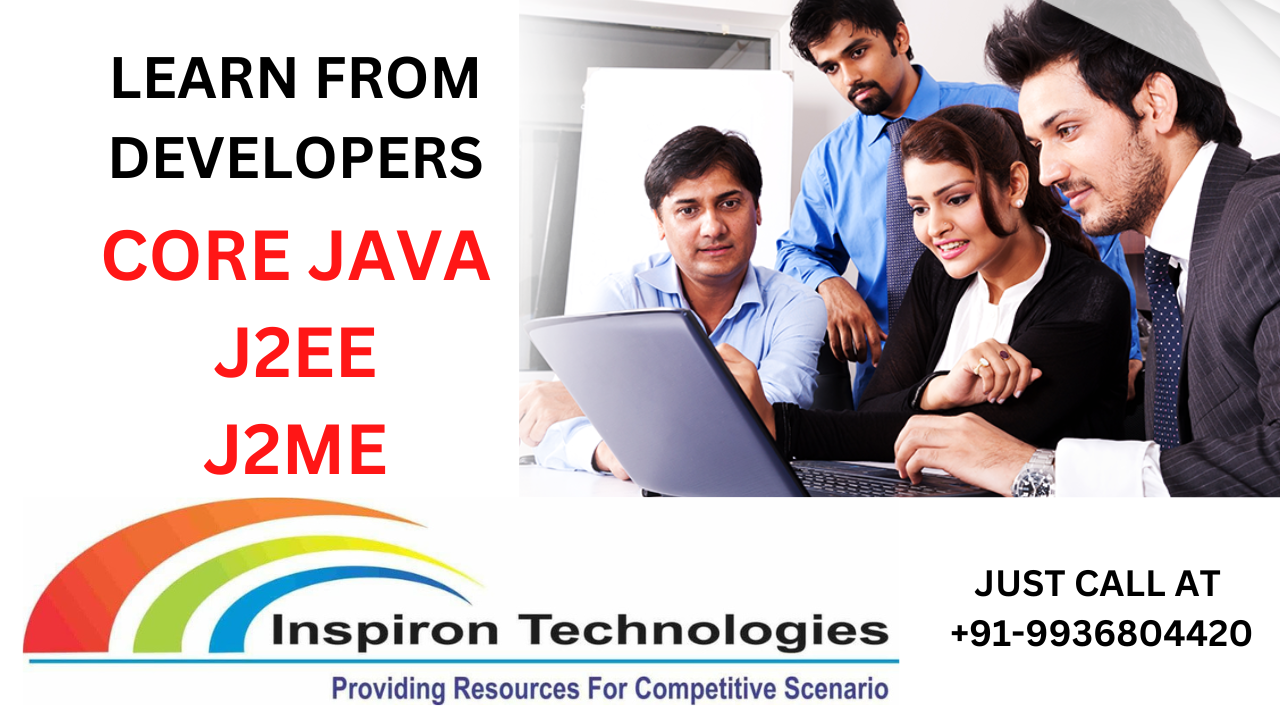
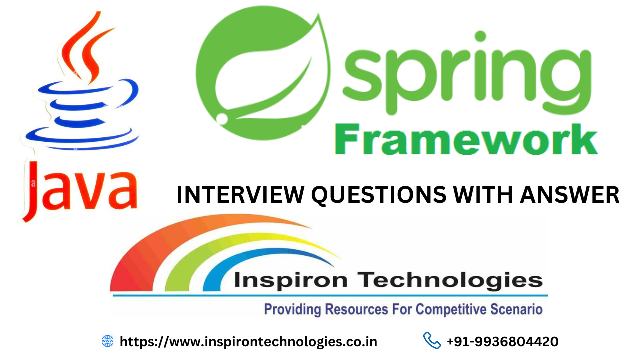
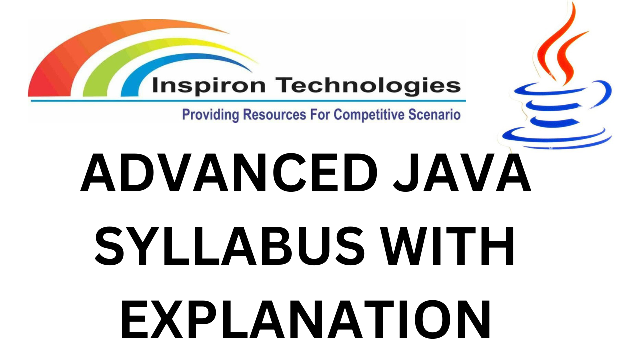
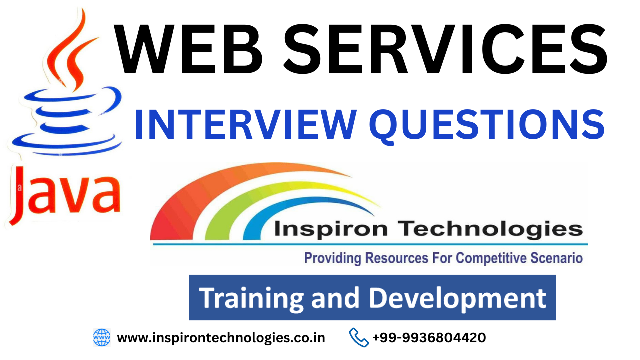
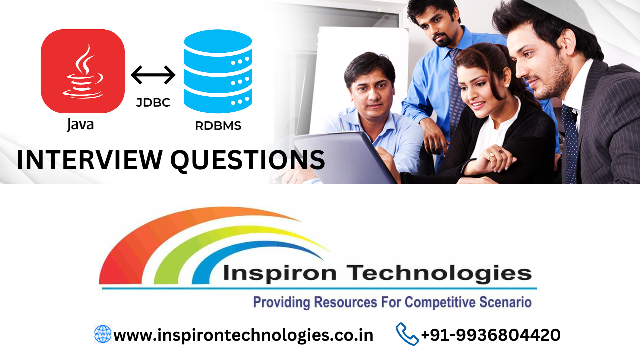
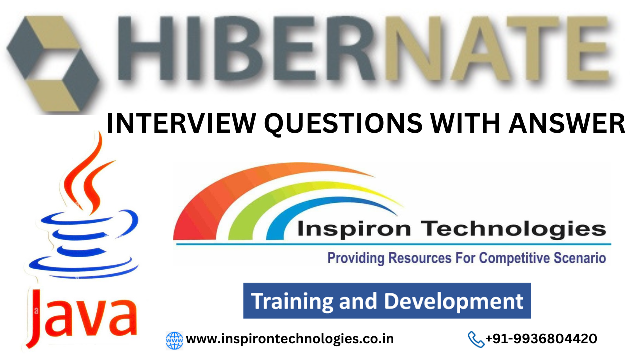
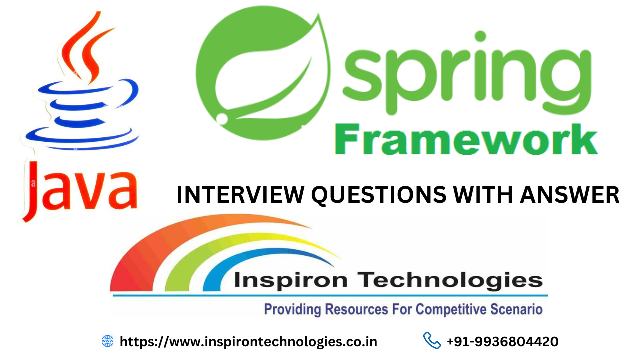
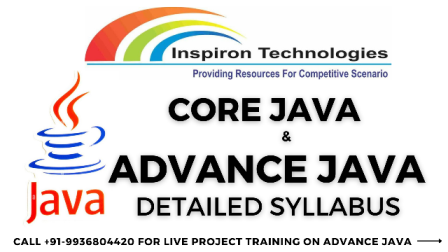
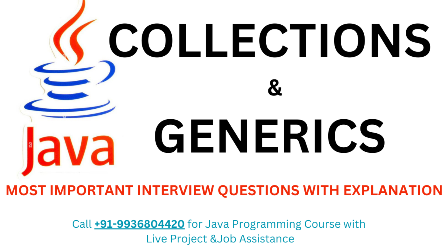
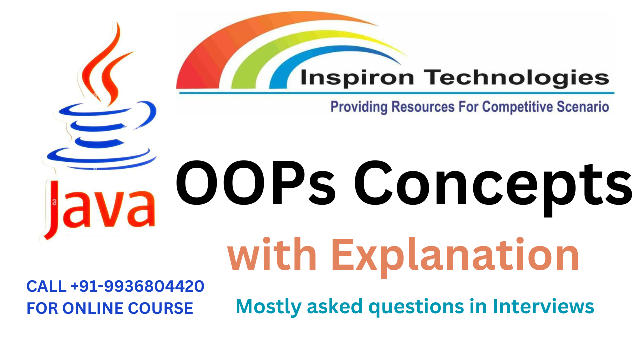
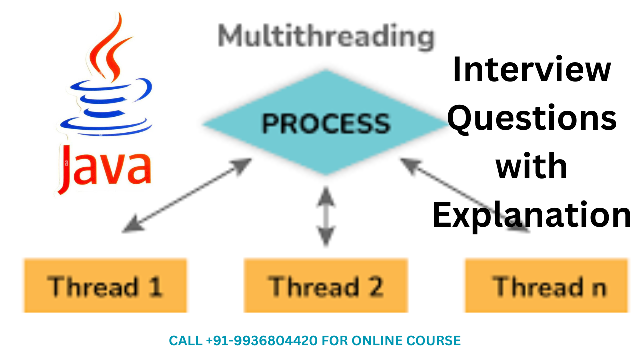
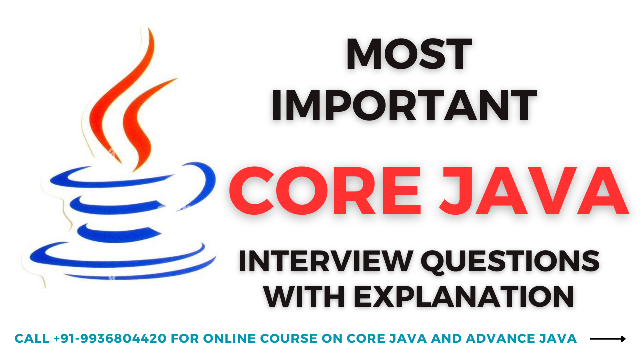
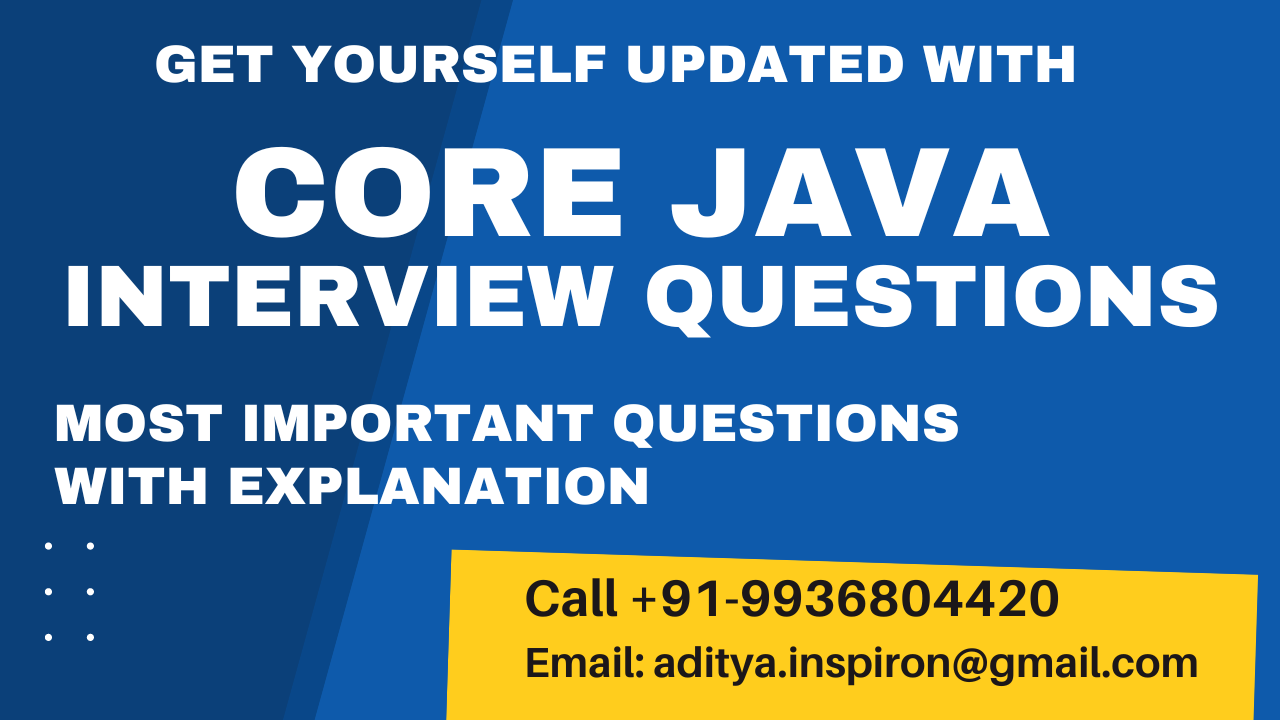
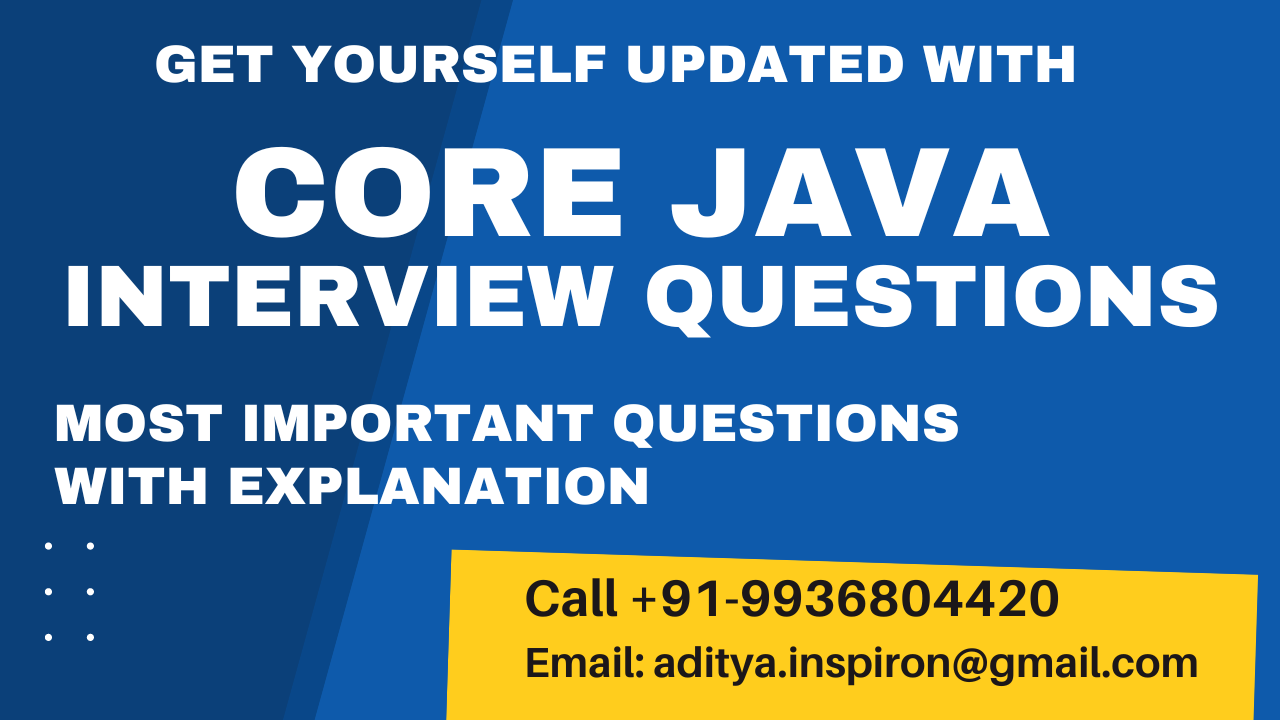
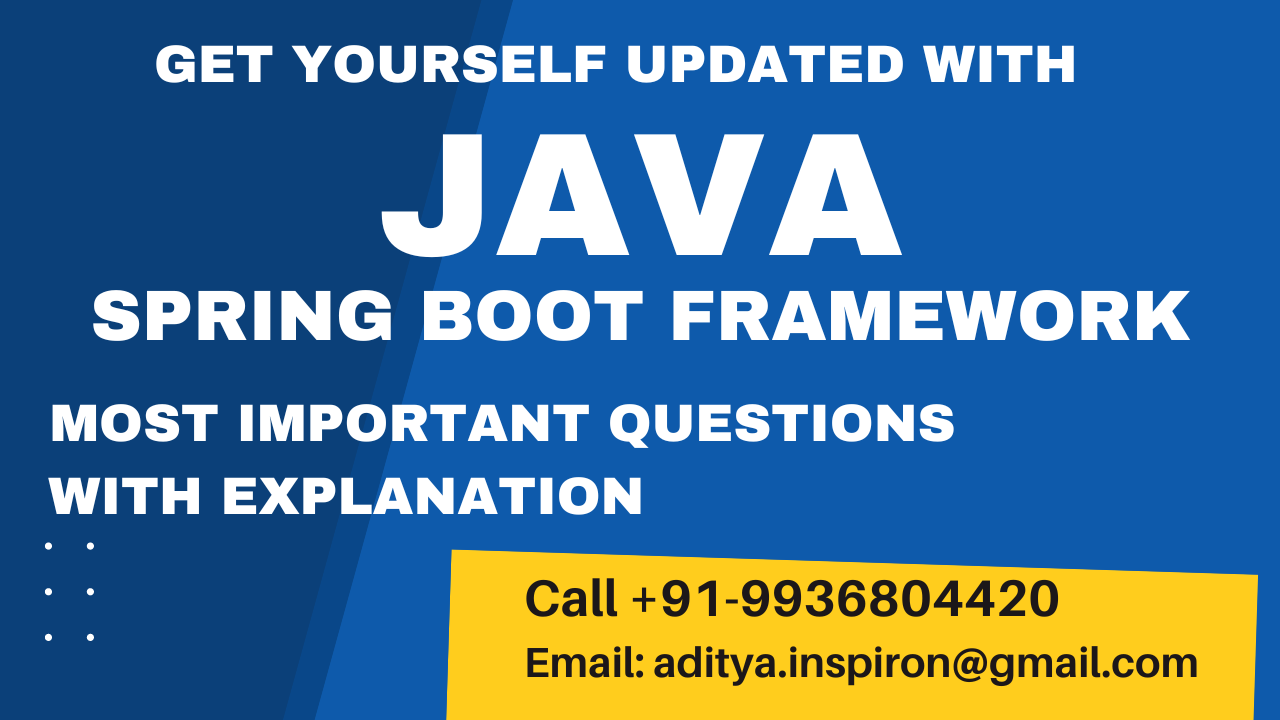
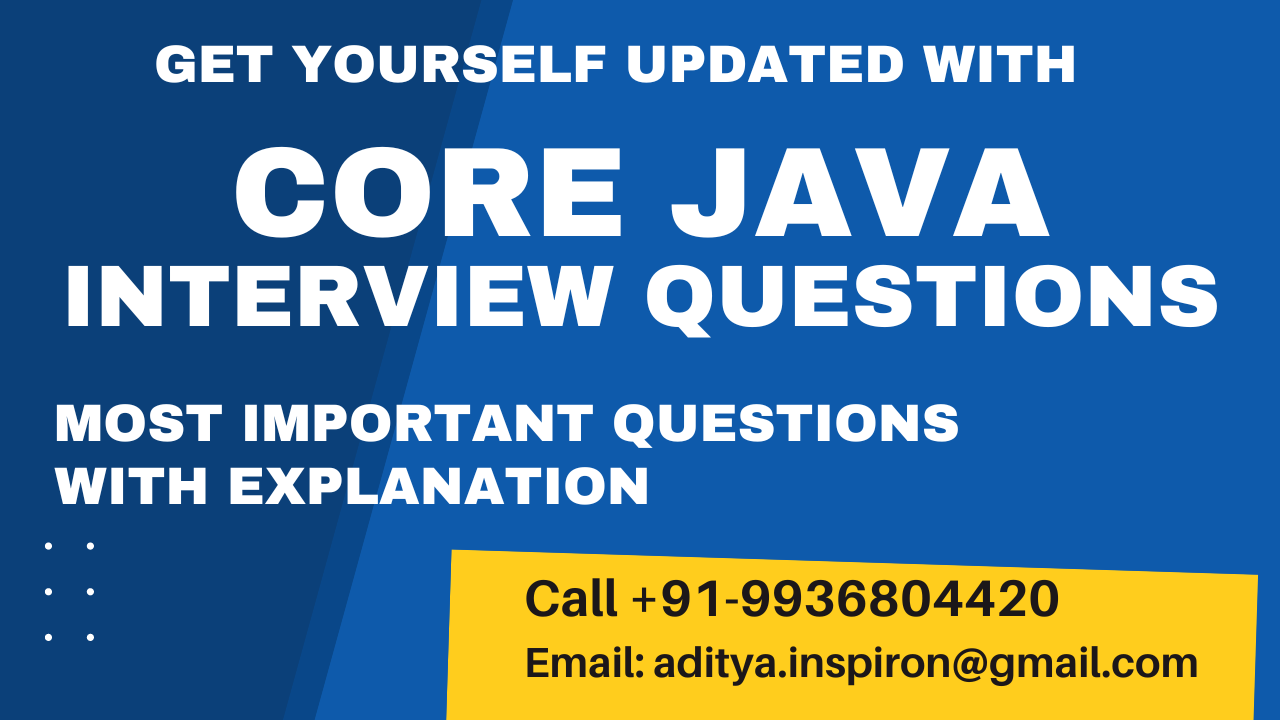
Categories
Popular Post
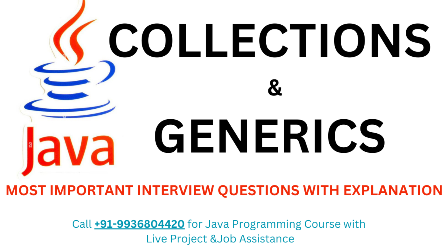
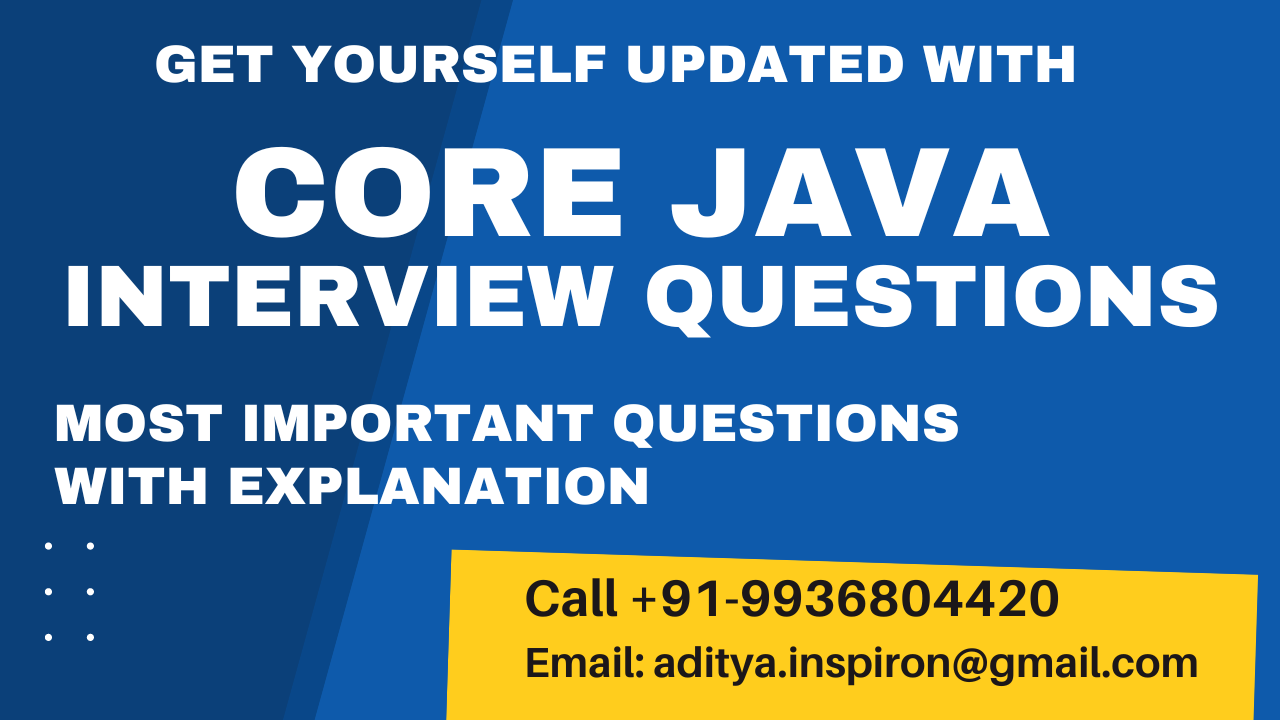
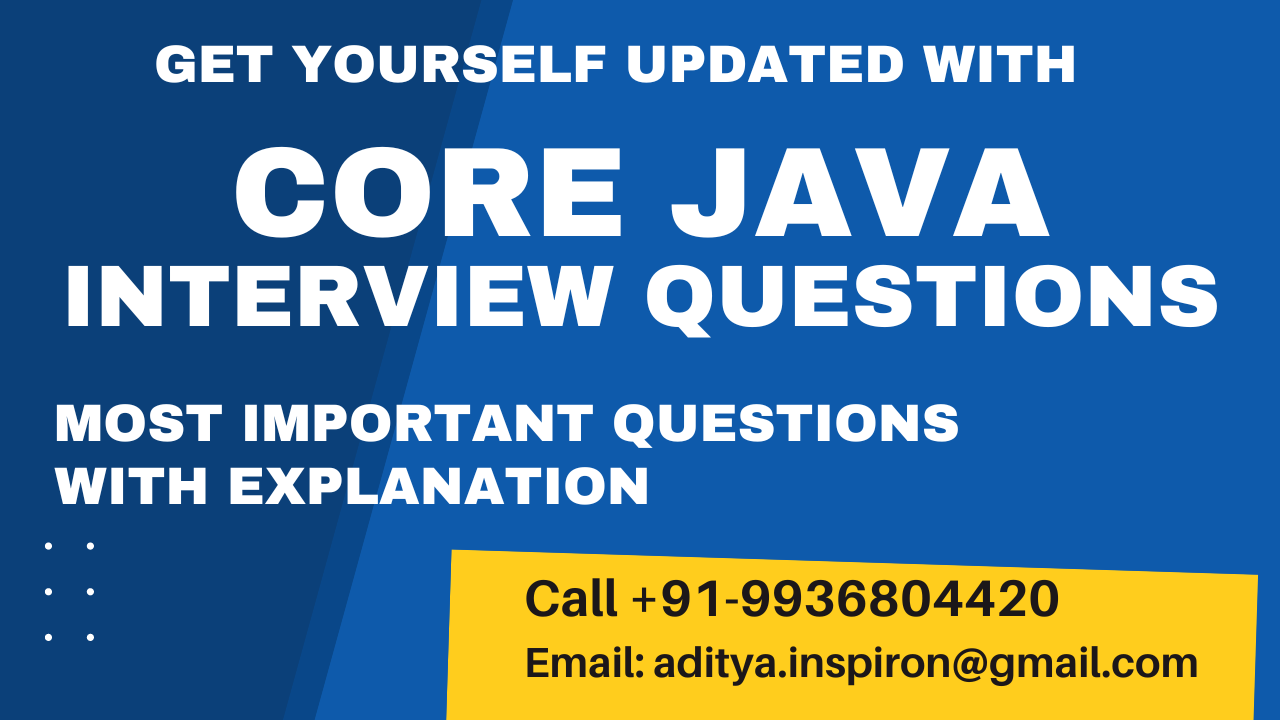
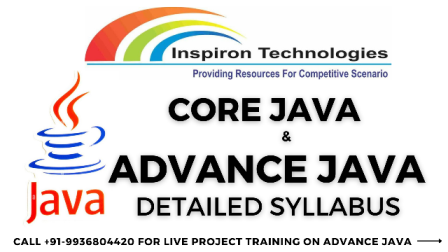
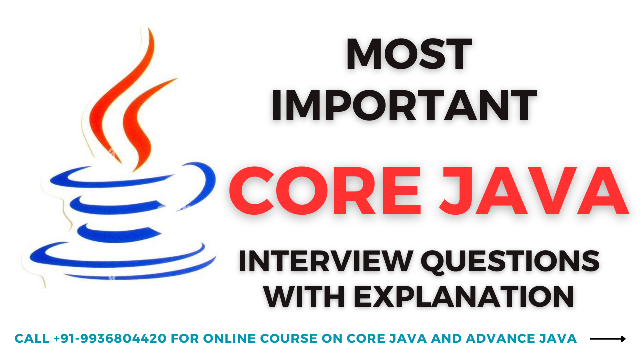
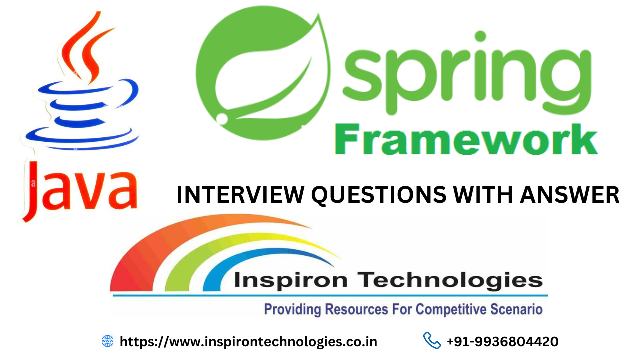
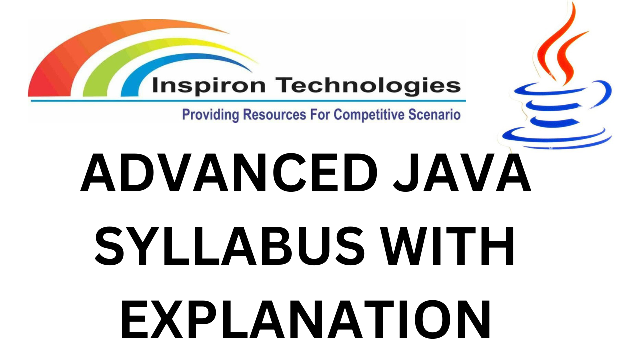
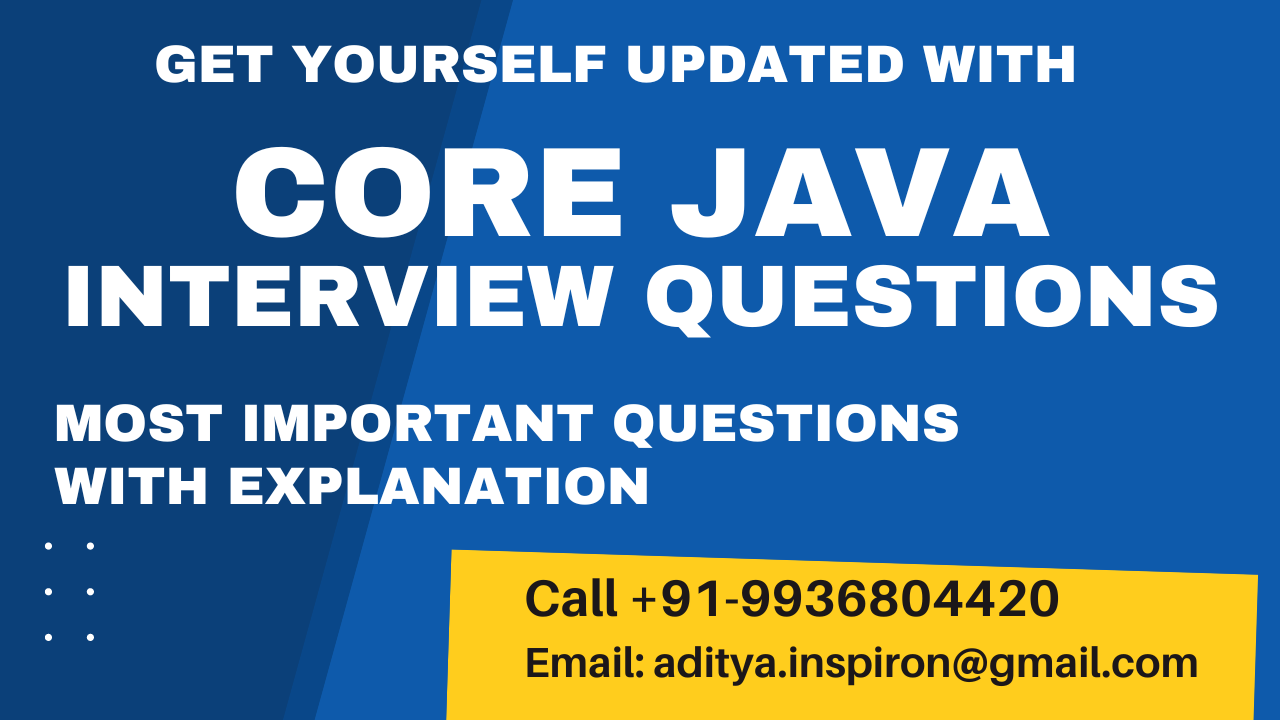
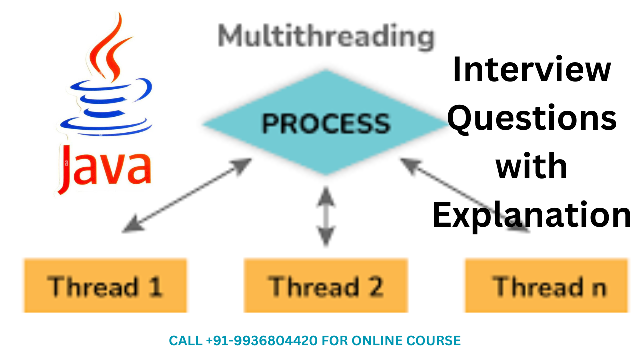
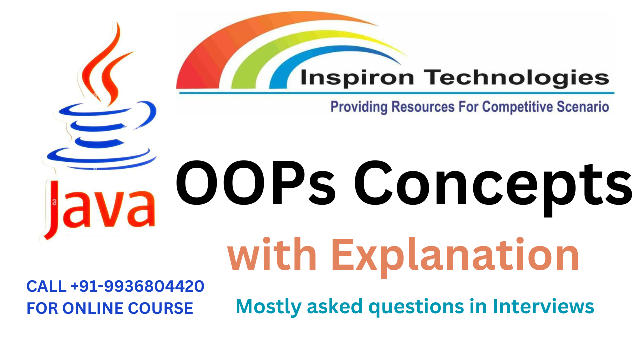
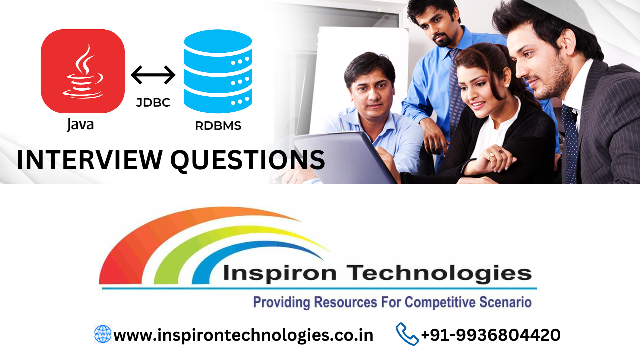
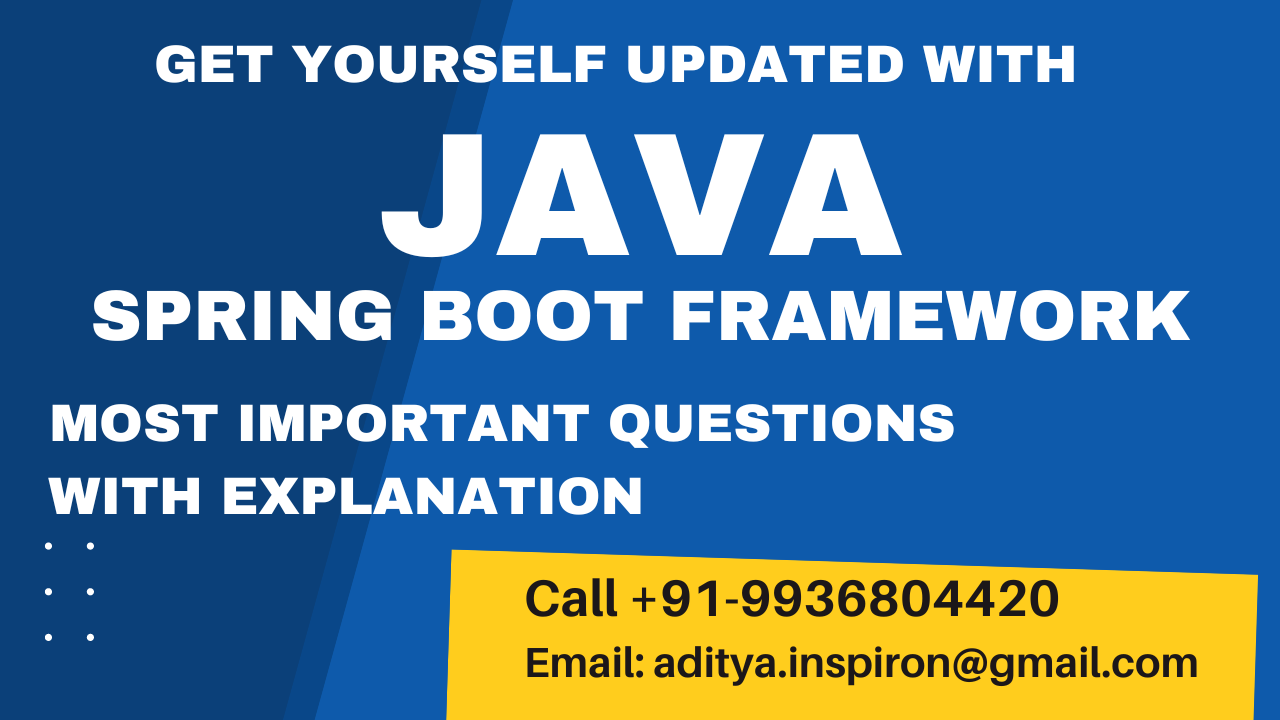
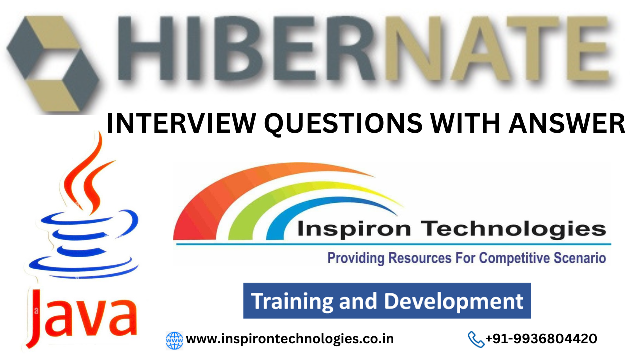
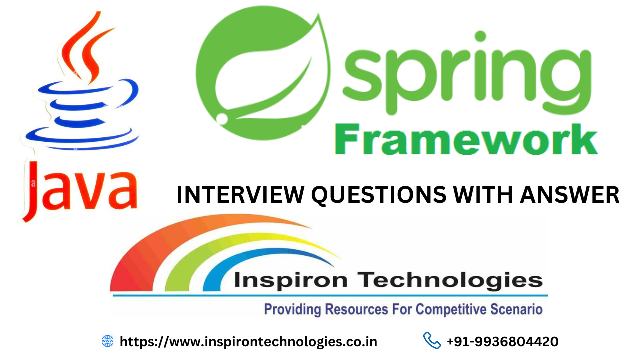
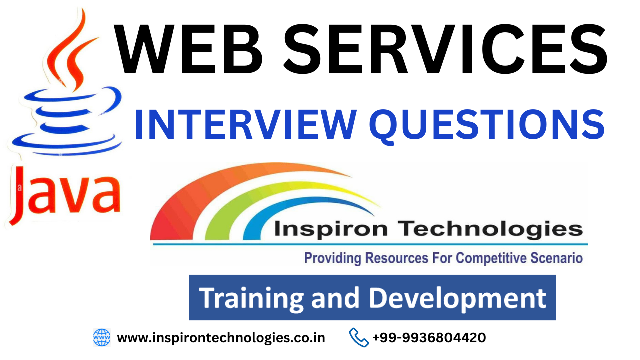
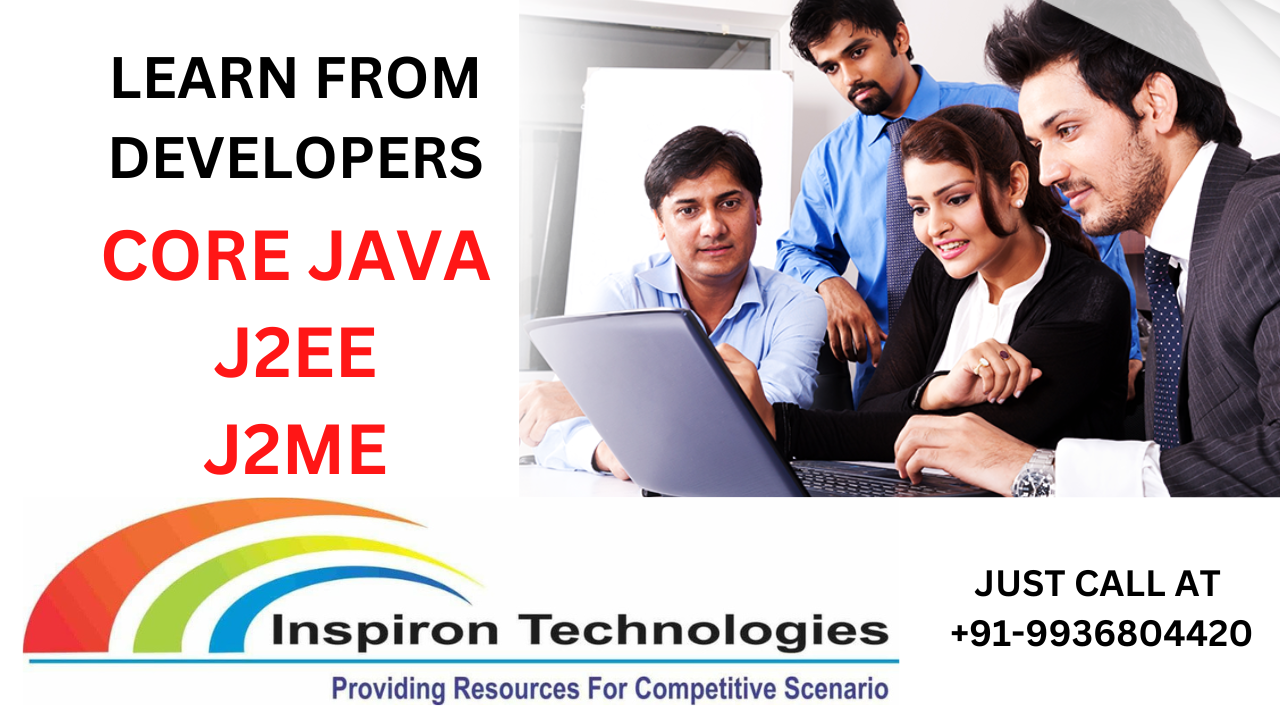
Leave a comment