JDBC Interview Questions
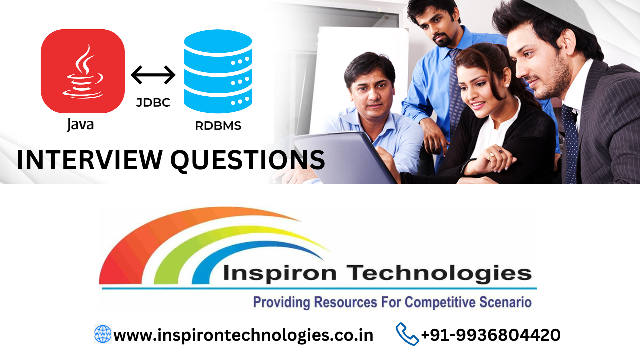
1. What is JDBC, and why is it used in Java?
Answer: JDBC stands for Java Database Connectivity. It is a Java-based API that allows Java applications to interact with databases. JDBC is used to connect and manipulate data in relational databases using Java code.
2. What are the different types of JDBC drivers, and how do they differ from each other?
Answer: There are four types of JDBC drivers:
Type-1 Driver (JDBC-ODBC Bridge Driver): This driver uses the ODBC (Open Database Connectivity) API to connect to the database. It is platform-dependent and requires ODBC to be installed. It is generally not recommended for production use.
Type-2 Driver (Native-API Driver): This driver uses a database-specific native library to connect to the database. It provides better performance than the Type-1 driver but is still platform-dependent.
Type-3 Driver (Network Protocol Driver): This driver translates JDBC calls into a database-independent network protocol, which is then translated to the database-specific protocol by a server. It is platform-independent but may not be as efficient as Type-2 drivers.
Type-4 Driver (Thin Driver or Direct-to-Database Driver): This driver communicates directly with the database server using the database's native protocol. It is platform-independent, high-performance, and the most commonly used driver for production applications.
3. How do you establish a database connection in Java using JDBC?
Answer: To establish a database connection in Java using JDBC, you need to follow these steps:
Load the database driver using Class.forName() (for Type-4 drivers, this step is often optional).
Create a connection to the database using DriverManager.getConnection().
Create a statement or prepared statement for executing SQL queries.
Execute SQL queries, retrieve results, and handle exceptions as needed.
Close the connection, statement, and result sets when done to release resources.
4. What is the difference between a Statement, PreparedStatement, and CallableStatement in JDBC?
Answer:
Statement: It is used for executing simple SQL queries without any parameters. It is not precompiled, which may lead to performance issues and security risks (SQL injection).
PreparedStatement: It is used for executing parameterized SQL queries. The SQL statement is precompiled, improving performance and security by preventing SQL injection.
CallableStatement: It is used for executing database stored procedures. It can also return output parameters, making it suitable for complex database operations.
5. What is SQL injection, and how can you prevent it when using JDBC?
Answer: SQL injection is a security vulnerability that occurs when untrusted data is directly included in SQL queries. To prevent SQL injection when using JDBC, you should use PreparedStatement or CallableStatement instead of Statement. These classes automatically handle escaping and quoting of parameters, making it much more difficult for attackers to inject malicious SQL code.
6. How do you handle exceptions in JDBC?
Answer: Exception handling is essential in JDBC. You should use try-catch blocks to handle exceptions that can occur during database operations. Common exceptions in JDBC include SQLException, ClassNotFoundException, and DataTruncation, among others. Proper exception handling ensures that your application gracefully handles errors and releases resources.
7. What is connection pooling, and why is it important in JDBC applications?
Answer: Connection pooling is a technique used to manage a pool of database connections that can be reused by multiple clients. It is important in JDBC applications to improve performance and reduce the overhead of opening and closing database connections. Connection pooling frameworks like Apache DBCP and HikariCP are commonly used to manage connection pools.
8. How can you retrieve data from a ResultSet in JDBC?
Answer: You can retrieve data from a ResultSet in JDBC by using methods like getString(), getInt(), getDouble(), etc., based on the data type of the columns in the result set. You can move through the result set using methods like next(). Here's an example:
ResultSet resultSet = statement.executeQuery("SELECT name, age FROM employees");
while (resultSet.next()) {
String name = resultSet.getString("name");
int age = resultSet.getInt("age");
// Process data here
}
These are some common JDBC interview questions and answers. Depending on the job position and the level of expertise required, you may encounter more advanced and specific questions as well.
9. What is the difference between ResultSet.TYPE_FORWARD_ONLY, ResultSet.TYPE_SCROLL_INSENSITIVE, and ResultSet.TYPE_SCROLL_SENSITIVE in JDBC?
Answer: These are different types of result set cursors in JDBC:
ResultSet.TYPE_FORWARD_ONLY: This type of result set allows you to move only forward through the records, and it is not sensitive to changes made by other clients. It is the most efficient but least flexible cursor type.
ResultSet.TYPE_SCROLL_INSENSITIVE: This result set allows bidirectional movement through records, and it is insensitive to changes made by other clients. It provides more flexibility but may be less efficient.
ResultSet.TYPE_SCROLL_SENSITIVE: Similar to ResultSet.TYPE_SCROLL_INSENSITIVE, it allows bidirectional movement through records, but it is sensitive to changes made by other clients. This type is the most flexible but may have some performance overhead.
10. What is the purpose of the ResultSetMetaData class in JDBC?
Answer: The ResultSetMetaData class is used to retrieve metadata about the columns in a ResultSet. It provides information about the number of columns, the column names, the data types, and other properties of the result set. This metadata is useful when dynamically processing query results or when building generic database applications.
11. Explain how you can perform batch processing in JDBC, and what are its benefits?
Answer: Batch processing in JDBC allows you to execute multiple SQL statements in a single batch, which can improve performance by reducing the number of round-trips to the database. Here's how you can perform batch processing:
Create a Statement or PreparedStatement.
Add multiple SQL statements using the addBatch() method.
Execute the batch using executeBatch().
Benefits of batch processing include reduced network overhead, faster execution of multiple statements, and better performance for bulk operations like data inserts or updates.
12. What is the purpose of the java.sql.Connection interface in JDBC?
Answer: The java.sql.Connection interface in JDBC is used to establish and manage a connection to a database. It provides methods to create statements, commit and roll back transactions, manage savepoints, and control various properties of the database connection.
13. What is the difference between a ResultSet and an UpdateCount in the execute method of a Statement or PreparedStatement in JDBC?
Answer: In JDBC, the execute method of a Statement or PreparedStatement can return either a ResultSet or an UpdateCount, depending on the type of SQL statement executed:
ResultSet: When a SELECT statement is executed, it returns a ResultSet containing the query results.
UpdateCount: For SQL statements like INSERT, UPDATE, DELETE, or DDL (Data Definition Language) statements, the execute method returns an UpdateCount, which represents the number of affected rows.
14. How can you handle transactions in JDBC, and what are the methods provided by the Connection interface for transaction management?
Answer: You can handle transactions in JDBC using the following methods provided by the Connection interface:
setAutoCommit(boolean autoCommit): This method allows you to enable or disable auto-commit mode. When auto-commit is disabled, you can manually commit or roll back transactions.
commit(): This method is used to commit the current transaction. All changes made during the transaction become permanent.
rollback(): This method is used to roll back the current transaction, undoing all changes made during the transaction.
By using these methods, you can control the transaction behavior in JDBC applications.
These additional questions cover more advanced topics related to JDBC. Remember to prepare thoroughly for your interview by understanding the core concepts and practical implementation of JDBC in Java.
15. What is connection pooling, and why is it important in JDBC applications?
Answer: Connection pooling is a technique used to manage a pool of database connections that can be reused by multiple clients. It is important in JDBC applications for several reasons:
Connection overhead: Establishing a new database connection is a relatively expensive operation in terms of time and resources. Connection pooling minimizes this overhead by reusing existing connections.
Resource management: Connection pooling frameworks help manage the number of concurrent connections to the database, preventing resource exhaustion and improving application scalability.
Improved performance: By reusing connections, the application can execute database operations more efficiently, resulting in better performance.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Networking Concepts, Machine Learning, Angular JS, Node JS, Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
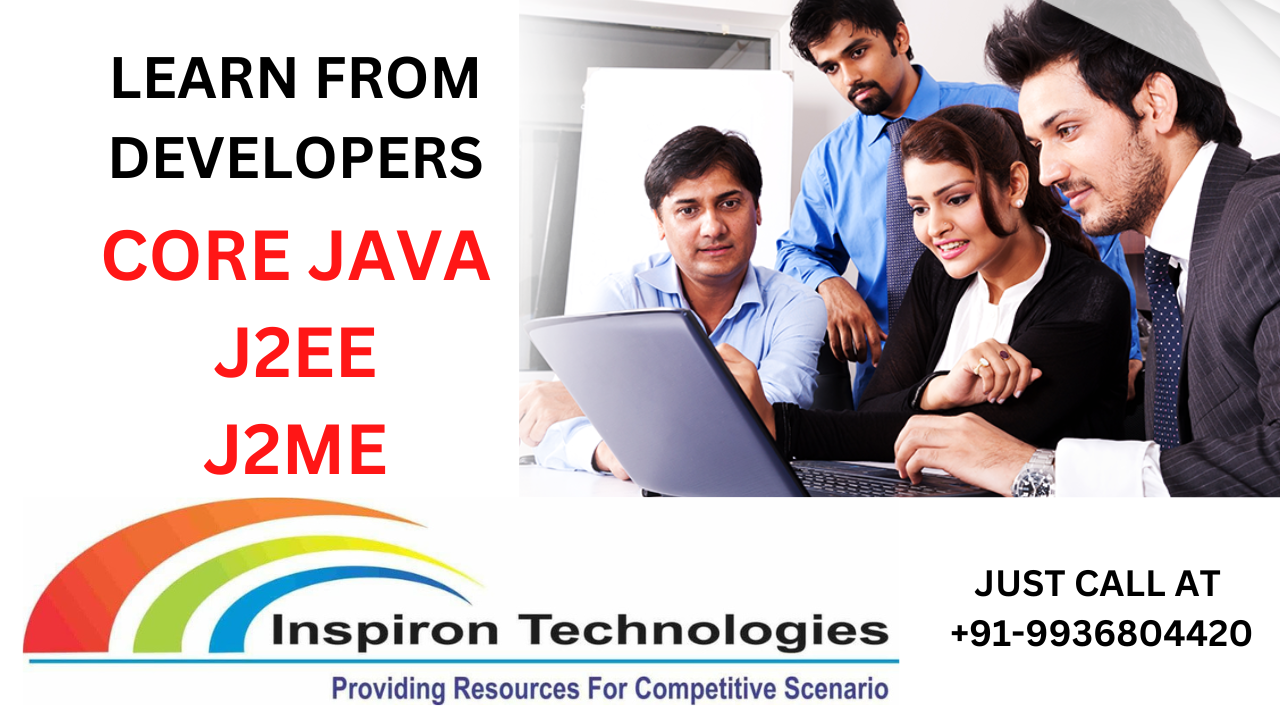
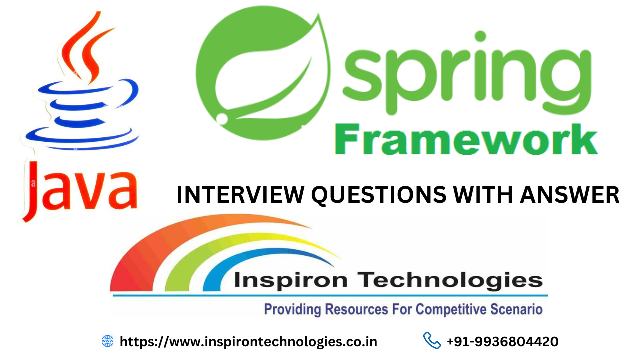
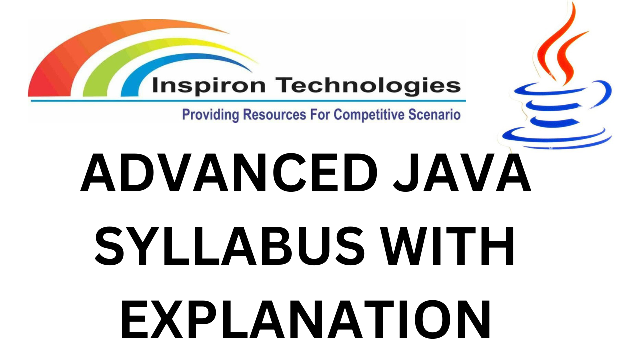
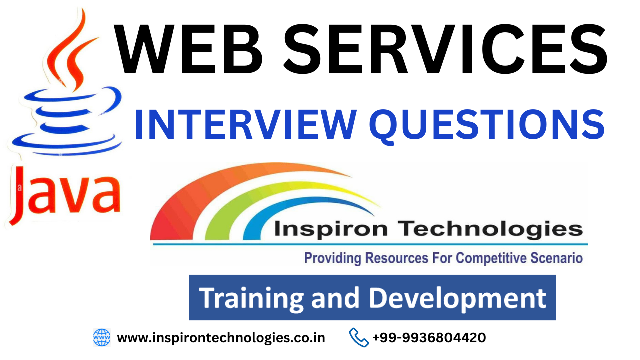
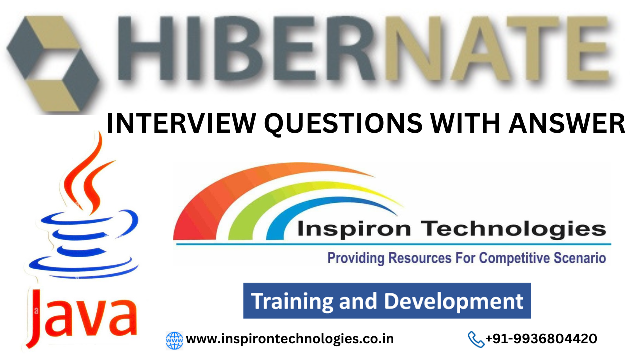
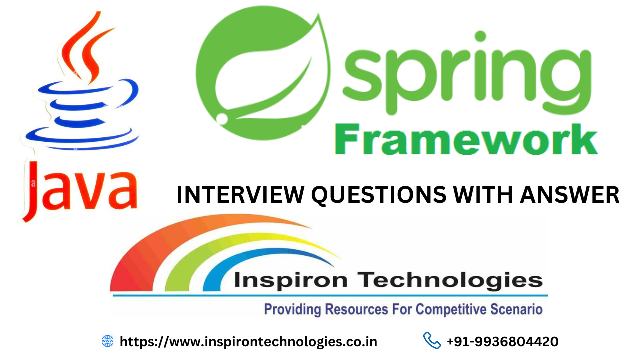
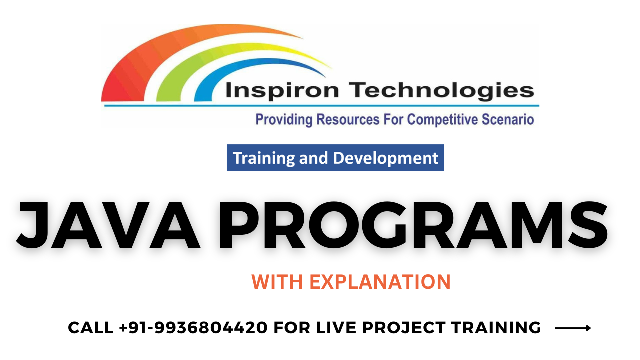
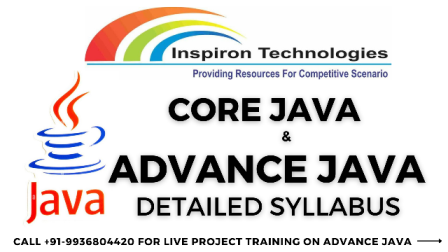
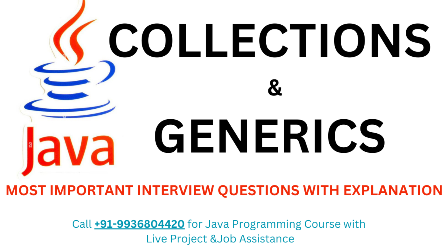
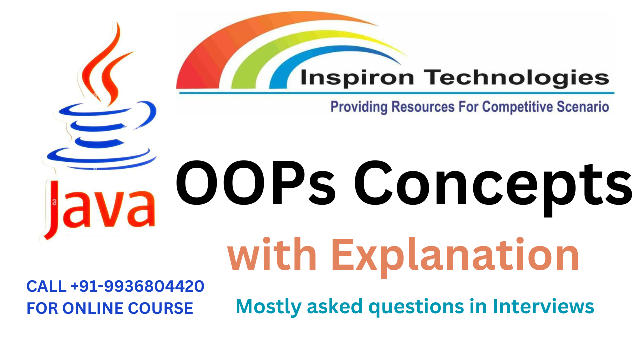
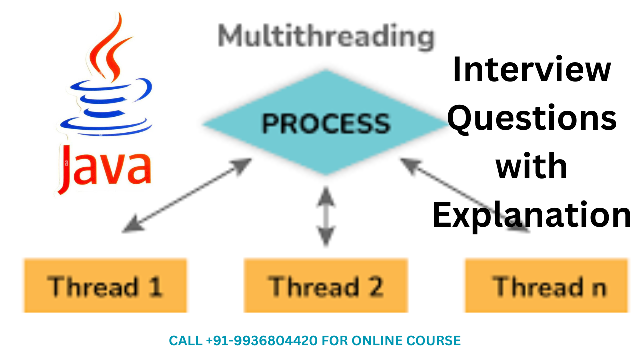
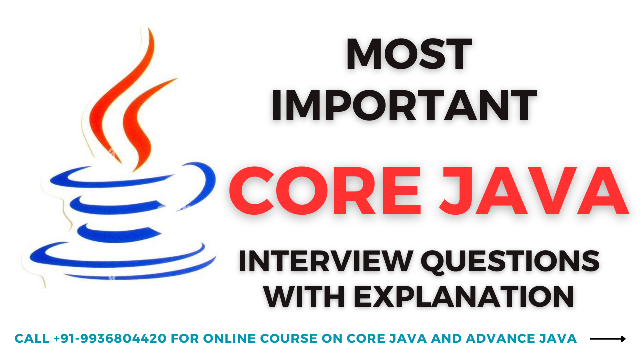
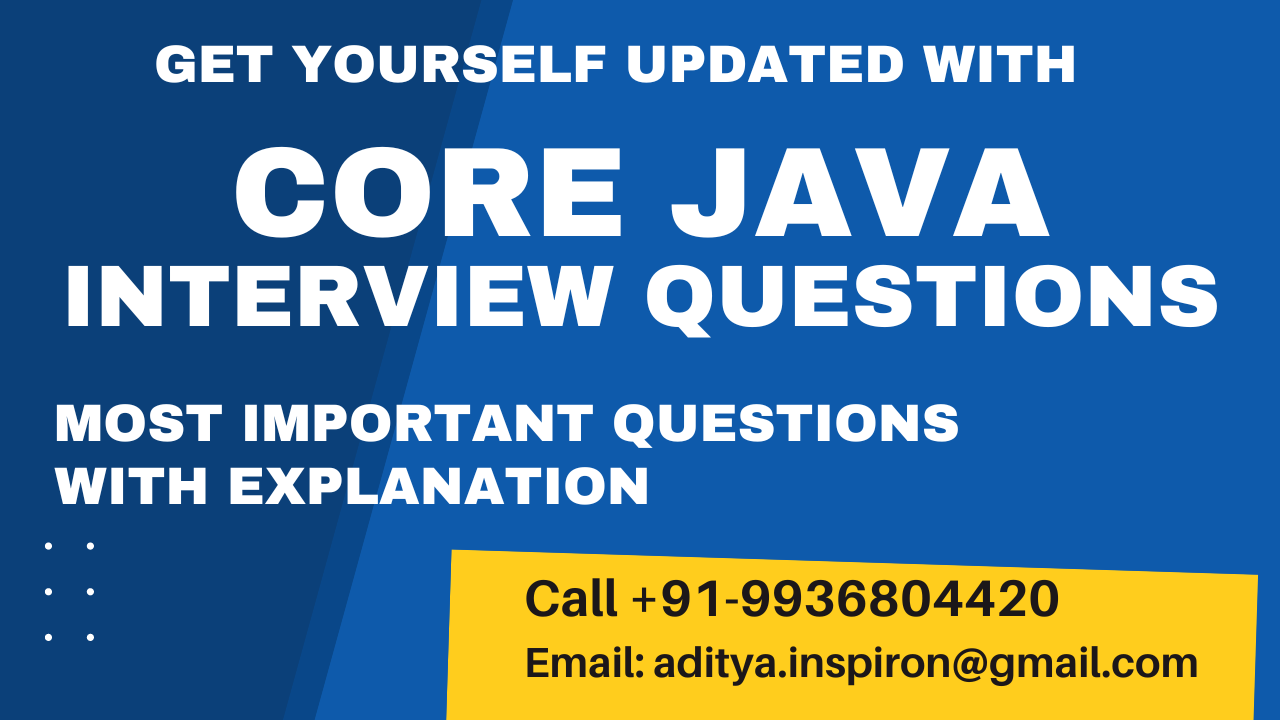
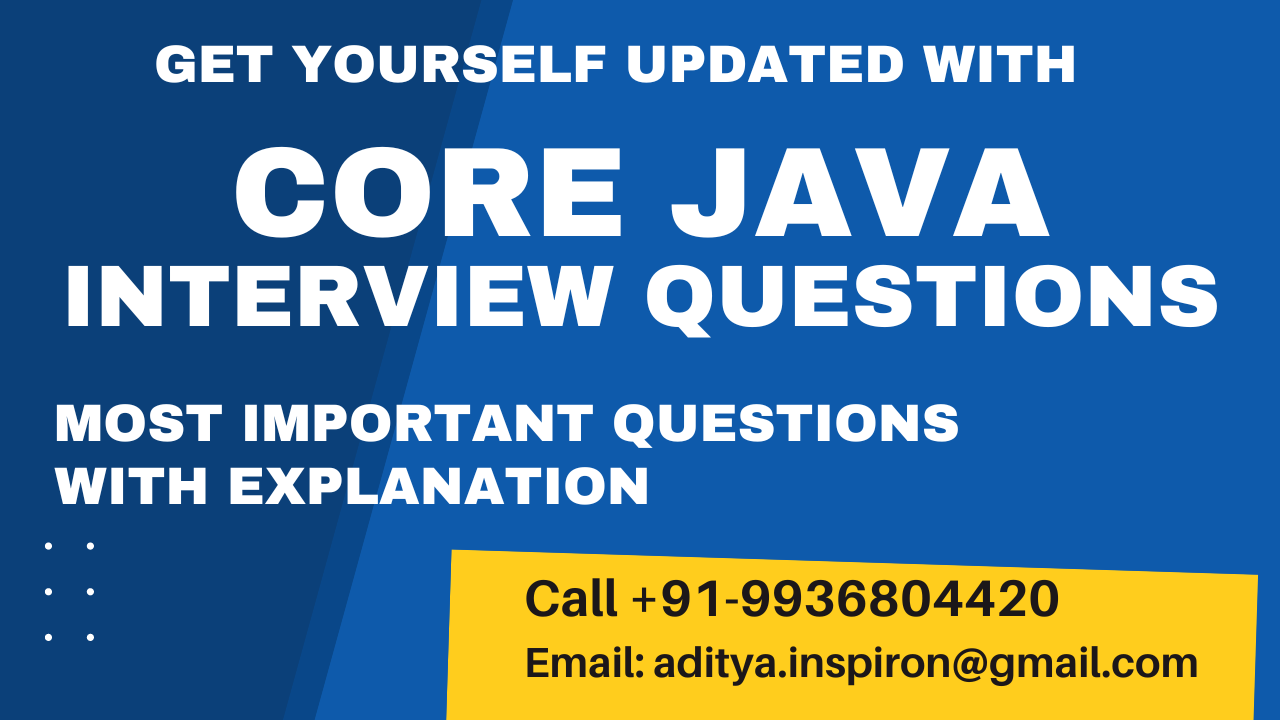
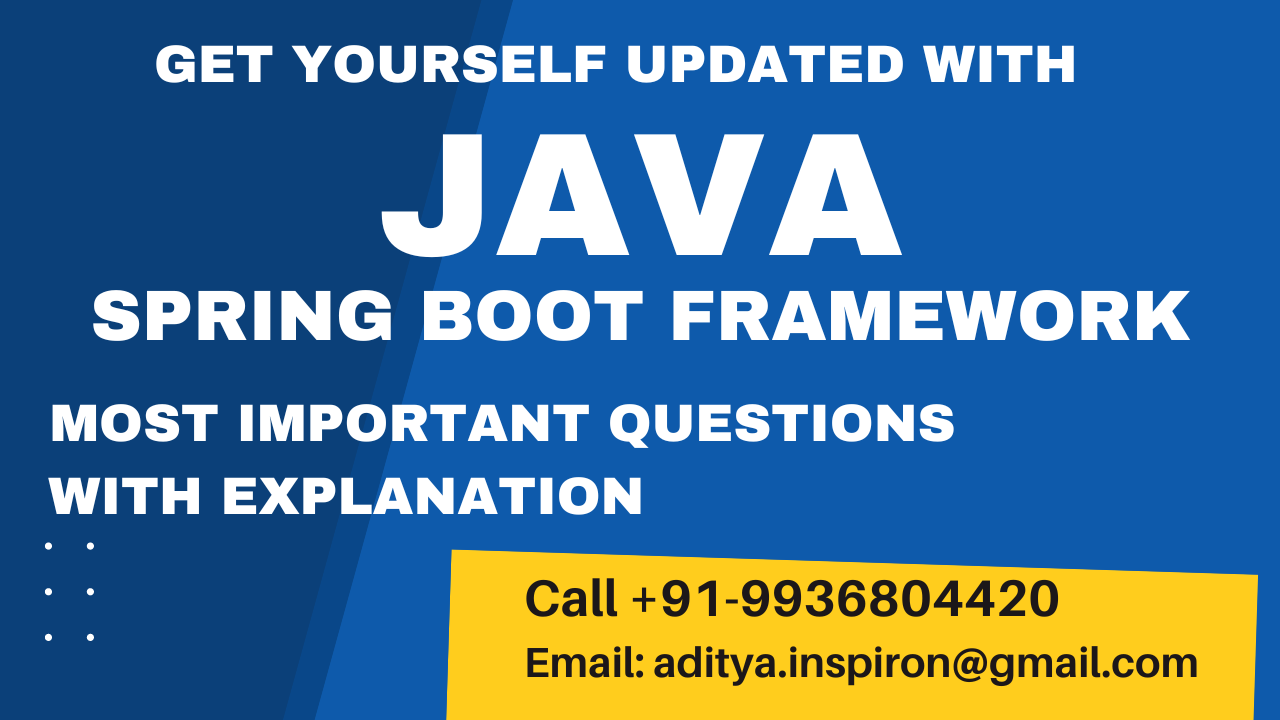
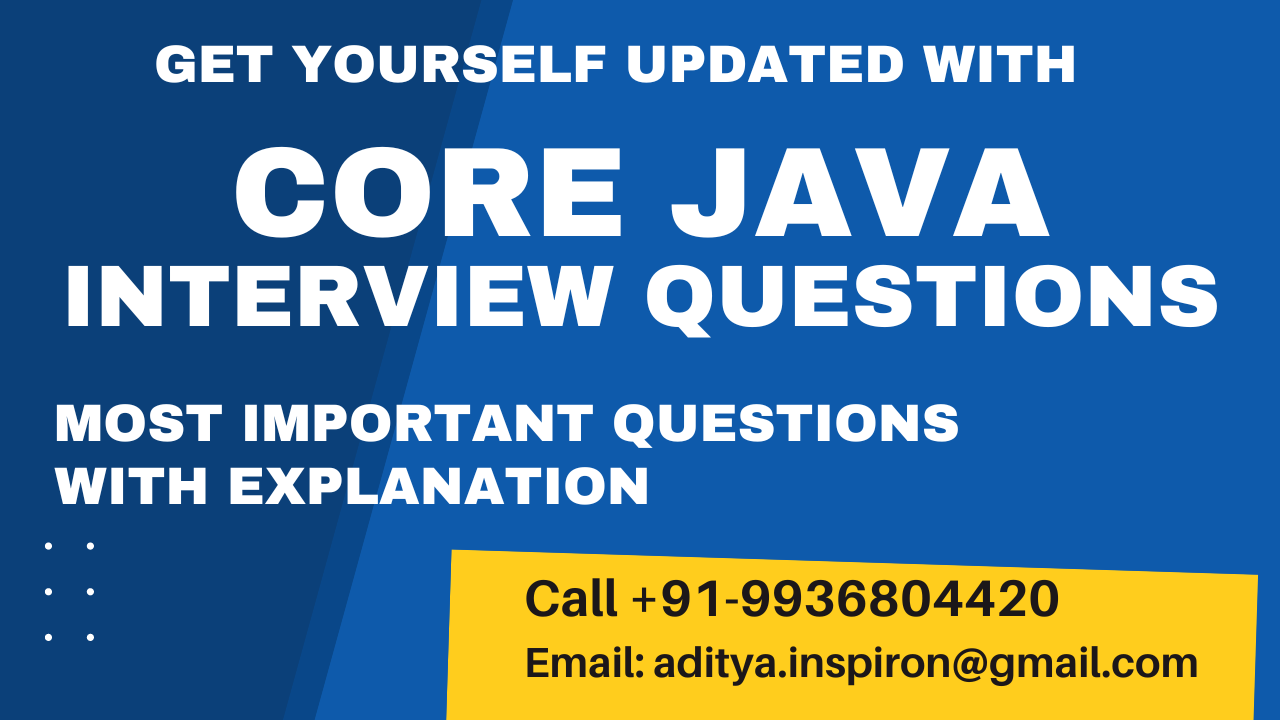
Categories
Popular Post
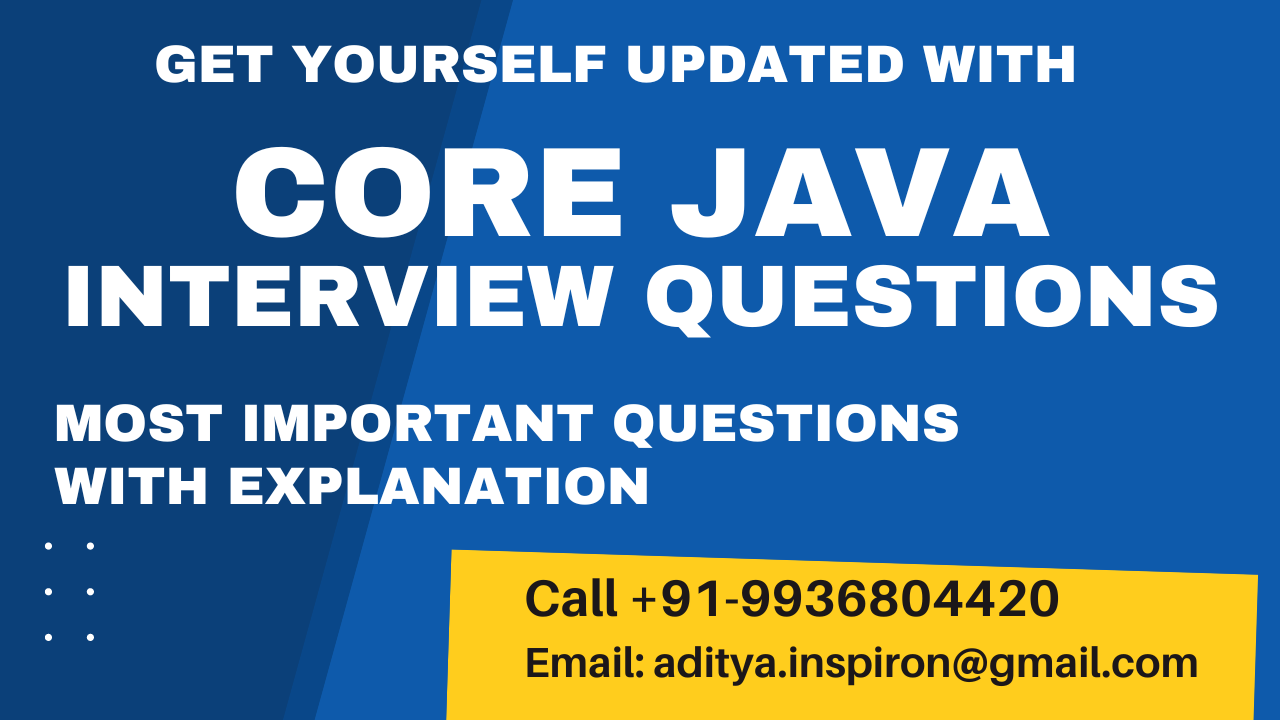
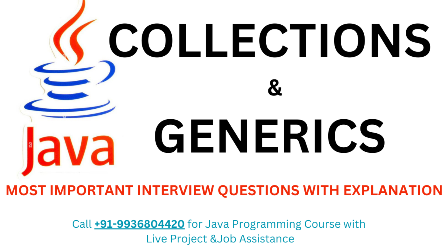
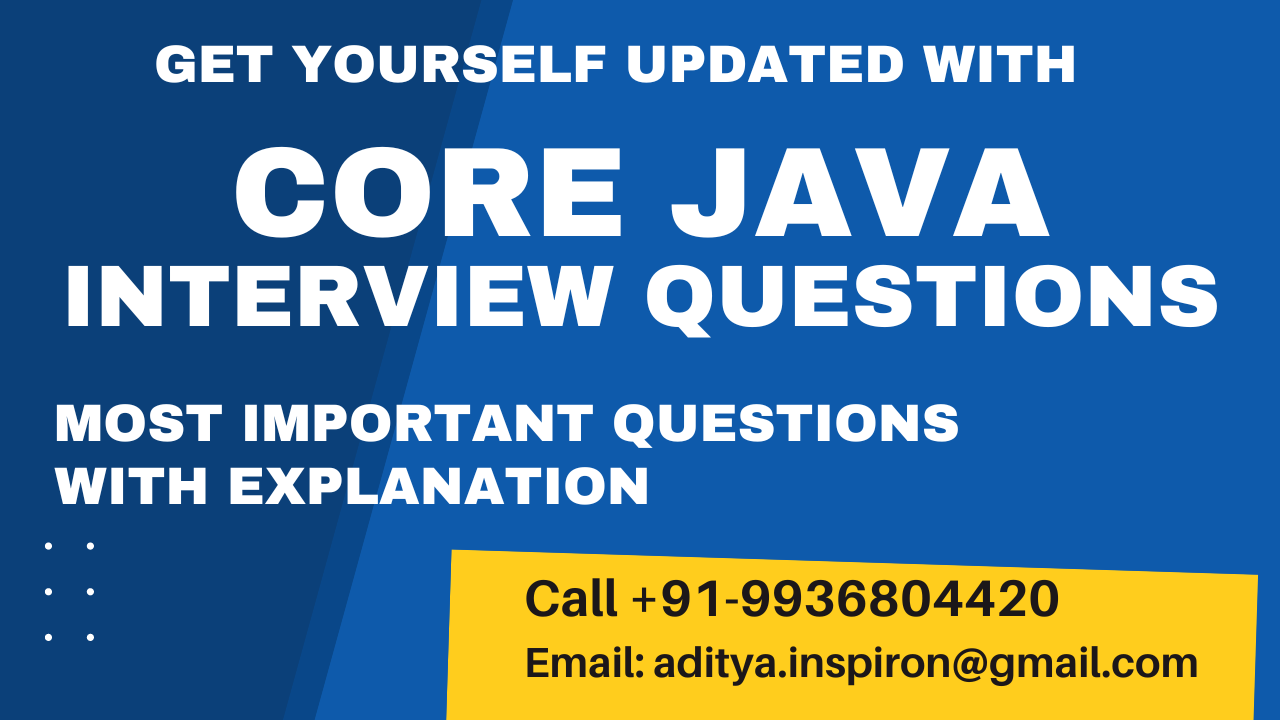
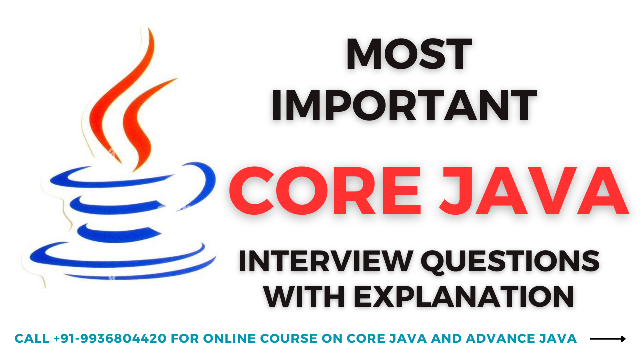
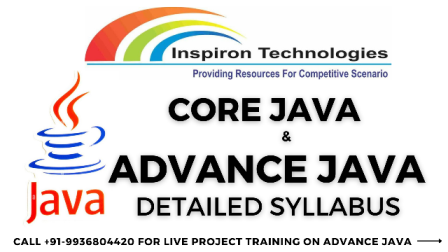
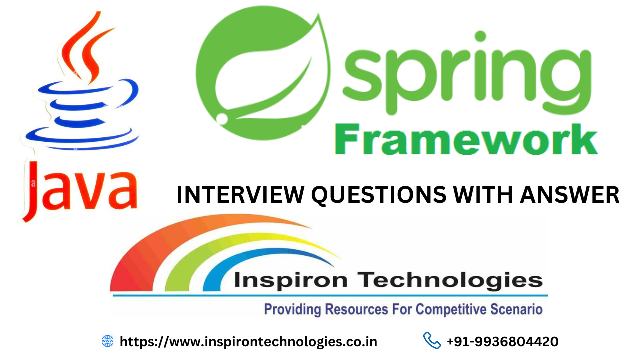
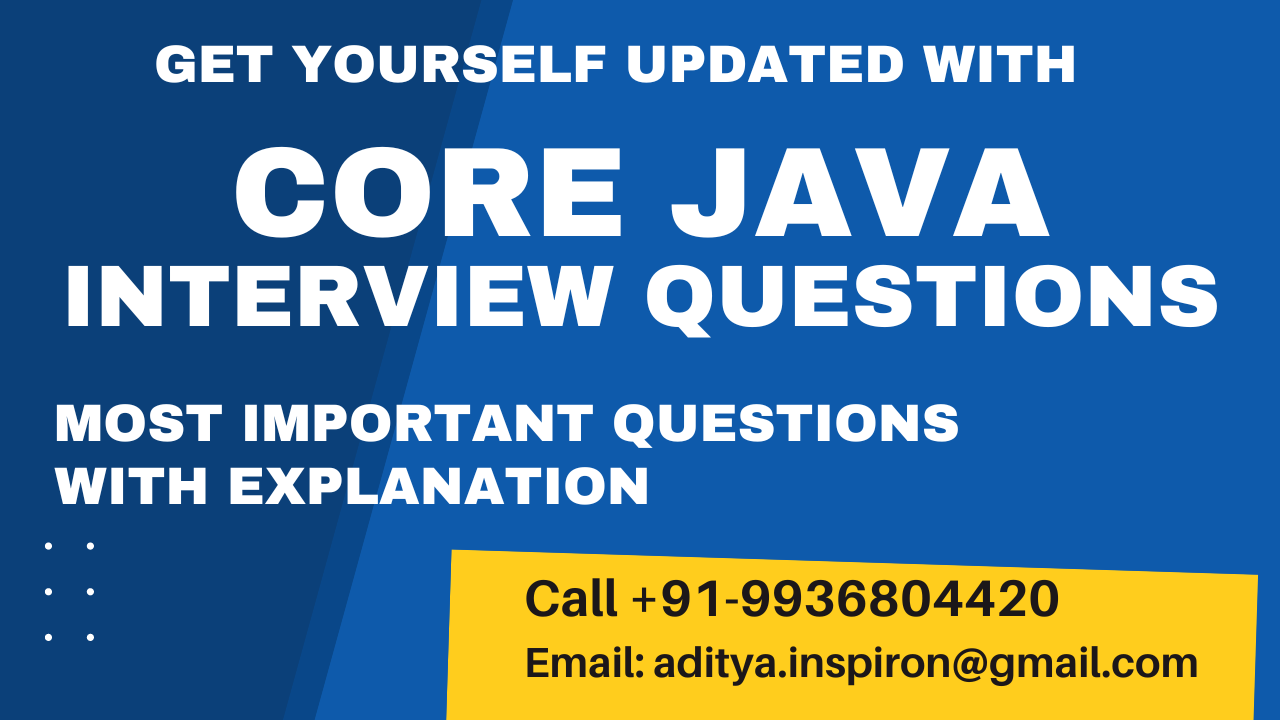
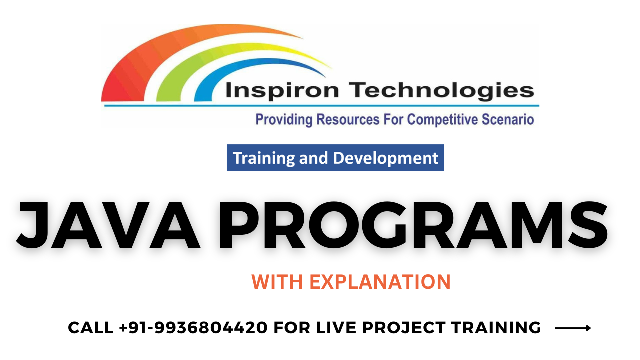
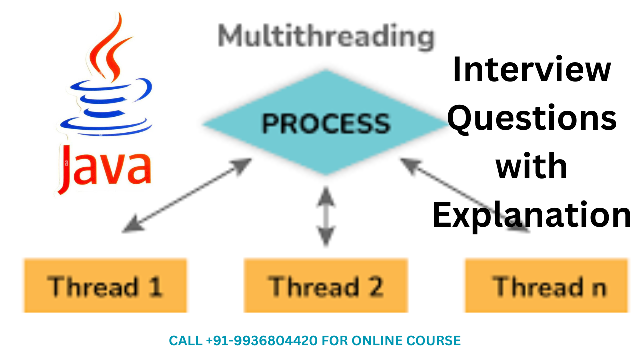
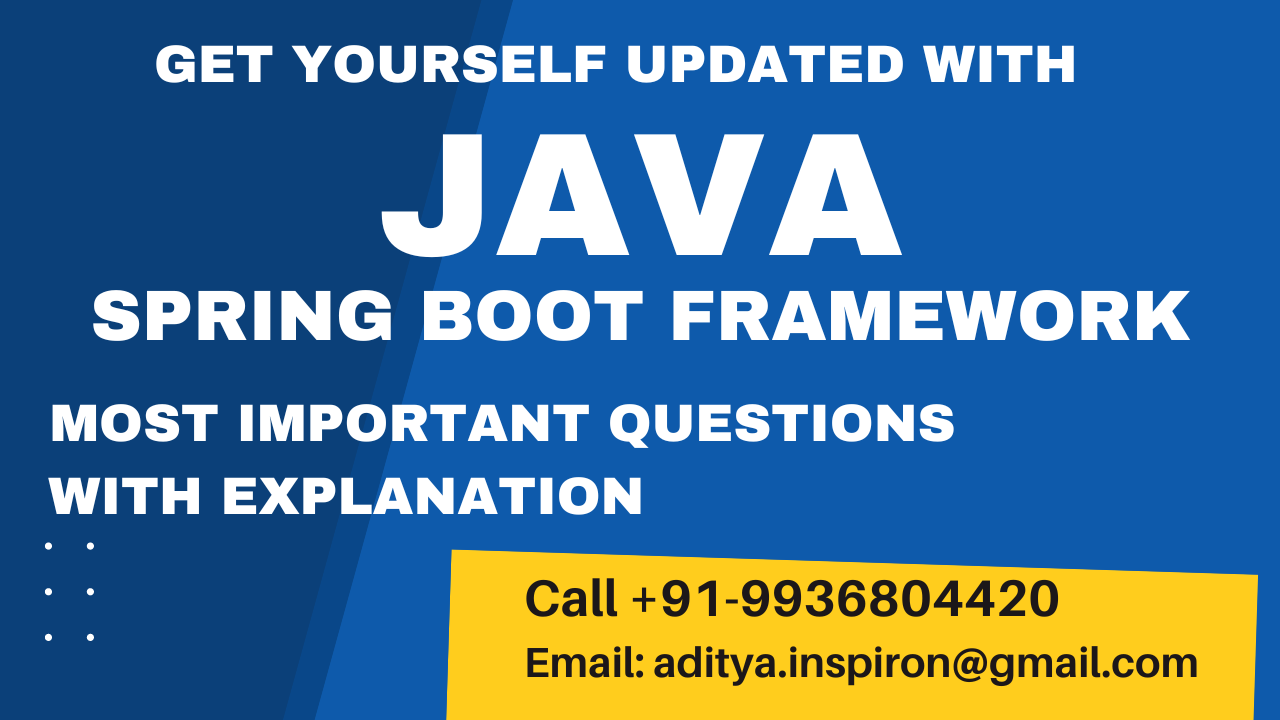
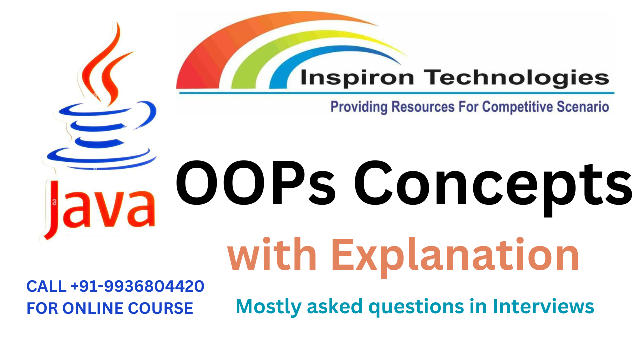
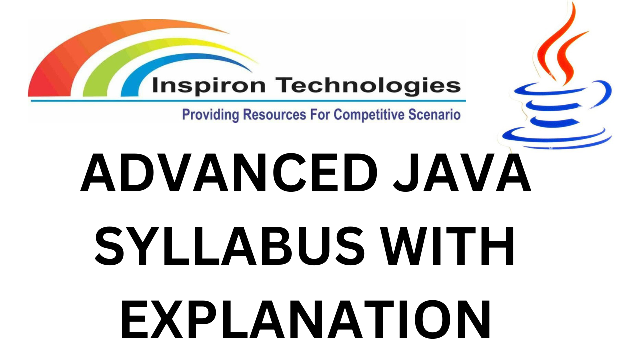
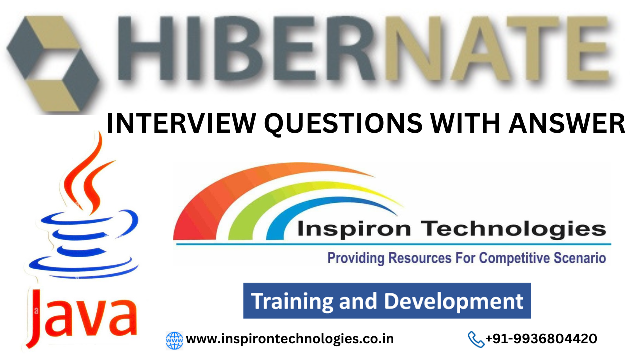
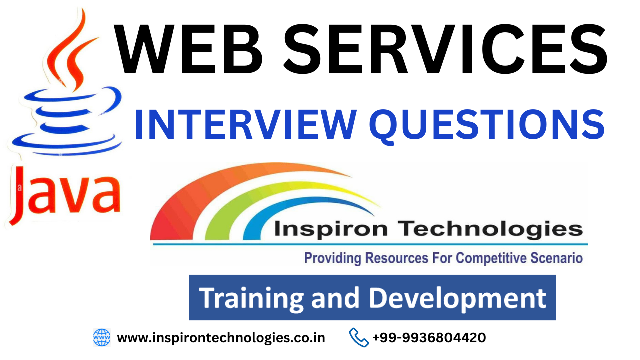
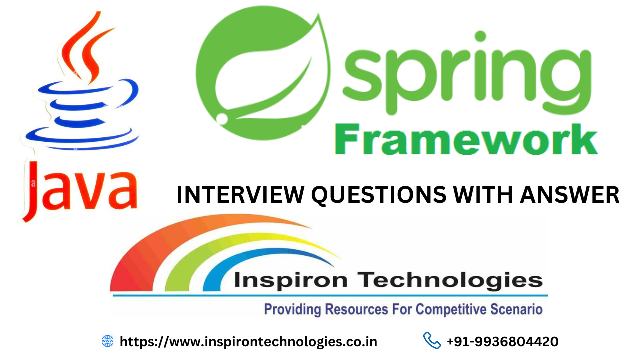
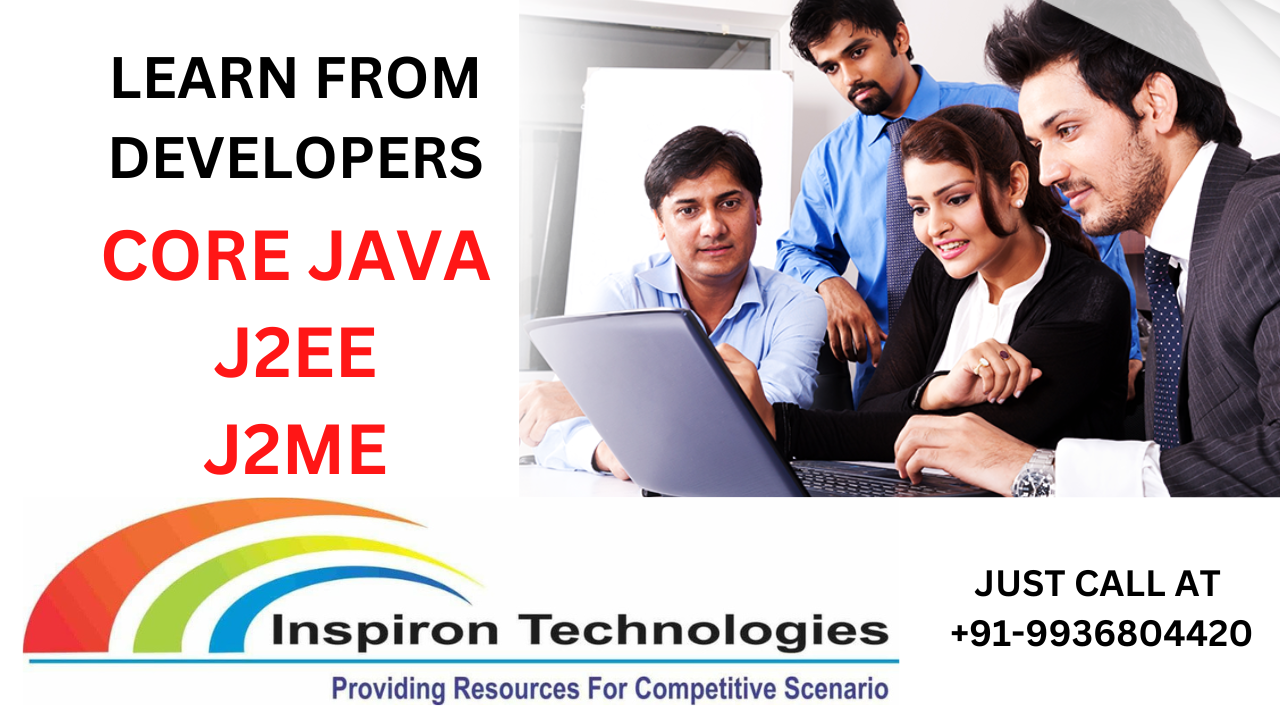
Leave a comment