Java Web Service Interview Questions
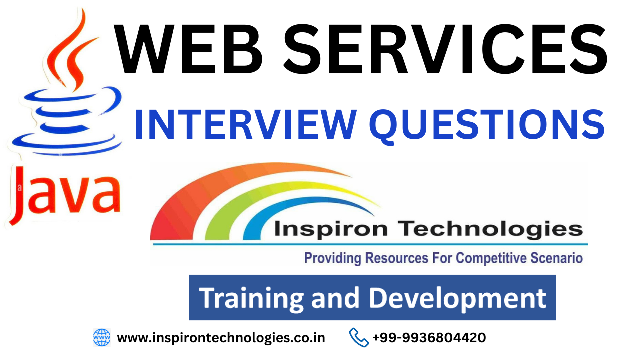
Java web services are a set of technologies and APIs that enable the development of web-based applications and services using the Java programming language. These services allow different software applications to communicate and interact with each other over the internet or a network. Here's a brief overview:
1. Java API for XML Web Services (JAX-WS): JAX-WS is a standard Java API for creating and consuming web services using XML. It allows you to build both SOAP (Simple Object Access Protocol) and RESTful web services.
2. SOAP Web Services: SOAP is a protocol for exchanging structured information in the implementation of web services. Java provides libraries and tools for creating, deploying, and consuming SOAP-based web services.
3. RESTful Web Services: REST (Representational State Transfer) is an architectural style for designing networked applications. Java provides frameworks like JAX-RS (Java API for RESTful Web Services) to build RESTful web services.
4. Web Service Endpoints: Java web services typically have endpoints, which are methods or URLs that clients can access to request specific functionality. These endpoints can be implemented using annotations and configured in deployment descriptors.
5. Data Serialization: Java web services often use XML or JSON for data serialization to ensure that data can be easily exchanged between different platforms and programming languages.
6. Security: Security is a crucial aspect of web services. Java provides various security mechanisms to protect data and ensure secure communication, including SSL/TLS, authentication, and authorization.
7. Deployment: Java web services can be deployed in various environments, including Java EE application servers, servlet containers, and cloud platforms. They can be hosted as standalone applications or integrated with existing systems.
8. Tooling: There are several tools available for developing, testing, and deploying Java web services, such as Apache CXF, Jersey, and Spring Boot.
Java web services are widely used for building distributed applications, microservices, and integrating diverse systems. They provide a robust and platform-independent way to create and consume web services, making them a fundamental technology for modern web-based applications.
1. What is a web service, and what are the types of web services in Java?
Answer: A web service is a software component designed to communicate over the internet or a network. In Java, there are two main types of web services:
SOAP Web Services: These use the Simple Object Access Protocol (SOAP) for communication and are based on XML. They provide a platform-independent way to exchange structured information.
RESTful Web Services: These follow the principles of Representational State Transfer (REST) and use standard HTTP methods (GET, POST, PUT, DELETE) for data exchange. They are typically more lightweight and widely used for web applications.
2. What is JAX-WS, and how does it relate to SOAP web services in Java?
Answer: JAX-WS stands for Java API for XML Web Services. It is a Java standard for building and consuming SOAP-based web services. JAX-WS provides annotations and APIs to create and work with web services. It simplifies the process of developing SOAP services in Java.
3. What is the difference between a SOAP message and a RESTful web service request?
Answer: SOAP messages are typically XML-based and include a standardized envelope structure with a header and body. In contrast, RESTful web service requests are simple HTTP requests with specific HTTP methods (GET, POST, PUT, DELETE) and use URL parameters and headers for data exchange. SOAP is more rigid and requires a predefined XML structure, while REST is more flexible and relies on the underlying HTTP protocol.
4. How do you create a RESTful web service in Java using JAX-RS?
Answer: To create a RESTful web service in Java using JAX-RS, follow these steps:
Define a resource class with methods annotated with JAX-RS annotations such as @Path, @GET, @POST, @PUT, and @DELETE to specify the URIs and HTTP methods.
Deploy the resource class in a Java EE application server or a servlet container.
Access the web service by making HTTP requests to the defined URIs.
5. What is the role of the @Path annotation in JAX-RS?
Answer: The @Path annotation in JAX-RS is used to define the base URI path for a resource class or a specific resource method. It specifies the part of the URI that is associated with the resource, allowing you to create hierarchical URIs for your RESTful web services.
6. How does JAX-RS support content negotiation in RESTful web services?
Answer: JAX-RS supports content negotiation through the @Produces and @Consumes annotations. The @Produces annotation specifies the media types that the resource can produce, and the @Consumes annotation specifies the media types it can consume. When a client makes a request, it can include the desired media type in the Accept header for content negotiation.
7. What is the purpose of the @PathParam annotation in JAX-RS?
Answer: The @PathParam annotation in JAX-RS is used to extract values from URI path parameters. It allows you to dynamically capture values from the URI and use them as method parameters in your resource class.
8. What is the role of the WSDL (Web Services Description Language) in SOAP web services?
Answer: WSDL is an XML-based language used to describe the structure of a SOAP web service. It defines the service's operations, input and output parameters, and communication details. WSDL is used to generate client stubs for consuming SOAP services and to provide a contract between service providers and consumers.
9. How do you secure a web service in Java, and what are some common security mechanisms?
Answer: Web services can be secured in Java using mechanisms like:
HTTPS/SSL for transport layer security: This encrypts data during transmission.
Authentication and authorization: Implementing username/password or token-based authentication and authorization mechanisms.
WS-Security for SOAP: It provides message-level security for SOAP web services.
OAuth and JWT for RESTful services: These are widely used for securing RESTful APIs.
10. What is the purpose of a RESTful API client, and how can you create one in Java?
Answer: A RESTful API client is used to consume and interact with RESTful web services. You can create a RESTful API client in Java using libraries like Apache HttpClient or the built-in HttpURLConnection class to make HTTP requests and handle responses, including JSON or XML parsing.
These Java web services interview questions cover essential concepts related to SOAP and RESTful web services, JAX-RS, content negotiation, security, and API clients. Make sure to understand these topics to excel in your Java web services interview.
11. What is the role of the @Produces annotation in JAX-RS, and how does it impact the response from a RESTful web service method?
Answer: The @Produces annotation in JAX-RS is used to specify the media types that a RESTful web service method can produce. It influences the HTTP Content-Type header of the response. For example, if a method is annotated with @Produces("application/json"), the response will have a Content-Type of application/json.
12. How can you handle errors and exceptions in a RESTful web service in Java?
Answer: In a RESTful web service, you can handle errors and exceptions by:
Using appropriate HTTP status codes in responses (e.g., 404 for resource not found, 500 for internal server error).
Providing error details in the response body, often in JSON or XML format.
Implementing exception handling mechanisms in the code to catch and handle exceptions gracefully.
13. What is the role of the @Consumes annotation in JAX-RS, and how does it impact the request to a RESTful web service method?
Answer: The @Consumes annotation in JAX-RS is used to specify the media types that a RESTful web service method can consume as request data. It influences the HTTP Content-Type header of the incoming request. For example, if a method is annotated with @Consumes("application/json"), it can accept JSON data in the request body.
14. Explain the role of the @RequestBody annotation in a Spring MVC-based RESTful web service and how it differs from @RequestParam.
Answer: In a Spring MVC-based RESTful web service, @RequestBody is used to extract the request body and map it to a Java object. It is often used for POST and PUT requests when data is sent in the request body. On the other hand, @RequestParam is used to extract data from the URL query parameters, typically used with GET requests. So, @RequestBody is used for reading the request body, while @RequestParam is used for reading query parameters.
15. What is the purpose of the @Path annotation in JAX-RS and how is it used to define resource URIs?
Answer: The @Path annotation in JAX-RS is used to specify the base URI path for a resource class or method. It allows you to define the part of the URI that is associated with the resource, making it possible to create hierarchical URIs for your RESTful web services. For example, if you annotate a class with @Path("/employees") and a method with @Path("/details"), the URI to access that method might be /employees/details.
16. What are the advantages and disadvantages of using XML and JSON for data exchange in web services?
Answer:
Advantages of using XML:
Extensible and structured data format.
Supports schemas and validation.
Suitable for document-centric data.
Disadvantages of using XML:
Verbosity, resulting in larger payloads.
Slower parsing and serialization.
Complex to work with in some cases.
Advantages of using JSON:
Lightweight and concise data format.
Faster parsing and serialization.
Easy to work with in JavaScript and modern programming languages.
Disadvantages of using JSON:
Less structured compared to XML.
Limited support for schemas and validation.
17. Explain the concept of versioning in RESTful web services, and how can you version your APIs?
Answer: Versioning in RESTful web services involves managing changes to the API over time while ensuring backward compatibility. There are several approaches to versioning, including:
URI versioning: Adding a version number to the URI path (e.g., /api/v1/resource).
Header versioning: Using a custom HTTP header (e.g., Accept-Version: v1) to specify the API version.
Media type versioning: Embedding the version in the media type (e.g., application/vnd.myapi.v1+json).
Content negotiation: Allowing clients to request a specific version using the Accept header.
Versioning helps maintain backward compatibility with existing clients while introducing new features or changes in the API.
18. How does REST differ from SOAP in terms of architectural style and data exchange format?
Answer: REST (Representational State Transfer) is an architectural style that emphasizes a stateless, client-server interaction using standard HTTP methods. It uses lightweight data exchange formats like JSON or XML and is more focused on resources and URIs.
SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in the implementation of web services. It relies on XML for data exchange and has a more rigid and formal structure. SOAP often uses HTTP, but it can use other protocols as well.
19. What is HATEOAS in the context of RESTful web services, and why is it important?
Answer: HATEOAS (Hypertext as the Engine of Application State) is a principle in RESTful web services where a response contains hyperlinks to related resources or actions. It allows clients to navigate the application's state and available actions dynamically. HATEOAS makes RESTful APIs self-descriptive and allows for better discoverability of resources and their relationships, reducing the need for prior knowledge of the API's structure.
20. What are the key components of a SOAP message, and how do they relate to a web service request or response?
Answer: A SOAP message consists of the following components:
Envelope: The root element that contains the entire message.
Header: Contains additional information, such as authentication or routing details.
Body: Contains the actual message data.
Fault: Optional element for reporting errors.
In a web service request, the body contains the request data, and in a response, it contains the response data. The header may contain any additional information required for the request or response. The fault element is used to convey errors if they occur.
These Java web services interview questions and answers cover various aspects of web service development, including REST, SOAP, versioning, data formats, and HATEOAS. Be prepared to discuss these concepts in your interview.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Networking Concepts, Machine Learning, Angular JS, Node JS, Flutter, Cyber Security, Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
People also read
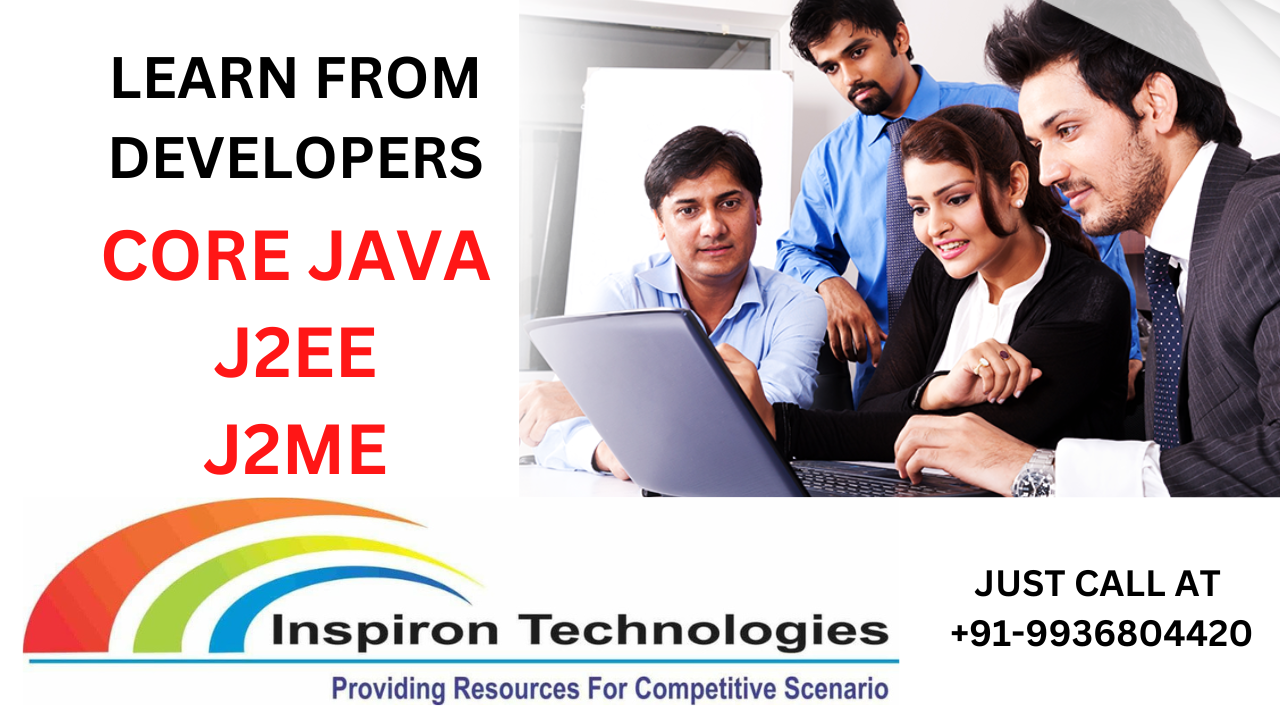
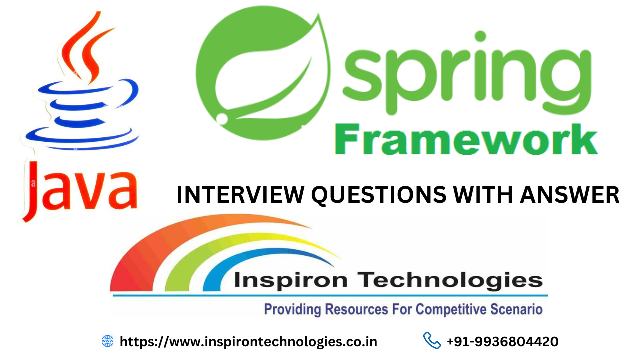
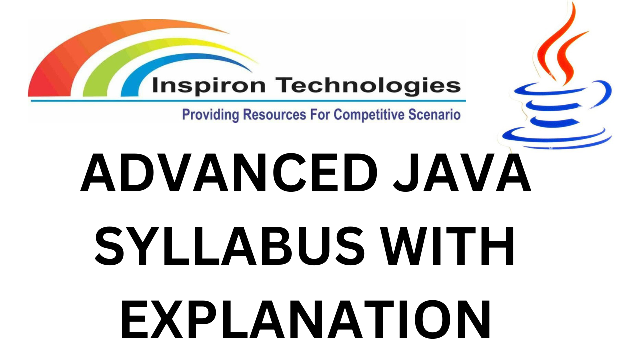
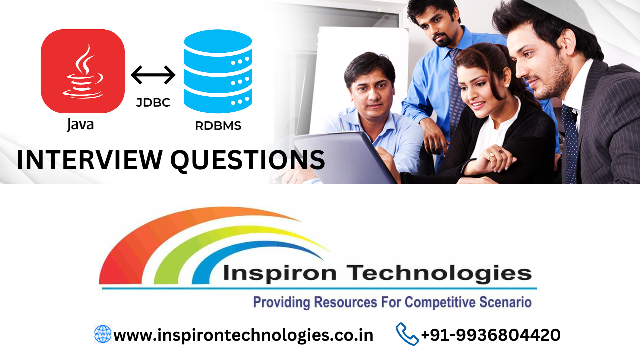
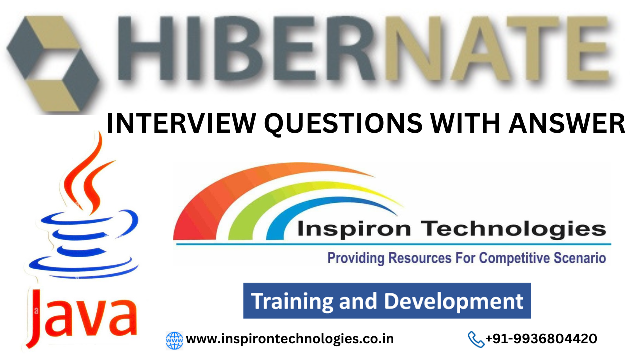
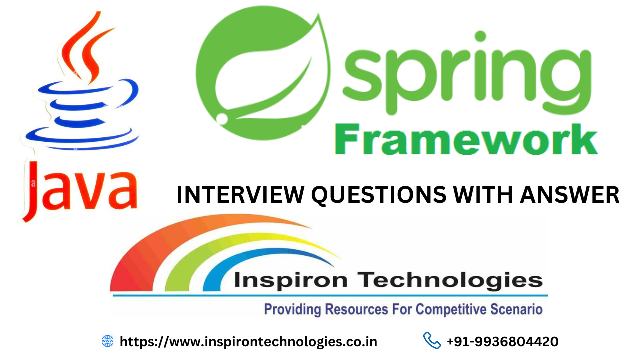
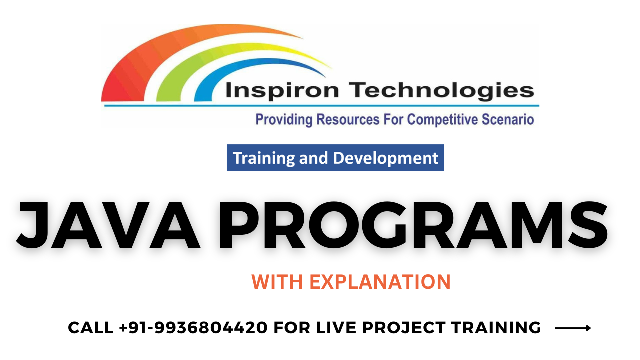
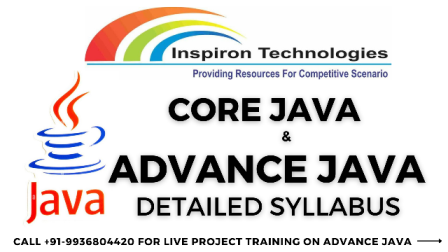
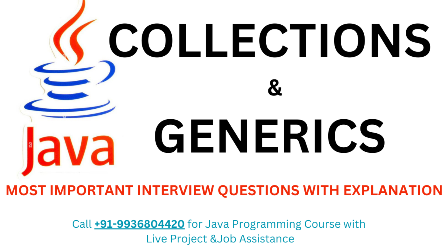
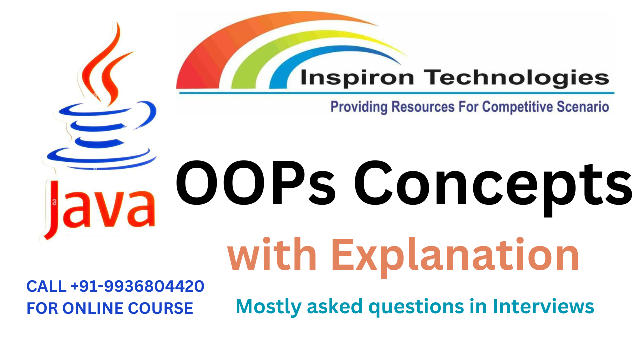
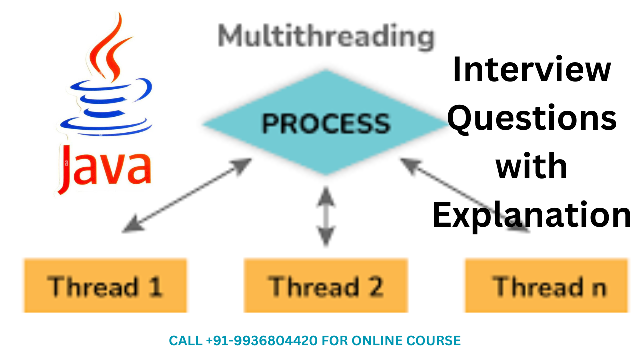
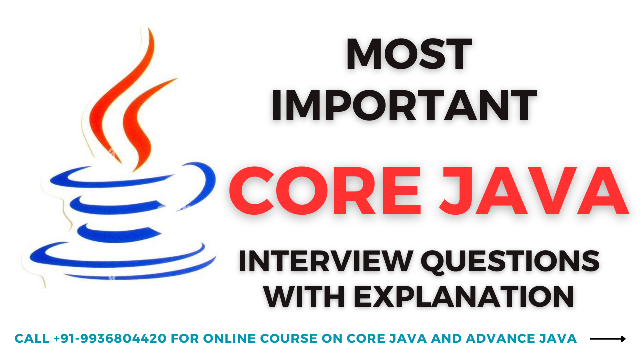
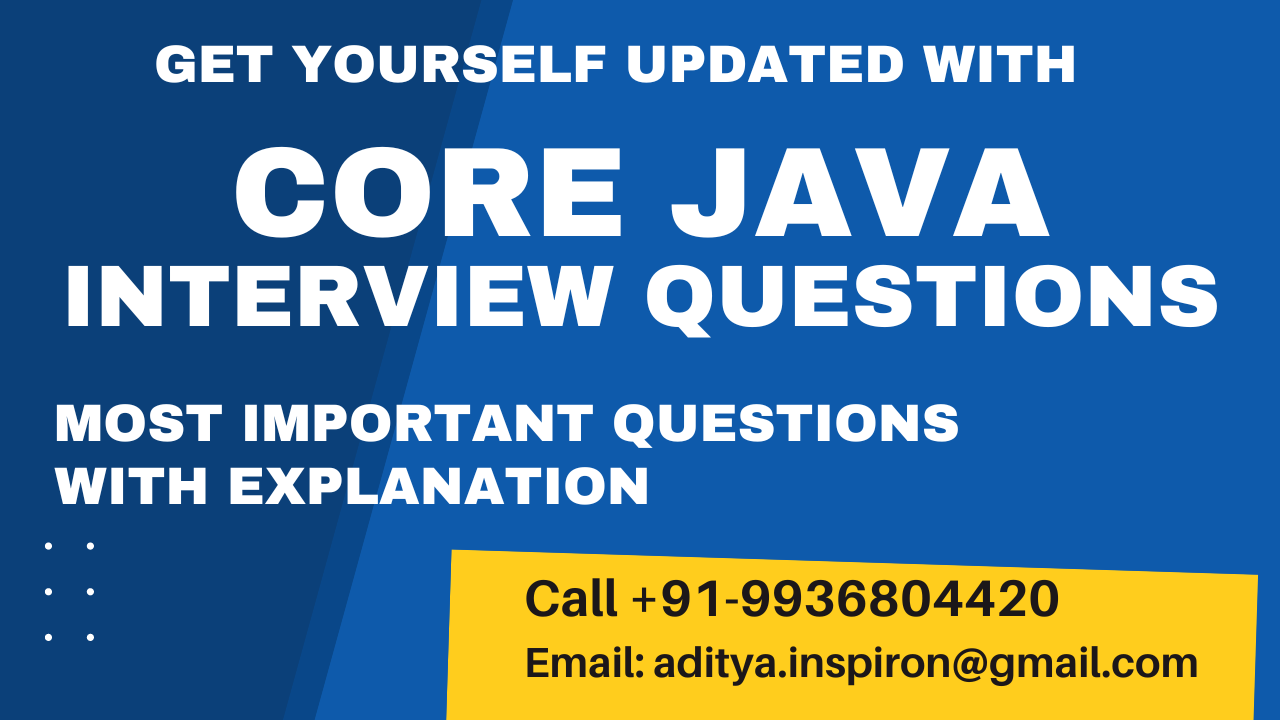
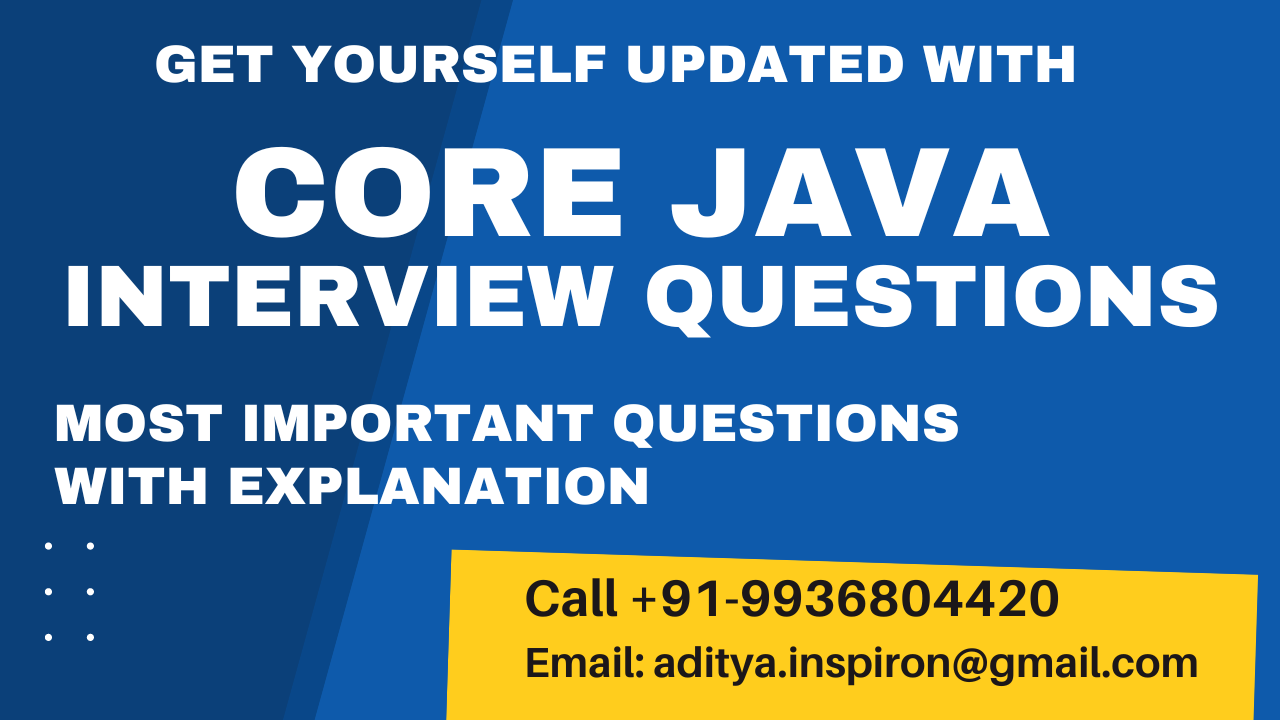
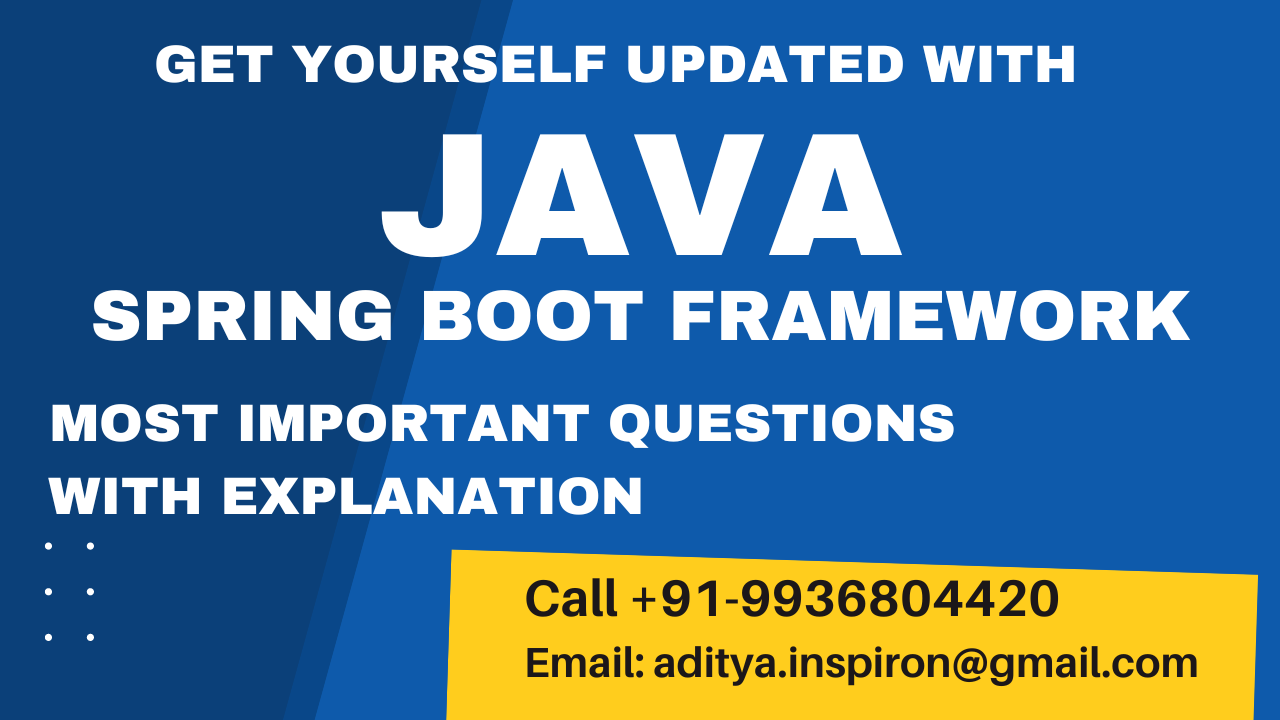
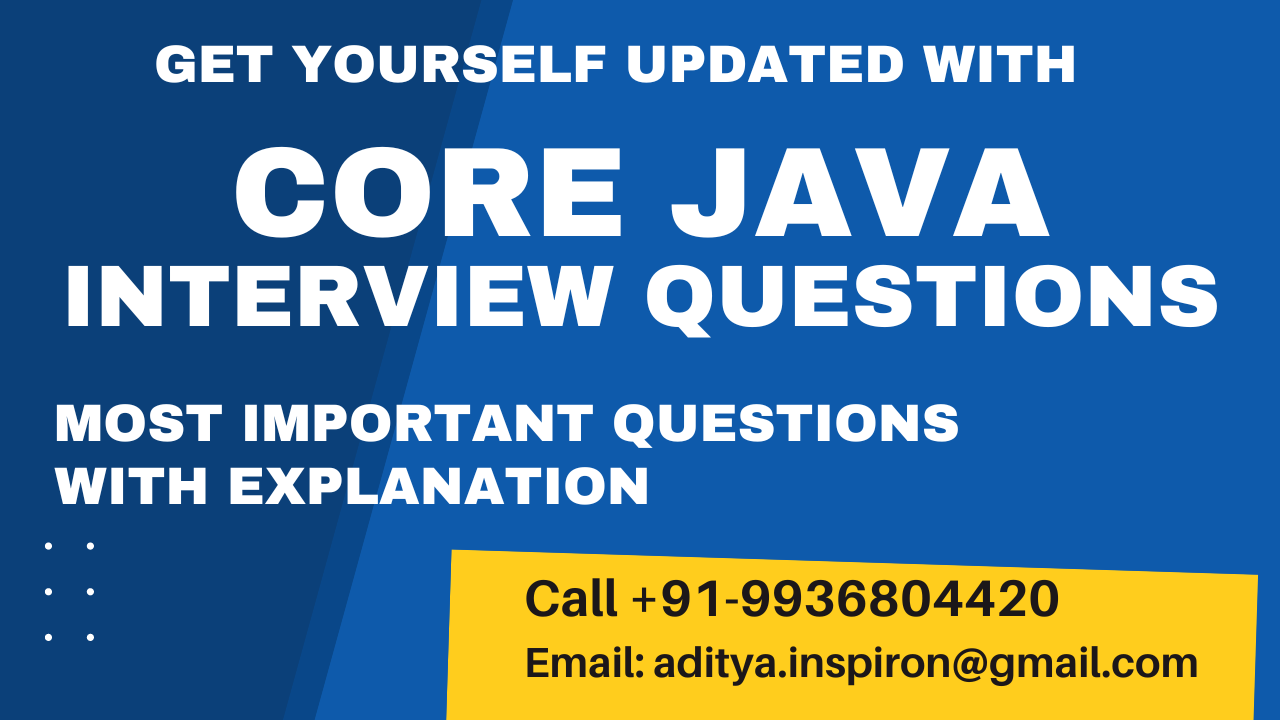
Categories
Popular Post
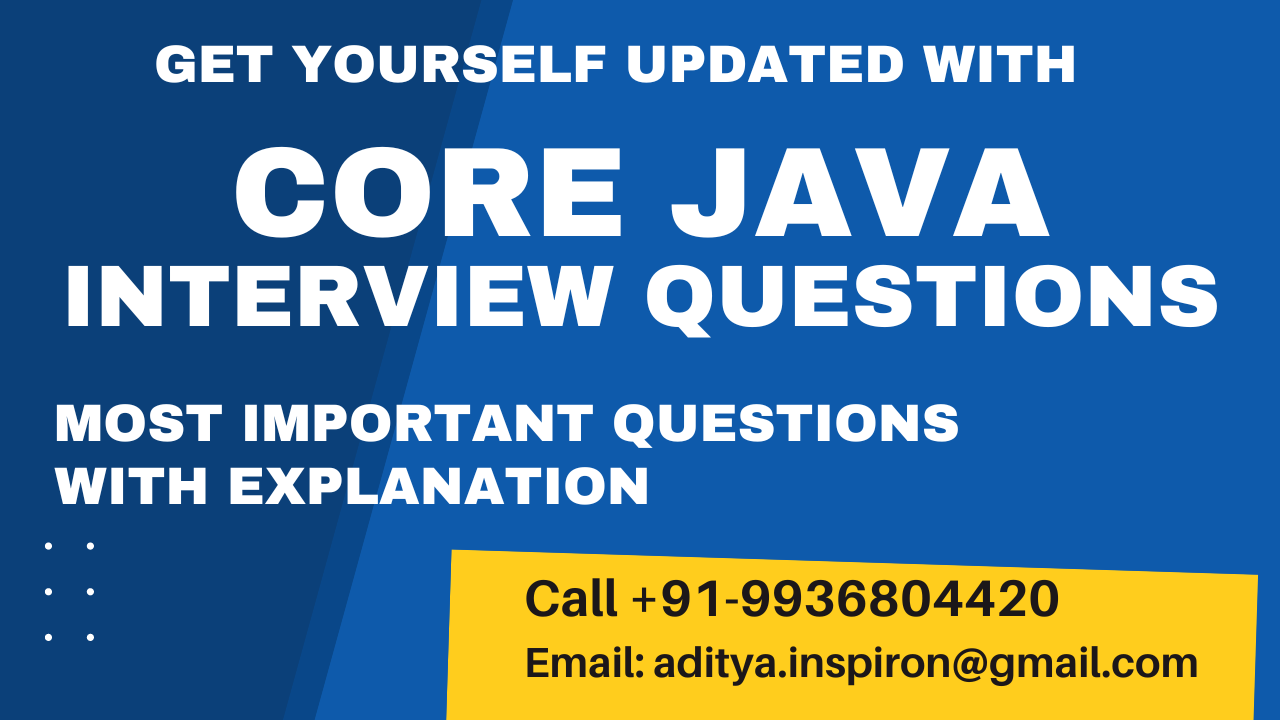
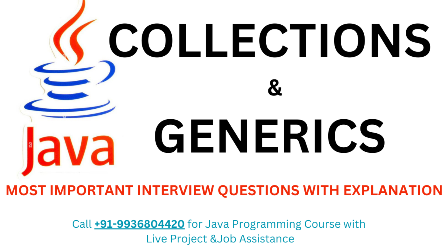
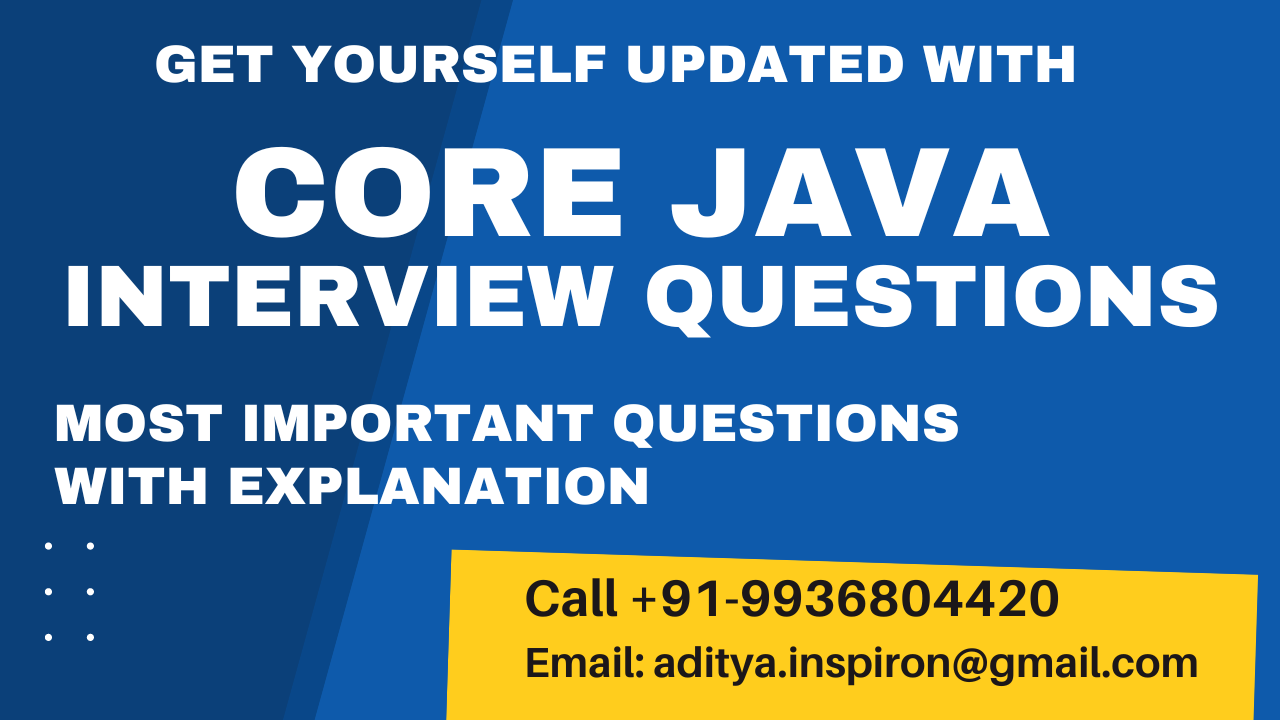
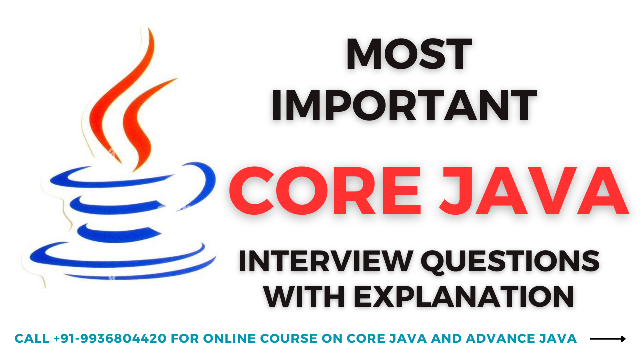
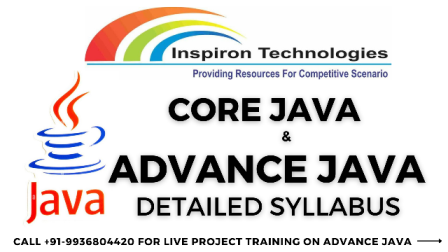
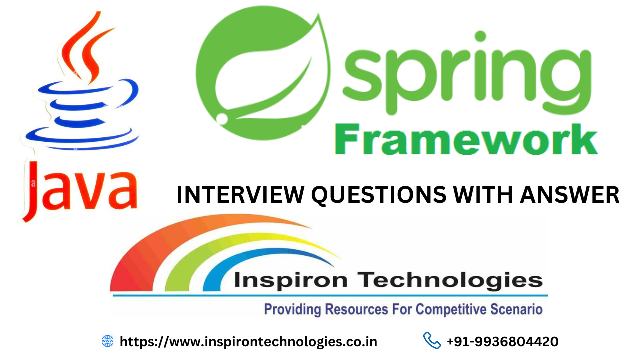
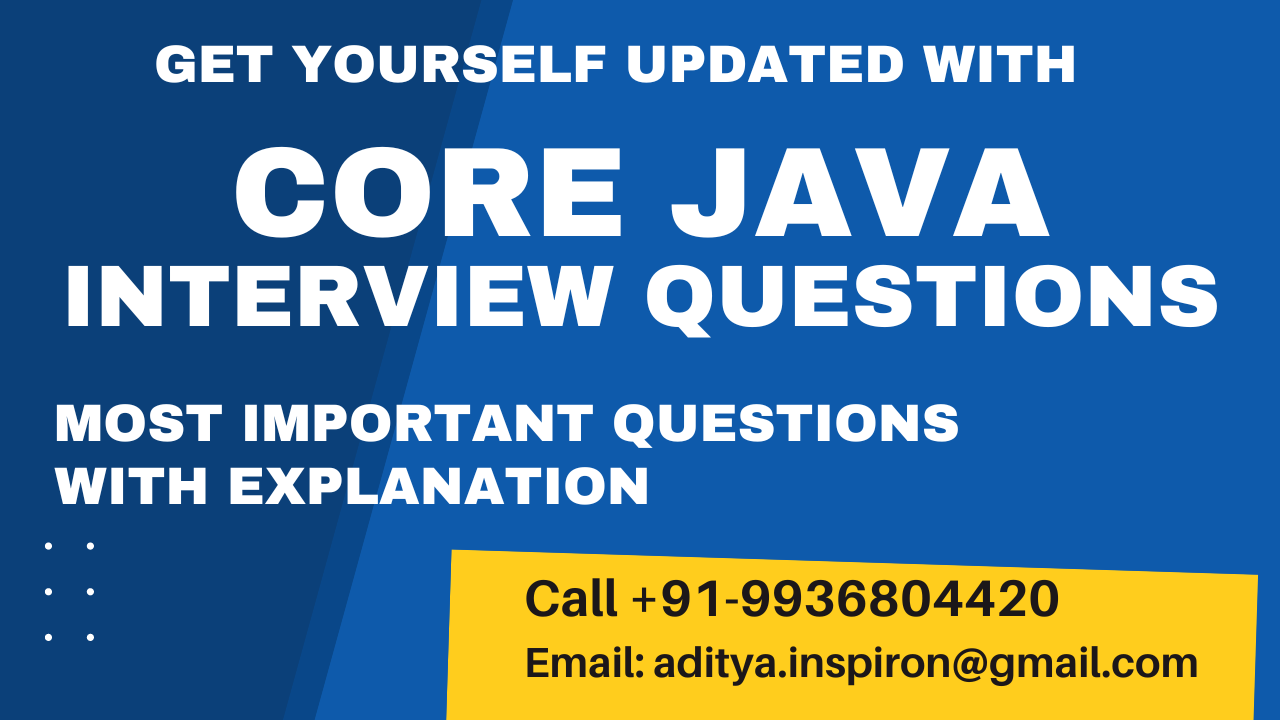
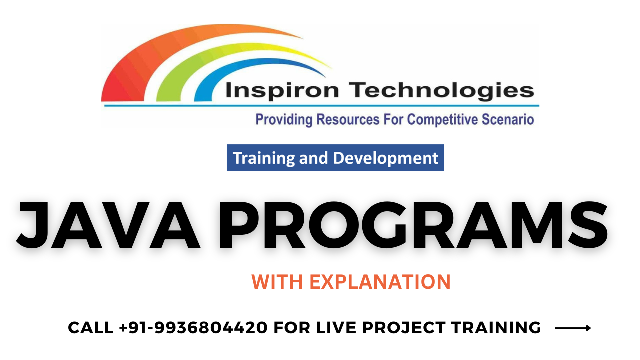
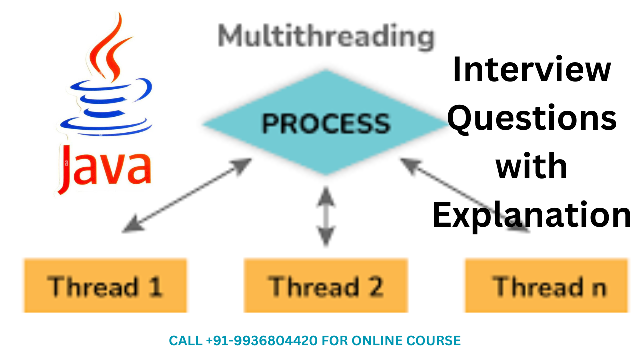
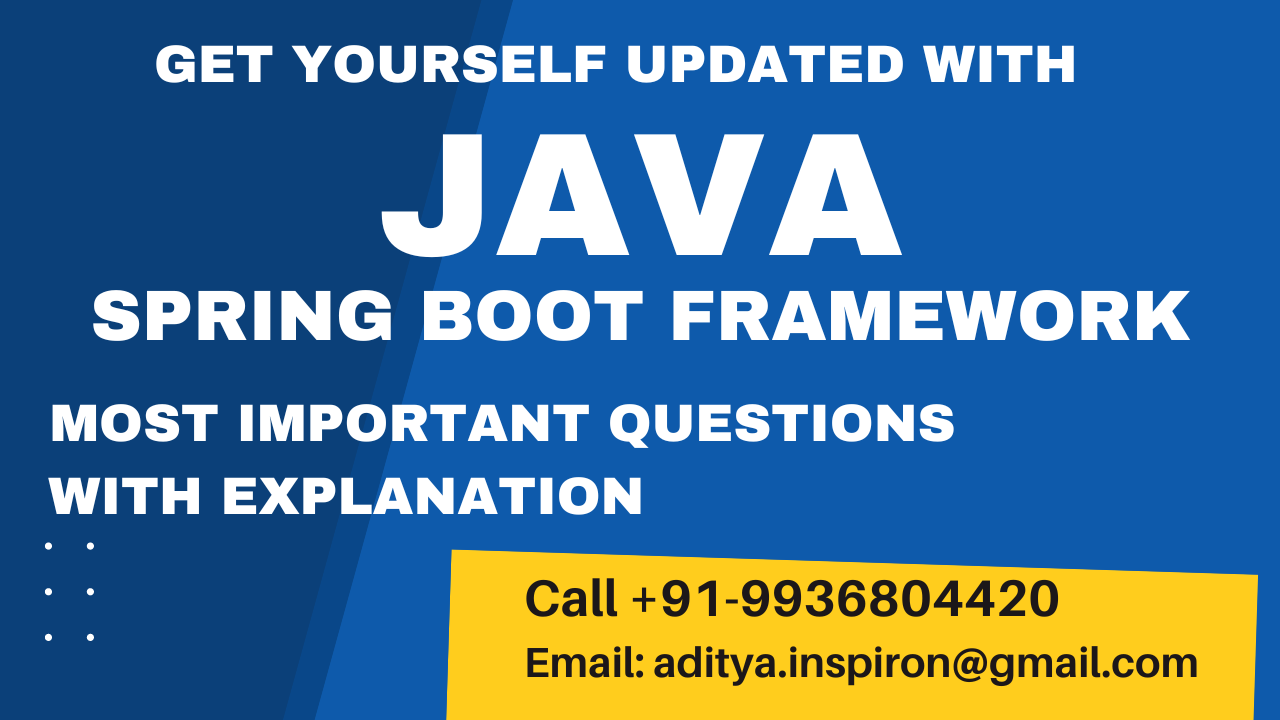
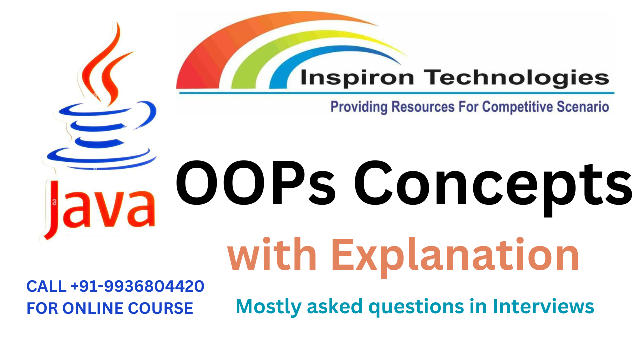
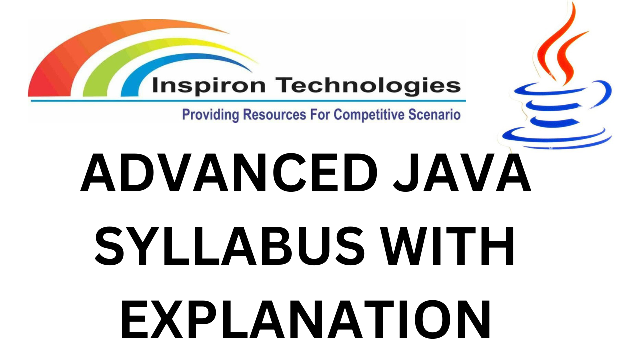
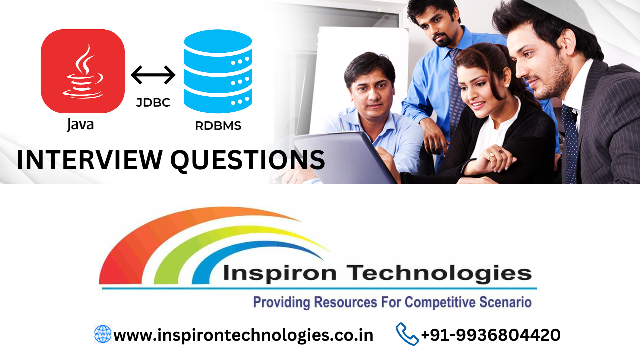
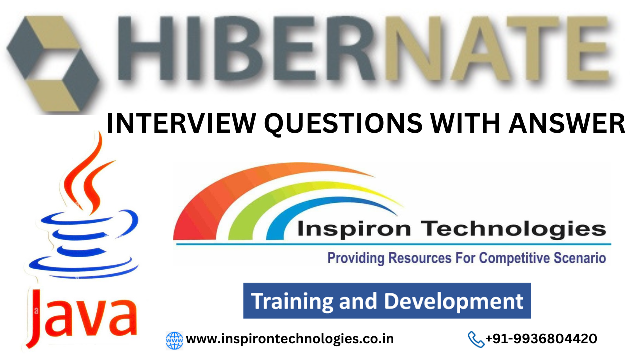
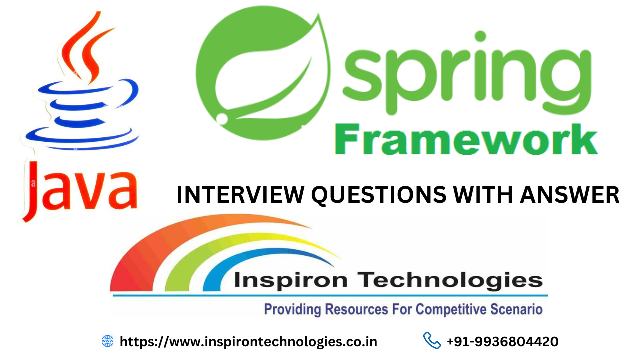
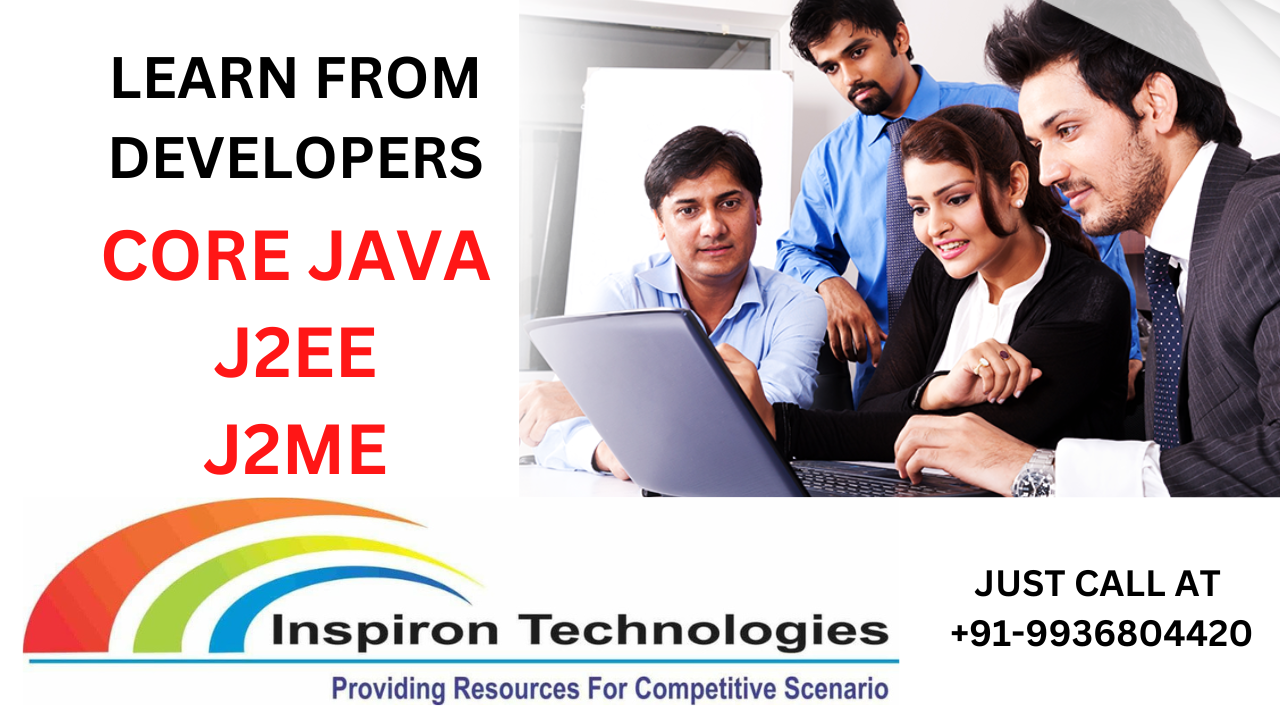
Leave a comment