Top 40 Java Collections and Generics Interview Questions
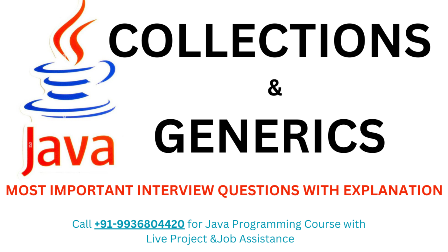
Java Collection and Generics Interview Questions with Explanation
1. What is the purpose of the Java Collection framework?
A. To provide a standard set of interfaces and implementations for storing and manipulating collections of objects.
B. To provide a standard set of interfaces and implementations for manipulating the file system.
C. To provide a standard set of interfaces and implementations for networking in Java.
D. To provide a standard set of interfaces and implementations for multithreading in Java.
Answer: A
Explanation: The Java Collection framework provides a standard set of interfaces and implementations for storing and manipulating collections of objects.
2. What is the difference between a List and a Set in the Java Collection framework?
A. A List is ordered and allows duplicates, while a Set is unordered and does not allow duplicates.
B. A List is unordered and allows duplicates, while a Set is ordered and does not allow duplicates.
C. A List is ordered and does not allow duplicates, while a Set is unordered and allows duplicates.
D. A List is unordered and does not allow duplicates, while a Set is ordered and allows duplicates.
Answer: A
Explanation: A List is ordered and allows duplicates, while a Set is unordered and does not allow duplicates.
3. What is the purpose of the Java Generics framework?
A. To provide a way to declare type parameters for classes, interfaces, and methods.
B. To provide a way to declare instance variables for classes and interfaces.
C. To provide a way to declare constants for classes and interfaces.
D. To provide a way to declare static methods for classes and interfaces.
Answer: A
Explanation: The Java Generics framework provides a way to declare type parameters for classes, interfaces, and methods.
4. What is a wildcard in Java Generics?
A. A type argument that can be any type.
B. A type argument that can be any subtype of a specified type.
C. A type argument that can be any supertype of a specified type.
D. A type argument that can be any type except for a specified type.
Answer: A
Explanation: A wildcard in Java Generics is a type argument that can be any type.
5. What is the difference between ArrayList and LinkedList in the Java Collection framework?
A. ArrayList is a resizable array implementation, while LinkedList is a doubly-linked list implementation.
B. ArrayList is a doubly-linked list implementation, while LinkedList is a resizable array implementation.
C. ArrayList is a singly-linked list implementation, while LinkedList is a resizable array implementation.
D. ArrayList is a resizable array implementation, while LinkedList is a singly-linked list implementation.
Answer: A
Explanation: ArrayList is a resizable array implementation, while LinkedList is a doubly-linked list implementation.
6. What is the difference between List and Set in Java?
Answer: List and Set are two types of Collection interfaces in Java. List is an ordered collection that allows duplicates, while Set is an unordered collection that does not allow duplicates.
Explanation: The List interface extends the Collection interface and represents an ordered collection of elements. List allows duplicate elements and elements can be inserted or removed at any position in the list. Some of the commonly used implementations of List interface are ArrayList, LinkedList, and Vector.
The Set interface, on the other hand, also extends the Collection interface, but it does not allow duplicate elements. Set is an unordered collection, which means that the order of elements is not guaranteed to be maintained. Some of the commonly used implementations of Set interface are HashSet, TreeSet, and LinkedHashSet.
7. What is the difference between ArrayList and LinkedList in Java?
Answer: ArrayList and LinkedList are two implementations of the List interface in Java. The main difference between them is in the way they store and retrieve elements.
Explanation: ArrayList stores elements in a contiguous block of memory, and each element can be accessed directly using an index. Retrieving an element at a specific index is a constant-time operation, but inserting or removing an element at the beginning or middle of the list is a slow operation as all elements need to be shifted to accommodate the change.
LinkedList, on the other hand, stores elements as a list of nodes, where each node contains a reference to the previous and next nodes in the list. Inserting or removing an element in the middle of the list is a fast operation as only the references need to be updated, but retrieving an element at a specific index is a slow operation as the list needs to be traversed from the beginning.
8. What is the purpose of the Java Collection framework?
Answer: The Java Collection framework provides a set of interfaces, classes, and algorithms to manage a group of objects, i.e., a collection of objects.
Explanation: The main purpose of the Java Collection framework is to provide a standardized and reusable way of managing a group of objects. It includes a set of interfaces such as List, Set, Map, Queue, etc., which define the behavior of a collection of objects. The framework also includes concrete implementations of these interfaces such as ArrayList, HashSet, HashMap, PriorityQueue, etc. that provide a specific implementation of the behavior defined by the interface.
The Collection framework provides a wide range of algorithms and operations to manipulate collections such as sorting, searching, filtering, and iteration. The framework is also designed to be extensible, which means that new types of collections can be easily added to the framework.
9. What is the purpose of Generics in Java?
Answer: Generics in Java provide a way to write code that can work with different types of objects, while providing compile-time type safety.
Explanation: Generics in Java allow us to write code that can work with different types of objects. By using Generics, we can specify the type of objects that a method or class can work with. This provides compile-time type safety, which means that the compiler can detect and report type mismatches at compile-time rather than at runtime.
For example, consider the following method without Generics:
arduino
Copy code
public static int findIndex(String[] arr, String value) {
for (int i = 0; i < arr.length; i++) {
if (arr[i].equals(value)) {
return i;
}
}
return -1;
}
This method can only work with arrays of Strings. If we want to find the index of an element in an array of integers, we need to write a separate method.
10. What is the purpose of Java Collections Framework?
a) To provide a uniform and efficient interface for collections of objects.
b) To provide a mechanism for storing objects in a database.
c) To provide a way to read and write objects to a file.
d) To provide a way to serialize objects.
Answer: a) To provide a uniform and efficient interface for collections of objects.
Explanation: Java Collections Framework provides a set of interfaces and classes to store and manipulate groups of objects. It provides a uniform and efficient interface for collections of objects, making it easier to write code that can work with different types of collections.
11. Which collection class provides a dynamic array implementation of the List interface?
a) ArrayList
b) LinkedList
c) HashSet
d) TreeMap
Answer: a) ArrayList
Explanation: ArrayList provides a dynamic array implementation of the List interface, which means that it allows for the addition and removal of elements from any position in the array.
12. What is the difference between ArrayList and LinkedList in Java?
a) ArrayList is implemented as a linked list, while LinkedList is implemented as an array.
b) ArrayList is faster for accessing elements at a specific index, while LinkedList is faster for inserting or removing elements from the middle of the list.
c) ArrayList is thread-safe, while LinkedList is not.
d) There is no difference between ArrayList and LinkedList in Java.
Answer: b) ArrayList is faster for accessing elements at a specific index, while LinkedList is faster for inserting or removing elements from the middle of the list.
Explanation: ArrayList is implemented as a dynamic array, which means that elements are stored in a contiguous block of memory. This makes it faster to access elements at a specific index. However, inserting or removing elements from the middle of the list requires shifting all the subsequent elements to make room or fill the gap, which can be slow for large lists. LinkedList, on the other hand, is implemented as a linked list of nodes, which means that elements are stored in non-contiguous blocks of memory. This makes it faster to insert or remove elements from the middle of the list, but slower to access elements at a specific index.
13. Which collection class guarantees the insertion order of its elements?
a) HashSet
b) TreeMap
c) LinkedHashMap
d) TreeSet
Answer: c) LinkedHashMap
Explanation: LinkedHashMap is a subclass of HashMap that maintains the insertion order of its elements. It stores the elements in a doubly-linked list, which allows for efficient iteration in insertion order.
14. What is the purpose of the Comparator interface in Java?
a) To compare two objects for equality.
b) To sort elements in a collection based on a specific order.
c) To convert a collection to an array.
d) To serialize a collection of objects.
Answer: b) To sort elements in a collection based on a specific order.
Explanation: The Comparator interface in Java provides a way to compare two objects for sorting purposes. It defines a compare() method that takes two objects as arguments and returns an integer value to indicate their order. By implementing this interface, you can specify a custom order for the elements in a collection.
15. What is a Collection in Java?
A Collection is a group of related objects (elements) that are stored and manipulated together. It is a framework provided by Java to handle groups of objects.
16. What are the types of Collections in Java?
The types of Collections in Java are List, Set, and Map.
17. What is the difference between List and Set in Java?
List is an ordered collection of elements that allows duplicates. Set is an unordered collection of unique elements.
18. What is an Iterator in Java?
Iterator is an interface that provides methods to iterate over a collection. It allows you to traverse the collection, access its elements, and remove them.
19. What is the difference between an Iterator and ListIterator in Java?
Iterator is used to iterate over a collection in a forward direction, whereas ListIterator is used to iterate over a list in both directions.
20. What is the difference between a Vector and an ArrayList in Java?
Both Vector and ArrayList are used to store objects dynamically. However, Vector is synchronized and ArrayList is not.
21. What is a LinkedList in Java?
LinkedList is a class that implements the List interface. It provides a doubly-linked list implementation of the List interface.
22. What is a Stack in Java?
Stack is a class that extends Vector and provides methods to perform stack operations (push and pop).
23. What is a Queue in Java?
Queue is an interface that defines methods for adding and removing elements in a FIFO (First-In-First-Out) manner.
24. What is the difference between a Queue and a Stack in Java?
A Queue is a data structure that follows a FIFO (First-In-First-Out) order of elements, whereas a Stack is a data structure that follows a LIFO (Last-In-First-Out) order of elements.
25. What is a HashMap in Java?
HashMap is a class that implements the Map interface. It provides a hash table implementation of the Map interface.
26. What is a TreeMap in Java?
TreeMap is a class that implements the SortedMap interface. It provides a red-black tree implementation of the Map interface.
27. What is a LinkedHashMap in Java?
LinkedHashMap is a class that extends HashMap and maintains the insertion order of elements.
28. What is a HashSet in Java?
HashSet is a class that implements the Set interface. It provides a hash table implementation of the Set interface.
29. What is a TreeSet in Java?
TreeSet is a class that implements the SortedSet interface. It provides a red-black tree implementation of the Set interface.
30. What is the difference between a HashMap and a TreeMap in Java?
HashMap is an unordered collection that uses a hash table to store key-value pairs, whereas TreeMap is an ordered collection that uses a red-black tree to store key-value pairs.
31. What is the difference between a HashSet and a TreeSet in Java?
HashSet is an unordered collection that uses a hash table to store unique elements, whereas TreeSet is an ordered collection that uses a red-black tree to store unique elements.
32. What is the difference between Comparable and Comparator in Java?
Comparable is an interface that defines a single method compareTo() that is used to compare objects of the same class. Comparator is an interface that defines a single method compare() that is used to compare objects of different classes.
33. What is Generics in Java?
Generics is a way of providing type safety for collections. It allows you to specify the type of objects that a collection can contain.
34. What is the syntax for Generics in Java?
The syntax for Generics in Java is as follows:
class ClassName<T> {
T variableName;
T methodName(T parameterName) {
// method implementation
}
}
In the above syntax, <T> indicates the use of a generic type parameter, which can be replaced with any valid identifier. The parameter can be used in the class definition, variable declaration, and method signature to ensure type safety and flexibility.
35. What is the purpose of the Java Collection Framework?
Answer: The Java Collection Framework provides a set of reusable data structures and algorithms for storing, manipulating, and accessing collections of objects. It helps in improving code quality, reducing development time, and increasing performance.
36. What is a generic type in Java?
Answer: A generic type in Java is a type that is parameterized over one or more other types. It allows the creation of classes, interfaces, and methods that can work with different types of objects, without sacrificing type safety.
37. How do you define a generic class in Java?
Answer: To define a generic class in Java, you need to use the following syntax:
class ClassName<T> {
// class definition
}
Here, <T> is the type parameter that can be replaced with any valid identifier.
38. What is the difference between an ArrayList and a LinkedList?
Answer: An ArrayList is implemented as a resizable array, whereas a LinkedList is implemented as a linked list of nodes. An ArrayList provides fast random access to its elements, while a LinkedList provides fast insertion and deletion of elements in the middle of the list.
39. What is a wildcard in Java generics?
Answer: A wildcard in Java generics is a special syntax that allows the creation of generic classes, interfaces, and methods that can work with different types of objects. The syntax for a wildcard is ?. It can be used to specify an unknown type or to define a bounded type parameter.
40. What is the purpose of the Java Collection interface?
Answer: The Java Collection interface is the root interface in the Java Collection Framework. It defines the basic operations that can be performed on a collection, such as adding, removing, and iterating over elements. It is implemented by all the standard collection classes in Java.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Core Java and Advance Java, Power BI, Tableau, AI, IOT, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com. Happy Learning
Team Inspiron Technologies
People also read
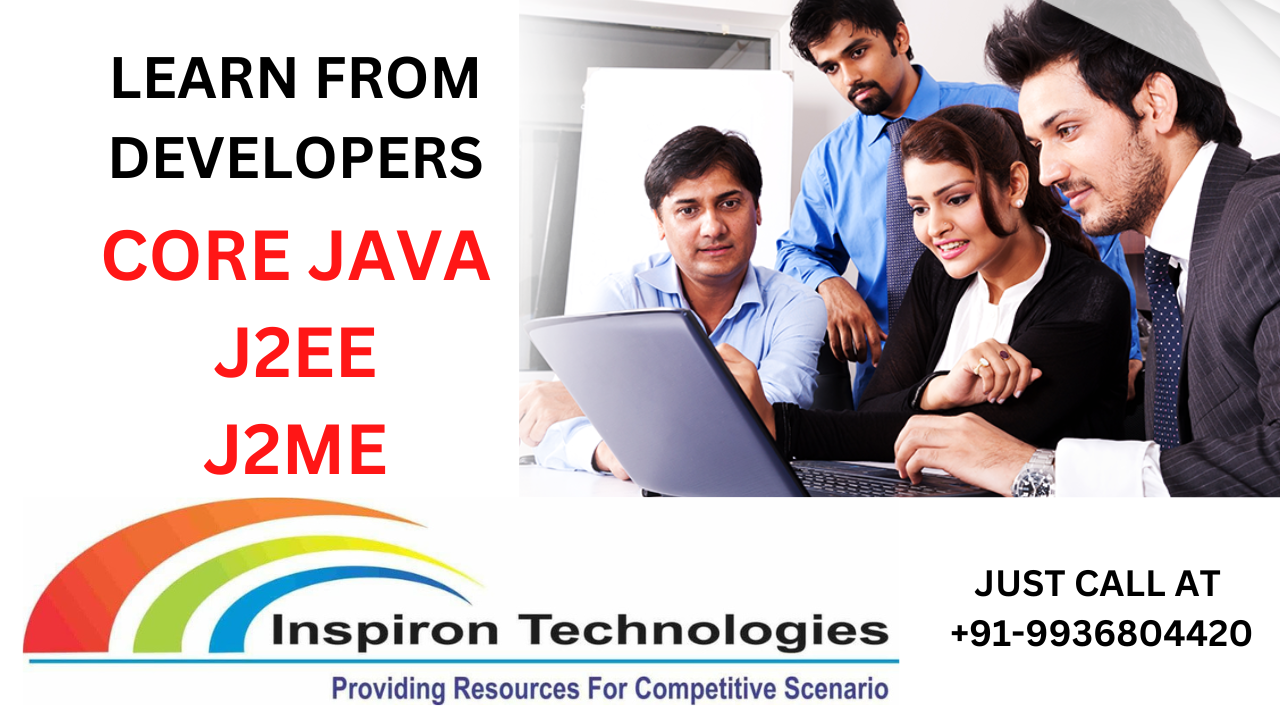
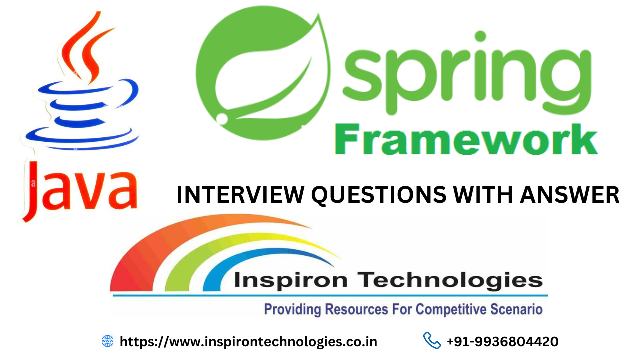
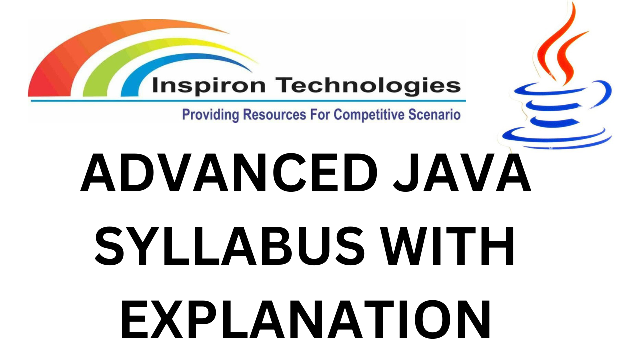
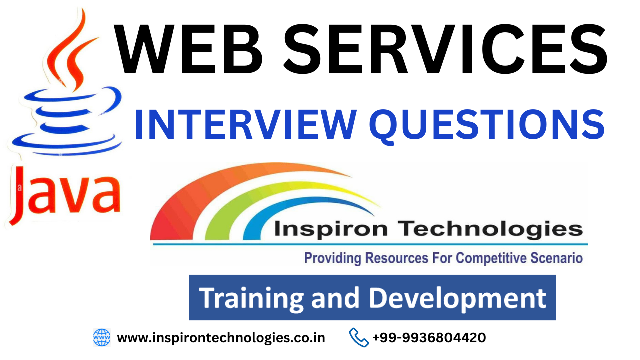
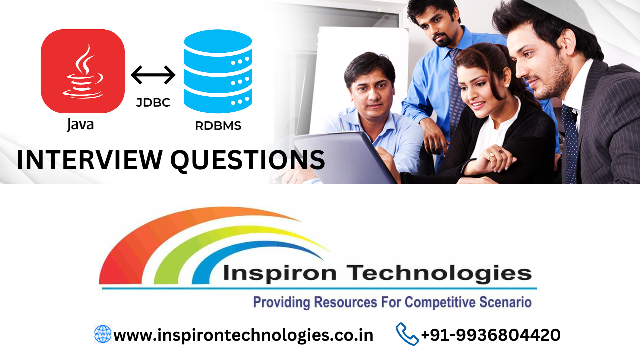
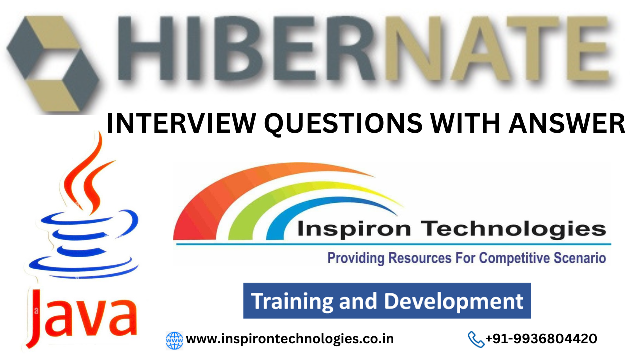
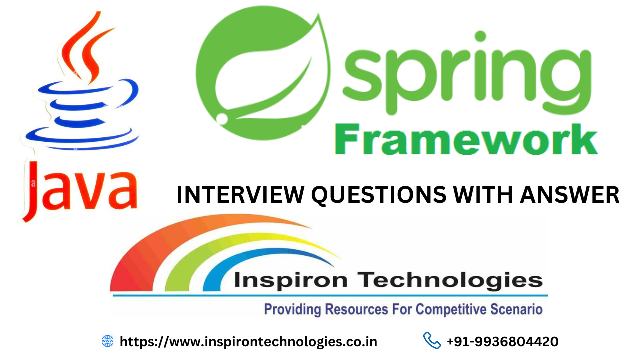
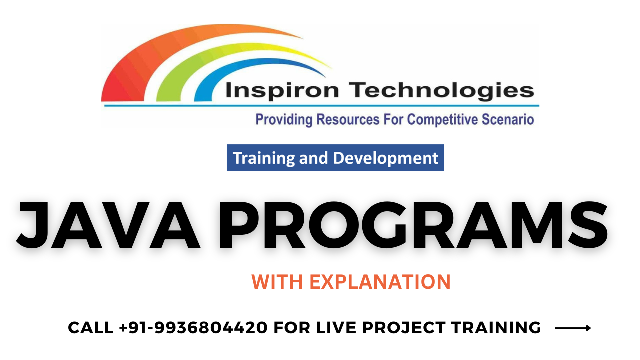
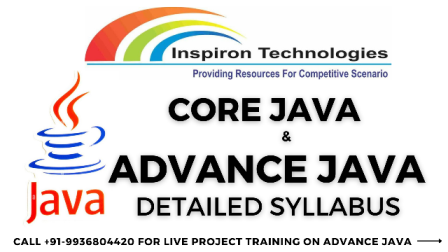
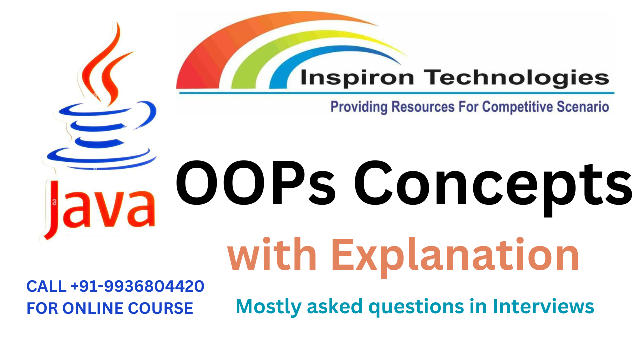
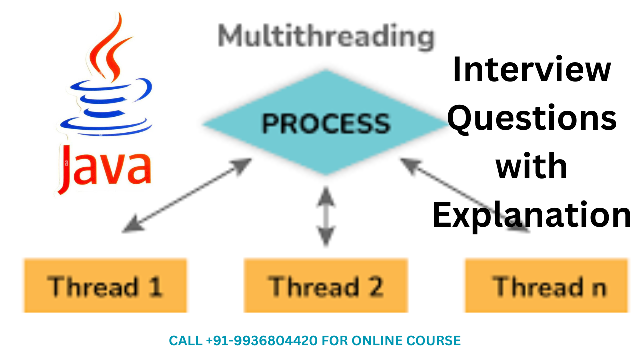
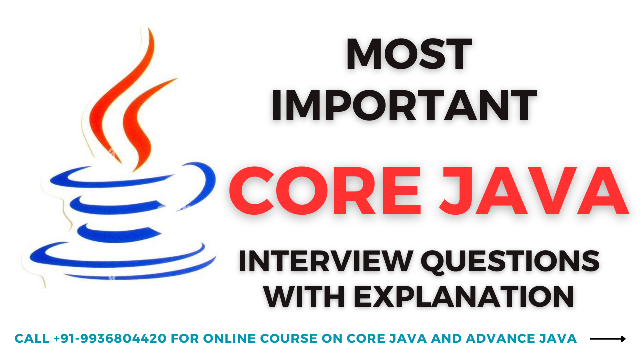
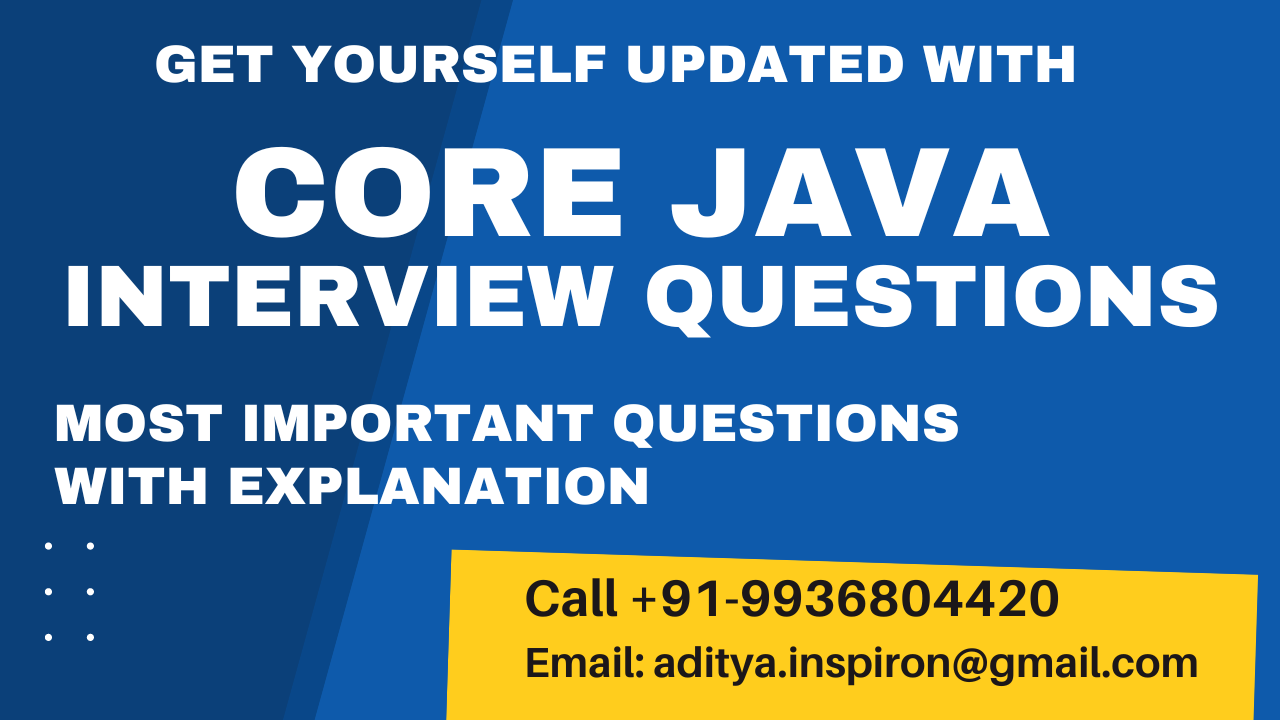
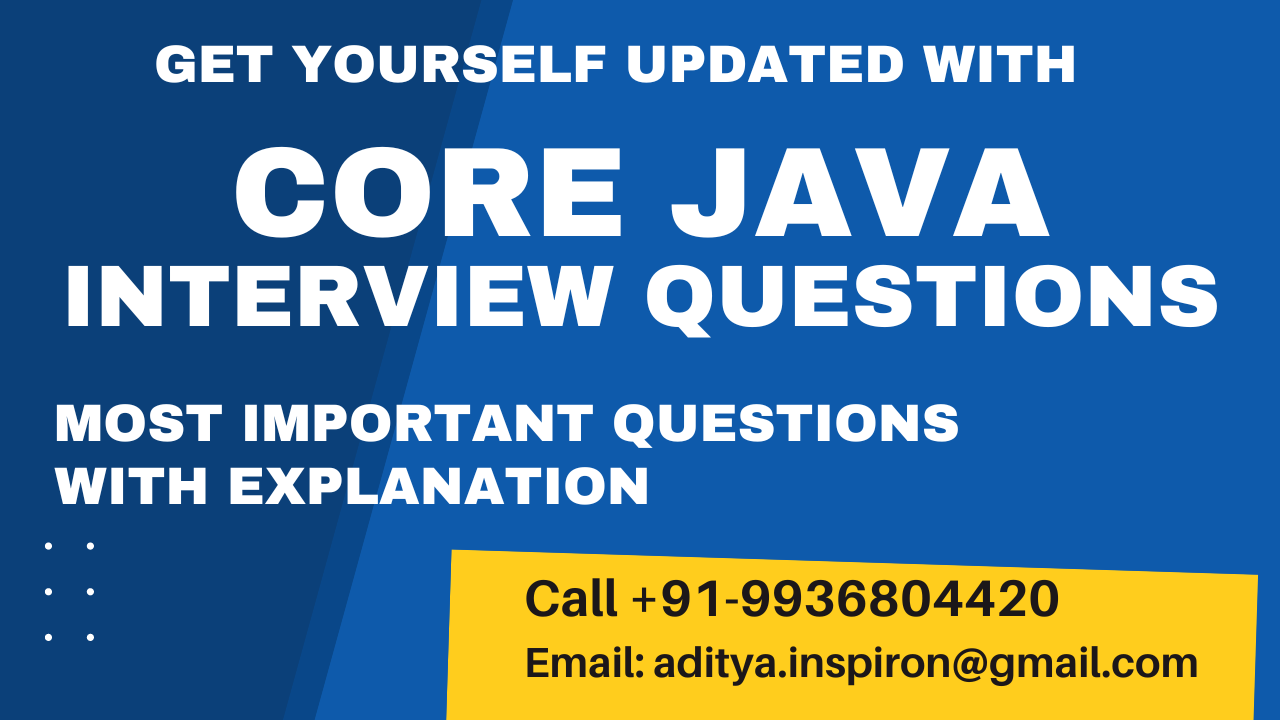
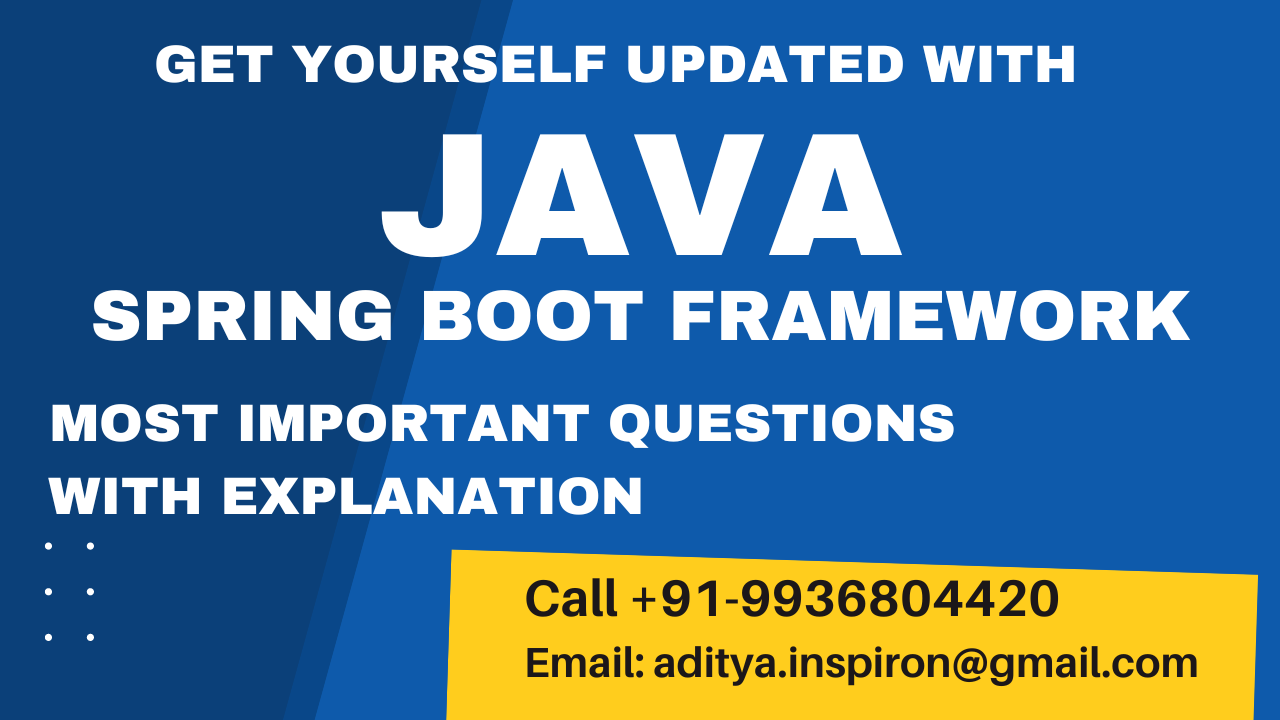
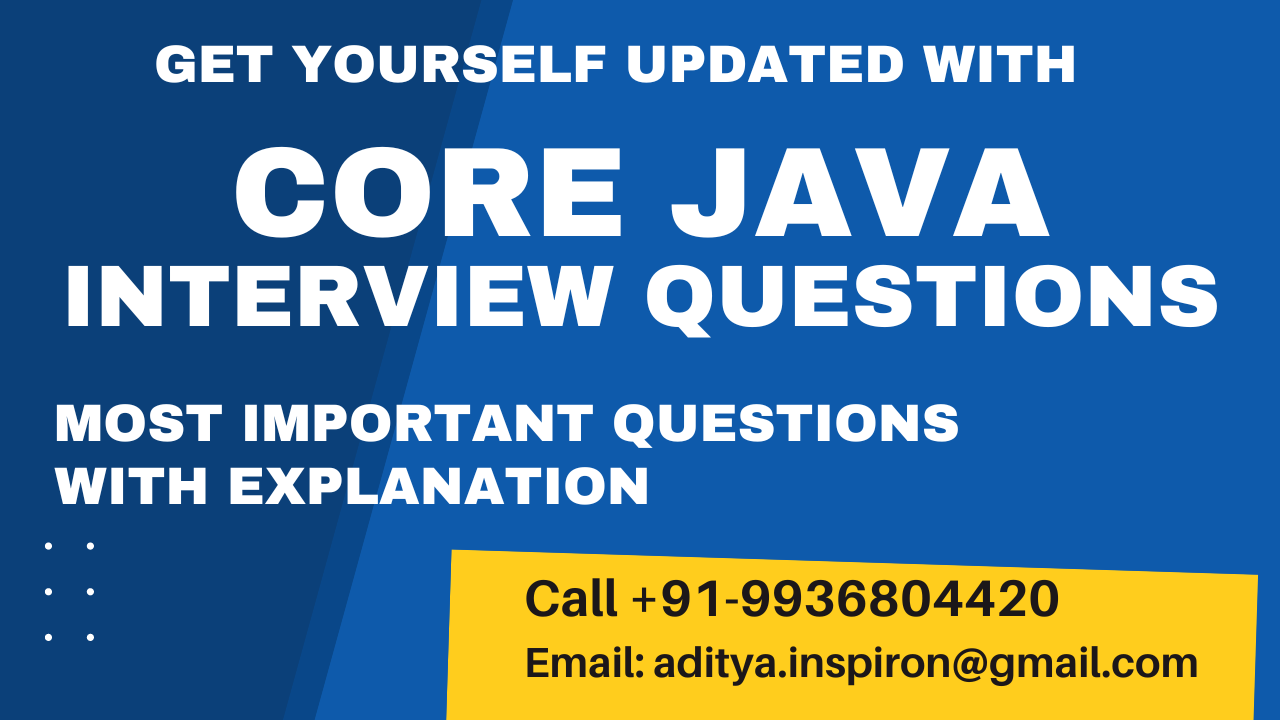
Categories
Popular Post
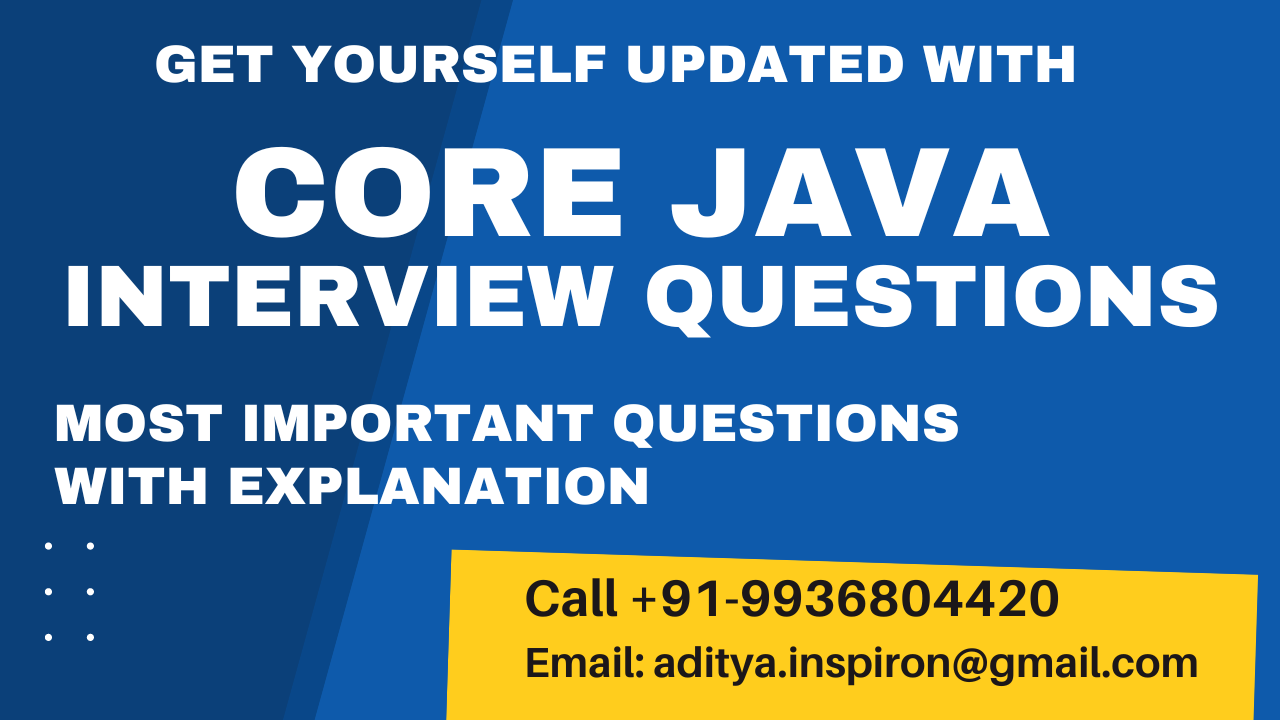
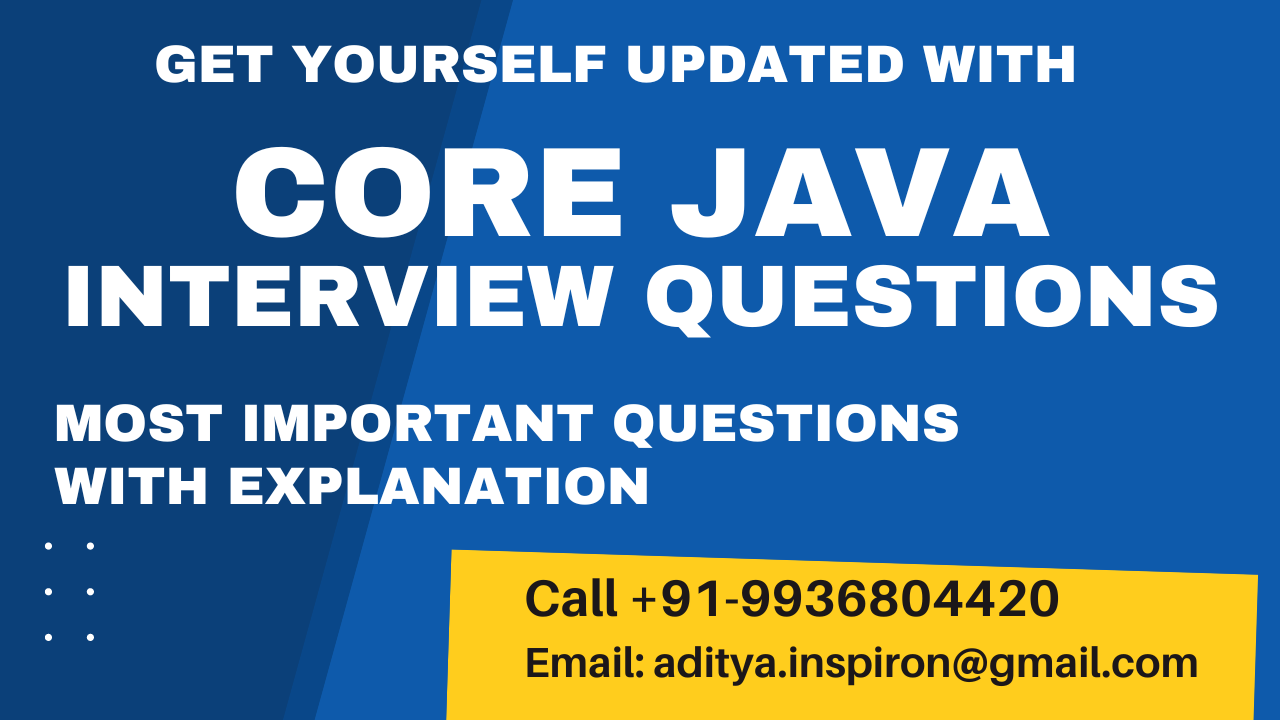
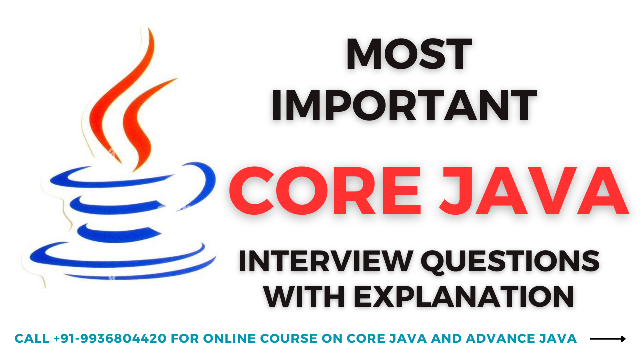
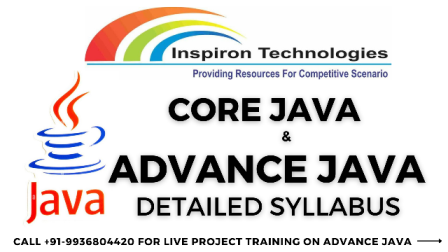
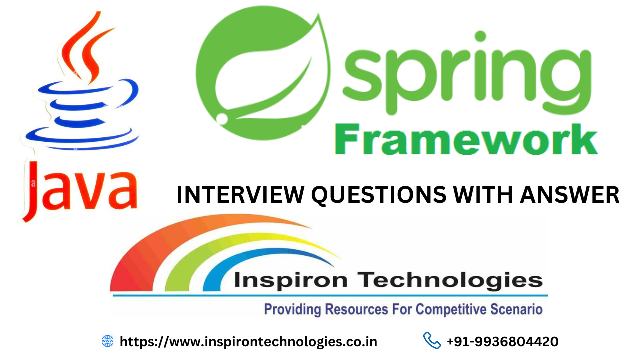
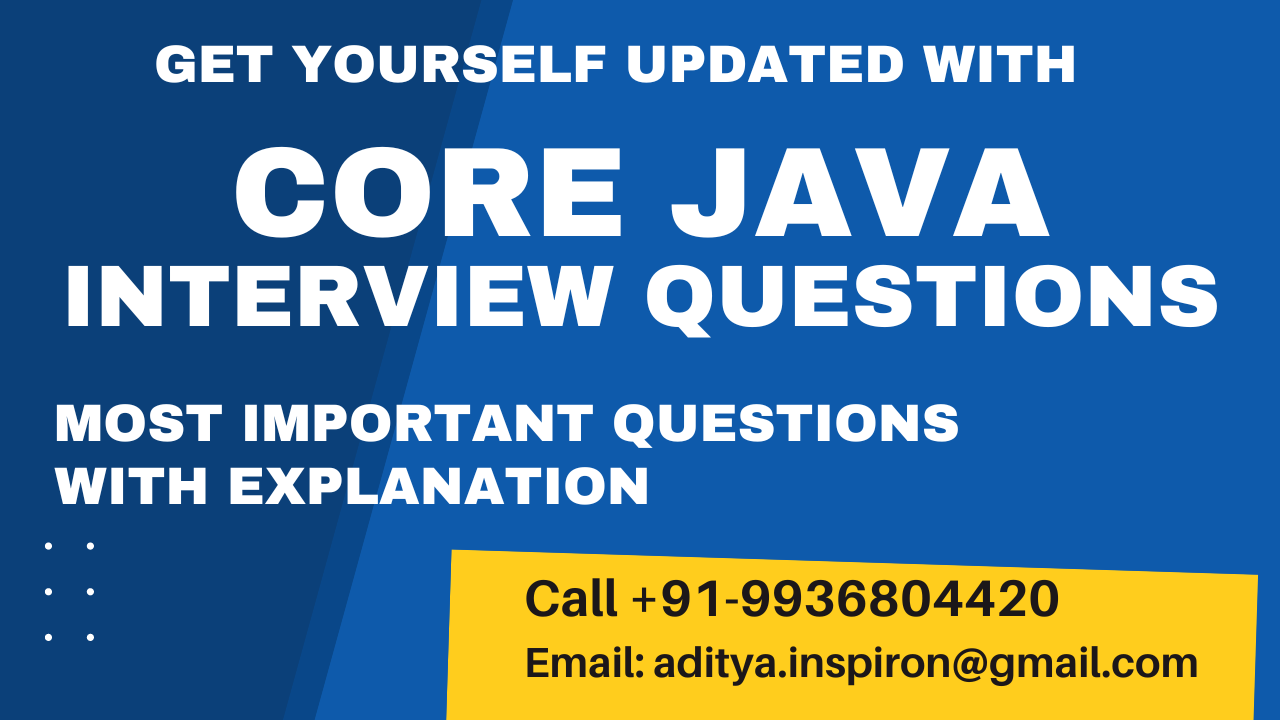
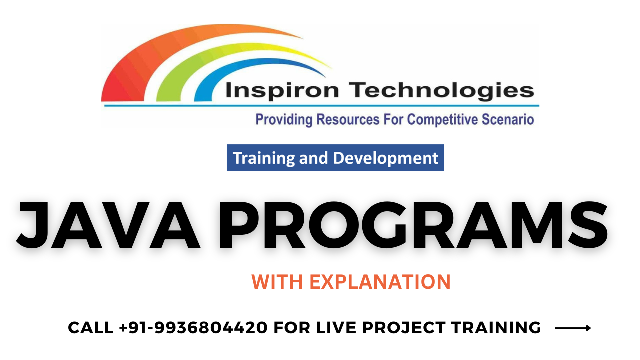
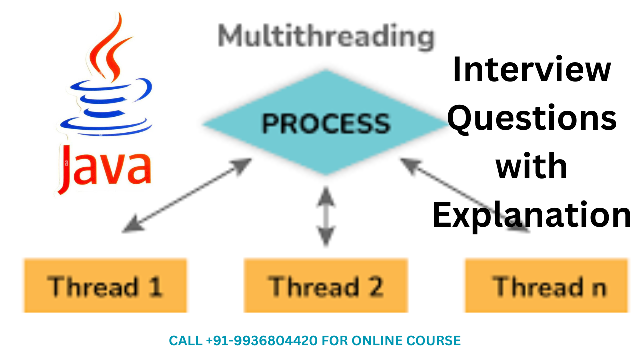
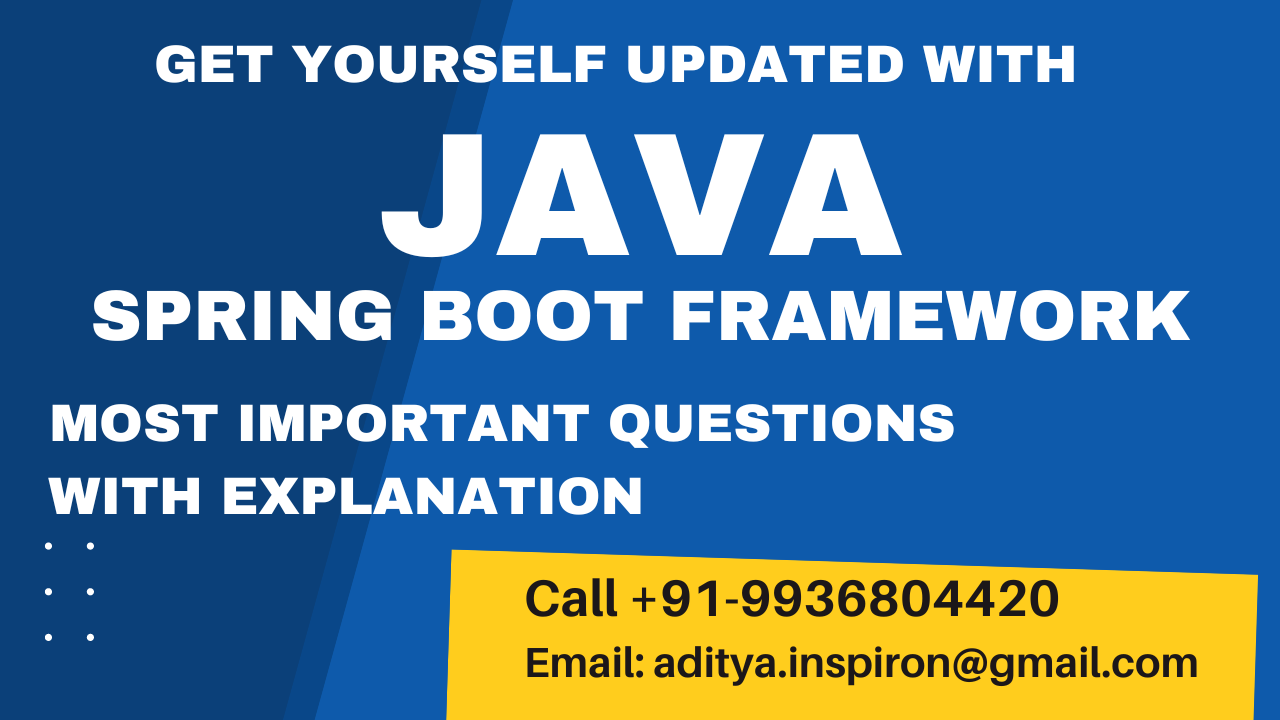
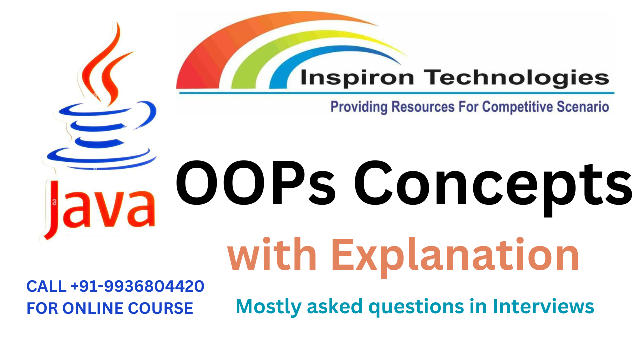
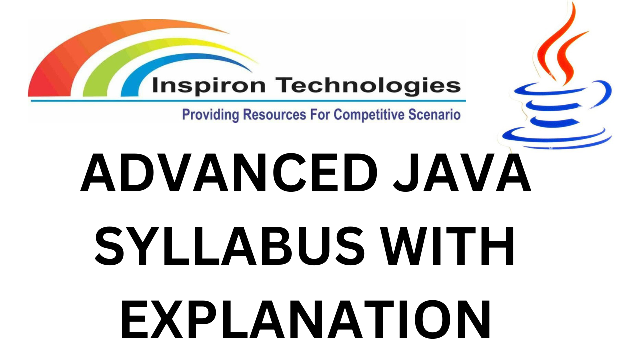
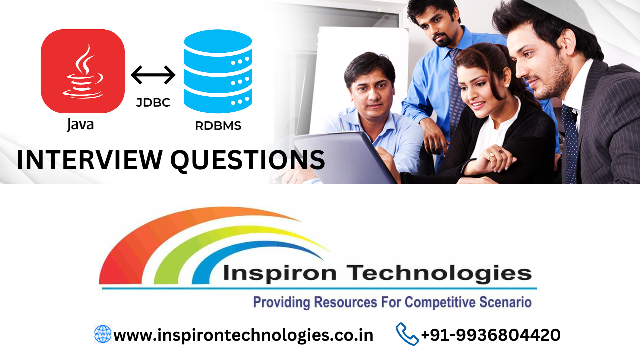
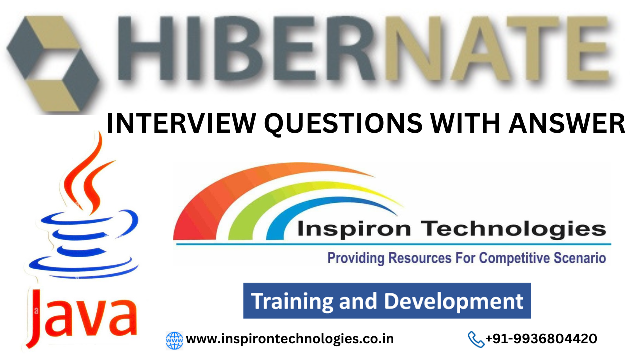
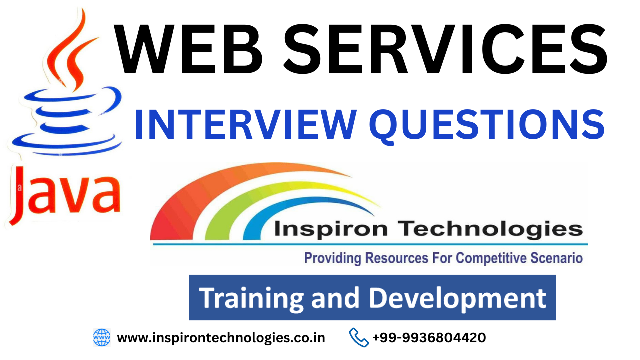
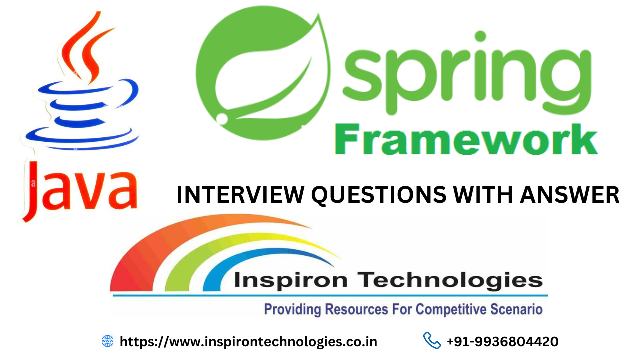
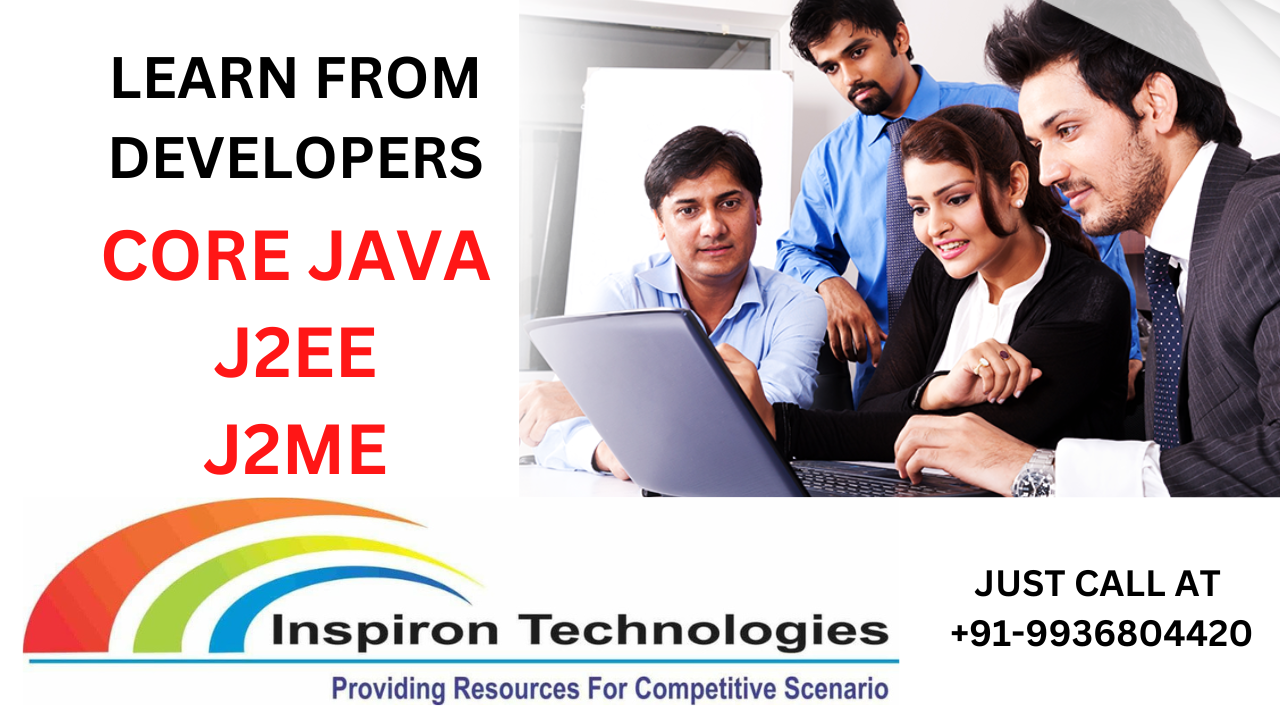
Leave a comment